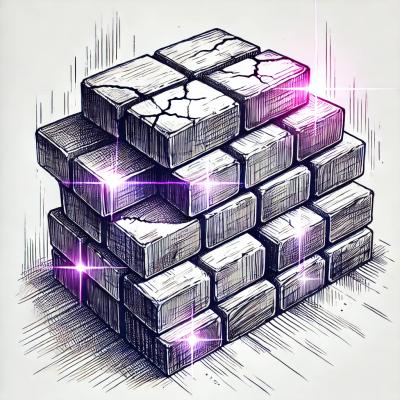
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
apidoc-mock
Advanced tools
Tired of overthinking mock solutions, use apidoc styled comments on your local files to create a mock NodeJS server.
You can serve up exactly what you need. Everything from a 200 status, to forcing a random custom status. You can even force a delay to see how your codebase will handle loading scenarios with a balls slow API response.
The basic requirements:
Generate a "happy path" mock server from apidoc @apiSuccessExample
annotations. Once the
server is set up correctly you should be able to update your code comments/annotations and have the mock(s) update with a
browser refresh.
NPM install...
$ npm i apidoc-mock
$ mock --help
Create a mock server from apiDoc comments.
Usage: mock [options]
Options:
-d, --docs Output directory used to compile apidocs [default: "./.docs"]
-p, --port Set mock port [default: 8000]
-s, --silent Silence apiDoc's output warnings, errors
[boolean] [default: true]
-w, --watch Watch single, or multiple directories
-h, --help Show help [boolean]
-v, --version Show version number [boolean]
If you have a project, you could setup a NPM script to do the following
$ mock -p 5000 -w src/yourDirectory -w src/anotherDirectory
Then follow the guide for apidoc. From there run the NPM script, and open localhost:5000/[PATH TO API ENDPOINT].
It's recommended you make sure to .gitignore
the .docs
directory that gets generated for apidocs
.
Since apidoc-mock
now runs locally we consider our Dockerfile
to be less of a focus and now in maintenance mode.
We no longer support an apidoc-mock
image hosted on dockerhub
or
Quay.io
which means you will either have to build your own container
image or use one of the existing older versions of apidoc-mock
still being hosted.
Docker has a beginner breakdown for image build and container run guide
The apidoc-mock
image comes preloaded with a "hello/world" example...
First, download the repository
Confirm Docker
, or an aliased version of podman
, is running
Next, open a terminal up and $ cd
into the local repository
Then, build the image
$ docker build -t apidoc-mock .
Then, run the container
$ docker run -d --rm -p 8000:8000 --name mock-api apidoc-mock && docker ps
Finally, you should be able to navigate to
To stop everything type...
$ docker stop mock-api
Using ApiDocs
The v0.5X.X+ range of ApiDocs, now, requires the description with its updated template (i.e.
@api {get} /hello/world/ [a description]
) if you want the docs to display. If you don't use that aspect of this package you can continue to leave it out.
Setup your API annotations first. @apiSuccessExample
is the only apiDoc example currently implemented.
/**
* @api {get} /hello/world/ Get
* @apiGroup Hello World
* @apiSuccess {String} foo
* @apiSuccess {String} bar
* @apiSuccessExample {json} Success-Response:
* HTTP/1.1 200 OK
* {
* "foo": "hello",
* "bar": "world"
* }
*/
const getExample = () => {};
/**
* @api {post} /hello/world/ Post
* @apiGroup Hello World
* @apiHeader {String} Authorization Authorization: Token AUTH_TOKEN
* @apiSuccess {String} foo
* @apiSuccess {String} bar
* @apiSuccessExample {json} Success-Response:
* HTTP/1.1 200 OK
* {
* "foo": "hello",
* "bar": "world"
* }
*/
const postExample = () => {};
Next
Then, make sure to .gitignore
the .docs
directory that gets generated for apidocs
.
Then, setup your NPM scripts
"scripts": {
"mock": "mock -p 5000 -w [PATH TO YOUR JS FILES] -w [ANOTHER PATH TO YOUR JS FILES]"
}
And then run your script
$ npm run mock
Make sure Docker
, or podman
, is running, and you've created a local image from the repository called apidoc-mock
. After that setup is something like...
