analytics-plugin-google-tag-manager
Advanced tools
Comparing version 0.0.2 to 0.1.0
@@ -1,96 +0,127 @@ | ||
'use strict'; | ||
var analyticsGtagManager = (function () { | ||
'use strict'; | ||
Object.defineProperty(exports, '__esModule', { value: true }); | ||
function _defineProperty(obj, key, value) { | ||
if (key in obj) { | ||
Object.defineProperty(obj, key, { | ||
value: value, | ||
enumerable: true, | ||
configurable: true, | ||
writable: true | ||
}); | ||
} else { | ||
obj[key] = value; | ||
} | ||
var _extends = Object.assign || function (target) { | ||
for (var i = 1; i < arguments.length; i++) { | ||
var source = arguments[i]; | ||
return obj; | ||
} | ||
for (var key in source) { | ||
if (Object.prototype.hasOwnProperty.call(source, key)) { | ||
target[key] = source[key]; | ||
function _objectSpread(target) { | ||
for (var i = 1; i < arguments.length; i++) { | ||
var source = arguments[i] != null ? arguments[i] : {}; | ||
var ownKeys = Object.keys(source); | ||
if (typeof Object.getOwnPropertySymbols === 'function') { | ||
ownKeys = ownKeys.concat(Object.getOwnPropertySymbols(source).filter(function (sym) { | ||
return Object.getOwnPropertyDescriptor(source, sym).enumerable; | ||
})); | ||
} | ||
ownKeys.forEach(function (key) { | ||
_defineProperty(target, key, source[key]); | ||
}); | ||
} | ||
return target; | ||
} | ||
return target; | ||
}; | ||
/* global dataLayer */ | ||
// Analytics Integration Configuration | ||
var config$1 = { | ||
assumesPageview: true | ||
}; | ||
function googleTagManager$1(userConfig) { | ||
// Allow for userland overides of base methods | ||
return { | ||
NAMESPACE: 'google-tag-manager', | ||
config: Object.assign({}, config$1, userConfig), | ||
initialize: function initialize(_ref) { | ||
var config = _ref.config; | ||
var containerId = config.containerId; | ||
/* global dataLayer */ | ||
var inBrowser = typeof window !== 'undefined'; | ||
if (!containerId) { | ||
throw new Error('No google tag manager containerId defined'); | ||
} | ||
// Analytics Integration Configuration | ||
var config = { | ||
assumesPageview: true | ||
}; | ||
if (typeof dataLayer === 'undefined') { | ||
/* eslint-disable */ | ||
(function (w, d, s, l, i) { | ||
w[l] = w[l] || []; | ||
w[l].push({ | ||
'gtm.start': new Date().getTime(), | ||
event: 'gtm.js' | ||
}); | ||
var f = d.getElementsByTagName(s)[0], | ||
j = d.createElement(s), | ||
dl = l != 'dataLayer' ? '&l=' + l : ''; | ||
j.async = true; | ||
j.src = 'https://www.googletagmanager.com/gtm.js?id=' + i + dl; | ||
f.parentNode.insertBefore(j, f); | ||
})(window, document, 'script', 'dataLayer', containerId); | ||
/* eslint-enable */ | ||
function googleTagManager(userConfig) { | ||
// Allow for userland overides of base methods | ||
return { | ||
NAMESPACE: 'google-tag-manager', | ||
config: Object.assign({}, config, userConfig), | ||
initialize: function initialize(_ref) { | ||
var config = _ref.config; | ||
var containerId = config.containerId; | ||
} | ||
}, | ||
page: function page(_ref2) { | ||
var payload = _ref2.payload, | ||
options = _ref2.options, | ||
