ansi-colors
Advanced tools
Comparing version 2.0.5 to 3.0.0
206
index.js
'use strict'; | ||
const util = require('util'); | ||
const ansiRegex = /\u001b\[[0-9]+m/ig; | ||
const colors = { enabled: true, visible: true, ansiRegex }; | ||
const symbols = process.platform === 'win32' ? { | ||
check: '√', | ||
cross: '×', | ||
info: 'i', | ||
line: '─', | ||
pointer: '>', | ||
pointerSmall: '»', | ||
question: '?', | ||
warning: '‼' | ||
} : { | ||
check: '✔', | ||
cross: '✖', | ||
info: 'ℹ', | ||
line: '─', | ||
pointer: '❯', | ||
pointerSmall: '›', | ||
question: '?', | ||
warning: '⚠' | ||
}; | ||
const colors = { enabled: true, visible: true }; | ||
const styles = colors.styles = {}; | ||
const styles = { | ||
// modifiers | ||
reset: [0, 0], | ||
bold: [1, 22], | ||
dim: [2, 22], | ||
italic: [3, 23], | ||
underline: [4, 24], | ||
inverse: [7, 27], | ||
hidden: [8, 28], | ||
strikethrough: [9, 29], | ||
function ansi(codes) { | ||
codes.open = `\u001b[${codes[0]}m`; | ||
codes.close = `\u001b[${codes[1]}m`; | ||
return codes; | ||
} | ||
// colors | ||
black: [30, 39], | ||
red: [31, 39], | ||
green: [32, 39], | ||
yellow: [33, 39], | ||
blue: [34, 39], | ||
magenta: [35, 39], | ||
cyan: [36, 39], | ||
white: [37, 39], | ||
gray: [90, 39], | ||
grey: [90, 39], | ||
function wrap(style, str, i) { | ||
let { open, close } = style; | ||
return open + (i > 0 ? str.split(close).join(open) : str) + close; | ||
} | ||
// bright colors | ||
blackBright: [90, 39], | ||
redBright: [91, 39], | ||
greenBright: [92, 39], | ||
yellowBright: [93, 39], | ||
blueBright: [94, 39], | ||
magentaBright: [95, 39], | ||
cyanBright: [96, 39], | ||
whiteBright: [97, 39], | ||
// background colors | ||
bgBlack: [40, 49], | ||
bgRed: [41, 49], | ||
bgGreen: [42, 49], | ||
bgYellow: [43, 49], | ||
bgBlue: [44, 49], | ||
bgMagenta: [45, 49], | ||
bgCyan: [46, 49], | ||
bgWhite: [47, 49], | ||
// bright background colors | ||
bgBlackBright: [100, 49], | ||
bgRedBright: [101, 49], | ||
bgGreenBright: [102, 49], | ||
bgYellowBright: [103, 49], | ||
bgBlueBright: [104, 49], | ||
bgMagentaBright: [105, 49], | ||
bgCyanBright: [106, 49], | ||
bgWhiteBright: [107, 49] | ||
}; | ||
const isString = val => val && typeof val === 'string'; | ||
const unstyle = val => isString(val) ? val.replace(ansiRegex, '') : val; | ||
const hasOpen = (input, open) => input.slice(0, open.length) === open; | ||
const hasClose = (input, close) => input.slice(-close.length) === close; | ||
const color = (str, style, hasNewline) => { | ||
const { open, close } = style; | ||
if (!(hasOpen(str, open) && hasClose(str, close))) { | ||
str = style.open + str.replace(style.closeRe, style.open) + style.close; | ||
} | ||
if (hasNewline) { | ||
return str.replace(/(\r?\n)/g, `${style.close}$1${style.open}`); | ||
} | ||
function style(input, stack) { | ||
let str = '' + input; | ||
let n = stack.length; | ||
let i = 0; | ||
while (n-- > 0) str = wrap(styles[stack[n]], str, i++); | ||
return str; | ||
}; | ||
function wrap(...args) { | ||
if (colors.visible === false) return ''; | ||
let str = util.format(...args); | ||
if (colors.enabled === false) return str; | ||
