Comparing version 0.0.0 to 0.0.1
@@ -0,1 +1,4 @@ | ||
export * from "./binary_search"; | ||
export * from "./heap"; | ||
export * from "./iterations"; | ||
export * from "./random"; |
@@ -0,1 +1,8 @@ | ||
import { Vector } from "../container/Vector"; | ||
import { CMath } from "../internal/numeric/CMath"; | ||
import { advance, distance } from "../iterator/global"; | ||
import { sort } from "./sorting"; | ||
@inline() | ||
export function randint<T>(x: T, y: T): T | ||
@@ -5,2 +12,46 @@ { | ||
return <T>value; | ||
} | ||
export function sample<InputIterator, OutputIterator> | ||
( | ||
first: InputIterator, last: InputIterator, | ||
output: OutputIterator, n: usize | ||
): OutputIterator | ||
{ | ||
// GENERATE REMAINDERS | ||
const step: usize = distance(first, last); | ||
const remainders: usize[] = []; | ||
for (let i: number = 0; i < step; ++i) | ||
remainders.push(i); | ||
//---- | ||
// CONSTRUCT INDEXES | ||
//---- | ||
const advances: Vector<usize> = new Vector(); | ||
n = CMath.min(n, step); | ||
// PICK SAMPLE INDEXES | ||
for (let i: usize = 0; i < n; ++i) | ||
{ | ||
const index: usize = randint<usize>(0, <usize>remainders.length - 1); | ||
advances.push_back(remainders.splice(index, 1)[0]); | ||
} | ||
sort<Vector.Iterator<usize>, (x: usize, y: usize) => boolean, usize>(advances.begin(), advances.end(), (x, y) => x < y); | ||
// CHANGE INDEXES TO ADVANCES | ||
for (let i: isize = n - 1; i >= 1; --i) | ||
advances.set(i, advances.at(i) - advances.at(i - 1)); | ||
//---- | ||
// FILL SAMPLES | ||
//---- | ||
for (let i: usize = 0; i < advances.size(); ++i) | ||
{ | ||
first = advance(first, advances.at(i)); | ||
output.value = first.value; | ||
output = output.next(); | ||
} | ||
return output; | ||
} |
@@ -6,6 +6,7 @@ import { IForwardIterator } from "../iterator/IForwardIterator"; | ||
import { SourcePointer } from "../internal/functional/SourcePointer"; | ||
import { distance } from "../iterator/global"; | ||
import { advance, distance } from "../iterator/global"; | ||
import { Comparator } from "../internal/functional/Comparator"; | ||
import { BinaryPredicator } from "../internal/functional/BinaryPredicator"; | ||
import { UnaryPredicator } from "../internal/functional/UnaryPredicator"; | ||
import { Comparator } from "../internal/functional/Comparator"; | ||
@@ -17,3 +18,3 @@ export class List<T> | ||
private end_: List.Iterator<T> = List.Iterator._Create(this.source_ptr_); | ||
private end_: List.Iterator<T> = List.Iterator._Create(this.source_ptr_, null, null, changetype<T>(0)); | ||
private begin_: List.Iterator<T> = this.end_; | ||
@@ -27,6 +28,6 @@ private size_: usize = 0; | ||
{ | ||
List.Iterator._Set_prev<T>(this.end_, null!); | ||
List.Iterator._Set_next<T>(this.end_, null!); | ||
List.Iterator._Set_prev<T>(this.end_, null); | ||
List.Iterator._Set_next<T>(this.end_, null); | ||
this.begin_ = this.end(); | ||
this.begin_ = this.end_; | ||
this.size_ = 0; | ||
@@ -37,3 +38,6 @@ } | ||
{ | ||
if (n < this.size()) | ||
this.erase(advance(this.end(), this.size() - n), this.end()); | ||
else if (n > this.size()) | ||
this.insert_repeatedly(this.end(), n - this.size(), changetype<T>(0)); | ||
} | ||
@@ -116,6 +120,5 @@ | ||
const it: List.Iterator<T> = List.Iterator._Create<T>(this.source_ptr_, prev, pos); | ||
const it: List.Iterator<T> = List.Iterator._Create<T>(this.source_ptr_, prev, pos, val); | ||
List.Iterator._Set_next<T>(prev, it); | ||
List.Iterator._Set_prev<T>(pos, it); | ||
it.value = val; | ||
@@ -148,9 +151,7 @@ ++this.size_; | ||
{ | ||
const it: List.Iterator<T> = List.Iterator._Create<T>(this.source_ptr_, prev); | ||
it.value = first.value; | ||
const it: List.Iterator<T> = List.Iterator._Create<T>(this.source_ptr_, prev, null, first.value); | ||
List.Iterator._Set_next<T>(prev, it); | ||
if (top === null) | ||
top = it; | ||
