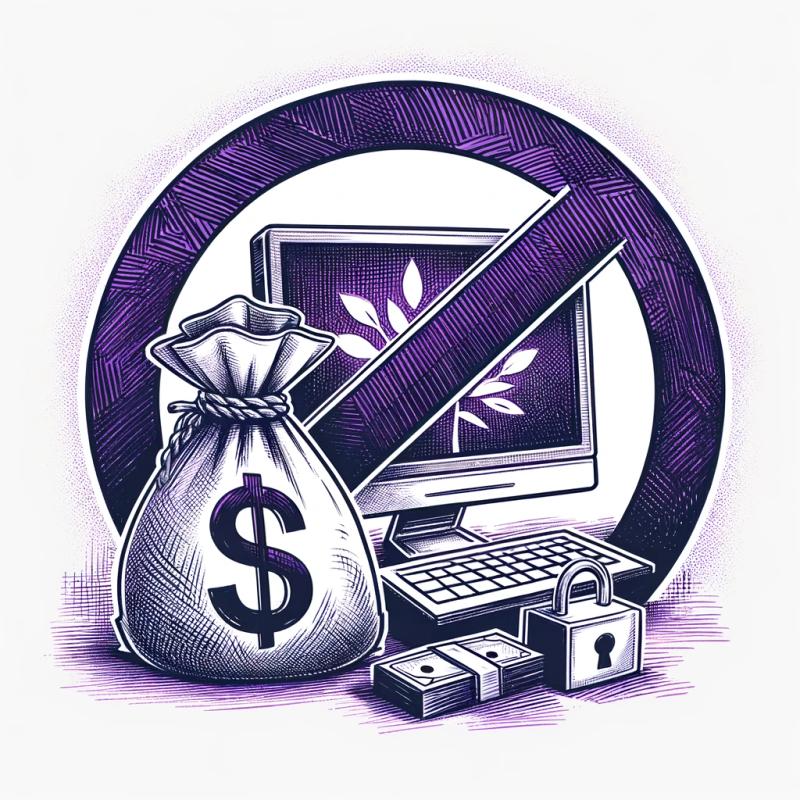
Security News
The Push to Ban Ransom Payments Is Gaining Momentum
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
angular-recaptcha3
Advanced tools
Readme
Angular v6+ integration with google recaptcha v3
Setup example:
const RECAPTCHA_OPTION = {
key: 'test-key',
size: 'invisible',
theme: 'light',
type: 'image',
tabindex: 0,
badge: 'bottomright',
language: 'ru',
show: true
}
Option description: https://developers.google.com/recaptcha/docs/display
const RECAPTCHA_LANGUAGE = 'ru'
Language codes: https://developers.google.com/recaptcha/docs/language
@NgModule({
imports: [
ReCaptchaModule.forRoot(RECAPTCHA_OPTION, RECAPTCHA_LANGUAGE)
]
})
To initialize the recaptcha you need to insert in the template
<recaptcha
[show]="false">
</recaptcha>
Recaptcha has parametrs:
reCAPTCHA v3 introduces a new concept: actions. When you specify an action name in each place you execute reCAPTCHA you enable two new features:
a detailed break-down of data for your top ten actions in the admin console adaptive risk analysis based on the context of the action (abusive behavior can vary) Importantly, when you verify the reCAPTCHA response you should also verify that the action name matches the one you expect.
constructor(
private recaptchaService: ReCaptchaService
) { }
// ...
recaptchaService.execute({action: 'homepage'}).then(token => {
//...
});
Note: actions may only contain alphanumeric characters and slashes, and must not be user-specific.
A more detailed description of ReCaptcha: https://developers.google.com/recaptcha/intro
FAQs
Angular v10+ integration with google recaptcha v3
The npm package angular-recaptcha3 receives a total of 382 weekly downloads. As such, angular-recaptcha3 popularity was classified as not popular.
We found that angular-recaptcha3 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Ransomware costs victims an estimated $30 billion per year and has gotten so out of control that global support for banning payments is gaining momentum.
Application Security
New SEC disclosure rules aim to enforce timely cyber incident reporting, but fear of job loss and inadequate resources lead to significant underreporting.
Security News
The Python Software Foundation has secured a 5-year sponsorship from Fastly that supports PSF's activities and events, most notably the security and reliability of the Python Package Index (PyPI).