if (str.trim() === '') return str; | ||
const newline = str.includes('\n'); | ||
for (const key of this.stack) { | ||
str = color(str, styles[key], newline); | ||
} | ||
return str; | ||
} | ||
function style(stack) { | ||
const create = (...args) => wrap.call(create, ...args); | ||
create.stack = stack; | ||
Reflect.setPrototypeOf(create, colors); | ||
return create; | ||
} | ||
function define(name, codes) { | ||
styles[name] = ansi(codes); | ||
function decorate(style) { | ||
style.open = `\u001b[${style[0]}m`; | ||
style.close = `\u001b[${style[1]}m`; | ||
style.closeRe = new RegExp(`\\u001b\\[${style[1]}m`, 'g'); | ||
return style; | ||
} | ||
let color = input => { | ||
if (colors.enabled === false) return input; | ||
if (colors.visible === false) return ''; | ||
let stack = color.stack; | ||
color.stack = [name]; | ||
return style(input, stack); | ||
}; | ||
for (const key of Object.keys(styles)) { | ||
decorate(styles[key]); | ||
Reflect.defineProperty(colors, key, { | ||
Reflect.setPrototypeOf(color, colors); | ||
Reflect.defineProperty(colors, name, { | ||
configurable: true, | ||
get() { | ||
return style(this.stack ? this.stack.concat(key) : [key]); | ||
if (!this.stack) { | ||
color.stack = [name]; | ||
} else if (!this.stack.includes(name)) { | ||
color.stack = this.stack.concat(name); | ||
} | ||
return color; | ||
} | ||
@@ -132,9 +50,53 @@ }); | ||
colors.stripColor = colors.strip = colors.unstyle = unstyle; | ||
colors.styles = styles; | ||
colors.symbols = symbols; | ||
colors.ok = (...args) => { | ||
return colors.green(symbols.check) + ' ' + util.format(...args); | ||
}; | ||
define('reset', [0, 0]); | ||
define('bold', [1, 22]); | ||
define('dim', [2, 22]); | ||
define('italic', [3, 23]); | ||
define('underline', [4, 24]); | ||
define('inverse', [7, 27]); | ||
define('hidden', [8, 28]); | ||
define('strikethrough', [9, 29]); | ||
define('black', [30, 39]); | ||
define('red', [31, 39]); | ||
define('green', [32, 39]); | ||
define('yellow', [33, 39]); | ||
define('blue', [34, 39]); | ||
define('magenta', [35, 39]); | ||
define('cyan', [36, 39]); | ||
define('white', [37, 39]); | ||
define('gray', [90, 39]); | ||
define('grey', [90, 39]); | ||
define('bgBlack', [40, 49]); | ||
define('bgRed', [41, 49]); | ||
define('bgGreen', [42, 49]); | ||
define('bgYellow', [43, 49]); | ||
define('bgBlue', [44, 49]); | ||
define('bgMagenta', [45, 49]); | ||
define('bgCyan', [46, 49]); | ||
define('bgWhite', [47, 49]); | ||
define('blackBright', [90, 39]); | ||
define('redBright', [91, 39]); | ||
define('greenBright', [92, 39]); | ||
define('yellowBright', [93, 39]); | ||
define('blueBright', [94, 39]); | ||
define('magentaBright', [95, 39]); | ||
define('cyanBright', [96, 39]); | ||
define('whiteBright', [97, 39]); | ||
define('bgBlackBright', [100, 49]); | ||
define('bgRedBright', [101, 49]); | ||
define('bgGreenBright', [102, 49]); | ||
define('bgYellowBright', [103, 49]); | ||
define('bgBlueBright', [104, 49]); | ||
define('bgMagentaBright', [105, 49]); | ||
define('bgCyanBright', [106, 49]); | ||
define('bgWhiteBright', [107, 49]); | ||
