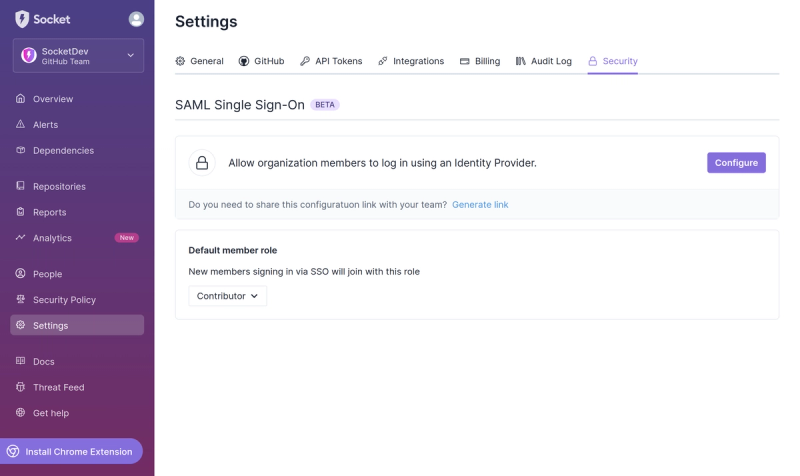
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
angular-html-parser
Advanced tools
Changelog
15.2.0 (2023-02-22)
InjectionToken
guards and resolvers are
deprecated. Instead, write guards as plain JavaScript functions and
inject dependencies with inject
from @angular/core
.| Commit | Type | Description | | -- | -- | -- | | 926c35f4ac | docs | Deprecate class and InjectionToken and resolvers (#47924) |
| Commit | Type | Description | | -- | -- | -- | | 54b24eb40f | feat | Add loaderParams attribute to NgOptimizedImage (#48907) |
| Commit | Type | Description | | -- | -- | -- | | 0cf11167f1 | fix | incorrectly detecting forward refs when symbol already exists in file (#48988) |
| Commit | Type | Description | | -- | -- | -- | | a154db8a81 | feat | add ng generate schematic to convert declarations to standalone (#48790) | | 345e737daa | feat | add ng generate schematic to convert to standalone bootstrapping APIs (#48848) | | e7318fc758 | feat | add ng generate schematic to remove unnecessary modules (#48832) |
| Commit | Type | Description | | -- | -- | -- | | 4ae384fd61 | feat | Allow auto-imports of a pipe via quick fix when its selector is used, both directly and via reexports. (#48354) | | 141333411e | feat | Introduce a new NgModuleIndex, and use it to suggest re-exports. (#48354) | | d0145033bd | fix | generate forwardRef for same file imports (#48898) |
| Commit | Type | Description |
| -- | -- | -- |
| 2796230e95 | fix | add enum
in mode
option in standalone
schema (#48851) |
| 816e76a578 | fix | automatically prune root module after bootstrap step (#49030) |
| bdbf21d04b | fix | avoid generating imports with forward slashes (#48993) |
| 32cf4e5cb9 | fix | avoid internal modules when generating imports (#48958) |
| 521ccfbe6c | fix | avoid interrupting the migration if language service lookup fails (#49010) |
| a40cd47aa7 | fix | avoid modifying testing modules without declarations (#48921) |
| 1afa6ed322 | fix | don't add ModuleWithProviders to standalone test components (#48987) |
| c98c6a8452 | fix | don't copy animations modules into the imports of test components (#49147) |
| 8389557848 | fix | don't copy unmigrated declarations into imports array (#48882) |
| f82bdc4b01 | fix | don't delete classes that may provide dependencies transitively (#48866) |
| 759db12e0b | fix | duplicated comments on migrated classes (#48966) |
| ba38178d19 | fix | generate forwardRef for same file imports (#48898) |
| 03fcb36cfd | fix | migrate HttpClientModule to provideHttpClient() (#48949) |
| 2de6dae16d | fix | migrate RouterModule.forRoot with a config object to use features (#48935) |
| 770191cf1f | fix | migrate tests when switching to standalone bootstrap API (#48987) |
| c7926b5773 | fix | move standalone migrations into imports (#48987) |
| 65c74ed93e | fix | normalize paths to posix (#48850) |
| 6377487b1a | fix | only exclude bootstrapped declarations from initial standalone migration (#48987) |
| e9e4449a43 | fix | preserve tsconfig in standalone migration (#48987) |
| ffad1b49d9 | fix | reduce number of files that need to be checked (#48987) |
