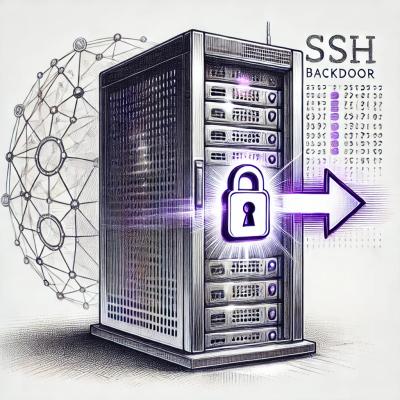
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
amqplib-plus
Advanced tools
Amqplib-plus amplifies the original npm amqplib library with OOP approach.
Amqplib-plus adds following features:
$ npm install amqplib-plus
import {Connection, Consumer, Publisher} from "amqplib-plus";
import {Channel, Message} from "amqplib";
import {CustomConsumer} from "./CustomConsumer"; // CustomConsumer is your own consumer implementation that extends amqplib-plus Consumer
const queue = { name: "some_queue_name", options: {} };
const msgContent = "some content";
// callback to be called when creating the consumer, before it starts consuming
// consumer wants to be sure the queue exists
const consumerPrepare: any = (ch: Channel) => {
return ch.assertQueue(queue.name, queue.options);
};
// the callback to be called on every consumed message
// you process the message as you like and tken ack/reject it as you wish
const handleMsg = (msg: Message, ch: Channel) => {
console.log(msg.content.toString());
ch.ack(msg);
};
// creates the connection to rabbitmq broker
const amqpConn = new Connection(
{ connectionString: 'amqp://guest:guest@localhost:5672/' },
console // you may use custom logger or avoid it to disable logging
);
// create the consumer using existing connection and start the consumption
const consumer = new CustomConsumer(conn, consumerPrepare, handleMsg);
consumer.consume(queue.name, {});
// callback to be called when creating the publisher before it starts publishing
// publisher wants to be sure the publishing queue exists
const publisherPrepare: any = (ch: Channel) => {
return ch.assertQueue(queue.name, queue.options);
};
// create the publisher using the existing connection
const publisher = new Publisher(conn, publisherPrepare);
// send a message that consumer should consume
publisher.sendToQueue(queue.name, Buffer.from(msgContent), {});
Create pull request to https://github.com/hanaboso/amqplib-plus
repository.
Please note that this lib is written in typescript. Your contribution is very welcome.
If you have running rabbitmq instance, set env variable values defined used in test/config.ts (default rabbitmq dsn is 'amqp://guest:guest@localhost:5672/') and run: npm run test
.
Or you can use custom rabbitmq instance in test by running RABBIT_DSN=amqp://guest:guest@yourserver:5672/ npm run test
Alternatively you can run: make test
which will start rabbitmq instance for you and run tests in docker-compose.
FAQs
Amqplib rabbitmq library object oriented enhancement.
We found that amqplib-plus demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.