List.Iterator._Set_next<T>(prev, it); | ||
prev = it; | ||
@@ -163,4 +164,4 @@ ++size; | ||
// RETURNS WITH FINALIZATION | ||
List.Iterator._Set_next<T>(prev, top!); | ||
List.Iterator._Set_prev<T>(pos, top!); | ||
List.Iterator._Set_next<T>(prev, top); | ||
List.Iterator._Set_prev<T>(pos, top); | ||
@@ -377,3 +378,3 @@ if (pos === this.begin_) | ||
--------------------------------------------------------------- */ | ||
private constructor(sourcePtr: SourcePointer<List<T>>) | ||
private constructor(sourcePtr: SourcePointer<List<T>>, prev: Iterator<T> | null, next: Iterator<T> | null, value: T) | ||
{ | ||
@@ -383,18 +384,15 @@ this.source_ptr_ = sourcePtr; | ||
this.prev_ = null; | ||
this.next_ = null; | ||
this.value_ = changetype<T>(0); | ||
this.prev_ = prev; | ||
this.next_ = next; | ||
this.value_ = value; | ||
} | ||
public static _Create<T>(sourcePtr: SourcePointer<List<T>>, prev: Iterator<T> | null = null, next: Iterator<T> | null = null): Iterator<T> | ||
@inline() | ||
public static _Create<T>(sourcePtr: SourcePointer<List<T>>, prev: Iterator<T> | null, next: Iterator<T> | null, value: T): Iterator<T> | ||
{ | ||
const ret: Iterator<T> = new Iterator(sourcePtr); | ||
if (prev) ret.prev_ = prev; | ||
if (next) ret.next_ = next; | ||
return ret; | ||
return new Iterator<T>(sourcePtr, prev, next, value); | ||
} | ||
@inline() | ||
public static _Set_prev<T>(it: Iterator<T>, prev: Iterator<T>): void | ||
public static _Set_prev<T>(it: Iterator<T>, prev: Iterator<T> | null): void | ||
{ | ||
@@ -405,3 +403,3 @@ it.prev_ = prev; | ||
@inline() | ||
public static _Set_next<T>(it: Iterator<T>, next: Iterator<T>): void | ||
public static _Set_next<T>(it: Iterator<T>, next: Iterator<T> | null): void | ||
{ | ||
@@ -408,0 +406,0 @@ it.next_ = next; |
@@ -1,14 +0,18 @@ | ||
import { TreeMultiSet } from "./TreeMultiSet"; | ||
import { Vector } from "./Vector"; | ||
import { Comparator } from "../internal/functional/Comparator"; | ||
import { less } from "../functional/comparators"; | ||
import { pop_heap, push_heap } from "../algorithm/heap"; | ||
export class PriorityQueue<T> | ||
{ | ||
private readonly container_: TreeMultiSet<T>; | ||
private readonly comp_: Comparator<T>; | ||
private data_: Vector<T>; | ||
private comp_: Comparator<T>; | ||
/* --------------------------------------------------------- | ||
CONSTURCTORS | ||
--------------------------------------------------------- */ | ||
public constructor(comp: Comparator<T> = (x, y) => less(x, y)) | ||
{ | ||
this.container_ = new TreeMultiSet(comp); | ||
this.data_ = new Vector(); | ||
this.comp_ = comp; | ||
@@ -18,11 +22,33 @@ } | ||
@inline() | ||
public data(): TreeMultiSet<T> | ||
public push(elem: T): void | ||
{ | ||
return this.container_; | ||
this.data_.push_back(elem); | ||
push_heap<Vector.Iterator<T>, Comparator<T, T>, T>(this.data_.begin(), this.data_.end(), this.comp_); | ||
} | ||
@inline() | ||
public pop(): void | ||
{ | ||
pop_heap<Vector.Iterator<T>, Comparator<T, T>, T>(this.data_.begin(), this.data_.end(), this.comp_); | ||
this.data_.pop_back(); | ||
} | ||
public swap(obj: PriorityQueue<T>): void | ||
{ | ||
const data: Vector<T> = this.data_; | ||
this.data_ = obj.data_; | ||
obj.data_ = data; | ||
const comp: Comparator<T> = this.comp_; | ||
this.comp_ = obj.comp_; | ||
obj.comp_ = comp; | ||
} | ||
/* --------------------------------------------------------- | ||
ACCESSORS | ||
--------------------------------------------------------- */ | ||
@inline() | ||
public size(): usize | ||
{ | ||
return this.container_.size(); | ||
return this.data_.size(); | ||
} | ||
@@ -33,3 +59,3 @@ | ||
{ | ||
return this.container_.empty(); | ||
return this.data_.empty(); | ||
} | ||
@@ -46,16 +72,4 @@ | ||
{ | ||
return this.container_.end().prev().value; | ||
} | ||
@inline() | ||
public push_back(elem: T): void | ||
{ | ||
