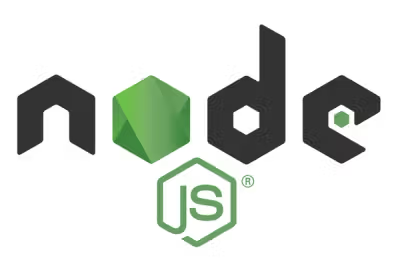
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Simple function for creating a string representation of an arbitrary javascript data value. Useful for printing bad argument values to console error output
$ npm install arginfo
Calling arginfo(arg)
returns a string representation of arg
using node.js' util.inspect
. It prefixes the value with the type of the argument (whether it is a primitive type or a built-in object) such that the formatting is consistent across all built-in types:
arginfo(2); // [number: 2]
arginfo({}); // [object: {}]
arginfo('?'); // [string: '?']
arginfo(true); // [boolean: true]
arginfo(/org/); // [RegExp: /org/]
arginfo(new Set()); // [Set: {}]
arginfo(new String('')); // [String: '']
arginfo(new Error('wtf')); // [Error: wtf]
var arginfo = require('arginfo');
function test(str) {
if('string' !== typeof str) {
console.error('test expects string. instead got: '+arginfo(str));
}
}
By default, arginfo will call util.inspect
with a depth
of null
. To limit the inspection depth of printing object properties, use arginfo.depth
. eg:
var arginfo = require('arginfo');
arginfo.depth(1);
arginfo({
a: {
b: {
c:'d'
}
}
}); // [object: { a: { b: [Object] } }]
Primitive types:
Built-in objects:
FAQs
Simple function for creating a string representation of an arbitrary javascript data value. Useful for printing bad argument values to console error output
We found that arginfo demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.