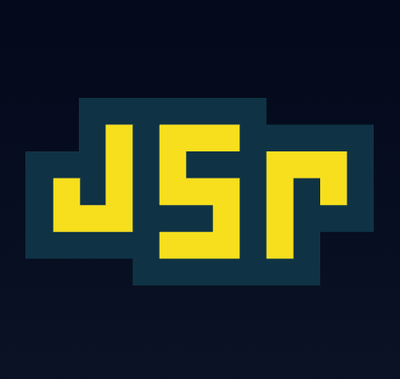
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
assemblyscript
Advanced tools
AssemblyScript defines a subset of TypeScript that it compiles to WebAssembly. It aims to provide everyone with an existing background in TypeScript and standard JavaScript-APIs with a comfortable way to compile to WebAssembly, eliminating the need to switch between languages or to learn new ones just for this purpose.
Try it out in your browser: dcode.io/AssemblyScript
How it works
A few insights to get an initial idea.
What to expect
General remarks on design decisions and trade-offs.
Example
Basic examples to get you started.
Usage
An introduction to the environment and its provided functionality.
Command line
How to use the command line utility.
API
How to use the API programmatically.
Additional documentation
A list of available documentation resources.
Building
How to build the compiler and its components yourself.
Under the hood, AssemblyScript rewires TypeScript's compiler API to Binaryen's compiler backend. The compiler itself is written in (and based upon) TypeScript and no binary dependencies are required to get started.
Every AssemblyScript program is valid TypeScript syntactically, but not necessarily semantically. The definitions required to start developing in AssemblyScript are provided by assembly.d.ts. See also: Usage
The compiler is able to produce WebAssembly binaries (.wasm) as well as their corresponding text format (.wast). Both Binaryen's s-expression format and, with a little help of WABT, official linear text format are supported. See also: CLI
The most prominent difference of JavaScript and any strictly typed language is that, in TypeScript/JavaScript, a variable can reference a value of any type. This implies that a JavaScript VM has to conduct additional book-keeping of a value's type in addition to its value and that it has to perform additional checks whenever a variable is accessed. Modern JavaScript VMs shortcut the overhead introduced by this and similar dynamic features by generating case-specific code based on statistical information collected just in time, effectively reducing the amount of checks to perform implicitly and thus speeding up execution significantly. Similarily, developers shortcut the overhead of remembering each variable's type by using TypeScript. The combination of both also makes a good match because it potentially aids the JIT compiler.
Nonetheless, TypeScript isn't a strictly typed language after all because it allows specific constructs to resort to JavaScript's dynamic features. For example, TypeScript allows annotating function parameters as omittable (i.e. someParameter?: number
), effectively resulting in a union type number | undefined
at runtime, just like it also allows declaring union types explicitly. Conceptionally, these constructs are incompatible with a strict, AOT-compiled type system unless relatively expensive workarounds are introduced. Hence...
Instead of trying to mimic TypeScript/JavaScript as closely as possible at the expense of performance (recap that: slower than similar code running in a JIT-compiling VM), AssemblyScript tries to support TypeScript features as closely as reasonable, not supporting certain JavaScript-specific dynamic constructs intentionally:
any
and undefined
are not supported by design&&
/ ||
expressions is always bool
Also note that AssemblyScript is a rather new and ambitious project developed by one guy and a hand full of occasional contributors. Expect bugs and breaking changes. Prepare to fix stuff yourself and to send a PR for it, unless you like the idea enough to consider sponsoring development.
export function add(a: int, b: double): short {
return (a + (b as int)) as short;
}
Compiles to:
(module
(type $iFi (func (param i32 f64) (result i32)))
(memory $0 256)
(export "memory" (memory $0))
(export "add" (func $add))
(func $add (type $iFi) (param $0 i32) (param $1 f64) (result i32)
(return
(i32.shr_s
(i32.shl
(i32.add
(get_local $0)
(i32.trunc_s/f64
(get_local $1)
)
)
(i32.const 16)
)
(i32.const 16)
)
)
)
)
See the pre-configured example project for a quickstart.
The stand-alone loader component provides an easy way to run and work with compiled WebAssembly modules:
$> npm install assemblyscript-loader
import load from "assemblyscript-loader"; // JS: var load = require("assemblyscript-loader").load;
load("path/to/module.wasm", {
imports: {
...
