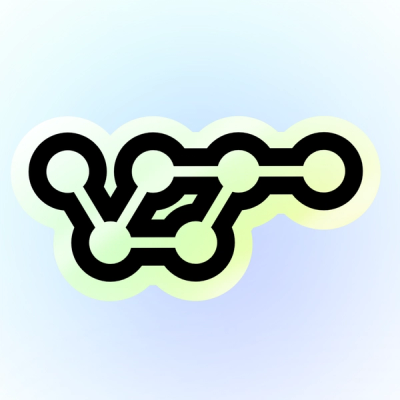
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Arweave JS is the JavaScript/TypeScript SDK for interacting with the Arweave network and uploading data ot the permaweb. It works in latest browsers and Node JS.
npm install --save arweave
Single bundle file (web only - use the NPM method if using Node).
<!-- Latest -->
<script src="https://unpkg.com/arweave/bundles/web.bundle.js"></script>
<!-- Latest, minified-->
<script src="https://unpkg.com/arweave/bundles/web.bundle.min.js"></script>
<!-- Specific version -->
<script src="https://unpkg.com/arweave@1.2.0/bundles/web.bundle.js"></script>
<!-- Specific version, minified -->
<script src="https://unpkg.com/arweave@1.2.0/bundles/web.bundle.min.js"></script>
const Arweave = require('arweave/node');
const instance = Arweave.init({
host: '127.0.0.1',
port: 1984
});
import Arweave from 'arweave/web';
const arweave = Arweave.init({
host: '127.0.0.1',
port: 1984
});
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Hello world</title>
<script src="https://unpkg.com/arweave/bundles/web.bundle.js"></script>
<script>
const arweave = Arweave.init({
host: '127.0.0.1',
port: 1984
});
arweave.network.getInfo().then(console.log);
</script>
</head>
<body>
</body>
</html>
The default port for nodes is 1984
.
A live list of public arweave nodes and IP adddresses can be found on this peer explorer.
{
host: 'arweave.net',// Hostname or IP address for a Arweave node
port: 80, // Port, defaults to 1984
protocol: 'https', // Network protocol http or https, defaults to http
timeout: 20000, // Network request timeouts in milliseconds
logging: false, // Enable network request logging
}
Here you can generate a new wallet address and private key (JWK), don't expose private keys or make them public as anyone with the key can use the corresponding wallet.
Make sure they're stored securely as they can never be recovered if lost.
Once AR has been sent to the address for a new wallet, the key can then be used to sign outgoing transactions.
arweave.wallets.generate().then((key) => {
console.log(key);
// {
// "kty": "RSA",
// "n": "3WquzP5IVTIsv3XYJjfw5L-t4X34WoWHwOuxb9V8w...",
// "e": ...
arweave.wallets.jwkToAddress(jwk).then((address) => {
console.log(address);
// 1seRanklLU_1VTGkEk7P0xAwMJfA7owA1JHW5KyZKlY
)};
});
arweave.wallets.jwkToAddress(jwk).then((address) => {
console.log(address);
//1seRanklLU_1VTGkEk7P0xAwMJfA7owA1JHW5KyZKlY
)};
Get the balance of a wallet, all amounts by default are returned in winston.
arweave.wallets.getBalance('1seRanklLU_1VTGkEk7P0xAwMJfA7owA1JHW5KyZKlY').then((balance) => {
let winston = balance;
let ar = arweave.ar.winstonToAr(balance);
console.log(winston);
//125213858712
console.log(ar);
//0.125213858712
});
arweave.wallets.getLastTransactionID('1seRanklLU_1VTGkEk7P0xAwMJfA7owA1JHW5KyZKlY').then((transactionId) => {
console.log(transactionId);
//3pXpj43Tk8QzDAoERjHE3ED7oEKLKephjnVakvkiHF8
});
Transactions are the building blocks of the Arweave permaweb, they can send AR betwen wallet addresses, or store data on the Arweave network.