this.container_.insert(elem); | ||
} | ||
@inline() | ||
public pop(): void | ||
{ | ||
this.container_.erase(this.container_.end().prev()); | ||
} | ||
return this.data_.front(); | ||
} | ||
} |
@@ -5,44 +5,57 @@ import { List } from "./List"; | ||
{ | ||
private container_: List<T>; | ||
private data_: List<T>; | ||
/* --------------------------------------------------------- | ||
CONSTURCTORS | ||
--------------------------------------------------------- */ | ||
public constructor() | ||
{ | ||
this.container_ = new List(); | ||
this.data_ = new List(); | ||
} | ||
@inline() | ||
public size(): usize | ||
public pop(): void | ||
{ | ||
return this.container_.size(); | ||
this.data_.pop_front(); | ||
} | ||
@inline() | ||
public empty(): boolean | ||
public push(value: T): void | ||
{ | ||
return this.container_.empty(); | ||
this.data_.push_back(value); | ||
} | ||
public swap(obj: Queue<T>): void | ||
{ | ||
const data: List<T> = this.data_; | ||
this.data_ = obj.data_; | ||
obj.data_ = data; | ||
} | ||
/* --------------------------------------------------------- | ||
ACCESSORS | ||
--------------------------------------------------------- */ | ||
@inline() | ||
public front(): T | ||
public size(): usize | ||
{ | ||
return this.container_.front(); | ||
return this.data_.size(); | ||
} | ||
@inline() | ||
public back(): T | ||
public empty(): boolean | ||
{ | ||
return this.container_.back(); | ||
return this.data_.empty(); | ||
} | ||
@inline() | ||
public pop(): void | ||
public front(): T | ||
{ | ||
this.container_.pop_front(); | ||
return this.data_.front(); | ||
} | ||
@inline() | ||
public push(value: T): void | ||
public back(): T | ||
{ | ||
this.container_.push_back(value); | ||
return this.data_.back(); | ||
} | ||
} |
@@ -5,37 +5,50 @@ import { Vector } from "./Vector"; | ||
{ | ||
private container_: Vector<T>; | ||
private data_: Vector<T>; | ||
/* --------------------------------------------------------- | ||
CONSTURCTORS | ||
--------------------------------------------------------- */ | ||
public constructor() | ||
{ | ||
this.container_ = new Vector<T>(); | ||
this.data_ = new Vector<T>(); | ||
} | ||
@inline() | ||
public size(): usize | ||
public pop(): void | ||
{ | ||
return this.container_.size(); | ||
this.data_.pop_back(); | ||
} | ||
@inline() | ||
public empty(): boolean | ||
public push(value: T): void | ||
{ | ||
return this.container_.empty(); | ||
this.data_.push_back(value); | ||
} | ||
public swap(obj: Stack<T>): void | ||
{ | ||
const data: Vector<T> = this.data_; | ||
this.data_ = obj.data_; | ||
obj.data_ = data; | ||
} | ||
/* --------------------------------------------------------- | ||
ACCESSORS | ||
--------------------------------------------------------- */ | ||
@inline() | ||
public top(): T | ||
public size(): usize | ||
{ | ||
return this.container_.back(); | ||
return this.data_.size(); | ||
} | ||
@inline() | ||
public pop(): void | ||
public empty(): boolean | ||
{ | ||
this.container_.pop_back(); | ||
return this.data_.empty(); | ||
} | ||
@inline() | ||
public push(value: T): void | ||
public top(): T | ||
{ | ||
this.container_.push_back(value); | ||
return this.data_.back(); | ||
} | ||
@@ -42,0 +55,0 @@ } |
@@ -22,3 +22,3 @@ import { IMapContainer } from "./IMapContainer"; | ||
private source_: SourceT; | ||
private end_: MapElementList.Iterator<Key, T, Unique, SourceT> = MapElementList.Iterator._Create(this, this.sequence_++); | ||
private end_: MapElementList.Iterator<Key, T, Unique, SourceT> = MapElementList.Iterator._Create(this, this.sequence_++, null, null, null); | ||
private begin_: MapElementList.Iterator<Key, T, Unique, SourceT> = this.end_; | ||
@@ -36,11 +36,6 @@ | ||
this.begin_ = this.end(); | ||
this.begin_ = this.end_; | ||
this.size_ = 0; | ||
} | ||
public resize(n: usize): void | ||
{ | ||
} | ||
