Comparing version 1.5.4 to 2.0.0
{ | ||
"name": "aptoma-alf", | ||
"version": "1.5.4", | ||
"name": "alf", | ||
"version": "2.0.0", | ||
"homepage": "https://github.com/aptoma/alf-dist", | ||
@@ -17,3 +17,8 @@ "authors": [ | ||
"tests" | ||
] | ||
], | ||
"dependencies": { | ||
"backbone": "^1.2.1", | ||
"jquery": "^2.1.4", | ||
"lodash": "^3.10.1" | ||
} | ||
} |
@@ -0,1 +1,6 @@ | ||
### 2.0.0 - 2015-08-26 | ||
* NEW: Use ES6 modules internally. | ||
* NEW: UMD bundles (see installation docs for more information). | ||
* CHANGED: `require('alf')` can no longer be used without using a module loader (RequireJS, Webpack, Browserify or similar). | ||
### 1.5.4 - 2015-07-02 | ||
@@ -2,0 +7,0 @@ * NEW: Add support for `data-select-inner` to use innerHTML instead of outerHTML on the matching node. It otherwise behaves just like `data-select` |
@@ -7,2 +7,3 @@ ## Introduction | ||
* [Installation](installation) | ||
* [Glossary](glossary) | ||
@@ -9,0 +10,0 @@ * [Building grids](templates/building-grids) |
374
grid.js
@@ -1,232 +0,237 @@ | ||
;(function (window, $, undefined) { | ||
import $ from 'jquery'; | ||
/** | ||
* Grid | ||
* | ||
* Absolute positions columns and cells based on class names | ||
* | ||
* Usage: | ||
* Class names for the wrapper (this.el) | ||
* cols-x: total number of columns in the grid | ||
* | ||
* Class names for cells: | ||
* col-x: start at column x | ||
* colspan-x: span across x columns | ||
* | ||
* @author Peter Rudolfsen <peter@aptoma.com> | ||
*/ | ||
var Grid = function (options) { | ||
var styles; | ||
/** | ||
* Grid | ||
* | ||
* Absolute positions columns and cells based on class names | ||
* | ||
* Usage: | ||
* Class names for the wrapper (this.el) | ||
* cols-x: total number of columns in the grid | ||
* | ||
* Class names for cells: | ||
* col-x: start at column x | ||
* colspan-x: span across x columns | ||
* | ||
* @author Peter Rudolfsen <peter@aptoma.com> | ||
*/ | ||
var Grid = function (options) { | ||
var styles; | ||
this.options = $.extend({ | ||
prefix: '' | ||
}, options); | ||
this.options = $.extend({ | ||
prefix: '' | ||
}, options); | ||
this.el = options.el; | ||
this.el = options.el; | ||
// The total height each column has available | ||
this.height = options.height || $(this.el).height(); | ||
// The total height each column has available | ||
this.height = options.height || $(this.el).height(); | ||
// Define lineHeight if you want to align columns to a "vertical grid" | ||
this.lineHeight = options.lineHeight || 0; | ||
// Define lineHeight if you want to align columns to a "vertical grid" | ||
this.lineHeight = options.lineHeight || 0; | ||
// Make sure to respect the padding | ||
styles = window.getComputedStyle(this.el, null); | ||
this.paddingTop = parseInt(styles.getPropertyValue('padding-top'), 10); | ||
this.paddingBottom = parseInt(styles.getPropertyValue('padding-bottom'), 10); | ||
this.height -= (this.paddingTop + this.paddingBottom); | ||
// Make sure to respect the padding | ||
styles = window.getComputedStyle(this.el, null); | ||
this.paddingTop = parseInt(styles.getPropertyValue('padding-top'), 10); | ||
this.paddingBottom = parseInt(styles.getPropertyValue('padding-bottom'), 10); | ||
this.height -= (this.paddingTop + this.paddingBottom); | ||
this._position(); | ||
}; | ||
this._position(); | ||
}; | ||
/** | ||
* Position all elements in the grid based on the class names | ||
* | ||
* Currently does not support multiple flexing elements in a single column | ||
* (flex = automatically adjust the height to the available space) | ||
* | ||
* @return {void} | ||
* @private | ||
*/ | ||
Grid.prototype._position = function () { | ||
