ang-google-maps
Advanced tools
Comparing version 1.3.0 to 1.3.1
{ | ||
"name": "ang-google-maps", | ||
"version": "1.3.0", | ||
"version": "1.3.1", | ||
"homepage": "https://github.com/khalednobani/ang-google-maps", | ||
@@ -5,0 +5,0 @@ "description": "Initialise Google Maps, Set Location, Drop Pins, Get Connected Routes between the current location and the destination.", |
@@ -8,5 +8,3 @@ (function(ang) { | ||
.filter('getByName', function() { | ||
return function(input, name) { | ||
for (var index = 0, length = ('length' in input) ? input.length : 0; index < length; index++) { | ||
@@ -19,11 +17,7 @@ | ||
} | ||
return -1; | ||
} | ||
}); | ||
function mainCtrl($scope, Direction, $Geocode, $rootScope, $filter) { | ||
var $Self = this; | ||
@@ -42,3 +36,2 @@ | ||
$scope.setInputFieldForMarker = function($Event, currentMarkerName) { | ||
// Get the name of current location | ||
@@ -57,19 +50,13 @@ $Geocode.getNames({coords: $Event.latLng}) | ||
}, function(err) { | ||
console.error(err); | ||
}); | ||
}; | ||
$scope.updatePickup = function($CoreModel, $Position) { | ||
console.log("Updating Pickup"); | ||
$scope.location['pickUp'] = $Position; | ||
$scope.setLocation(); | ||
}; | ||
$scope.handleMapClick = function($Coords, $Pixel, $Za) { | ||
if ($scope.$MarkerList[0] != $scope.currentMarker) return new Error("Can't create a marker."); | ||
@@ -98,7 +85,5 @@ | ||
$scope.$MarkerList.splice(0, 1); | ||
}; | ||
$scope.handleMarkerDrop = function($Event, $Model, $AutoCompScope) { | ||
$Geocode.getNames({ | ||
@@ -112,14 +97,10 @@ coords: $Event.latLng | ||
}); | ||
}; | ||
$scope.setPickup = function($Position, $Model, $CoreModel) { | ||
$scope.location['pickUp'] = $Position; | ||
$scope.setLocation(); | ||
}; | ||
$scope.setDropoff = function($Position, $Model, $CoreModel, name) { | ||
$scope.location[name] = $Position; | ||
@@ -145,7 +126,5 @@ | ||
$scope.setLocation(); | ||
}; | ||
$scope.getWaypoints = function() { | ||
var $List = []; | ||
@@ -168,7 +147,5 @@ | ||
return $List; | ||
}; | ||
$scope.setCurrentDestination = function(name) { | ||
if (!name) return; | ||
@@ -185,7 +162,5 @@ | ||
$scope.setLocation(); | ||
}; | ||
$scope.setLocation = function($Position, $Model, $CoreModel) { | ||
var current = $scope.location['pickUp'], | ||
