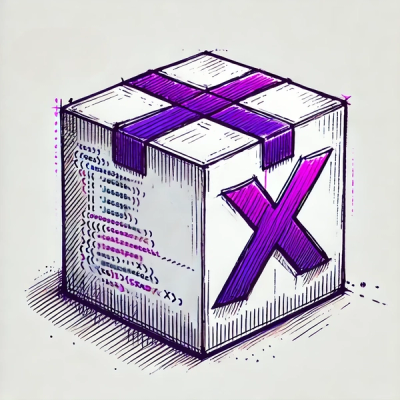
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
animazione
Advanced tools
simple easing animations using requestAnimationFrame
$ npm install --save animazione
<div class="box"></div>
const box = document.querySelector('.box');
const a = new Animazione({
render: renderLeft, // render function for each frame
target: box, // set the context to bind the render function to
duration: 400, // animation step duration
initialValue: 0, // initial value passed to render function
endValue: 500, // end value to be reached in the render function
easing: t => -0.5 * (Math.cos(Math.PI*t) - 1), // easing function
onComplete: () => { console.log('first finished') }, // callback on animation step completed
}).andThen({ // multiple steps can be chained
render: renderTop,
duration: 400,
initialValue: 0,
endValue: 500,
onComplete: () => { console.log('second finished') }
})
.loop() // a set of step animations can be looped forever
.start(); // start the animation
function renderLeft(val) {
this.style['left'] = val + 'px';
}
function renderTop(val) {
this.style['top'] = val + 'px';
}
Create a new Animazione instance.
new Animazione(animation)
animation step
type: Object
render
{function} Render function
noop
target
{Any} Context for the render function
null
duration
{integer} Step duration
0
easing
{function} Easing function
t => t
(linear easing)initialValue
0
endValue
1
fps
60
loop
false
onComplete
noop
start()
Start the animation
andThen(animation)
Chain another animation step
animation
and animation stepwait(duration)
pause the animation
duration
{integer} pause duration in msloop()
Make the animation an infinite loop of all the currently defined steps
stop()
Stop the animationFAQs
simple easing animations using requestAnimationFrame
We found that animazione demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.