instance = _ref2.instance; | ||
if (!containerId) { | ||
throw new Error('No google tag manager containerId defined'); | ||
} | ||
if (inBrowser && typeof dataLayer === 'undefined') { | ||
/* eslint-disable */ | ||
(function (w, d, s, l, i) { | ||
w[l] = w[l] || []; | ||
w[l].push({ 'gtm.start': new Date().getTime(), event: 'gtm.js' }); | ||
var f = d.getElementsByTagName(s)[0], | ||
j = d.createElement(s), | ||
dl = l != 'dataLayer' ? '&l=' + l : ''; | ||
j.async = true; | ||
j.src = 'https://www.googletagmanager.com/gtm.js?id=' + i + dl; | ||
f.parentNode.insertBefore(j, f); | ||
})(window, document, 'script', 'dataLayer', containerId); | ||
/* eslint-enable */ | ||
} | ||
}, | ||
page: function page(_ref2) { | ||
var payload = _ref2.payload, | ||
options = _ref2.options, | ||
instance = _ref2.instance; | ||
if (typeof dataLayer !== 'undefined') { | ||
dataLayer.push(payload.properties); | ||
} | ||
}, | ||
track: function track(_ref3) { | ||
var payload = _ref3.payload, | ||
options = _ref3.options; | ||
if (inBrowser && typeof dataLayer !== 'undefined') { | ||
dataLayer.push(payload.properties); | ||
} | ||
}, | ||
track: function track(_ref3) { | ||
var payload = _ref3.payload, | ||
options = _ref3.options; | ||
if (typeof dataLayer !== 'undefined') { | ||
var anonymousId = payload.anonymousId, | ||
userId = payload.userId, | ||
properties = payload.properties, | ||
category = payload.category; | ||
var formattedPayload = properties; | ||
if (inBrowser && typeof dataLayer !== 'undefined') { | ||
var anonymousId = payload.anonymousId, | ||
userId = payload.userId, | ||
properties = payload.properties, | ||
category = payload.category; | ||
if (userId) { | ||
formattedPayload.userId = userId; | ||
} | ||
var formattedPayload = properties; | ||
if (userId) { | ||
formattedPayload.userId = userId; | ||
if (anonymousId) { | ||
formattedPayload.anonymousId = anonymousId; | ||
} | ||
if (!category) { | ||
formattedPayload.category = 'All'; | ||
} | ||
console.log('gtag push', _objectSpread({ | ||
event: payload.event | ||
}, formattedPayload)); | ||
dataLayer.push(_objectSpread({ | ||
event: payload.event | ||
}, formattedPayload)); | ||
} | ||
if (anonymousId) { | ||
formattedPayload.anonymousId = anonymousId; | ||
} | ||
if (!category) { | ||
formattedPayload.category = 'All'; | ||
} | ||
dataLayer.push(_extends({ | ||
event: payload.event | ||
}, formattedPayload)); | ||
}, | ||
loaded: function loaded() { | ||
return !!(window.dataLayer && Array.prototype.push !== window.dataLayer.push); | ||
} | ||
}, | ||
loaded: function loaded() { | ||
if (!inBrowser) return true; | ||
return !!(window.dataLayer && Array.prototype.push !== window.dataLayer.push); | ||
} | ||
}; | ||
} | ||
}; | ||
} | ||
exports.config = config; | ||
exports.default = googleTagManager; | ||
/* This module will shake out unused code and work in browser and node 🎉 */ | ||
var index = googleTagManager$1; | ||
return index; | ||
}()); |
{ | ||
"name": "analytics-plugin-google-tag-manager", | ||
"version": "0.0.2", | ||
"version": "0.1.0", | ||