@@ -221,13 +196,4 @@ destination = $scope.location[$scope.currentDestination]; | ||
$scope.handleDirectionChange = function($Leg, $parentScope, $Directions) { | ||
$scope.orderedWaypoints = $Directions.routes[0].waypoint_order; | ||
console.log("$scope.orderedWaypoints"); | ||
console.log($scope.orderedWaypoints); | ||
return; | ||
console.log('Handle on change'); | ||
console.log(arguments); | ||
$parentScope['pickUp'] = $scope['pickUp'] = $Leg.current.name; | ||
@@ -237,8 +203,4 @@ $parentScope[$scope.currentDestination] = $scope[$scope.currentDestination] = $Leg.destination.name; | ||
$scope.location[$scope.currentDestination] = $Leg.destination.coords; | ||
console.log("Before Updating dropoffs"); | ||
console.log(JSON.stringify($scope.dropOffs)); | ||
$scope.updateWaypoints($parentScope, $Leg, $Leg.waypoints); | ||
}; | ||
@@ -245,0 +207,0 @@ |
{ | ||
"name": "ang-google-maps", | ||
"version": "1.3.0", | ||
"version": "1.3.1", | ||
"description": "Module to initialize Google Maps API", | ||
@@ -5,0 +5,0 @@ "main": "index.js", |
@@ -5,12 +5,14 @@ # ang-google-maps | ||
## Features | ||
1. Adding Google Directions | ||
2. Listening to the changes of directions event. | ||
3. Supporting multiple waypoints / dropoffs on the map (Max is 8). | ||
4. Centering map to the current location using Geolocation feature. | ||
5. Dropping the marker into the map on `click` event. | ||
6. Attaching the callback for marker's `click` event while dropping the marker into the map / textfield input filled (AutoComplete). | ||
7. Deleting the marker from the map with deletingMarker. | ||
8. Reset direction's route. | ||
1. Adding Google Directions | ||
2. Listening to the changes of directions event. | ||
3. Supporting multiple waypoints / dropoffs on the map (Max is 8). | ||
4. Centering map to the current location using Geolocation feature. | ||
5. Dropping the marker into the map on `click` event. | ||
6. Attaching the callback for marker's `click` event while dropping the marker into the map / textfield input filled (AutoComplete). | ||
7. Deleting the marker from the map with deletingMarker. | ||
8. Reset direction's route. | ||
9. Drawing polyline. | ||
10. Getting the marker object. | ||
### Screenshots | ||
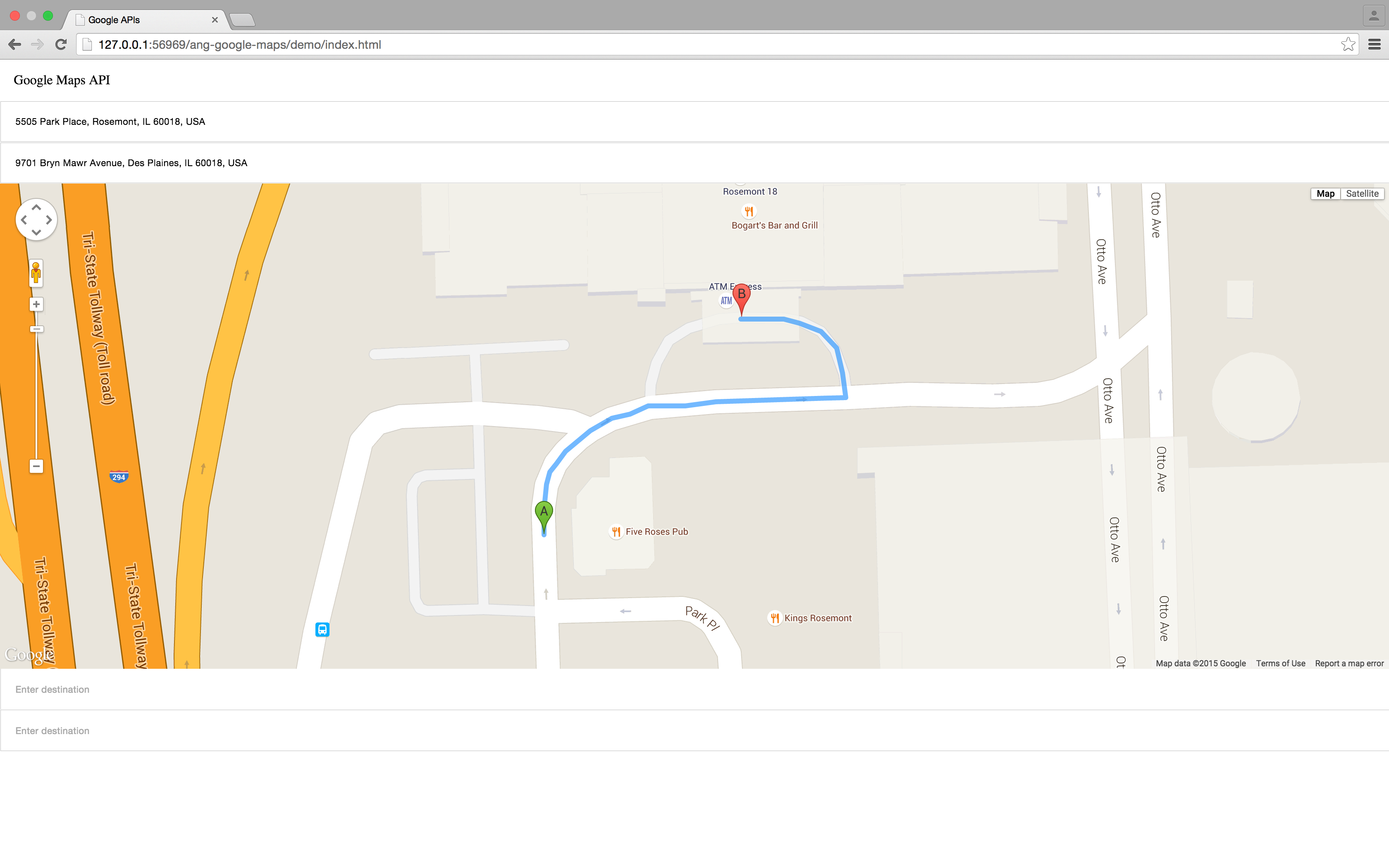 | ||
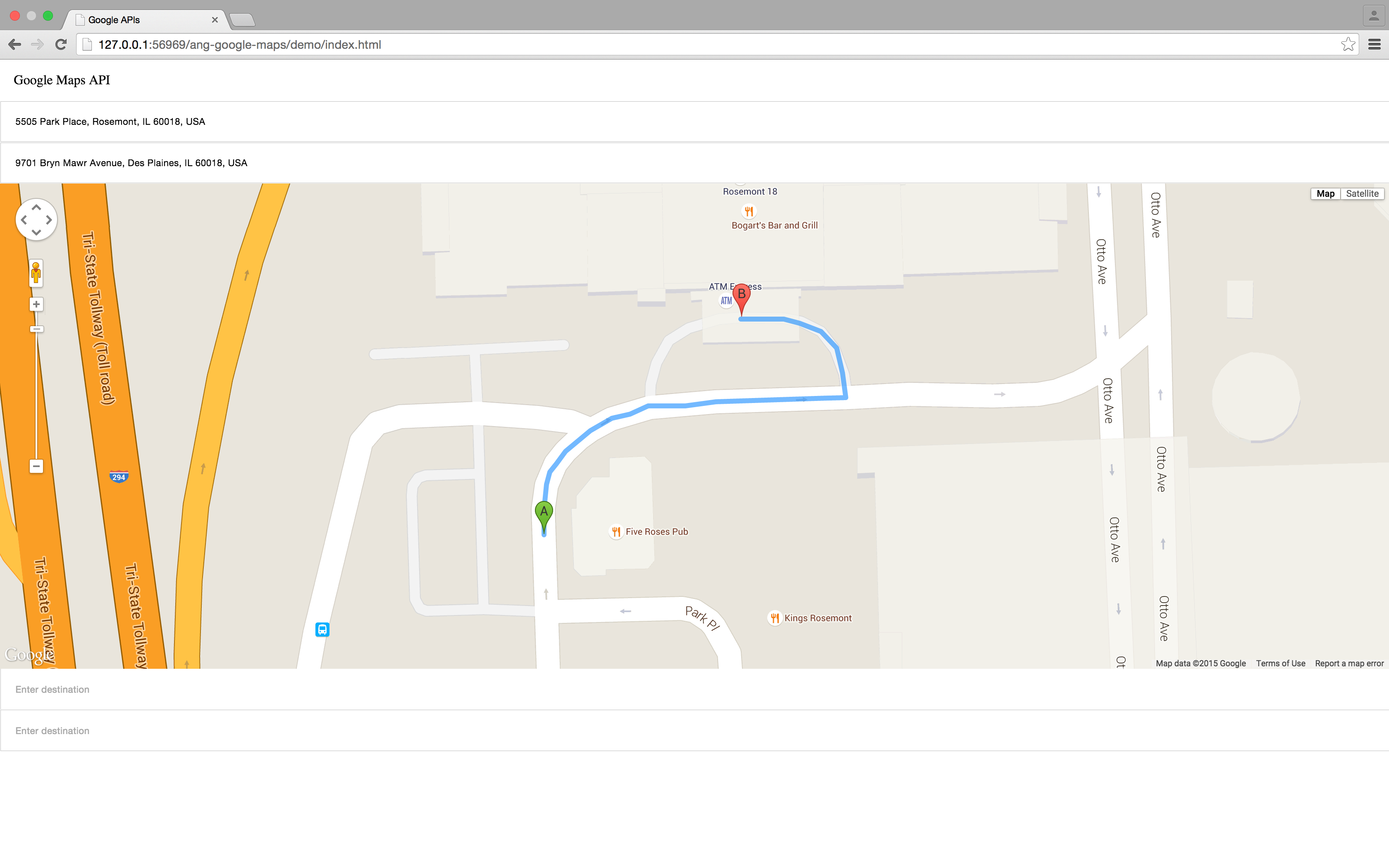 |
@@ -109,3 +109,3 @@ (function(ang, g) { | ||