var that = this, | ||
columns = [], | ||
numCols = this.el.className.match(/cols\-([0-9]+)/); | ||
/** | ||
* Position all elements in the grid based on the class names | ||
* | ||
* Currently does not support multiple flexing elements in a single column | ||
* (flex = automatically adjust the height to the available space) | ||
* | ||
* @return {void} | ||
* @private | ||
*/ | ||
Grid.prototype._position = function () { | ||
var that = this; | ||
var columns = []; | ||
var numCols = this.el.className.match(/cols\-([0-9]+)/); | ||
if (!numCols) { | ||
throw new Error('Failed to find number of columns'); | ||
} | ||
if (!numCols) { | ||
throw new Error('Failed to find number of columns'); | ||
} | ||
// Initialize each column | ||
numCols = parseInt(numCols[1], 10); | ||
for (var i = 0; i < numCols; i++) { | ||
columns[i] = { | ||
remainingHeight: this.height, | ||
cells: [] | ||
}; | ||
} | ||
// Initialize each column | ||
numCols = parseInt(numCols[1], 10); | ||
for (var i = 0; i < numCols; i++) { | ||
columns[i] = { | ||
remainingHeight: this.height, | ||
cells: [] | ||
}; | ||
} | ||
$(this.el).find('[class*="col-"]').each(function () { | ||
var col = parseInt(this.className.match(/col\-([0-9]+)/)[1], 10) - 1, | ||
span = this.className.match(/colspan\-([0-9]+)/), | ||
cell = that._createCellFromEl(this); | ||
$(this.el).find('[class*="col-"]').each(function () { | ||
var col = parseInt(this.className.match(/col\-([0-9]+)/)[1], 10) - 1; | ||
var span = this.className.match(/colspan\-([0-9]+)/); | ||
var cell = that._createCellFromEl(this); | ||
span = span ? parseInt(span[1], 10) : 1; | ||
span = span ? parseInt(span[1], 10) : 1; | ||
if ($(this).css('display') === 'none') { | ||
return; | ||
} | ||
if ($(this).css('display') === 'none') { | ||
return; | ||
} | ||
// Cells that span across multiple columns are added to | ||
// the array for each column it spans across | ||
for (var i = col; i < (col + span); i++) { | ||
columns[i].cells.push(cell); | ||
} | ||
}); | ||
// Cells that span across multiple columns are added to | ||
// the array for each column it spans across | ||
for (var i = col; i < (col + span); i++) { | ||
columns[i].cells.push(cell); | ||
} | ||
}); | ||
this._calcMetrics(columns); | ||
this._calcMetrics(columns); | ||
$.each(columns, function (index, column) { | ||
that._positionColumn(index, column); | ||
}); | ||
}; | ||
$.each(columns, function (index, column) { | ||
that._positionColumn(index, column); | ||
}); | ||
}; | ||
/** | ||
* Create a cell object from an HTML element | ||
* | ||
* Contains all required properties to calculate the grid | ||
* | ||
* @param {HTMLElement} el | ||
* @return {Object} | ||
*/ | ||
Grid.prototype._createCellFromEl = function (el) { | ||
var flex = $(el).hasClass(this._class('flex')); | ||
/** | ||
* Create a cell object from an HTML element | ||
* | ||
* Contains all required properties to calculate the grid | ||
* | ||
* @param {HTMLElement} el | ||
* @return {Object} | ||
*/ | ||
Grid.prototype._createCellFromEl = function (el) { | ||
var flex = $(el).hasClass(this._class('flex')); | ||
return { | ||
el: el, | ||
height: 0, | ||
innerHeight: 0, | ||
top: 0, | ||
flex: flex | ||
}; | ||
return { | ||
el, | ||
flex, | ||
height: 0, | ||
innerHeight: 0, | ||
top: 0 | ||
}; | ||
}; | ||
Grid.prototype._calcMetrics = function (columns) { | ||
var colTop, colBottom, numColumns, numCells, cell, $cell, i, j, styles, marginBottom | ||
// jshint maxcomplexity:false | ||
// jshint maxstatements:32 | ||
Grid.prototype._calcMetrics = function (columns) { | ||
var colTop, colBottom, numColumns, numCells, cell, $cell, i, j, styles, marginBottom; | ||
