What is as-table?
The 'as-table' npm package is a simple utility for formatting arrays of objects into a readable table format. It is particularly useful for displaying tabular data in a console or terminal environment.
What are as-table's main functionalities?
Basic Table Formatting
This feature allows you to format an array of objects into a readable table. The code sample demonstrates how to use 'as-table' to display a list of people with their names and ages in a tabular format.
const asTable = require('as-table');
const data = [
{ name: 'Alice', age: 30 },
{ name: 'Bob', age: 25 },
{ name: 'Charlie', age: 35 }
];
console.log(asTable(data));
Custom Column Formatting
This feature allows you to customize the formatting of specific columns. The code sample shows how to append 'years' to the age column values.
const asTable = require('as-table');
const data = [
{ name: 'Alice', age: 30 },
{ name: 'Bob', age: 25 },
{ name: 'Charlie', age: 35 }
];
const options = {
age: x => x + ' years'
};
console.log(asTable.configure(options)(data));
Column Alignment
This feature allows you to align the content of specific columns. The code sample demonstrates how to right-align the age column.
const asTable = require('as-table');
const data = [
{ name: 'Alice', age: 30 },
{ name: 'Bob', age: 25 },
{ name: 'Charlie', age: 35 }
];
const options = {
align: { age: 'right' }
};
console.log(asTable.configure(options)(data));
Other packages similar to as-table
cli-table
The 'cli-table' package is another utility for formatting data into tables for the console. It offers more advanced features such as nested tables, custom styles, and text wrapping. Compared to 'as-table', 'cli-table' provides more customization options but may be more complex to use.
table
The 'table' package is a highly configurable library for generating text tables. It supports features like text wrapping, column alignment, and custom border styles. It is more feature-rich than 'as-table' and is suitable for more complex table formatting needs.
easy-table
The 'easy-table' package is designed for simple and quick table formatting. It offers basic features like column alignment and custom formatting. It is similar to 'as-table' in terms of simplicity and ease of use, but with slightly different API and customization options.
as-table

A simple function that print objects and arrays as ASCII tables. Supports ANSI styling and weird 💩 Unicode emoji symbols (they won't break the layout), thanks to printable-characters
.
npm install as-table
Printing objects
asTable = require ('as-table')
asTable ([ { foo: true, string: 'abcde', num: 42 },
{ foo: false, string: 'qwertyuiop', num: 43 },
{ string: null, num: 44 } ])
foo string num
----------------------
true abcde 42
false qwertyuiop 43
null 44
Printing arrays
asTable ([['qwe', '123456789', 'zxcvbnm'],
['qwerty', '12', 'zxcvb'],
['qwertyiop', '1234567', 'z']])
qwe 123456789 zxcvbnm
qwerty 12 zxcvb
qwertyiop 1234567 z
Limiting total width by proportionally trimming cells + setting columns delimiter
asTable.configure ({ maxTotalWidth: 22, delimiter: ' | ' }) (data)
qwe | 1234… | zxc…
qwer… | 12 | zxc…
qwer… | 1234… | z
Right align
asTable.configure ({ right: true }) (data)
foo bar baz
-----------------------------
qwe 123456789 zxcvbnm
qwerty 12 zxcvb
qwertyiop 1234567 z
Providing a custom object printer
asTable.configure ({ print: x => (typeof x === 'boolean') ? (x ? 'yes' : 'no') : String (x) }) (data)
foo string num
--------------------
yes abcde 42
no qwertyuiop 43
null 44
The callback also receives a field name (in case of objects) or a column index (in case of arrays):
asTable = require ('as-table').configure ({
print (x, k) {
if (k === 'timestamp') return new Date (x).toGMTString()
return String (x)
}
})
asTable ([ { name: 'A', timestamp: 1561202591572 },
{ name: 'B', timestamp: 1558524240034 } ])
Obtaining a pre-configured function
asTable = require ('as-table').configure ({ maxTotalWidth: 25, delimiter: ' | ' })
asTable (data)
Customizing the title rendering and the header separator
With string coloring by ansicolor
(just for the demo purposes, any library will fit):
asTable = require ('as-table').configure ({ title: x => x.bright, delimiter: ' | '.dim.cyan, dash: '-'.bright.cyan })
console.log (
asTable ([ { foo: true, string: 'abcde', num: 42 },
{ foo: false, string: 'qwertyuiop'.bgMagenta.green.bright, num: 43 } ])
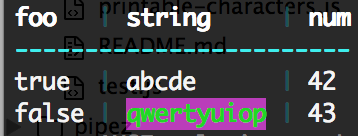