"description": "Google tag manager plugin for 'analytics' pkg", | ||
"author": "David Wells", | ||
"license": "MIT", | ||
"homepage": "https://github.com/DavidWells/analytics#readme", | ||
"keywords": [ | ||
@@ -12,9 +9,8 @@ "analytics", | ||
], | ||
"main": "dist/analytics-plugin-google-tag-manager.js", | ||
"module": "dist/analytics-plugin-google-tag-manager.esm.js", | ||
"author": "David Wells", | ||
"license": "MIT", | ||
"scripts": { | ||
"test": "echo \"Error: no test specified\" && exit 1", | ||
"build": "node ../../scripts/plugins/build.js --nameSpace google-tag-manager", | ||
"build:dev": "node ../../scripts/plugins/build.js --nameSpace google-tag-manager --env dev", | ||
"watch": "node ../../scripts/plugins/watch.js --nameSpace google-tag-manager", | ||
"build": "node ../../scripts/build/index.js", | ||
"watch": "node ../../scripts/build/_watch.js", | ||
"release:patch": "npm version patch && npm publish", | ||
@@ -25,10 +21,25 @@ "release:minor": "npm version minor && npm publish", | ||
}, | ||
"main": "lib/analytics-plugin-google-tag-manager.cjs.js", | ||
"globalName": "analyticsGtagManager", | ||
"jsnext:main": "lib/analytics-plugin-google-tag-manager.es.js", | ||
"module": "lib/analytics-plugin-google-tag-manager.es.js", | ||
"browser": { | ||
"./lib/analytics-plugin-google-tag-manager.cjs.js": "./lib/analytics-plugin-google-tag-manager.browser.cjs.js", | ||
"./lib/analytics-plugin-google-tag-manager.es.js": "./lib/analytics-plugin-google-tag-manager.browser.es.js" | ||
}, | ||
"files": [ | ||
"dist", | ||
"lib", | ||
"dist", | ||
"package.json", | ||
"package-lock.json", | ||
"README.md" | ||
], | ||
"gitHead": "ad272eb263ac6a9c8eb69c467909e26309a650f1" | ||
"homepage": "https://github.com/DavidWells/analytics#readme", | ||
"repository": { | ||
"type": "git", | ||
"url": "git+https://github.com/DavidWells/analytics.git" | ||
}, | ||
"devDependencies": { | ||
"@babel/core": "^7.2.2", | ||
"@babel/preset-env": "^7.3.1" | ||
}, | ||
"gitHead": "cfd94ff8bf094a6024e824260238bc03eaf0d70f" | ||
} |
@@ -15,3 +15,3 @@ # Google analytics plugin for `analytics` | ||
import Analytics from 'analytics' | ||
import gtagManager from 'analytics-plugin-google-tag-manager' | ||
import googleTagManager from 'analytics-plugin-google-tag-manager' | ||
@@ -22,3 +22,5 @@ const analytics = Analytics({ | ||
plugins: [ | ||
gtagManager() | ||
googleTagManager({ | ||
containerId: 'Your-containerId' | ||
}) | ||
] | ||
@@ -28,2 +30,10 @@ }) | ||
## Setup | ||
Make sure you have your google tags manager setup to fire on Page views. | ||
If you are using a SPA you want to listen to history changes as well. | ||
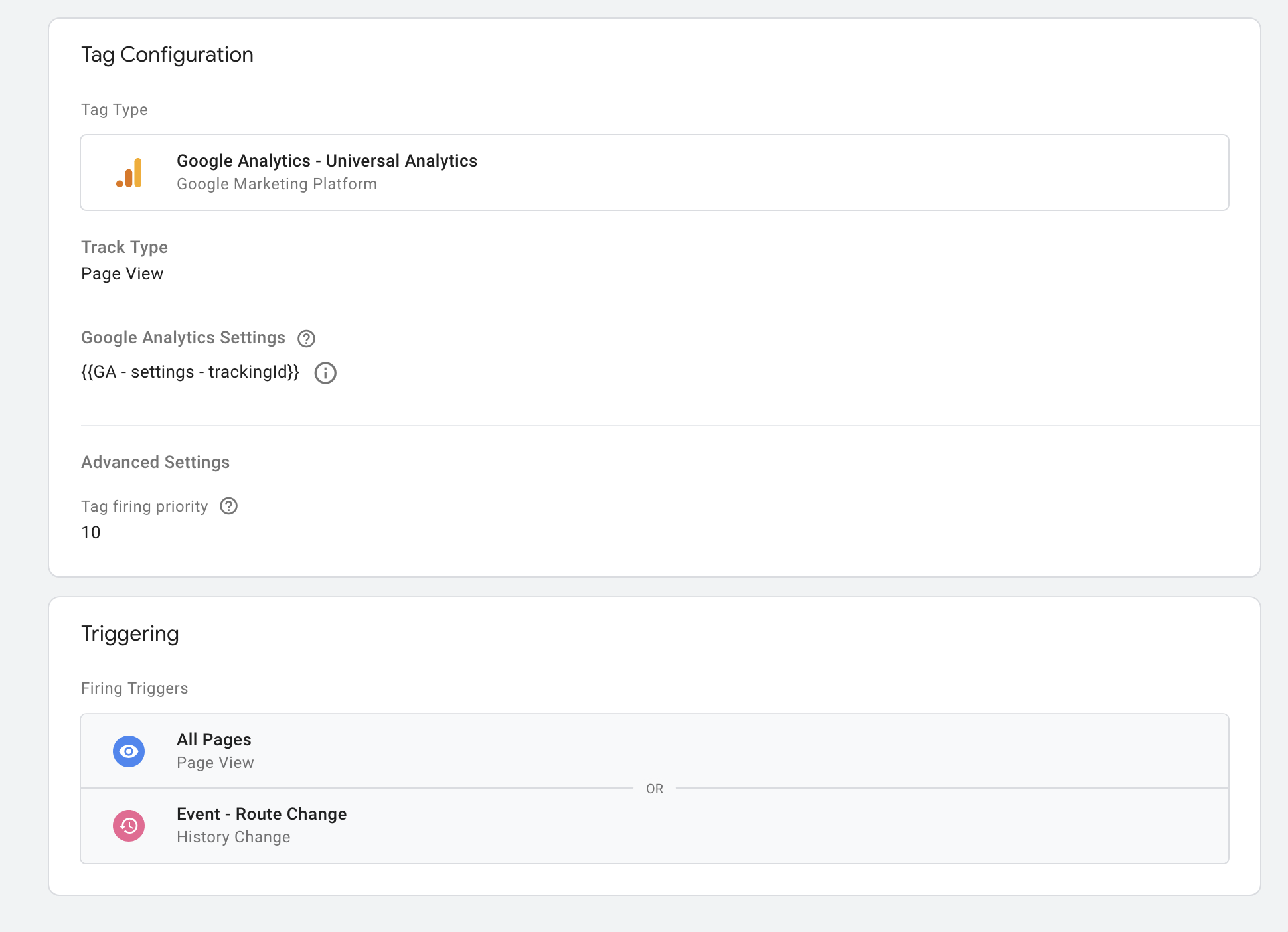 | ||
See the [full list of analytics provider plugins](https://github.com/DavidWells/analytics#current-plugins) in the main repo. |
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
No repository
Supply chain riskPackage does not have a linked source code repository. Without this field, a package will have no reference to the location of the source code use to generate the package.
Found 1 instance in 1 package
37
0
16420
2
447
1