colTop = this._class('col-top'); | ||
colBottom = this._class('col-bottom'); | ||
colTop = this._class('col-top'); | ||
colBottom = this._class('col-bottom'); | ||
numColumns = columns.length; | ||
numColumns = columns.length; | ||
for (i = 0; i < numColumns; i++) { | ||
numCells = columns[i].cells.length; | ||
for (i = 0; i < numColumns; i++) { | ||
numCells = columns[i].cells.length; | ||
for (j = 0; j < numCells; j++) { | ||
cell = columns[i].cells[j]; | ||
$cell = $(cell.el); | ||
$cell.removeClass(colTop + ' ' + colBottom); | ||
for (j = 0; j < numCells; j++) { | ||
cell = columns[i].cells[j]; | ||
$cell = $(cell.el); | ||
$cell.removeClass(colTop + ' ' + colBottom); | ||
if (j === 0) { | ||
cell.isTop = true; | ||
$cell.addClass(colTop); | ||
} | ||
if (j === 0) { | ||
cell.isTop = true; | ||
$cell.addClass(colTop); | ||
} | ||
if (j === (numCells - 1)) { | ||
cell.isBottom = true; | ||
$cell.addClass(colBottom); | ||
} | ||
if (j === (numCells - 1)) { | ||
cell.isBottom = true; | ||
$cell.addClass(colBottom); | ||
} | ||
} | ||
} | ||
// Batch calculatation for DOM performance | ||
for (i = 0; i < numColumns; i++) { | ||
numCells = columns[i].cells.length; | ||
// Batch calculatation for DOM performance | ||
for (i = 0; i < numColumns; i++) { | ||
numCells = columns[i].cells.length; | ||
for (j = 0; j < numCells; j++) { | ||
cell = columns[i].cells[j]; | ||
$cell = $(cell.el); | ||
for (j = 0; j < numCells; j++) { | ||
cell = columns[i].cells[j]; | ||
$cell = $(cell.el); | ||
cell.height = cell.flex ? 0 : $cell.outerHeight(); | ||
cell.height = cell.flex ? 0 : $cell.outerHeight(); | ||
// If marginTop on the top element does not align to the vertical grid, | ||
// it'll mess up the relationship between the different columns. | ||
// This is required to make sure that columns with different number of rows still align properly | ||
if (cell.isTop) { | ||
styles = window.getComputedStyle(cell.el, null); | ||
marginTop = $cell.css('margin-top'); | ||
columns[i].marginTop = parseInt(styles.getPropertyValue('margin-top'), 10); | ||
columns[i].marginTopOffset = columns[i].marginTop % this.lineHeight; | ||
// If marginTop on the top element does not align to the vertical grid, | ||
// it'll mess up the relationship between the different columns. | ||
// This is required to make sure that columns with different number of rows still align properly | ||
if (cell.isTop) { | ||
styles = window.getComputedStyle(cell.el, null); | ||
columns[i].marginTop = parseInt(styles.getPropertyValue('margin-top'), 10); | ||
columns[i].marginTopOffset = columns[i].marginTop % this.lineHeight; | ||
if (!cell.flex) { | ||
cell.innerHeight = $cell.height(); | ||
if (cell.innerHeight === 0) { | ||
marginBottom = parseInt(styles.getPropertyValue('margin-bottom'), 10); | ||
cell.height -= marginBottom; | ||
} | ||
if (!cell.flex) { | ||
cell.innerHeight = $cell.height(); | ||
if (cell.innerHeight === 0) { | ||
marginBottom = parseInt(styles.getPropertyValue('margin-bottom'), 10); | ||
cell.height -= marginBottom; | ||
} | ||
} | ||
} | ||
columns[i].remainingHeight -= cell.height; | ||
} | ||
columns[i].remainingHeight -= cell.height; | ||
} | ||
}; | ||
} | ||
}; | ||
/** | ||
* Position a single column | ||
* | ||
* Helper method for _position | ||
* | ||
* @param {number} index | ||
* @param {HTMLElement} column | ||
* @return {void} | ||
* @private | ||
*/ | ||
Grid.prototype._positionColumn = function (index, column) { | ||
var that = this, top = this.paddingTop; | ||
/** | ||
* Position a single column | ||
* | ||
* Helper method for _position | ||
* | ||
* @param {number} index | ||
