Comparing version 0.8.0 to 1.0.0
/// <reference types="node" /> | ||
import { RequestHandler } from 'fastify'; | ||
import { ServerResponse, IncomingMessage } from 'http'; | ||
interface IOpts { | ||
import { RequestHandler, Plugin } from 'fastify'; | ||
import { ServerResponse, IncomingMessage, Server } from 'http'; | ||
interface Opts { | ||
message?: string; | ||
@@ -12,3 +12,6 @@ readinessURL?: string; | ||
} | ||
export default function arecibo(fastify: any, opts: IOpts, next: (err?: Error) => void): void; | ||
export {}; | ||
interface Arecibo extends Plugin<Server, IncomingMessage, ServerResponse, Opts> { | ||
default: Arecibo; | ||
} | ||
declare const _default: Arecibo; | ||
export = _default; |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { | ||
value: true | ||
}); | ||
exports.default = arecibo; | ||
var _schema = require("./schema"); | ||
var __importDefault = (this && this.__importDefault) || function (mod) { | ||
return (mod && mod.__esModule) ? mod : { "default": mod }; | ||
}; | ||
const fastify_plugin_1 = __importDefault(require("fastify-plugin")); | ||
const schema_1 = require("./schema"); | ||
const READINESS_URL = '/arecibo/readiness'; | ||
@@ -87,26 +84,15 @@ const LIVENESS_URL = '/arecibo/liveness'; | ||
`; | ||
const defaultResponse = message => (_, reply) => { | ||
reply.type('text/html').send(message); | ||
const defaultResponse = (message) => (_, reply) => { | ||
reply.type('text/html').send(message); | ||
}; | ||
function arecibo(fastify, opts, next) { | ||
const _opts$message = opts.message, | ||
message = _opts$message === void 0 ? defaultMessage : _opts$message, | ||
_opts$readinessURL = opts.readinessURL, | ||
readinessURL = _opts$readinessURL === void 0 ? READINESS_URL : _opts$readinessURL, | ||
_opts$livenessURL = opts.livenessURL, | ||
livenessURL = _opts$livenessURL === void 0 ? LIVENESS_URL : _opts$livenessURL; | ||
const readinessCallback = opts.readinessCallback || defaultResponse(message); | ||
const livenessCallback = opts.livenessCallback || defaultResponse(message); | ||
fastify.get(readinessURL, { | ||
schema: _schema.readinessSchema | ||
}, readinessCallback); | ||
fastify.get(livenessURL, { | ||
schema: _schema.livenessSchema | ||
}, livenessCallback); | ||
next(); | ||
} | ||
module.exports = exports.default; | ||
const arecibo = fastify_plugin_1.default(function (fastify, opts, next) { | ||
const { message = defaultMessage, readinessURL = READINESS_URL, livenessURL = LIVENESS_URL, } = opts; | ||
const readinessCallback = opts.readinessCallback || defaultResponse(message); | ||
const livenessCallback = opts.livenessCallback || defaultResponse(message); | ||
fastify.get(readinessURL, { schema: schema_1.readinessSchema }, readinessCallback); | ||
fastify.get(livenessURL, { schema: schema_1.livenessSchema }, livenessCallback); | ||
next(); | ||
}, { fastify: '2.x' }); | ||
arecibo.default = arecibo; | ||
module.exports = arecibo; | ||
//# sourceMappingURL=index.js.map |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { | ||
value: true | ||
}); | ||
exports.livenessSchema = exports.readinessSchema = void 0; | ||
const readinessSchema = { | ||
description: 'The kubelet uses liveness probes to know when to restart a Container', | ||
tags: ['arecibo'], | ||
summary: 'Readiness Probe route', | ||
response: { | ||
200: { | ||
description: 'All Systems Ready!', | ||
type: 'string' | ||
} | ||
} | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
exports.readinessSchema = { | ||
