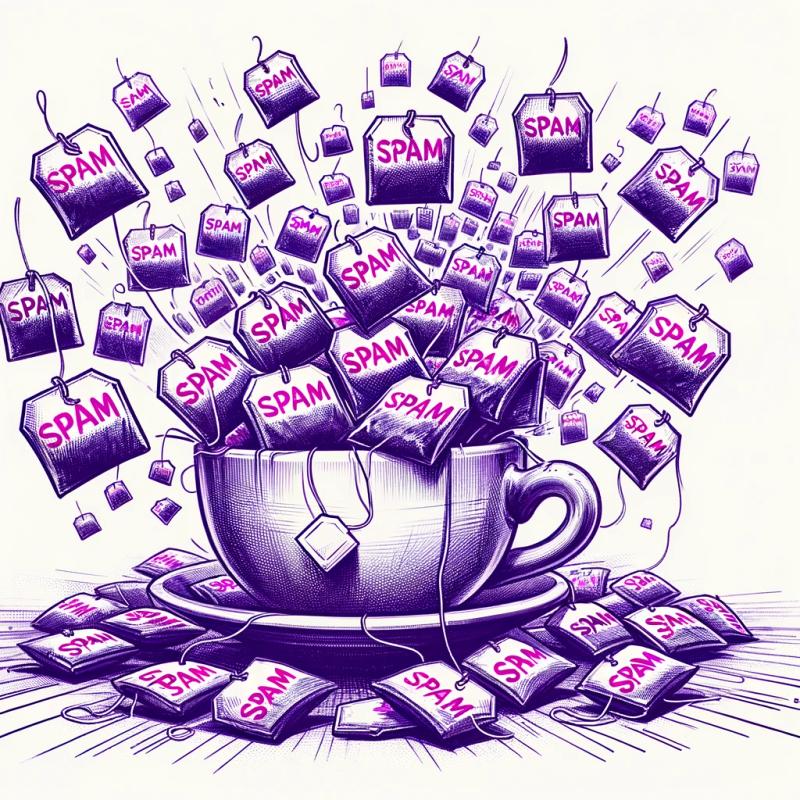
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
array-objectify
Advanced tools
Readme
Hierarchically transform an array of similar objects into a single object.
npm install --save array-objectify
output = arrayObjectify(hierarchy, data[, keepRepetetions])
hierarchy
is an array
: Determines the order of parsing.data
is an obect
: Defines the data we want to transform.keepRepetetions
is boolean
(optional, default value is false
): If this flag is set, child arrays of the result will contain the exact values gotten from the input data without checking for duplication. (look at the example)const arrayObjectify = require('array-objectify');
// this is the data we want to parse into new object format
const data = [
{
fruit: 'apple',
color: 'yellow',
content: 'Vitamin Y',
nickName: 'Y-diple'
},
{
fruit: 'apple',
color: 'yellow',
content: 'Vitamin X',
nickName: 'X-diple'
},
{
fruit: 'mango',
color: 'red',
content: 'Vitamin yellow',
nickName: 'yellow-mango'
},
{
fruit: 'apple',
color: 'green',
content: 'Vitamin green',
nickName: 'green-mango'
}
];
// now we've to mention the order of hierarchy in which array-objectify should parse the data
const hierarchy = ['fruit', 'color'];
// parse data in the order of given hierarchy
const objectified = arrayObjectify(hierarchy, data);
console.log(objectified);
/* output=>
{
"apple": {
"yellow": {
"content": [
"Vitamin Y",
"Vitamin X"
],
"nickName": [
"Y-diple",
"X-diple"
]
},
"green": {
"content": [
"Vitamin green"
],
"nickName": [
"green-mango"
]
}
},
"mango": {
"red": {
"content": [
"Vitamin yellow"
],
"nickName": [
"yellow-mango"
]
}
}
}
*/
output = arrayObjectify(hierarchy, data, keepRepetetions)
.
data
is the array
of objects
we want to convert.hierarchy
is an array
of hierarchically ordered object field names in which the package should parse the data.keepRepetetions
is Boolean
and this argument is optional. Default value is false
.hierarchy
will be the child of the last parent. Consider the below example:const data = [
{
fruit: 'apple',
color: 'yellow',
content: 'Vitamin Y',
nickName: 'Y-diple'
},
{
fruit: 'apple',
color: 'yellow',
content: 'Vitamin X',
nickName: 'X-diple'
},
{
fruit: 'mango',
color: 'red',
content: 'Vitamin yellow',
nickName: 'yellow-mango'
},
{
fruit: 'mango',
color: 'red',
content: 'Vitamin yellow',
nickName: 'yellow-mango-dupe'
},
{
fruit: 'apple',
color: 'green',
content: 'Vitamin green',
nickName: 'green-mango'
}
];
Let's say we want to covert this whole array into an object, based on the field name fruit
. And then each fruit need to be again ordered based on the field name color
. Rest of the fields names (content
and nickName
) are local to each colored fruit. So we should not want them to mention it on hierarchy
.
Thus the hierarchy goes like:
const hierarchy = ['fruit', 'color'];
Those fields which are not mentioned in the hierarchy
will come under the last hierarchical parent, in our case, the color
field.
Now the parsed output would be as follows:
const out = arrayObjectify(hierarchy, data); // keepRepetetions = fasle (default)
console.log(out);
output =>
{
"apple": {
"yellow": {
"content": ["Vitamin Y","Vitamin X"],
"nickName": ["Y-diple","X-diple"]
},
"green": {
"content": ["Vitamin green"],
"nickName": ["green-mango"]
}
},
"mango": {
"red": {
"content": ["Vitamin yellow"],
"nickName": ["yellow-mango","yellow-mango-dupe"]
}
}
}
Let's run this again with keepRepetetions
turned on, so that you can visually differentiate the action of keepRepetetions
flag:
let out = arrayObjectify(hierarchy, data, true);
console.log(out);
output =>
{
"apple": {
"yellow": {
"content": ["Vitamin Y","Vitamin X"],
"nickName": ["Y-diple","X-diple"]
},
"green": {
"content": ["Vitamin green"],
"nickName": ["green-mango"]
}
},
"mango": {
"red": {
"content": ["Vitamin yellow","Vitamin yellow"],
"nickName": ["yellow-mango","yellow-mango-dupe"]
}
}
}
Look at the mango
field: Vitamin yellow
is repeating. In some usecases, it may requre things in that way. It will not repeat if keepRepetetions
is turned off.
Still unclear? Please rise an issue.
Enjoy :)
MIT © Vajahath Ahmed
FAQs
Hierarchically transform an array of objects into a single object
The npm package array-objectify receives a total of 6 weekly downloads. As such, array-objectify popularity was classified as not popular.
We found that array-objectify demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.