* @param {HTMLElement} column | ||
* @return {void} | ||
* @private | ||
*/ | ||
Grid.prototype._positionColumn = function (index, column) { | ||
var that = this; | ||
var top = this.paddingTop; | ||
$.each(column.cells, function (index, cell) { | ||
var $cell = $(cell.el), css = {}, rest = 0; | ||
$.each(column.cells, function (index, cell) { | ||
var $cell = $(cell.el); | ||
var css = {}; | ||
var rest = 0; | ||
// only snap to the vertical grid if it's not the top cell | ||
// AND if it's a flexing cell | ||
if (that.lineHeight && !cell.isTop && !(cell.isBottom && !cell.flex)) { | ||
rest = ((top - column.marginTopOffset - that.paddingTop) % that.lineHeight); | ||
// only snap to the vertical grid if it's not the top cell | ||
// AND if it's a flexing cell | ||
if (that.lineHeight && !cell.isTop && !(cell.isBottom && !cell.flex)) { | ||
rest = ((top - column.marginTopOffset - that.paddingTop) % that.lineHeight); | ||
if (rest !== 0) { | ||
rest = (that.lineHeight - rest); | ||
column.remainingHeight -= rest; | ||
top += rest; | ||
} | ||
if (rest !== 0) { | ||
rest = (that.lineHeight - rest); | ||
column.remainingHeight -= rest; | ||
top += rest; | ||
} | ||
} | ||
// Push it down to the lowest position so it doesn't overlap with any of the cells above it | ||
if (!cell.isTop || cell.top < top) { | ||
cell.top = top; | ||
} | ||
// Push it down to the lowest position so it doesn't overlap with any of the cells above it | ||
if (!cell.isTop || cell.top < top) { | ||
cell.top = top; | ||
} | ||
css.top = cell.top + 'px'; | ||
css.top = cell.top + 'px'; | ||
if (cell.flex) { | ||
var margin = parseInt($cell.css('margin-bottom'), 10) + parseInt($cell.css('margin-top'), 10); | ||
if (cell.flex) { | ||
var margin = parseInt($cell.css('margin-bottom'), 10) + parseInt($cell.css('margin-top'), 10); | ||
cell.height = column.remainingHeight; | ||
css.height = (cell.height > margin ? cell.height - margin : 0) + 'px'; | ||
} | ||
cell.height = column.remainingHeight; | ||
css.height = (cell.height > margin ? cell.height - margin : 0) + 'px'; | ||
} | ||
$cell.css(css); | ||
top += cell.height; | ||
}); | ||
}; | ||
$cell.css(css); | ||
top += cell.height; | ||
}); | ||
}; | ||
/** | ||
* Prefix a class name | ||
* | ||
* @param {string} string | ||
* @return {string} prefixed string | ||
*/ | ||
Grid.prototype._class = function (string) { | ||
return this.options.prefix + string; | ||
}; | ||
/** | ||
* Prefix a class name | ||
* | ||
* @param {string} string | ||
* @return {string} prefixed string | ||
*/ | ||
Grid.prototype._class = function (string) { | ||
return this.options.prefix + string; | ||
}; | ||
if (typeof window !== 'undefined') { | ||
$.fn.grid = function (options) { | ||
@@ -238,5 +243,4 @@ options = options || {}; | ||
}; | ||
} | ||
window.Grid = Grid; | ||
}(this, window.Zepto || window.jQuery)); | ||
export default Grid; |
{ | ||
"name": "alf", | ||
"version": "1.5.4", | ||
"version": "2.0.0", | ||
"description": "Aptoma Layout Framework", | ||
@@ -9,2 +9,3 @@ "repository": { | ||
}, | ||
"main": "alf.js", | ||
"author": "Peter Rudolfsen <peter@aptoma.com>", | ||
@@ -16,3 +17,7 @@ "license": "Proprietary", | ||
"homepage": "https://github.com/aptoma/alf-dist", | ||
"dependencies": {} | ||
"dependencies": { | ||
"backbone": "^1.2.1", | ||
"jquery": "^2.1.4", | ||
"lodash": "^3.10.1" | ||
} | ||
} |
@@ -6,7 +6,53 @@ ALF - Aptoma Layout Framework (distribution version) | ||
ALF can be installed with [Bower](http://bower.io/) or [npm](https://www.npmjs.org/). | ||
ALF can be installed with [Bower](http://bower.io/) or [npm](https://www.npmjs.org/). We highly recommend using NPM instead of Bower, as most bundlers work better with NPM. | ||