"scripts": {
"mock:run": "docker stop mock-api-test; docker run -i --rm -p [YOUR PORT]:8000 -v \"$(pwd)[PATH TO YOUR JS FILES]:/app/data\" --name mock-api-test apidoc-mock",
"mock:stop": "docker stop mock-api-test"
}
You'll need to pick a port like... -p 8000:8000
and a directory path to pull the apiDoc code comments/annotations from... -v \"$(pwd)/src:/app/data\"
.
Then, run your scripts
$ npm run mock:setup
$ npm run mock:run
Finally, navigate to
the docs, http://localhost:[YOUR PORT]/docs/
the api, http://localhost:[YOUR PORT]/[PATH TO API ENDPOINT]
Apidoc Mock adds in a few different custom flags to help you identify or demonstrate API responses
Get random responses from both success
and error
examples with the @apiMock {RandomResponse}
annotation
/**
* @api {get} /hello/world/ Get
* @apiGroup Hello World
* @apiMock {RandomResponse}
* @apiSuccess {String} foo
* @apiSuccess {String} bar
* @apiSuccessExample {json} Success-Response:
* HTTP/1.1 200 OK
* {
* "foo": "hello",
* "bar": "world"
* }
* @apiSuccessExample {json} Success-Response:
* HTTP/1.1 200 OK
* {
* "lorem": "dolor",
* "ipsum": "est"
* }
* @apiError {String} bad
* @apiError {String} request
* @apiErrorExample {json} Error-Response:
* HTTP/1.1 400 OK
* {
* "bad": "hello",
* "request": "world"
* }
*/
const getExample = () => {};
Get a random success
response with the @apiMock {RandomSuccess}
annotation. Or get a random error
with the @apiMock {RandomError}
annotation
/**
* @api {get} /hello/world/ Get
* @apiGroup Hello World
* @apiMock {RandomSuccess}
* @apiSuccess {String} foo
* @apiSuccess {String} bar
* @apiSuccessExample {json} Success-Response:
* HTTP/1.1 200 OK
* {
* "foo": "hello",
* "bar": "world"
* }
* @apiSuccessExample {json} Success-Response:
* HTTP/1.1 200 OK
* {
* "lorem": "dolor",
* "ipsum": "est"
* }
* @apiError {String} bad
* @apiError {String} request
* @apiErrorExample {json} Error-Response:
* HTTP/1.1 400 OK
* {
* "bad": "hello",
* "request": "world"
* }
*/
const getExample = () => {};
Force a specific response status with the @apiMock {ForceStatus} [STATUS GOES HERE]
annotation. If you use a status without a supporting example the response status is still forced, but with fallback content.
/**
* @api {get} /hello/world/ Get
* @apiGroup Hello World
* @apiMock {ForceStatus} 400
* @apiSuccess {String} foo
* @apiSuccess {String} bar
* @apiSuccessExample {json} Success-Response:
* HTTP/1.1 200 OK
* {
* "foo": "hello",
* "bar": "world"
* }
* @apiError {String} bad
* @apiError {String} request
* @apiErrorExample {json} Error-Response:
* HTTP/1.1 400 OK
* {
* "bad": "hello",
* "request": "world"
* }
*/
const getExample = () => {};
Delay a response status with the @apiMock {DelayResponse} [MILLISECONDS GO HERE]
annotation.
/**
* @api {get} /hello/world/ Get
* @apiGroup Hello World
* @apiMock {DelayResponse} 3000
* @apiSuccess {String} foo
* @apiSuccess {String} bar
* @apiSuccessExample {json} Success-Response:
* HTTP/1.1 200 OK
* {
* "foo": "hello",
* "bar": "world"
* }
* @apiError {String} bad
* @apiError {String} request
* @apiErrorExample {json} Error-Response:
* HTTP/1.1 400 OK
* {
* "bad": "hello",
* "request": "world"
* }
*/
const getExample = () => {};
Contributing? Guidelines can be found here CONTRIBUTING.md.
5.0.0 (2024-06-14)
⚠ BREAKING CHANGES
FAQs
Create a mock server from apiDoc comments.
The npm package apidoc-mock receives a total of 27 weekly downloads. As such, apidoc-mock popularity was classified as not popular.
We found that apidoc-mock demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.