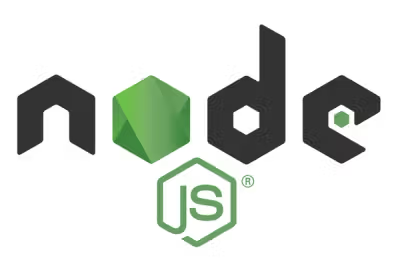
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
assert-options
Advanced tools
The assert-options npm package is a utility for validating and asserting options in JavaScript objects. It helps ensure that the options passed to a function or module are valid and meet the expected criteria.
Basic Option Validation
This feature allows you to validate and merge user-provided options with default options. If the user does not provide an option, the default value is used.
const assertOptions = require('assert-options');
function myFunction(options) {
const defaultOptions = { a: 1, b: 2 };
options = assertOptions(options, defaultOptions);
console.log(options);
}
myFunction({ a: 10 }); // { a: 10, b: 2 }
myFunction(); // { a: 1, b: 2 }
Strict Option Validation
This feature enforces strict validation, ensuring that only the specified options are allowed. If an unknown option is provided, an error is thrown.
const assertOptions = require('assert-options');
function myFunction(options) {
const defaultOptions = { a: 1, b: 2 };
options = assertOptions(options, defaultOptions, { strict: true });
console.log(options);
}
myFunction({ a: 10, c: 3 }); // Throws an error because 'c' is not a valid option
Nested Option Validation
This feature supports validation of nested options, allowing you to merge and validate deeply nested objects.
const assertOptions = require('assert-options');
function myFunction(options) {
const defaultOptions = { a: 1, b: { c: 2, d: 3 } };
options = assertOptions(options, defaultOptions);
console.log(options);
}
myFunction({ b: { c: 10 } }); // { a: 1, b: { c: 10, d: 3 } }
myFunction(); // { a: 1, b: { c: 2, d: 3 } }
Joi is a powerful schema description language and data validator for JavaScript. It allows you to create blueprints or schemas for JavaScript objects to ensure validation. Compared to assert-options, Joi offers more advanced and flexible validation capabilities, including support for complex data types and custom validation rules.
Yup is a JavaScript schema builder for value parsing and validation. It is similar to Joi but is often preferred for its simplicity and ease of use. Like assert-options, Yup can validate and transform objects, but it provides more comprehensive validation features and better integration with form libraries.
Prop-types is a runtime type checking library for React props. While it is primarily used for validating React component props, it can also be used for general object validation. Compared to assert-options, prop-types is more focused on type checking rather than option validation and merging.
Smart options
-object handling, with one line of code:
Strongly-typed, built with TypeScript 5.x strict
mode, for JavaScript clients.
This module automates proper options handling - parsing + setting defaults in one line.
Although this library is implemented in TypeScript, its objective is mainly to help JavaScript clients, because TypeScript itself can handle invalid options and defaults natively.
$ npm i assert-options
const { assertOptions } = require('assert-options');
function functionWithOptions(options) {
options = assertOptions(options, {first: 123, second: null});
// options is a safe object here, with all missing defaults set.
}
When default values are not needed, you can just use an array of strings:
function functionWithOptions(options) {
options = assertOptions(options, ['first', 'second']);
// the result is exactly the same as using the following:
// options = assertOptions(options, {first: undefined, second: undefined});
// options is a safe object here, without defaults.
}
You can override how errors are thrown, by creating the assert
function yourself,
and specifying a custom handler:
const {createAssert} = require('assert-options');
// must implement IOptionsErrorHandler protocol
class MyErrorHanler {
handle(err, ctx) {
// throw different errors, based on "err"
// for reference, see DefaultErrorHandler implementation
}
}
const assert = createAssert(new MyErrorHanler());
assertOptions(options, defaults)
When options
is null
/undefined
, new {}
is returned, applying defaults
as specified.
When options
contains an unknown property, Error Option "name" is not recognized.
is thrown.
When a property in options
is missing or undefined
, its value is set from the defaults
,
provided it is available and its value is not undefined
.
When options
is not null
/undefined
, it must be of type object
, or else TypeError is thrown:
Invalid "options" parameter: value
.
Parameter defaults
is required, as a non-null
object or an array of strings, or else TypeError
is thrown: Invalid "defaults" parameter: value
.
createAssert(handler)
Creates a new assert function, using a custom error handler that implements IOptionsErrorHandler
protocol.
For example, the default assertOptions
is created internally like this:
const {createOptions, DefaultErrorHandler} = require('assert-options');
const assertOptions = createAssert(new DefaultErrorHandler());
FAQs
Generic options parameter handling.
The npm package assert-options receives a total of 358,068 weekly downloads. As such, assert-options popularity was classified as popular.
We found that assert-options demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.