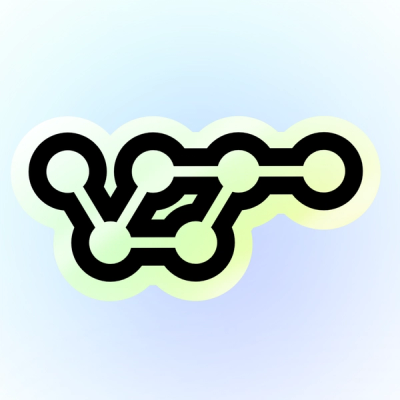
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
array-tools
Advanced tools
Useful functions for working with arrays
Example
var a = require("array-tools");
Array
Array.<object>
Array
boolean
Array
object
Array
Array
Array
Array
Array
Array
Array
Array
Plucks the value of the specified property from each object in the input array
Param | Type | Description |
---|---|---|
arrayOfObjects | Array.<object> | the input array of objects |
...property | string | the property(s) to pluck |
Example
> var data = [
... {one: 1, two: 2},
... {two: "two"},
... {one: "one", two: "zwei"},
... ];
undefined
> a.pluck(data, "one");
[ 1, 'one' ]
> a.pluck(data, "two");
[ 2, 'two', 'zwei' ]
> a.pluck(data, "one", "two");
[ 1, 'two', 'one' ]
Array.<object>
return a copy of the input arrayOfObjects
containing objects having only the cherry-picked properties
Param | Type | Description |
---|---|---|
arrayOfObjects | Array.<object> | the input |
...property | string | the properties to include in the result |
Example
> data = [
{ one: "un", two: "deux", three: "trois" },
{ two: "two", one: "one" },
{ four: "quattro" },
{ two: "zwei" }
]
> a.pick(data, "two")
[ { two: 'deux' },
{ two: 'two' },
{ two: 'zwei' } ]
Array
Takes input and guarantees an array back. Result can be one of three things:
arguments
) to a real arraynull
or undefined
to an empty arrayParam | Type | Description |
---|---|---|
input | * | the input value to convert to an array |
Example
> a.arrayify(null)
[]
> a.arrayify(0)
[ 0 ]
> a.arrayify([ 1, 2 ])
[ 1, 2 ]
> function f(){ return a.arrayify(arguments); }
undefined
> f(1,2,3)
[ 1, 2, 3 ]
boolean
returns true if a value, or nested object value exists in an array
Param | Type | Description |
---|---|---|
array | Array | the array to search |
value | * | the value to search for |
Example
> a.exists([ 1, 2, 3 ], 2)
true
> a.exists([ { result: false }, { result: false } ], { result: true })
false
> a.exists([ { result: true }, { result: false } ], { result: true })
true
> a.exists([ { result: true }, { result: true } ], { result: true })
true
Array
returns an array containing items from arrayOfObjects
where key/value pairs
from query
are matched identically
Param | Type | Description |
---|---|---|
arrayOfObjects | Array | the array to search |
query | query | an object containing the key/value pairs you want to match |
Example
> dudes = [{ name: "Jim", age: 8}, { name: "Clive", age: 8}, { name: "Hater", age: 9}]
[ { name: 'Jim', age: 8 },
{ name: 'Clive', age: 8 },
{ name: 'Hater', age: 9 } ]
> a.where(dudes, { age: 8})
[ { name: 'Jim', age: 8 },
{ name: 'Clive', age: 8 } ]
object
returns the first item from arrayOfObjects
where key/value pairs
from query
are matched identically
Param | Type | Description |
---|---|---|
arrayOfObjects | Array | the array to search |
query | query | an object containing the key/value pairs you want to match |
Example
> dudes = [{ name: "Jim", age: 8}, { name: "Clive", age: 8}, { name: "Hater", age: 9}]
[ { name: 'Jim', age: 8 },
{ name: 'Clive', age: 8 },
{ name: 'Hater', age: 9 } ]
> a.findWhere(dudes, { age: 8})
{ name: 'Jim', age: 8 }
Array
Returns the input minus the specified values.
Param | Type | Description |
---|---|---|
input | Array | the input array |
toRemove | * | a single, or array of values to omit |
Example
> a.without([ 1, 2, 3 ], 2)
[ 1, 3 ]
> a.without([ 1, 2, 3 ], [ 2, 3 ])
[ 1 ]
Array
merge two arrays into a single array of unique values
Param | Type | Description |
---|---|---|
array1 | Array | First array |
array2 | Array | Second array |
idKey | string | the unique ID property name |
Example
> var array1 = [ 1, 2 ], array2 = [ 2, 3 ];
undefined
> a.union(array1, array2)
[ 1, 2, 3 ]
> var array1 = [ { id: 1 }, { id: 2 } ], array2 = [ { id: 2 }, { id: 3 } ];
undefined
> a.union(array1, array2)
[ { id: 1 }, { id: 2 }, { id: 3 } ]
> var array2 = [ { id: 2, blah: true }, { id: 3 } ]
undefined
> a.union(array1, array2)
[ { id: 1 },
{ id: 2 },
{ id: 2, blah: true },
{ id: 3 } ]
> a.union(array1, array2, "id")
[ { id: 1 }, { id: 2 }, { id: 3 } ]
Array
Returns the initial elements which both input arrays have in common
Param | Type | Description |
---|---|---|
a | Array | first array to compare |
b | Array | second array to compare |
Example
> a.commonSequence([1,2,3], [1,2,4])
[ 1, 2 ]
Array
reduces an array to unique values
Param | Type | Description |
---|---|---|
array | Array | input array |
Example
> n = [1,6,6,7,1]
[ 1, 6, 6, 7, 1 ]
> a.unique(n)
[ 1, 6, 7 ]
Array
splice from index
until test
fails
Param | Type | Description |
---|---|---|
array | Array | the input array |
index | number | the position to begin splicing from |
test | RegExp | the test to continue splicing while true |
...elementN | * | the elements to add to the array |
Example
> letters = ["a", "a", "b"]
[ 'a', 'a', 'b' ]
> a.spliceWhile(letters, 0, /a/, "x")
[ 'a', 'a' ]
> letters
[ 'x', 'b' ]
Array
Removes items from array
which satisfy the query. Modifies the input array, returns the extracted.
Returns: Array
- the extracted items.
Param | Type | Description |
---|---|---|
array | Array | the input array, modified directly |
query | function | object | Per item in the array, if either the function returns truthy or the exists query is satisfied, the item is extracted |
Array
flatten an array of arrays into a single array
Since: 1.4.0
Todo
Example
> numbers = [ 1, 2, [ 3, 4 ], 5 ]
[ 1,
2,
[ 3, 4 ],
5 ]
> a.flatten(numbers)
[ 1, 2, 3, 4, 5 ]
documented by jsdoc-to-markdown.
© 2015 Lloyd Brookes 75pound@gmail.com
FAQs
Lightweight, use-anywhere toolkit for working with array data.
The npm package array-tools receives a total of 2,504 weekly downloads. As such, array-tools popularity was classified as popular.
We found that array-tools demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.