}
}).then(module => {
...
// i.e. call module.exports.main()
});
$> npm install assemblyscript --save-dev
The environment is configured by either referencing assembly.d.ts directly or by using a tsconfig.json
that simply extends tsconfig.assembly.json, like so:
{
"extends": "./node_modules/assemblyscript/tsconfig.assembly.json",
"include": [
"./*.ts"
]
}
The tsconfig.json
-approach is recommended to inherit other important settings as well.
Once configured, the following AssemblyScript-specific types become available:
Type | Alias | Native type | sizeof | Description |
---|---|---|---|---|
sbyte | int8 | i32 | 1 | An 8-bit signed integer. |
byte | uint8 | i32 | 1 | An 8-bit unsigned integer. |
short | int16 | i32 | 2 | A 16-bit signed integer. |
ushort | uint16 | i32 | 2 | A 16-bit unsigned integer. |
int | int32 | i32 | 4 | A 32-bit signed integer. |
uint | uint32 | i32 | 4 | A 32-bit unsigned integer. |
long | int64 | i64 | 8 | A 64-bit signed integer. |
ulong | uint64 | i64 | 8 | A 64-bit unsigned integer. |
uintptr | - | i32 / i64 | 4 / 8 | A 32-bit unsigned integer when targeting 32-bit WebAssembly. A 64-bit unsigned integer when targeting 64-bit WebAssembly. |
float | float32 | f32 | 4 | A 32-bit float. |
double | float64 | f64 | 8 | A 64-bit float. |
bool | - | i32 | 1 | A 1-bit unsigned integer. |
void | - | none | - | No return type |
While generating a warning to avoid type confusion, the JavaScript types number
and boolean
resolve to double
and bool
respectively.
WebAssembly-specific operations are available as built-in functions that translate to the respective opcode directly:
int
, shift: int
): int
long
, shift: long
): long
int
, shift: int
): int
long
, shift: long
): long
int
): int
long
): long
int
): int
long
): long
int
): int
long
): long
double
): double
float
): float
double
): double
float
): float
double
): double
float
): float
double
): double
float
): float
double
): double
float
): float
double
): double
float
): float
double
, right: double
): double
NaN
, returns NaN
.float
, right: float
): float
NaN
, returns NaN
.double
, right: double
): double
NaN
, returns NaN
.float
, right: float
): float
NaN
, returns NaN
.double
, y: double
): double
x
and the sign of y
.float
, y: float
): float
x
and the sign of y
.float
): int
double
): long
int
): float
long
): double
int
uint
): int
-1
on failure.void
The following AssemblyScript-specific operations are implemented as built-ins as well:
T
>(): uintptr
T1
,T2
>(value: T1
): T2
T1
to a value of type T2
. Useful for casting classes to pointers and vice-versa. Does not perform any checks.double
): bool
float
): bool
double
): bool
float
): bool
These constants are present as immutable globals (note that optimizers might inline them):
double
float
double
float
By default, standard memory management routines based on dlmalloc and musl will be linked statically and can be configured to be exported to the embedder:
uintptr
): uintptr
uintptr
): void
uintptr
, src: uintptr
, size: uintptr
): uintptr
uintptr
, c: int
, size: uintptr
): uintptr
c
. Usually used to reset it to all 0
s.uintptr
, vr: uintptr
, n: uintptr
): int
0
if both are equal, otherwise vl[i] - vr[i]
at the first difference's byte offset i
.Linking in memory management routines adds about 11kb to a module. Once WebAssembly exposes the garbage collector natively, there'll be other options as well. Note that the new
operator depends on malloc
and will break when --no-malloc
is specified (and no other malloc
is present). Also note that calling grow_memory
where malloc
is present will most likely break malloc
as it expects contiguous memory.
Type coercion requires an explicit cast where precision or signage is lost respectively is implicit where it is maintained. For example, to cast a double
to an int
:
function example(value: double): int {
return value as int; // translates to the respective opcode
}
Global WebAssembly imports can be declare
d anywhere while WebAssembly exports are export
ed from the entry file (the file specified when calling asc
or Compiler.compileFile
). Aside from that, imports and exports work just like in TypeScript.
