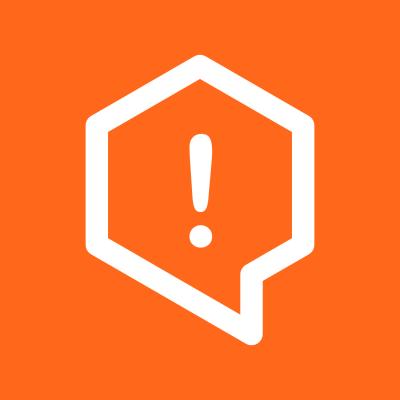
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
angular-webdav
Advanced tools
WebDAV library for Angular. The [WebDAV protocol](https://en.wikipedia.org/wiki/WebDAV) is an extension of the HTTP protocols with extra methods.
WebDAV library for Angular. The WebDAV protocol is an extension of the HTTP protocols with extra methods.
$ yarn add angular-webdav
and then from your Angular AppModule
:
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
import { WebDAVModule } from 'angular-webdav';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
WebDAVModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
import { Component } from '@angular/core';
import {WebDAV, Header} from 'angular-webdav';
@Component({
selector: 'my-app',
template: `<h1>Hello {{name}}</h1>`,
})
export class AppComponent { name = 'Angular';
constructor(private webdav: WebDAV){
const header = new Headers();
const url = 'https://your.url'
header.append('Authorization', 'Basic ' + btoa('username:password'));
//header.append('Destination', '${url}/newfile_directory');
//header.append('Depth', '300');
header.append('Content-Type', 'text/xml');
const body=`
<?xml version="1.0"?>
<d:propertyupdate xmlns:d="DAV:" xmlns:oc="http://owncloud.org/ns">
<d:set>
<d:prop>
<d:lastmodified>Fri, 13 Feb 2015 00:00:00 GMT</d:lastmodified>
</d:prop>
</d:set>
</d:propertyupdate>
`
// webdav.get(`${url}/Photos/Paris.jpg`, {headers: header})
// webdav.mkcol(`${url}/newdir`, {headers: header})
// webdav.move(`${url}/newdir`, {headers: header})
// webdav.copy(`${url}/newdir`, {headers: header})
// webdav.delete(`${url}/newdir2`, {headers: header})
// webdav.propfind(`${url}/`, {headers: header})
// webdav.put(`${url}/test`, body, {headers: header})
webdav.proppatch(`${url}/test`, body, {headers: header})
.subscribe();
}
}
To generate all *.js
, *.d.ts
and *.metadata.json
files:
$ git clone https://github.com/esanzgar/angular-webdav.git
$ cd angular-webdav
$ yarn
$ yarn build
To lint all *.ts
files:
$ yarn lint
Apache-2.0 © Eduardo Sanz García
FAQs
WebDAV library for Angular. The [WebDAV protocol](https://en.wikipedia.org/wiki/WebDAV) is an extension of the HTTP protocols with extra methods.
The npm package angular-webdav receives a total of 6 weekly downloads. As such, angular-webdav popularity was classified as not popular.
We found that angular-webdav demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.