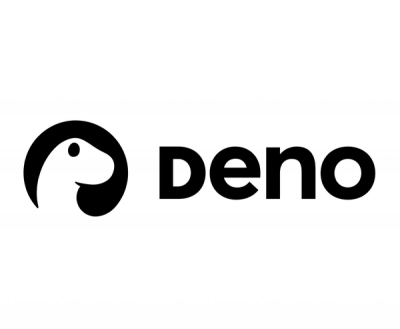
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
before, after, and around hooks for sync and async functions
also known as aspect-oriented programming
npm install --save aspects
const { before, after, around } = require('aspects').sync
const { all: catNames, random: catName } = require('cat-names')
// suppose a function
function hello (name) {
return `Hello, ${name}!`
}
console.log(hello(catName()))
// Hello, Misty!
// what if we could hook into _before_
// the function runs to check its arguments?
function onlyCats ([name]) {
return (catNames.indexOf(name) !== -1)
? [name]
: new Error(`${name} is not a cat name.`)
}
console.log(before(hello, onlyCats)(catName()))
// Hello, Bandit!
console.log(before(hello, onlyCats)(reverse(catName())))
// Error: aloL is not a cat name.
// at before.sync (example.js:13:7)
// at before.js:8:17
// at Object.<anonymous> (example.js:17:13)
// or _after_ to change the return value?
function loud (value) {
return value.toUpperCase()
}
console.log(after(hello, loud)(catName()))
// HELLO, SNICKERS!
// or _around_, to wrap the function?
function character (fn, args) {
return fn.apply(null, args.map(a => a[0])) + '!!!'
}
console.log(around(hello, character)(catName()))
// Hello, M!!!!
// utility function
function reverse (str) {
return str.split('').reverse().join('')
}
aspects = require('aspects')
{ before, after, around } = aspects
fn = before.sync(fn, hook)
fn
is sync function: uses return
hook
is function of shape (args) => Error | newArgs
fn = before.async(fn, hook)
fn
is async function: the last argument is an error-first callback
hook
is function of shape (args, cb) => cb(Error, newArgs)
fn = after.sync(fn, hook)
fn
is sync function: uses return
hook
is function of shape (value) => Error | newValue
fn = after.async(fn, hook)
fn
is async function: the last argument is an error-first callback
hook
is function of shape (value, cb) => cb(Error, newValue)
fn = around.sync(fn, hook)
fn
is sync function: uses return
hook
is function of shape (fn, args) => newFn
fn = around.async(fn, hook)
fn
is async function: the last argument is an error-first callback
hook
is function of shape (fn, args, cb) => newFn
The Apache License
Copyright © 2016 Michael Williams
Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions and limitations under the License.
FAQs
before, after, and around hooks for sync and sync functions
The npm package aspects receives a total of 1 weekly downloads. As such, aspects popularity was classified as not popular.
We found that aspects demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.