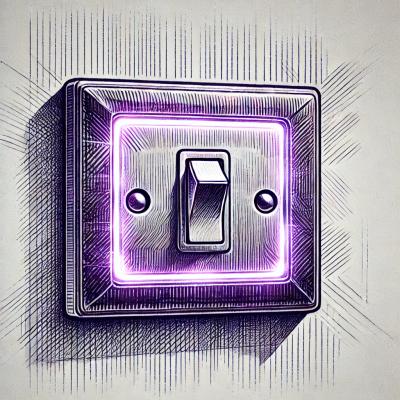
Research
Security News
Kill Switch Hidden in npm Packages Typosquatting Chalk and Chokidar
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
array-range
Advanced tools
The array-range npm package is a simple utility for generating arrays of numbers within a specified range. It is useful for creating sequences of numbers for various purposes, such as iteration, pagination, or testing.
Generate a range of numbers
This feature allows you to generate an array of numbers starting from a specified value up to, but not including, another specified value.
const range = require('array-range');
const numbers = range(1, 5); // [1, 2, 3, 4]
Generate a range with a step
This feature allows you to generate an array of numbers with a specified step value, providing more control over the sequence.
const range = require('array-range');
const numbers = range(0, 10, 2); // [0, 2, 4, 6, 8]
Generate a descending range
This feature allows you to generate a descending array of numbers by specifying a start value greater than the end value.
const range = require('array-range');
const numbers = range(5, 1); // [5, 4, 3, 2]
Lodash is a popular utility library that provides a wide range of functions for common programming tasks, including array manipulation. The `_.range` function in Lodash offers similar functionality to array-range, allowing you to generate arrays of numbers within a specified range with optional step values.
Underscore is another utility library that provides a variety of functions for working with arrays, objects, and other data types. The `_.range` function in Underscore is similar to the one in array-range, enabling you to create arrays of numbers within a specified range.
The range-generator package provides a simple and flexible way to generate ranges of numbers. It offers similar functionality to array-range, allowing you to create sequences of numbers with specified start, end, and step values.
Tiny module to create a new dense array with the specified range.
var range = require('array-range')
range(3) // -> [ 0, 1, 2 ]
range(1, 4) // -> [ 1, 2, 3 ]
Mainly useful for functional programming. ES6 examples:
var array = require('array-range')
array(5).map( x => x*x )
// -> [ 0, 1, 4, 9, 16 ]
array(2, 10).filter( x => x%2===0 )
// -> [ 2, 4, 6, 8 ]
It can also be useful for creating a fixed size dense array. Cleaner than apply
and does not create an intermediate array:
array(5)
//vs.
Array.apply(null, new Array(5))
array(start, end)
Creates a new dense array with a length of end-start
elements. start
is inclusive, end
is exclusive. Negative values also work, e.g. range(-10, 10)
array(len)
Creates a new dense array with len
number of elements, from zero to len-1
.
If len
is unspecified, it defaults to zero (empty array).
MIT, see LICENSE.md for details.
FAQs
creates a new array with given range
The npm package array-range receives a total of 117,965 weekly downloads. As such, array-range popularity was classified as popular.
We found that array-range demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.