description: 'The kubelet uses liveness probes to know when to restart a Container', | ||
tags: ['arecibo'], | ||
summary: 'Readiness Probe route', | ||
response: { | ||
200: { | ||
description: 'All Systems Ready!', | ||
type: 'string', | ||
}, | ||
}, | ||
}; | ||
exports.readinessSchema = readinessSchema; | ||
const livenessSchema = { | ||
description: 'The kubelet uses readiness probes to know when a Container is ready to start accepting traffic', | ||
tags: ['arecibo'], | ||
summary: 'Liveness Probe route', | ||
response: { | ||
200: { | ||
description: 'All Systems Ready!', | ||
type: 'string' | ||
} | ||
} | ||
exports.livenessSchema = { | ||
description: 'The kubelet uses readiness probes to know when a Container is ready to start accepting traffic', | ||
tags: ['arecibo'], | ||
summary: 'Liveness Probe route', | ||
response: { | ||
200: { | ||
description: 'All Systems Ready!', | ||
type: 'string', | ||
}, | ||
}, | ||
}; | ||
exports.livenessSchema = livenessSchema; | ||
//# sourceMappingURL=schema.js.map |
{ | ||
"name": "arecibo", | ||
"version": "0.8.0", | ||
"version": "1.0.0", | ||
"description": "Fastify plugin that respondes to kubernetes readiness and liveness probes.", | ||
"main": "./lib/index.js", | ||
"module": "./lib-es/index.js", | ||
"main": "./lib", | ||
"types": "./lib/index.d.ts", | ||
"scripts": { | ||
"type-check": "tsc -p ./tsconfig.json --noEmit", | ||
"clean-build": "rimraf ./lib ./lib-esm && mkdir lib lib-esm", | ||
"clean-build": "rimraf ./lib && mkdir lib", | ||
"prebuild": "npm-run-all clean-build lint type-check", | ||
"build": "npm run build-types && npm-run-all build-js build-esm", | ||
"build-types": "tsc --emitDeclarationOnly", | ||
"build-js": "babel ./src --out-dir lib --extensions \".ts,.tsx\" -s", | ||
"build-esm": "tsc -m es6 --outDir lib-esm --declaration false", | ||
"build": "tsc -p ./tsconfig.json", | ||
"prettier": "prettier --loglevel warn --write \"src/**/*.{ts,tsx}\"", | ||
@@ -43,18 +39,9 @@ "prelint": "npm run prettier", | ||
"devDependencies": { | ||
"@babel/cli": "^7.0.0", | ||
"@babel/core": "^7.0.0", | ||
"@babel/plugin-proposal-class-properties": "^7.0.0", | ||
"@babel/plugin-proposal-object-rest-spread": "^7.0.0", | ||
"@babel/plugin-transform-modules-commonjs": "^7.0.0", | ||
"@babel/preset-env": "^7.0.0", | ||
"@babel/preset-typescript": "^7.0.0", | ||
"@types/node": "^10.9.3", | ||
"babel-plugin-add-module-exports": "^1.0.0", | ||
"fastify": "^1.11.1", | ||
"npm-run-all": "^4.1.3", | ||
"prettier": "^1.14.2", | ||
"rimraf": "^2.6.2", | ||
"tslint": "^5.11.0", | ||
"tslint-config-prettier": "^1.15.0", | ||
"typescript": "^3.0.3" | ||
"@types/node": "10.9.3", | ||
"npm-run-all": "4.1.5", | ||
"prettier": "1.16.4", | ||
"rimraf": "2.6.3", | ||
"tslint": "5.13.0", | ||
"tslint-config-prettier": "^1.18.0", | ||
"typescript": "~3.3.3333" | ||
}, | ||
@@ -64,3 +51,7 @@ "files": [ | ||
"/lib-esm" | ||
] | ||
], | ||
"dependencies": { | ||
"fastify": "~2.0.0", | ||
"fastify-plugin": "~1.5.0" | ||
} | ||
} |
<div align="center"> | ||
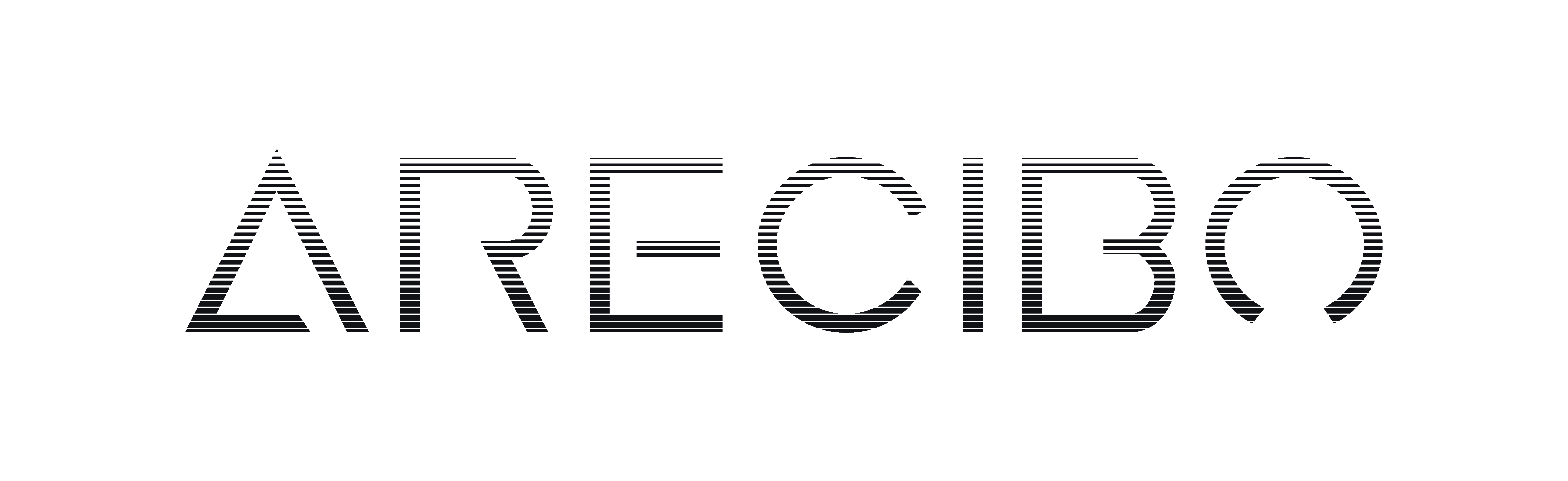 | ||
</div> | ||
@@ -10,3 +10,3 @@ | ||
[](https://www.npmjs.com/package/arecibo) | ||
[](https://www.npmjs.com/package/arecibo) | ||
[](https://www.npmjs.com/package/arecibo) | ||
[](https://github.com/prettier/prettier) | ||
@@ -18,2 +18,3 @@ [](https://snyk.io/test/github/nucleode/arecibo?targetFile=package.json) | ||
## Installation | ||
This module only supports 2.x.x versions of fastify. | ||
@@ -24,9 +25,11 @@ ```bash | ||