// ansiRegex modified from node.js readline: https://git.io/fNWFP | ||
colors.ansiRegex = /[\u001b\u009b][[()#;?]*(?:[0-9]{1,4}(?:;[0-9]{0,4})*)?[0-9A-ORZcf-nqry=><]/g; | ||
colors.unstyle = val => typeof val === 'string' ? val.replace(colors.ansiRegex, '') : val; | ||
colors.symbols = require('./symbols'); | ||
module.exports = colors; |
{ | ||
"name": "ansi-colors", | ||
"description": "Easily add ANSI colors to your text and symbols in the terminal.", | ||
"version": "2.0.5", | ||
"description": "Easily add ANSI colors to your text and symbols in the terminal. A faster drop-in replacement for chalk, kleur and turbocolor (without the dependencies and rendering bugs).", | ||
"version": "3.0.0", | ||
"homepage": "https://github.com/doowb/ansi-colors", | ||
@@ -21,5 +21,7 @@ "author": "Brian Woodward (https://github.com/doowb)", | ||
"index.js", | ||
"symbols.js", | ||
"types/index.d.ts" | ||
], | ||
"main": "index.js", | ||
"types": "./types/index.d.ts", | ||
"engines": { | ||
@@ -34,3 +36,3 @@ "node": ">=6" | ||
"justified": "^1.0.1", | ||
"mocha": "^3.5.3", | ||
"mocha": "^5.2.0", | ||
"text-table": "^0.2.0" | ||
@@ -59,2 +61,3 @@ }, | ||
"bold", | ||
"clorox", | ||
"colors", | ||
@@ -69,2 +72,3 @@ "cyan", | ||
"italic", | ||
"kleur", | ||
"magenta", | ||
@@ -74,2 +78,3 @@ "red", | ||
"strikethrough", | ||
"turbocolor", | ||
"underline", | ||
@@ -79,3 +84,2 @@ "white", | ||
], | ||
"types": "./types/index.d.ts", | ||
"verb": { | ||
@@ -107,5 +111,7 @@ "toc": false, | ||
"colors", | ||
"supports-color" | ||
"kleur", | ||
"supports-color", | ||
"turbocolor" | ||
] | ||
} | ||
} |
295
README.md
# ansi-colors [](https://www.npmjs.com/package/ansi-colors) [](https://npmjs.org/package/ansi-colors) [](https://npmjs.org/package/ansi-colors) [](https://travis-ci.org/doowb/ansi-colors) [](https://ci.appveyor.com/project/doowb/ansi-colors) | ||
> Easily add ANSI colors to your text and symbols in the terminal. | ||
> Easily add ANSI colors to your text and symbols in the terminal. A faster drop-in replacement for chalk, kleur and turbocolor (without the dependencies and rendering bugs). | ||
@@ -15,4 +15,2 @@ Please consider following this project's author, [Brian Woodward](https://github.com/doowb), and consider starring the project to show your :heart: and support. | ||
ansi-colors is a Node.js library for adding colors to text in the terminal. A more performant drop-in replacement for chalk, with no dependencies. | ||
 | ||
@@ -22,14 +20,14 @@ | ||
* Minimal - No dependencies! ([chalk](https://github.com/chalk/chalk) has 7 dependencies in its tree) | ||
* Safe - Does not modify the `String.prototype` | ||
* [Fast](#benchmarks) - Loads 5x faster and renders styles 10x faster than [chalk](https://github.com/chalk/chalk). | ||
* [Conditional color support](#conditional-color-support) | ||
ansi-colors is _the fastest Node.js library for terminal styling_. A more performant drop-in replacement for chalk, with no dependencies. | ||
* _Blazing fast_ - The fastest terminal styling library in node.js (See [Beware of false claims!](#beware-of-false-claims))! | ||