The create transaction methods simply creates and returns an unsigned transaction object, you must sign the transaction and submit it separeately using the transactions.sign
and transactions.submit
methods.
Modifying a transaction object after signing it will invalidate the signature, this will cause it to be rejected by the network if submitted in that state. Transaction prices are based on the size of the data field, so modifying the data field after a transaction has been created isn't recommended as you'll need to manually update the price.
The transaction ID is a hash of the transaction signature, so a transaction ID can't be known until its contents are finalised and it has been signed.
Data transactions are used to store data on the Arweave permaweb, they can contain HTML data and be serverd like webpages or they can contain any arbitrary data.
let key = await arweave.wallets.generate();
// Plain text
let transactionA = arweave.createTransaction({
data: '<html><head><meta charset="UTF-8"><title>Hello world!</title></head><body></body></html>'
}, jwk);
// Buffer
let transactionB = arweave.createTransaction({
data: Buffer.from('Some data', 'utf8')
}, jwk);
console.log(transactionA);
// Transaction {
// last_tx: '',
// owner: 'wgfbaaSXJ8dszMabPo-...',
// tags: [],
// target: '',
// quantity: '0',
// data: 'eyJhIjoxfQ',
// reward: '321879995',
// signature: '' }
let key = await arweave.wallets.generate();
// Send 10.5 AR to 1seRanklLU_1VTGkEk7P0xAwMJfA7owA1JHW5KyZKlY
let transaction = arweave.createTransaction({
target: '1seRanklLU_1VTGkEk7P0xAwMJfA7owA1JHW5KyZKlY',
quantity: arweave.ar.arToWinston('10.5')
}, jwk);
console.log(transaction);
// Transaction {
// last_tx: '',
// owner: '14fXfoRDMFS5yTpUT7ODzj...',
// tags: [],
// target: '1seRanklLU_1VTGkEk7P0xAwMJfA7owA1JHW5KyZKlY',
// quantity: '10500000000000',
// data: '',
// reward: '2503211
// signature: '' }
Metadata can be added to transactions through tags, these are simple key/value attributes that can be used to document the contents of a transaction or provide related data.
ARQL uses tags when searching for transactions.
The Content-Type
is a reserved tag and is used to set the data content type. For example, a transaction with HTML data and a content type tag of text/html
will be served as a HTML page and render correctly in browsers,
if the content type is set to text/plain
then it will be served as a plain text document and not render in browsers.
let key = await arweave.wallets.generate();
let transaction = await arweave.createTransaction({
data: '<html><head><meta charset="UTF-8"><title>Hello world!</title></head><body></body></html>',
}, key);
transaction.addTag('Content-Type', 'text/html');
transaction.addTag('key2', 'value2');
console.log(transaction);
// Transaction {
// last_tx: '',
// owner: 's8zPWNlBMiJFLcvpH98QxnI6FoPar3vCK3RdT...',
// tags: [
// Tag { name: 'Q29udGVudC1UeXBl', value: 'dGV4dC9odG1s' },
// Tag { name: 'a2V5Mg', value: 'dmFsdWUy' }
// ],
// target: '',
// quantity: '0',
// data: 'PGh0bWw-PGhlYWQ-PG1ldGEgY2hh...',
// reward: '329989175',
// signature: '' }
let key = await arweave.wallets.generate();
let transaction = await arweave.createTransaction({
target: '1seRanklLU_1VTGkEk7P0xAwMJfA7owA1JHW5KyZKlY',
quantity: arweave.ar.arToWinston('10.5')
}, key);
await arweave.transactions.sign(transaction, key);
console.log(transaction);
// Signature and id fields are now populated
// Transaction {
// last_tx: '',
// owner: '2xu89EaA5zENRRsbOh4OscMcy...',
// tags: [],
// target: '1seRanklLU_1VTGkEk7P0xAwMJfA7owA1JHW5KyZKlY',
// quantity: '10500000000000',
// data: '',
// reward: '250321179212',
// signature: 'AbFjlpEHTN6_SKWsUSMAzalImOVxNm86Z8hoTZcItkYBJLx...'