## Usage | ||
## Usage in Node.js | ||
```javascript | ||
const arecibo = require('arecibo') // or import * as arecibo from 'arecibo' | ||
const arecibo = require('arecibo') | ||
// or import arecibo from 'arecibo' | ||
// or import * as arecibo from 'arecibo' | ||
fastify.register(arecibo, { | ||
message: 'Put here your custom message', // optional, default to original arecibo message | ||
message: 'Put here your custom message', // optional, default to original arecibo message | ||
readinessURL: '/put/here/your/custom/url', // optional, deafult to /arecibo/readiness | ||
@@ -39,5 +42,8 @@ livenessURL: '/put/here/your/custom/url', // optional, deafult to /arecibo/liveness | ||
``` | ||
### Note for typescript users | ||
On Kubernetes add deployment manifest | ||
If you set `"esModuleInterop": true` you must import this module using `import arecibo from 'arecibo'`. | ||
## On Kubernetes add deployment manifest | ||
```yaml | ||
@@ -75,3 +81,3 @@ ... | ||
## Fun fact: where does the name come from? | ||
## Fun fact: where does the name come from? | ||
The name is inspired by the Arecibo message, a 1974 interstellar radio message carrying basic information about humanity and Earth sent to globular star cluster M13 in the hope that extraterrestrial intelligence might receive and decipher it. The message was broadcast into space a single time via frequency modulated radio waves at a ceremony to mark the remodelling of the Arecibo radio telescope in Puerto Rico on 16 November 1974. |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
No v1
QualityPackage is not semver >=1. This means it is not stable and does not support ^ ranges.
Found 1 instance in 1 package
7
0
79
11990
2
10
158
1
+ Addedfastify@~2.0.0
+ Addedfastify-plugin@~1.5.0
+ Addedabstract-logging@1.0.0(transitive)
+ Addedajv@6.12.6(transitive)
+ Addedarchy@1.0.0(transitive)
+ Addedatomic-sleep@1.0.0(transitive)
+ Addedavvio@6.5.0(transitive)
+ Addedbourne@1.3.3(transitive)
+ Addedcookie@0.4.2(transitive)
+ Addeddebug@4.4.0(transitive)
+ Addeddeepmerge@4.3.1(transitive)
+ Addedfast-decode-uri-component@1.0.1(transitive)
+ Addedfast-deep-equal@3.1.3(transitive)
+ Addedfast-json-stable-stringify@2.1.0(transitive)
+ Addedfast-json-stringify@1.21.0(transitive)
+ Addedfast-redact@2.1.0(transitive)
+ Addedfast-safe-stringify@2.1.1(transitive)
+ Addedfastify@2.0.1(transitive)
+ Addedfastify-plugin@1.5.0(transitive)
+ Addedfastq@1.19.0(transitive)
+ Addedfind-my-way@2.2.5(transitive)
+ Addedflatstr@1.0.12(transitive)
+ Addedforwarded@0.2.0(transitive)
+ Addedinherits@2.0.4(transitive)
+ Addedipaddr.js@1.9.1(transitive)
+ Addedjson-schema-traverse@0.4.1(transitive)
+ Addedlight-my-request@3.8.0(transitive)
+ Addedmiddie@4.1.0(transitive)
+ Addedms@2.1.3(transitive)
+ Addedpath-to-regexp@4.0.5(transitive)
+ Addedpino@5.17.0(transitive)
+ Addedpino-std-serializers@2.5.0(transitive)
+ Addedproxy-addr@2.0.7(transitive)
+ Addedpunycode@2.3.1(transitive)
+ Addedquick-format-unescaped@3.0.3(transitive)
+ Addedreadable-stream@3.6.2(transitive)
+ Addedret@0.2.2(transitive)
+ Addedreusify@1.0.4(transitive)
+ Addedsafe-buffer@5.2.1(transitive)
+ Addedsafe-regex2@2.0.0(transitive)
+ Addedsemver@5.7.2(transitive)
+ Addedsemver-store@0.3.0(transitive)
+ Addedset-cookie-parser@2.7.1(transitive)
+ Addedsonic-boom@0.7.7(transitive)
+ Addedstring-similarity@4.0.4(transitive)
+ Addedstring_decoder@1.3.0(transitive)
+ Addedtiny-lru@6.1.0(transitive)
+ Addeduri-js@4.4.1(transitive)
+ Addedutil-deprecate@1.0.2(transitive)