* _Drop-in replacement_ for [chalk](https://github.com/chalk/chalk), [turbocolor](https://github.com/doowb/turbocolor), [kleur](https://github.com/lukeed/kleur), etc. | ||
* _No dependencies_ (Chalk has 7 dependencies in its tree!) | ||
* _Safe_ - Does not modify the `String.prototype` like [colors](https://github.com/Marak/colors.js). | ||
* Supports [nested colors](#nested-colors) | ||
* Supports [chained colors](#chained-colors)! | ||
* Includes commonly used [symbols](#symbols) | ||
* Exposes a method for [stripping ANSI codes](#strip-ansi-codes) | ||
* [printf-like](#printf-formatting) formatting | ||
* Supports [chained colors](#chained-colors) | ||
* [Toggle color support](#toggle-color-support) on or off. | ||
See a [comparison to other libraries](#comparison) | ||
## Usage | ||
@@ -48,15 +46,5 @@ | ||
## Features | ||
### Chained colors | ||
Colors take multiple arguments. | ||
```js | ||
console.log(c.red('Some', 'red', 'text', 'to', 'display')); | ||
``` | ||
### Chained styles | ||
Supports chained styles. | ||
```js | ||
console.log(c.bold.red('this is a bold red message')); | ||
@@ -69,6 +57,4 @@ console.log(c.bold.yellow.italic('this is a bold yellow italicized message')); | ||
### Nested styles | ||
### Nested colors | ||
Supports nested styles. | ||
```js | ||
@@ -84,3 +70,3 @@ // using template literals | ||
### Conditional color support | ||
### Toggle color support | ||
@@ -101,23 +87,5 @@ Easily enable/disable colors. | ||
## printf-like formatting | ||
Uses node's built-in [util.format()](https://nodejs.org/api/util.html#util_util_format_format_args) to achieve printf-like formatting. The first argument is a string containing zero or more placeholder tokens. Each placeholder token is replaced with the converted value from the corresponding argument. | ||
```js | ||
console.log(c.bold.red('%s:%s', 'foo', 'bar', 'baz')); | ||
``` | ||
 | ||
Even works with nested colors! | ||
```js | ||
console.log(c.bold('%s:%s:%s', 'foo', c.red('bar'), 'baz')); | ||
``` | ||
 | ||
## Strip ANSI codes | ||
Use the `.unstyle` method to manually strip ANSI codes from a string. | ||
Use the `.unstyle` method to strip ANSI codes from a string. | ||
@@ -133,161 +101,137 @@ ```js | ||
### Colors | ||
| Colors | Background Colors | Bright Colors | Bright Background Colors | | ||
| --- | --- | --- | --- | | ||
| black | bgBlack | blackBright | bgBlackBright | | ||
| red | bgRed | redBright | bgRedBright | | ||
| green | bgGreen | greenBright | bgGreenBright | | ||
| yellow | bgYellow | yellowBright | bgYellowBright | | ||
| blue | bgBlue | blueBright | bgBlueBright | | ||
| magenta | bgMagenta | magentaBright | bgMagentaBright | | ||
| cyan | bgCyan | cyanBright | bgCyanBright | | ||
| white | bgWhite | whiteBright | bgWhiteBright | | ||
| gray | | | | | ||
| grey | | | | | ||
* `black` | ||
* `blue` | ||
* `cyan` | ||
* `gray` (U.S.) and `grey` (everyone else) | ||
* `green` | ||
* `magenta` | ||
* `red` | ||
* `white` | ||
* `yellow` | ||
_(`gray` is the U.S. spelling, `grey` is more commonly used in the Canada and U.K.)_ | ||
### Bright colors | ||
### Style modifiers | ||
* `blueBright` | ||
* `cyanBright` | ||
* `greenBright` | ||
* `magentaBright` | ||
