What is ansi-colors?
The ansi-colors npm package is a utility for styling strings in the terminal with ANSI escape codes. It allows developers to add color and style to text output in command-line applications.
What are ansi-colors's main functionalities?
Text Colors
Apply text color to strings. The example shows how to make text red.
"const colors = require('ansi-colors');\nconsole.log(colors.red('This is red text'));"
Background Colors
Apply background color to strings. The example shows how to give text a red background.
"const colors = require('ansi-colors');\nconsole.log(colors.bgRed('This has a red background'));"
Text Styles
Apply text styles like bold, italic, underline, etc. The example shows how to make text bold.
"const colors = require('ansi-colors');\nconsole.log(colors.bold('This is bold text'));"
Chaining Styles
Chain multiple styles together. The example shows text that is blue, bold, and underlined.
"const colors = require('ansi-colors');\nconsole.log(colors.blue.bold.underline('This is blue, bold, and underlined'));"
Custom Themes
Create custom themes by combining styles. The example defines a custom theme with styles for error and warning messages.
"const colors = require('ansi-colors');\nconst customTheme = {\n error: colors.red.bold,\n warning: colors.yellow.italic\n};\nconsole.log(customTheme.error('Error message'));\nconsole.log(customTheme.warning('Warning message'));"
Other packages similar to ansi-colors
chalk
Chalk is a popular package similar to ansi-colors that provides a chainable API for styling strings. It offers a more extensive API and additional features like template literal support.
kleur
Kleur is a lightweight alternative to ansi-colors, focusing on performance. It has a similar API but does not support older versions of Node.js.
colorette
Colorette is another lightweight package for coloring terminal text. It aims to be fast and simple, with a minimalistic API compared to ansi-colors.
ansi-colors

Easily add ANSI colors to your text and symbols in the terminal. A faster drop-in replacement for chalk, kleur and turbocolor (without the dependencies and rendering bugs).
Please consider following this project's author, Brian Woodward, and consider starring the project to show your :heart: and support.
Install
Install with npm:
$ npm install --save ansi-colors

Why use this?
ansi-colors is the fastest Node.js library for terminal styling. A more performant drop-in replacement for chalk, with no dependencies.
Usage
const c = require('ansi-colors');
console.log(c.red('This is a red string!'));
console.log(c.green('This is a red string!'));
console.log(c.cyan('This is a cyan string!'));
console.log(c.yellow('This is a yellow string!'));

Chained colors
console.log(c.bold.red('this is a bold red message'));
console.log(c.bold.yellow.italic('this is a bold yellow italicized message'));
console.log(c.green.bold.underline('this is a bold green underlined message'));

Nested colors
console.log(c.yellow(`foo ${c.red.bold('red')} bar ${c.cyan('cyan')} baz`));
console.log(c.yellow('foo', c.red.bold('red'), 'bar', c.cyan('cyan'), 'baz'));