// entry.ts
import { myOtherExportThatDoesntBecomeAWebAssemblyExport } from "./imported";
declare function myImport(): void;
export function myExport(): void {
myOtherExportThatDoesntBecomeAWebAssemblyExport();
}
Currently, imports can also be pulled from different namespaces by separating the namespace and the function with a $
character.
declare function Math$random(): double;
Naming a function start
with no arguments and a void
return type will automatically make it the start function that is being called on load even before returning to the embedder.
function start(): void {
...
}
The command line compiler asc
works similar to TypeScript's tsc
:
Syntax: asc [options] entryFile
Options:
--config, -c Specifies a JSON configuration file with command line options.
Will look for 'asconfig.json' in the entry's directory if omitted.
--outFile, -o Specifies the output file name. Emits text format if ending with .wast
(sexpr) or .wat (linear). Prints to stdout if omitted.
--optimize, -O Runs optimizing binaryen IR passes.
--validate, -v Validates the module.
--quiet, -q Runs in quiet mode, not printing anything to console.
--target, -t Specifies the target architecture:
wasm32 Compiles to 32-bit WebAssembly [default]
wasm64 Compiles to 64-bit WebAssembly
--memoryModel, -m Specifies the memory model to use / how to proceed with malloc etc.:
malloc Bundles malloc etc. [default]
exportmalloc Bundles malloc etc. and exports each
importmalloc Imports malloc etc. from 'env'
bare Excludes malloc etc. entirely
--textFormat, -f Specifies the format to use for text output:
sexpr Emits s-expression syntax (.wast) [default]
linear Emits official linear syntax (.wat)
Text format only is emitted when used without --textFile.
--textFile Can be used to save text format alongside a binary in one command.
--help, -h Displays this help message.
A configuration file (usually named asconfig.json
) using the long option keys above plus a special key entryFile
specifying the path to the entry file can be used to reuse options between invocations.
It's also possible to use the API programmatically:
Compiler.compileFile(filename: string
, options?: CompilerOptions
): binaryen.Module | null
Compiles the specified entry file to a WebAssembly module. Returns null
on failure.
Compiler.compileString(source: string
, options?: CompilerOptions
): binaryen.Module | null
Compiles the specified entry file source to a WebAssembly module. Returns null
on failure.
Compiler.lastDiagnostics: typescript.Diagnostic[]
Contains the diagnostics generated by the last invocation of compilerFile
or compileString
.
CompilerOptions
AssemblyScript compiler options.
boolean
false
.boolean
true
. Disable this when building a dynamically linked library.CompilerTarget | string
CompilerTarget.WASM32
.CompilerMemoryModel | string
CompilerMemoryModel.MALLOC
.CompilerTarget
Compiler target.
CompilerMemoryModel
Compiler memory model.
import { Compiler, CompilerTarget, CompilerMemoryModel, typescript } from "assemblyscript";
const module = Compiler.compileString(`
export function add(a: int, b: int): int {
return a + b;
}
`, {
target: CompilerTarget.WASM32,
memoryModel: CompilerMemoryModel.MALLOC,
silent: true
});
console.error(typescript.formatDiagnostics(Compiler.lastDiagnostics));
if (!module)
throw Error("compilation failed");
module.optimize();
if (!module.validate())
throw Error("validation failed");
const textFile = module.emitText();
const wasmFile = module.emitBinary();
...
module.dispose();
Remember to call binaryen.Module#dispose()
once you are done with a module to free its resources. This is necessary because binaryen.js has been compiled from C/C++ and doesn't provide automatic garbage collection.
Clone the GitHub repository and install the development dependencies:
$> git clone https://github.com/dcodeIO/AssemblyScript.git
$> cd AssemblyScript
$> npm install
Afterwards, to build the distribution files to dist/, run:
$> npm run build
To build the documentation to docs/api/, run:
$> npm run docs
Running the tests (ideally on node.js >= 8):
$> npm test
License: Apache License, Version 2.0
FAQs
A TypeScript-like language for WebAssembly.
The npm package assemblyscript receives a total of 34,104 weekly downloads. As such, assemblyscript popularity was classified as popular.
We found that assemblyscript demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.