* `redBright` | ||
* `whiteBright` | ||
* `yellowBright` | ||
* dim | ||
* **bold** | ||
### Background colors | ||
* hidden | ||
* _italic_ | ||
* `bgBlack` | ||
* `bgBlue` | ||
* `bgCyan` | ||
* `bgGreen` | ||
* `bgMagenta` | ||
* `bgRed` | ||
* `bgWhite` | ||
* `bgYellow` | ||
* underline | ||
* inverse | ||
* ~~strikethrough~~ | ||
**Bright background colors** | ||
* reset | ||
* `bgBlackBright` | ||
* `bgBlueBright` | ||
* `bgCyanBright` | ||
* `bgGreenBright` | ||
* `bgMagentaBright` | ||
* `bgRedBright` | ||
* `bgWhiteBright` | ||
* `bgYellowBright` | ||
## Performance | ||
### Modifiers | ||
MacBook Pro, Intel Core i7, 2.3 GHz, 16 GB. | ||
* `bold` | ||
* `dim` | ||
* `hidden` | ||
* `inverse` | ||
* `italic` _(Not widely supported)_ | ||
**Libraries tested** | ||
* `reset` | ||
* `strikethrough` _(Not widely supported)_ | ||
* ansi-colors v3.0.0 | ||
* chalk v2.4.1 | ||
* turbocolor v2.4.5 | ||
* kleur v2.0.1 | ||
* `underline` | ||
### Beware of false claims! | ||
## Symbols | ||
You might have seen claims from [kleur](https://github.com/lukeed/kleur) or [turbocolor](https://github.com/jorgebucaran/turbocolor) that they are "faster than ansi-colors". Both libraries are unofficial forks of ansi-colors, and in an attempt to appear faster and differentiate from ansi-colors, _both libraries removed crucial code that was necessary for resetting chained colors_. | ||
A handful of common useful symbols are available on the `c.symbols` property. | ||
To illustrate the bug, simply do the following with `kleur` (as of v2.0.1): | ||
```js | ||
console.log(c.symbols); | ||
const kleur = require('kleur'); | ||
const red = kleur.bold.underline.red; | ||
console.log(kleur.bold('I should be bold and white')); | ||
const blue = kleur.underline.blue; | ||
console.log(kleur.underline('I should be underlined and white')); | ||
``` | ||
### Available symbols | ||
Same with `turbocolor` (as of v2.4.5): | ||
**Windows** | ||
```js | ||
const turbocolor = require('turbocolor'); | ||
const red = turbocolor.bold.underline.red; | ||
console.log(turbocolor.bold('I should be bold and white')); | ||
* check: `√` | ||
* cross: `×` | ||
* ellipsis: `'...` | ||
* info: `i` | ||
* line: `─` | ||
* pointer: `'>` | ||
* pointerSmall: `»` | ||
* question: `?` | ||
* questionSmall: `﹖` | ||
* warning: `‼` | ||
const blue = turbocolor.underline.blue; | ||
console.log(turbocolor.underline('I should be underlined and white')); | ||
``` | ||
**Other platforms** | ||
Both libraries render the following: | ||
* check: `✔` | ||
* cross: `✖` | ||
* ellipsis: `…` | ||
* info: `ℹ` | ||
* line: `─` | ||
* pointer: `❯` | ||
* pointerSmall: `›` | ||
* question: `?` | ||
* questionSmall: `﹖` | ||
* warning: `⚠` | ||
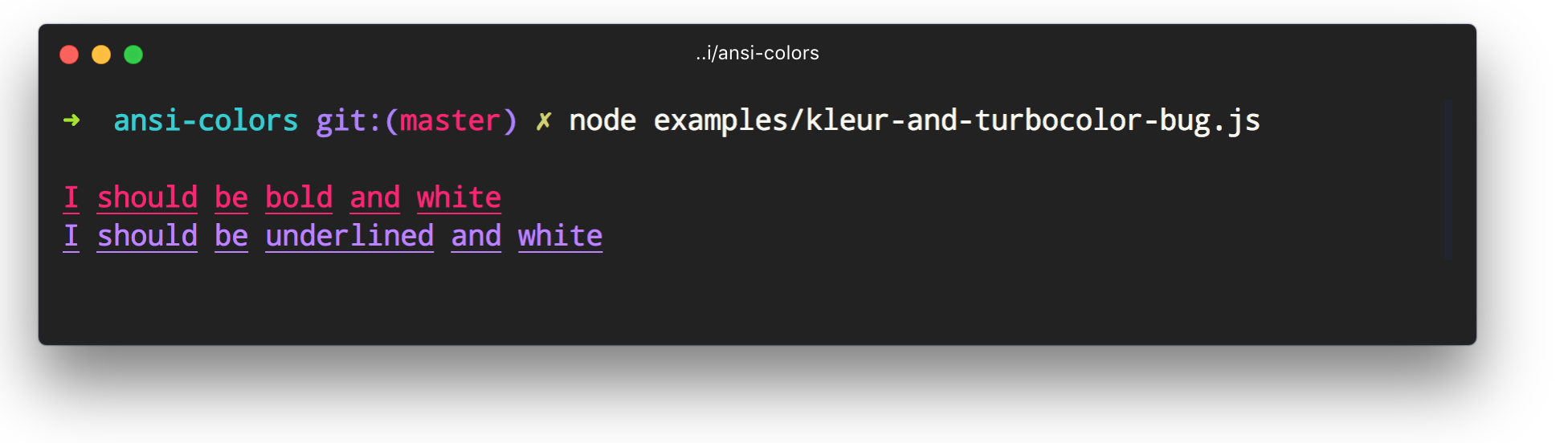 | ||
## Benchmarks | ||
**Other pitfalls** | ||
MacBook Pro, Intel Core i7, 2.3 GHz, 16 GB. | ||
Beyond the aforementioned rendering bug, neither kleur nor turbocolor can be used as a drop-in replacement for chalk: | ||
* both libraries fail half of the ansi-colors unit tests (chalk passes them all) | ||
* neither library supports bright colors (chalk and ansi-colors do) | ||