Toggle color support
Easily enable/disable colors.
const c = require('ansi-colors');
c.enabled = false;
c.enabled = require('color-support').stdout;
console.log(c.red('I will only be colored red if the terminal supports colors'));
Strip ANSI codes
Use the .unstyle
method to strip ANSI codes from a string.
console.log(c.unstyle(c.blue.bold('foo bar baz')));
Available styles
Note that bright and bright-background colors are not always supported.
Colors | Background Colors | Bright Colors | Bright Background Colors |
---|
black | bgBlack | blackBright | bgBlackBright |
red | bgRed | redBright | bgRedBright |
green | bgGreen | greenBright | bgGreenBright |
yellow | bgYellow | yellowBright | bgYellowBright |
blue | bgBlue | blueBright | bgBlueBright |
magenta | bgMagenta | magentaBright | bgMagentaBright |
cyan | bgCyan | cyanBright | bgCyanBright |
white | bgWhite | whiteBright | bgWhiteBright |
gray | | | |
grey | | | |
(gray
is the U.S. spelling, grey
is more commonly used in the Canada and U.K.)
Style modifiers
-
dim
-
bold
-
hidden
-
italic
-
underline
-
inverse
-
strikethrough
-
reset
Performance
MacBook Pro, Intel Core i7, 2.3 GHz, 16 GB.
Libraries tested
- ansi-colors v3.0.0
- chalk v2.4.1
- turbocolor v2.4.5
- kleur v2.0.1
Beware of false claims!
You might have seen claims from kleur or turbocolor that they are "faster than ansi-colors". Both libraries are unofficial forks of ansi-colors, and in an attempt to appear faster and differentiate from ansi-colors, both libraries removed crucial code that was necessary for resetting chained colors.
To illustrate the bug, simply do the following with kleur
(as of v2.0.1):
const kleur = require('kleur');
const red = kleur.bold.underline.red;
console.log(kleur.bold('I should be bold and white'));
const blue = kleur.underline.blue;
console.log(kleur.underline('I should be underlined and white'));
Same with turbocolor
(as of v2.4.5):
const turbocolor = require('turbocolor');
const red = turbocolor.bold.underline.red;
console.log(turbocolor.bold('I should be bold and white'));
const blue = turbocolor.underline.blue;
console.log(turbocolor.underline('I should be underlined and white'));
Both libraries render the following:
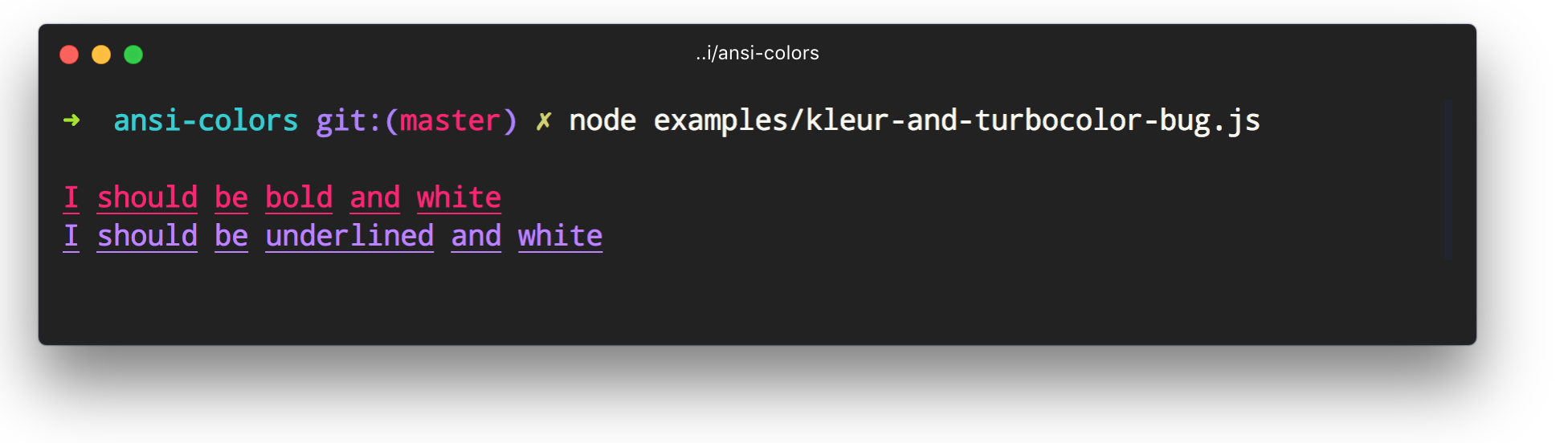
Other pitfalls
Beyond the aforementioned rendering bug, neither kleur nor turbocolor can be used as a drop-in replacement for chalk:
- both libraries fail half of the ansi-colors unit tests (chalk passes them all)
- neither library supports bright colors (chalk and ansi-colors do)
- neither library supports bright-background colors (chalk and ansi-colors do)
- turbocolor swaps bright-background colors for background colors. (surprise! turbocolor gives you unexpected colors in the terminal!)
Load time
Time it takes to load the first time require()
is called:
- ansi-colors -
0.794ms
- chalk -
13.158ms
- kleur -
1.699ms
- turbocolor -
5.352ms
Benchmarks
(Note that both kleur
(as of v2.0.1) and turbocolor
(as of v2.4.5) have a rendering bug that creates the illusion of appearing faster with stacked colors.)
# All Colors
ansi-colors x 370,403 ops/sec ±2.29% (88 runs sampled))
kleur x 203,945 ops/sec ±0.60% (92 runs sampled)
turbocolor x 365,739 ops/sec ±1.15% (88 runs sampled)
chalk x 9,472 ops/sec ±2.28% (81 runs sampled))
# Chained colors
ansi-colors x 21,671 ops/sec ±0.71% (89 runs sampled)
kleur x 44,641 ops/sec ±0.87% (88 runs sampled)
turbocolor x 53,270 ops/sec ±1.45% (87 runs sampled)
chalk x 1,906 ops/sec ±2.11% (83 runs sampled)
# Nested colors
ansi-colors x 206,363 ops/sec ±2.03% (93 runs sampled)
kleur x 75,443 ops/sec ±0.90% (92 runs sampled)
turbocolor x 85,856 ops/sec ±0.24% (96 runs sampled)
chalk x 4,029 ops/sec ±1.91% (82 runs sampled)
About
Contributing
Pull requests and stars are always welcome. For bugs and feature requests, please create an issue.
Running Tests
Running and reviewing unit tests is a great way to get familiarized with a library and its API. You can install dependencies and run tests with the following command:
$ npm install && npm test
Building docs
(This project's readme.md is generated by verb, please don't edit the readme directly. Any changes to the readme must be made in the .verb.md readme template.)
To generate the readme, run the following command:
$ npm install -g verbose/verb
Related projects
You might also be interested in these projects:
Contributors
Author
Brian Woodward
License
Copyright © 2018, Brian Woodward.
Released under the MIT License.
This file was generated by verb-generate-readme, v0.6.0, on August 16, 2018.