} | ||
/** | ||
@@ -291,2 +291,178 @@ * Factory of google map's marker | ||
/** | ||
* Extends maps's object. | ||
* | ||
* @param Object $Maps. | ||
*/ | ||
function ExtendsMaps($Maps) { | ||
var $Maps = $Maps || {}, | ||
$RefMaps = { $Maps: $Maps }; | ||
$RefMaps.searchMarkers = function(name) { | ||
var $Data = { code: -1 }; | ||
for (var index = 0, length = this.$Maps.markers.length; index < length; index++) { | ||
if (this.$Maps.markers[index]['name'] == name) { | ||
$Data = { code: index, $E: this.$Maps.markers[index] }; | ||
break; | ||
} | ||
} | ||
return $Data; | ||
}; | ||
/** | ||
* Assign _$Marker service into map's object. | ||
*/ | ||
$Maps._$Marker = { | ||
get: function(name) { | ||
return $RefMaps.searchMarkers(name); | ||
} | ||
}; | ||
$Maps._$Polyline = { | ||
/** | ||
* Draws the polyline into the map. | ||
*/ | ||
draw: function() { | ||
}, | ||
/** | ||
* Processes steps object. | ||
* | ||
* @param Array $Steps [{}, {}] | ||
* | ||
* @return Array $Paths [{lat: , lng: }, {lat: , lng: }] | ||
*/ | ||
processingSteps: function($Steps) { | ||
var $Steps = $Steps || undefined, | ||
$Paths = []; | ||
if (!$Steps.length) throw new Error("$Steps can't be empty / undefined"); | ||
for (var index = 0, length = $Steps.length; index < length; index++) { | ||
var $Path = (typeof $Steps[index] == 'object') ? $Steps[index]['path'] : undefined; | ||
if (!$Path) throw new Error("$Path must be object"); | ||
for (var index2 = 0, length2 = $Path.length; index2 < length2; index2++) { | ||
$Paths.push({ | ||
lat: (typeof $Path[index2]['lat'] == 'function') ? $Path[index2]['lat']() : $Path[index2]['lat'] || 0, | ||
lng: (typeof $Path[index2]['lng'] == 'function') ? $Path[index2]['lng']() : $Path[index2]['lng'] || 0, | ||
}); | ||
} | ||
} | ||
return $Paths; | ||
}, | ||
/** | ||
* Gets the paths from the $Directions. | ||
* | ||
* @param Object $Directions | ||
* @param String latlng {32.49129291,8.23124124} | ||
* | ||
* @return Array $Paths. | ||
*/ | ||
getPaths: function($Directions, latlng) { | ||
var $Routes = $Directions.routes; | ||
if (!$Routes.length) throw new Error("Routes can't be empty / undefined @ getPaths method"); | ||
var $Legs = ($Routes[0]) ? $Routes[0].legs : undefined; | ||
if (!$Legs.length) throw new Error("Legs can't be empty / undefined @ getPaths method"); | ||