* neither library supports bright-background colors (chalk and ansi-colors do) | ||
* turbocolor swaps bright-background colors for background colors. (surprise! turbocolor gives you unexpected colors in the terminal!) | ||
### Load time | ||
Time it takes to load the module the first time: | ||
Time it takes to load the first time `require()` is called: | ||
``` | ||
chalk: 11.795ms | ||
clorox: 1.019ms | ||
ansi-colors: 0.867ms | ||
``` | ||
* ansi-colors - `0.794ms` | ||
* chalk - `13.158ms` | ||
* kleur - `1.699ms` | ||
* turbocolor - `5.352ms` | ||
### Performance | ||
### Benchmarks | ||
_(Note that both `kleur` (as of v2.0.1) and `turbocolor` (as of v2.4.5) have a [rendering bug](#beware-of-false-claims) that creates the illusion of appearing faster with stacked colors.)_ | ||
``` | ||
# All Colors | ||
ansi-colors x 104,559 ops/sec ±0.80% (91 runs sampled) | ||
chalk x 8,869 ops/sec ±2.09% (82 runs sampled) | ||
clorox x 1,315 ops/sec ±2.19% (76 runs sampled) | ||
ansi-colors x 370,403 ops/sec ±2.29% (88 runs sampled)) | ||
kleur x 203,945 ops/sec ±0.60% (92 runs sampled) | ||
turbocolor x 365,739 ops/sec ±1.15% (88 runs sampled) | ||
chalk x 9,472 ops/sec ±2.28% (81 runs sampled)) | ||
# Stacked colors | ||
ansi-colors x 36,208 ops/sec ±0.65% (88 runs sampled) | ||
chalk x 1,789 ops/sec ±1.77% (80 runs sampled) | ||
clorox x 528 ops/sec ±2.00% (78 runs sampled) | ||
# Chained colors | ||
ansi-colors x 21,671 ops/sec ±0.71% (89 runs sampled) | ||
kleur x 44,641 ops/sec ±0.87% (88 runs sampled) | ||
turbocolor x 53,270 ops/sec ±1.45% (87 runs sampled) | ||
chalk x 1,906 ops/sec ±2.11% (83 runs sampled) | ||
# Nested colors | ||
ansi-colors x 42,828 ops/sec ±0.36% (90 runs sampled) | ||
chalk x 4,082 ops/sec ±1.93% (83 runs sampled) | ||
clorox x 628 ops/sec ±2.10% (60 runs sampled) | ||
ansi-colors x 206,363 ops/sec ±2.03% (93 runs sampled) | ||
kleur x 75,443 ops/sec ±0.90% (92 runs sampled) | ||
turbocolor x 85,856 ops/sec ±0.24% (96 runs sampled) | ||
chalk x 4,029 ops/sec ±1.91% (82 runs sampled) | ||
``` | ||
## Comparison | ||
## About | ||
| **Feature** | **ansi-colors** | **chalk** | **clorox** | **colors** | | ||
| --- | --- | --- | --- | --- | | ||
| Nested colors | yes | yes | no | yes | | ||
| Chained colors | yes | yes | You must call `.toString()` on result | yes | | ||
| Toggle color support | yes | yes | no | yes | | ||
| printf-like formatting | yes | no | no | no | | ||
| Includes symbols | yes | no | no | no | | ||
<details> | ||
<summary><strong>Contributing</strong></summary> | ||
## About | ||
Pull requests and stars are always welcome. For bugs and feature requests, [please create an issue](../../issues/new). | ||
### Related projects | ||
</details> | ||
* [ansi-wrap](https://www.npmjs.com/package/ansi-wrap): Create ansi colors by passing the open and close codes. | [homepage](https://github.com/jonschlinkert/ansi-wrap "Create ansi colors by passing the open and close codes.") | ||
* [strip-color](https://www.npmjs.com/package/strip-color): Strip ANSI color codes from a string. No dependencies. | [homepage](https://github.com/jonschlinkert/strip-color "Strip ANSI color codes from a string. No dependencies.") | ||