npm install aptoma-alf | ||
bower install aptoma-alf | ||
npm install alf | ||
bower install alf | ||
## Which bundle? | ||
There are 2: | ||
* `alf.js` - **Default**. Defaults to depending on jQuery ([browser support](https://jquery.com/browser-support/)), but also works with Zepto (([browser support](http://zeptojs.com/#browsers)). You may switch to Zepto in your bundler config (see below). | ||
* `alf-deps.js` - Bundled with Zepto. All-in-one bundle with all dependencides included. Can also be included in your own app-bundle using a module bundler, but that's only recommended if your app is centered mainly around ALF. Consider using `alf.js` if you have overlapping dependencies. | ||
**ALF is using the [UMD](https://github.com/umdjs/umd) pattern**. This means that it will look for dependencies and register itself in the system it finds first of the following: | ||
* CommonJS2 | ||
* AMD | ||
* CommonJS | ||
* Window | ||
## Webpack tips | ||
We recommend using [NPM](https://www.npmjs.com/), but if you have to use [Bower](http://bower.io/), there's some [more info at the webpack site](http://webpack.github.io/docs/usage-with-bower.html) about how you configure it. | ||
**Example config using Webpack with NPM** | ||
```javascript | ||
module.exports = { | ||
entry: { | ||
'my-bundle': "./my-app.js", | ||
}, | ||
output: { | ||
path: __dirname + '/dist', | ||
filename: '[name].js', | ||
libraryTarget: 'var', | ||
library: 'MyApp' | ||
}, | ||
resolve: { | ||
root: [ | ||
// Use this to make Webpack always prefer locally installed modules instead of the ones inside ALF. This will prevent including dependencies twice in your bundle (like lodash) | ||
path.resolve(__dirname, 'node_modules'), | ||
], | ||
alias: { | ||
// This is recommended, as it will prevent submodules from using underscore when lodash is available. Will reduce the file size. | ||
underscore: 'lodash', | ||
// Use to replace with zepto when using ALF without dependencies | ||
jquery: 'npm-zepto' | ||
} | ||
} | ||
}; | ||
``` | ||
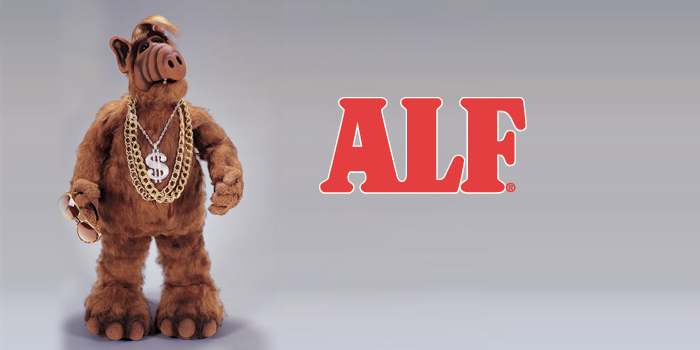 |
Sorry, the diff of this file is too big to display
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Minified code
QualityThis package contains minified code. This may be harmless in some cases where minified code is included in packaged libraries, however packages on npm should not minify code.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
New author
Supply chain riskA new npm collaborator published a version of the package for the first time. New collaborators are usually benign additions to a project, but do indicate a change to the security surface area of a package.
Found 1 instance in 1 package
Uses eval
Supply chain riskPackage uses dynamic code execution (e.g., eval()), which is a dangerous practice. This can prevent the code from running in certain environments and increases the risk that the code may contain exploits or malicious behavior.
Found 1 instance in 1 package
Dynamic require
Supply chain riskDynamic require can indicate the package is performing dangerous or unsafe dynamic code execution.
Found 1 instance in 1 package
Minified code
QualityThis package contains minified code. This may be harmless in some cases where minified code is included in packaged libraries, however packages on npm should not minify code.
Found 1 instance in 1 package
30
2438
2
58
0
1
471205
3
+ Addedbackbone@^1.2.1
+ Addedjquery@^2.1.4
+ Addedlodash@^3.10.1
+ Addedbackbone@1.6.0(transitive)
+ Addedjquery@2.2.4(transitive)
+ Addedlodash@3.10.1(transitive)
+ Addedunderscore@1.13.7(transitive)