| ba7a757cc5 | fix | return correct alias when conflicting import exists (#49139) |
| 49a7c9f94a | fix | standalone migration incorrectly throwing path error for multi app projects (#48958) |
| 584976e6c8 | fix | support --defaults in standalone migration (#48921) |
| 03f47ac901 | fix | use consistent quotes in generated imports (#48876) |
| ebae506d89 | fix | use import remapper in root component (#49046) |
| 40c976c909 | fix | use NgForOf instead of NgFor (#49022) |
| 4ac25b2aff | perf | avoid re-traversing nodes when resolving bootstrap call dependencies (#49010) |
| 26cb7ab2e6 | perf | speed up language service lookups (#49010) |
| Commit | Type | Description | | -- | -- | -- | | bf4ad38117 | fix | remove styles from DOM of destroyed components (#48298) |
| Commit | Type | Description | | -- | -- | -- | | 25e220a23a | fix | avoid duplicate TransferState info after renderApplication call (#49094) |
| Commit | Type | Description | | -- | -- | -- | | 31b94c762f | feat | Add a withNavigationErrorHandler feature to provideRouter (#48551) | | dedac8d3f7 | feat | Add test helper for trigger navigations in tests (#48552) |
Alan Agius, Alex Castle, Alex Rickabaugh, Andrew Kushnir, Andrew Scott, Dylan Hunn, Ikko Eltociear Ashimine, Ilyass, Jessica Janiuk, Joey Perrott, John Manners, Kalbarczyk, Kristiyan Kostadinov, Matthieu Riegler, Paul Gschwendtner, Pawel Kozlowski, Virginia Dooley, Walid Bouguima, cexbrayat and mgechev
<!-- CHANGELOG SPLIT MARKER --><a name="15.1.5"></a>
Readme
An HTML parser extracted from Angular with some modifications
# using npm
npm install --save angular-html-parser
# using yarn
yarn add angular-html-parser
import {parse} from 'angular-html-parser';
const {rootNodes, errors} = parse(`
<!DOCTYPE html>
<html>
<head>
<title>Hello world!</title>
</head>
<body>
<div>Hello world!</div>
</body>
</html>
`);
declare function parse(input: string, options?: Options): ng.ParseTreeResult;
interface Options {
/**
* any element can self close
*
* defaults to false
*/
canSelfClose?: boolean;
/**
* support [`htm`](https://github.com/developit/htm) component closing tags (`<//>`)
*
* defaults to false
*/
allowHtmComponentClosingTags?: boolean;
/**
* do not lowercase tag names before querying their tag definitions
*
* defaults to false
*/
isTagNameCaseSensitive?: boolean;
/**
* customize tag content type
*
* defaults to the content type defined in the HTML spec
*/
getTagContentType?: (
tagName: string,
prefix: string,
hasParent: boolean,
attrs: Array<{prefix: string; name: string; value?: string | undefined}>
) => void | ng.TagContentType;
/**
* tokenize angular control flow block syntax
*/
tokenizeAngularBlocks?: boolean,
}
CDATA
nodeDocType
nodenameSpan
field to Element
and Attribute
Comment#sourceSpan
<!...>
, <?...>
)type
property to nodes# build
yarn run build
# test
yarn run test
MIT © Ika
FAQs
A HTML parser extracted from Angular with some modifications
The npm package angular-html-parser receives a total of 63,049 weekly downloads. As such, angular-html-parser popularity was classified as popular.
We found that angular-html-parser demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.