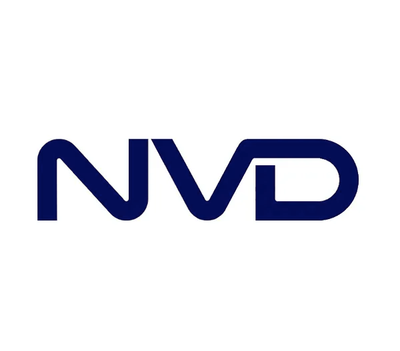
Security News
NIST Misses 2024 Deadline to Clear NVD Backlog
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
aspida | aspida-mock | openapi2aspida | pathpida |
---|
@aspida/axios | @aspida/ky | @aspida/fetch | @aspida/node-fetch |
---|
TypeScript friendly HTTP client wrapper for the browser and node.js.
$ mkdir apis
GET: /v1/users/?limit={number}
POST: /v1/users
apis/v1/users/index.ts
type User = {
id: number
name: string
}
export type Methods = {
get: {
query?: {
limit: number
}
resBody: User[]
}
post: {
reqBody: {
name: string
}
resBody: User
}
}
GET: /v1/users/${userId}
PUT: /v1/users/${userId}
apis/v1/users/_userId@number.ts
Specify the type of path variable “userId” starting with underscore with “@number”
If not specified with @, the default path variable type is "number | string"
type User = {
id: number
name: string
}
export type Methods = {
get: {
resBody: User
}
put: {
reqBody: {
name: string
}
resBody: User
}
}
package.json
{
"scripts": {
"api:build": "aspida --build"
}
}
$ npm run api:build
> apis/$api.ts was built successfully.
src/index.ts
import aspida from "@aspida/axios"
import api from "../apis/$api"
;(async () => {
const userId = 0
const limit = 10
const client = api(aspida())
await client.v1.users.post({ data: { name: "taro" } })
const res = await client.v1.users.get({ query: { limit } })
console.log(res)
// req -> GET: /v1/users/?limit=10
// res -> { status: 200, data: [{ id: 0, name: 'taro' }], headers: {...} }
const user = await client.v1.users._userId(userId).$get()
console.log(user)
// req -> GET: /v1/users/0
// res -> { id: 0, name: 'taro' }
})()
Create a configuration file at the root of the project
aspida.config.js
module.exports = { input: "src" }
Specify baseURL in configuration file
module.exports = { input: "apis", baseURL: "https://example.com/api" }
If you want to define multiple API endpoints, specify them in an array
module.exports = [{ input: "api1" }, { input: "api2", baseURL: "https://example.com/api" }]
aspida leaves GET parameter serialization to standard HTTP client behavior
If you want to serialize manually, you can use config object of HTTP client
src/index.ts
import axios from "axios"
import qs from "qs"
import aspida from "@aspida/axios"
import api from "../apis/$api"
;(async () => {
const client = api(
aspida(axios, { paramsSerializer: params => qs.stringify(params, { indices: false }) })
)
const users = await client.v1.users.$get({
// config: { paramsSerializer: (params) => qs.stringify(params, { indices: false }) },
query: { ids: [1, 2, 3] }
})
console.log(users)
// req -> GET: /v1/users/?ids=1&ids=2&ids=3
// res -> [{ id: 1, name: 'taro1' }, { id: 2, name: 'taro2' }, { id: 3, name: 'taro3' }]
})()
apis/v1/users/index.ts
export type Methods = {
post: {
reqFormat: FormData
reqBody: {
name: string
icon: ArrayBuffer
}
resBody: {
id: number
name: string
}
}
}
src/index.ts
import aspida from "@aspida/axios"
import api from "../apis/$api"
;(async () => {
const client = api(aspida())
const user = await client.v1.users.$post({
data: {
name: "taro",
icon: imageBuffer
}
})
console.log(user)
// req -> POST: h/v1/users
// res -> { id: 0, name: 'taro' }
})()
apis/v1/users/index.ts
export type Methods = {
post: {
reqFormat: URLSearchParams
reqBody: {
name: string
}
resBody: {
id: number
name: string
}
}
}
src/index.ts
import aspida from "@aspida/axios"
import api from "../apis/$api"
;(async () => {
const client = api(aspida())
const user = await client.v1.users.$post({ data: { name: "taro" } })
console.log(user)
// req -> POST: /v1/users
// res -> { id: 0, name: 'taro' }
})()
apis/v1/users/index.ts
export type Methods = {
get: {
query: {
name: string
}
resBody: ArrayBuffer
}
}
src/index.ts
import aspida from "@aspida/axios"
import api from "../apis/$api"
;(async () => {
const client = api(aspida())
const user = await client.v1.users.$get({ query: { name: "taro" } })
console.log(user)
// req -> GET: /v1/users/?name=taro
// res -> ArrayBuffer
})()
aspida is licensed under a MIT License.
FAQs
TypeScript friendly HTTP client wrapper for the browser and node.js
The npm package aspida receives a total of 19,723 weekly downloads. As such, aspida popularity was classified as popular.
We found that aspida demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.