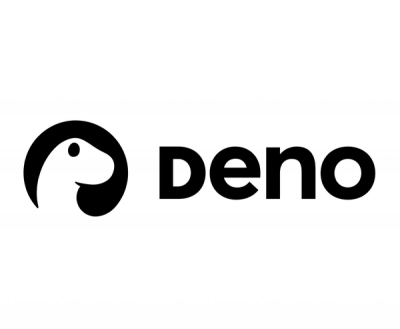
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
This is a fork of arale.class and arale.events. The core of arale projects.
The Class and Events of Arale is very easy to use, and stable now. Test cases with 97% coverage, and you don't have to worry about the 3% left.
Read it in Chinese at Arale.Class.
Class.create([parent], [properties])
create a new class:
/* pig.js */
var Class = require('arale').Class;
var Pig = Class.create({
initialize: function(name) {
this.name = name;
},
talk: function() {
console.log('I am' + this.name);
}
});
module.exports = Pig;
Every class created by Class.create
has an extend
method:
/* red-pig.js */
var Pig = require('./pig');
var RedPig = Pig.extend({
initialize: function(name) {
RedPig.superclass.initialize.call(this, name);
},
color: 'red'
});
module.exports = RedPig;
You can also mixin other classes:
/* flyable.js */
exports.fly = function() {
console.log('I am flying');
};
/* flyable-red-pig.js */
var RedPig = require('./red-pig');
var Flyable = require('./flyable');
var FlyableRedPig = RedPig.extend({
Implements: Flyable,
initialize: function(name) {
FlyableRedPig.superclass.initialize.call(this, name);
}
});
module.exports = FlyableRedPig;
The other way of mixin:
/* flyable-red-pig-extension.js */
var FlyableRedPig = require('./flyable-red-pig');
FlyableRedPig.implement({
swim: function() {
console.log('I can swim');
}
});
Transfrom a function to class:
function Animal() {
}
Animal.prototype.talk = function() {};
var Dog = Class(Animal).extend({
swim: function() {}
});
Read it in Chinese at Arale.Events.
There are two ways of implementing events:
var Events = require('arale').Events;
var object = new Events();
object.on('expand', function() {
console.log('expanded');
});
object.trigger('expand');
The other way:
var Events = require('arale').Events;
function Dog() {
}
Events.mixTo(Dog);
Dog.prototype.sleep = function() {
this.trigger('sleep');
};
var dog = new Dog();
dog.on('sleep', function() {
console.log('the dog is sleeping');
});
dog.sleep();
object.on(event, callback, [context])
You can pass a context to change the this.
post.on('saved', callback, that);
There is an all
event:
proxy.on('all', function(eventName) {
object.trigger(eventName);
});
object.off([event], [callback], [context])
// remove the onChange callback of change event
object.off('change', onChange);
// remove all callbacks of change event
object.off('change');
// remove all onChange callbacks of every event
object.off(null, onChange);
// remove all events for the context
object.off(null, null, context);
// remove all events of the object
object.off();
object.trigger(event, [*args])
var obj = new Events();
obj.on('x y', fn);
// equals:
obj.on('x').on('y');
FAQs
Arale Class and Events
We found that arale demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.