for (var index = 0 , length = $Legs.length; index < length; index++) { | ||
var $StartLocation = $Legs[index]['start_location'] || {}; | ||
if ('start_location' in $Legs[index]) { | ||
var lat = (typeof $StartLocation.lat == 'function') ? $StartLocation.lat() : $StartLocation.lat || 0, | ||
lng = (typeof $StartLocation.lng == 'function') ? $StartLocation.lng() : $StartLocation.lng || 0; | ||
if (latlng == (lat + ',' + lng)) { | ||
console.log("Matched"); | ||
console.log("Do Something"); | ||
return this.processingSteps($Legs[index]['steps']); | ||
} | ||
} | ||
} | ||
}, | ||
/** | ||
* Gets the polyline's object. | ||
*/ | ||
getPolyline: function(name) { | ||
var $Data = { code: -1 }; | ||
if ($Maps.O$polyline) { | ||
if ($Maps.O$polyline[name]) $Data = { code: 1, $E: $Maps.O$polyline[name] }; | ||
} | ||
return $Data; | ||
}, | ||
/** | ||
* Clears the polyline from the map. | ||
* | ||
* @param Object $Polyline. | ||
*/ | ||
clearPolyline: function($Polyline) { | ||
var $Polyline = $Polyline || {}; | ||
if ('setMap' in $Polyline && typeof $Polyline.setMap == 'function') $Polyline.setMap(null); | ||
}, | ||
/** | ||
* Draws the polyline into the map. | ||
* | ||
*/ | ||
addPolyline: function($Configs) { | ||
if ($Maps['O$polyline'][$Configs['name']]) { | ||
$Maps['O$polyline'][$Configs['name']].setMap(null); | ||
delete $Maps['O$polyline'][$Configs['name']]; | ||
} | ||
var $Configs = $Configs || [], | ||
$PathPolyline = new google.maps.Polyline({ | ||
path: $Configs['paths'] || [], | ||
geodesic: $Configs['geodesic'] || true, | ||
strokeColor: $Configs['strokeColor'] || '#19CA93', | ||
strokeOpacity: $Configs['strokeOpacity'] || 1, | ||
strokeWeight: $Configs['strokeWeight'] || 4, | ||
map: $Maps | ||
}); | ||
if ($Configs['name']) $Maps['O$polyline'][$Configs['name']] = $PathPolyline; | ||
return $PathPolyline; | ||
} | ||
}; | ||
} | ||
function initMapModel() { | ||
@@ -301,2 +477,3 @@ | ||
this.map.location = {}; | ||
this.map.O$polyline = {}; | ||
this.map.addingMarker = addingMarker; | ||
@@ -321,2 +498,3 @@ this.map.ondirectionchange = this.ondirectionchange || undefined; | ||
attachMapEvents.call(this); | ||
ExtendsMaps(this.map); | ||
@@ -323,0 +501,0 @@ } |
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
580430
1220
17