// id: 'iHVHijWvKbIa0ZA9IbuKtOxJdNO9qyey6CIH324zQWI'
Once a transaction is submitted to the network it'll be broadcast around all nodes and mined into a block.
let key = await arweave.wallets.generate();
let transaction = await arweave.createTransaction({
target: '1seRanklLU_1VTGkEk7P0xAwMJfA7owA1JHW5KyZKlY',
quantity: arweave.ar.arToWinston('10.5')
}, key);
await arweave.transactions.sign(transaction, key);
const response = await arweave.transactions.post(transaction);
console.log(response.status);
// 200
// HTTP response codes (200 - ok, 400 - invalid transaction, 500 - error)
arweave.transactions.getStatus('bNbA3TEQVL60xlgCcqdz4ZPHFZ711cZ3hmkpGttDt_U').then(status => {
console.log(status);
// 200
})
Fetch a transaction from the connected arweave node. The data and tags are base64 encoded, these can be decoded using the built in helper methods.
const transaction = arweave.transactions.get('bNbA3TEQVL60xlgCcqdz4ZPHFZ711cZ3hmkpGttDt_U').then(transaction => {
console.log(transaction);
// Transaction {
// last_tx: 'cO5gl_d5ARnaoBtu2Vas8skgLg-6KnC9gH8duWP7Ll8',
// owner: 'pJjRtSRLpHUVAKCtWC9pjajI_VEpiPEEAHX0k...',
// tags: [
// Tag { name: 'Q29udGVudC1UeXBl', value: 'dGV4dC9odG1s' },
// Tag { name: 'VXNlci1BZ2VudA', value: 'QXJ3ZWF2ZURlcGxveS8xLjEuMA' }
// ],
// target: '',
// quantity: '0',
// data: 'CjwhRE9DVFlQRSBodG1sPgo8aHRtbCBsYW5nPSJlbiI...',
// reward: '1577006493',
// signature: 'NLiRQSci56KVNk-x86eLT1TyF1ST8pzE...',
// id: 'bNbA3TEQVL60xlgCcqdz4ZPHFZ711cZ3hmkpGttDt_U' }
// })
});
const transaction = arweave.transactions.get('bNbA3TEQVL60xlgCcqdz4ZPHFZ711cZ3hmkpGttDt_U').then(transaction => {
// Use the get method to get a specific transaction field.
console.log(transaction.get('signature'));
// NLiRQSci56KVNk-x86eLT1TyF1ST8pzE-s7jdCJbW-V...
console.log(transaction.get('data'));
//CjwhRE9DVFlQRSBodG1sPgo8aHRtbCBsYW5nPSJlbiI-C...
// Get the data base64 decoded as a Uint8Array byte array.
console.log(transaction.get('data', {decode: true}));
//Uint8Array[10,60,33,68,79,67,84,89,80,69...
// Get the data base64 decoded as a string.
console.log(transaction.get('data', {decode: true, string: true}));
//<!DOCTYPE html>
//<html lang="en">
//<head>
// <meta charset="UTF-8">
// <meta name="viewport" content="width=device-width, initial-scale=1.0">
// <title>ARWEAVE / PEER EXPLORER</title>
transaction.get('tags').forEach(tag => {
let key = tag.get('name', {decode: true, string: true});
let value = tag.get('value', {decode: true, string: true});
console.log(`${key} : ${value}`);
});
// Content-Type : text/html
// User-Agent : ArweaveDeploy/1.1.0
});
FAQs
Arweave JS client library
The npm package arweave receives a total of 41,100 weekly downloads. As such, arweave popularity was classified as popular.
We found that arweave demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.