<details> | ||
<summary><strong>Running Tests</strong></summary> | ||
### Contributing | ||
Running and reviewing unit tests is a great way to get familiarized with a library and its API. You can install dependencies and run tests with the following command: | ||
Pull requests and stars are always welcome. For bugs and feature requests, [please create an issue](../../issues/new). | ||
```sh | ||
$ npm install && npm test | ||
``` | ||
### Contributors | ||
</details> | ||
| **Commits** | **Contributor** | | ||
| --- | --- | | ||
| 21 | [doowb](https://github.com/doowb) | | ||
| 10 | [jonschlinkert](https://github.com/jonschlinkert) | | ||
| 6 | [lukeed](https://github.com/lukeed) | | ||
| 2 | [Silic0nS0ldier](https://github.com/Silic0nS0ldier) | | ||
| 1 | [chapterjason](https://github.com/chapterjason) | | ||
<details> | ||
<summary><strong>Building docs</strong></summary> | ||
### Building docs | ||
_(This project's readme.md is generated by [verb](https://github.com/verbose/verb-generate-readme), please don't edit the readme directly. Any changes to the readme must be made in the [.verb.md](.verb.md) readme template.)_ | ||
@@ -301,10 +245,22 @@ | ||
### Running tests | ||
</details> | ||
Running and reviewing unit tests is a great way to get familiarized with a library and its API. You can install dependencies and run tests with the following command: | ||
### Related projects | ||
```sh | ||
$ npm install && npm test | ||
``` | ||
You might also be interested in these projects: | ||
* [ansi-wrap](https://www.npmjs.com/package/ansi-wrap): Create ansi colors by passing the open and close codes. | [homepage](https://github.com/jonschlinkert/ansi-wrap "Create ansi colors by passing the open and close codes.") | ||
* [strip-color](https://www.npmjs.com/package/strip-color): Strip ANSI color codes from a string. No dependencies. | [homepage](https://github.com/jonschlinkert/strip-color "Strip ANSI color codes from a string. No dependencies.") | ||
### Contributors | ||
| **Commits** | **Contributor** | | ||
| --- | --- | | ||
| 32 | [doowb](https://github.com/doowb) | | ||
| 10 | [jonschlinkert](https://github.com/jonschlinkert) | | ||
| 6 | [lukeed](https://github.com/lukeed) | | ||
| 2 | [Silic0nS0ldier](https://github.com/Silic0nS0ldier) | | ||
| 1 | [madhavarshney](https://github.com/madhavarshney) | | ||
| 1 | [chapterjason](https://github.com/chapterjason) | | ||
### Author | ||
@@ -314,4 +270,5 @@ | ||
* [github/doowb](https://github.com/doowb) | ||
* [twitter/doowb](https://twitter.com/doowb) | ||
* [GitHub Profile](https://github.com/doowb) | ||
* [Twitter Profile](https://twitter.com/doowb) | ||
* [LinkedIn Profile](https://linkedin.com/in/woodwardbrian) | ||
@@ -325,2 +282,2 @@ ### License | ||
_This file was generated by [verb-generate-readme](https://github.com/verbose/verb-generate-readme), v0.7.0, on July 16, 2018._ | ||
_This file was generated by [verb-generate-readme](https://github.com/verbose/verb-generate-readme), v0.6.0, on August 16, 2018._ |
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
19857
6
255
273