/* --------------------------------------------------------- | ||
@@ -232,3 +227,3 @@ ACCCESSORS | ||
private next_: Iterator<Key, T, Unique, SourceT> | null; | ||
private value_: Entry<Key, T> | null; | ||
private readonly value_: Entry<Key, T> | null; | ||
@@ -238,3 +233,10 @@ /* --------------------------------------------------------------- | ||
--------------------------------------------------------------- */ | ||
private constructor(container: MapElementList<Key, T, Unique, SourceT>, uid: usize) | ||
private constructor | ||
( | ||
container: MapElementList<Key, T, Unique, SourceT>, | ||
uid: usize, | ||
prev: Iterator<Key, T, Unique, SourceT> | null, | ||
next: Iterator<Key, T, Unique, SourceT> | null, | ||
value: Entry<Key, T> | null | ||
) | ||
{ | ||
@@ -245,7 +247,8 @@ this.container_ = container; | ||
this.prev_ = null; | ||
this.next_ = null; | ||
this.value_ = null; | ||
this.prev_ = prev; | ||
this.next_ = next; | ||
this.value_ = value; | ||
} | ||
@inline() | ||
public static _Create<Key, T, | ||
@@ -260,13 +263,8 @@ Unique extends boolean, | ||
uid: usize, | ||
prev: Iterator<Key, T, Unique, SourceT> | null = null, | ||
next: Iterator<Key, T, Unique, SourceT> | null = null, | ||
value: Entry<Key, T> | null = null | ||
prev: Iterator<Key, T, Unique, SourceT> | null, | ||
next: Iterator<Key, T, Unique, SourceT> | null, | ||
value: Entry<Key, T> | null | ||
): Iterator<Key, T, Unique, SourceT> | ||
{ | ||
const ret: Iterator<Key, T, Unique, SourceT> = new Iterator(container, uid); | ||
if (prev) ret.prev_ = prev; | ||
if (next) ret.next_ = next; | ||
ret.value_ = value; | ||
return ret; | ||
return new Iterator(container, uid, prev, next, value); | ||
} | ||
@@ -273,0 +271,0 @@ |
@@ -19,3 +19,3 @@ import { ISetContainer } from "./ISetContainer"; | ||
private source_: SourceT; | ||
private end_: SetElementList.Iterator<Key, Unique, SourceT> = SetElementList.Iterator._Create(this, this.uid_++); | ||
private end_: SetElementList.Iterator<Key, Unique, SourceT> = SetElementList.Iterator._Create(this, this.uid_++, null, null, changetype<Key>(0)); | ||
private begin_: SetElementList.Iterator<Key, Unique, SourceT> = this.end_; | ||
@@ -33,3 +33,3 @@ | ||
this.begin_ = this.end(); | ||
this.begin_ = this.end_; | ||
this.size_ = 0; | ||
@@ -124,4 +124,3 @@ } | ||
const it: SetElementList.Iterator<Key, Unique, SourceT> = SetElementList.Iterator._Create(this, this.uid_++, prev, pos); | ||
SetElementList.Iterator._Set_value<Key, Unique, SourceT>(it, value); | ||
const it: SetElementList.Iterator<Key, Unique, SourceT> = SetElementList.Iterator._Create(this, this.uid_++, prev, pos, value); | ||
SetElementList.Iterator._Set_next<Key, Unique, SourceT>(prev, it); | ||
@@ -157,9 +156,7 @@ SetElementList.Iterator._Set_prev<Key, Unique, SourceT>(pos, it); | ||
{ | ||
const it: SetElementList.Iterator<Key, Unique, SourceT> = SetElementList.Iterator._Create(this, this.uid_++, prev, null); | ||
SetElementList.Iterator._Set_value<Key, Unique, SourceT>(it, first.value); | ||
const it: SetElementList.Iterator<Key, Unique, SourceT> = SetElementList.Iterator._Create(this, this.uid_++, prev, null, first.value); | ||
SetElementList.Iterator._Set_next<Key, Unique, SourceT>(prev, it); | ||
if (top === null) | ||
top = it; | ||
SetElementList.Iterator._Set_next<Key, Unique, SourceT>(prev, it); | ||
prev = it; | ||
@@ -232,3 +229,3 @@ ++size; | ||
private next_: Iterator<Key, Unique, SourceT> | null; | ||
private value_: Key; | ||
private readonly value_: Key; | ||
@@ -238,3 +235,10 @@ /* --------------------------------------------------------------- | ||
--------------------------------------------------------------- */ | ||
private constructor(container: SetElementList<Key, Unique, SourceT>, uid: usize) | ||
private constructor | ||
( | ||
container: SetElementList<Key, Unique, SourceT>, | ||
uid: usize, | ||
prev: Iterator<Key, Unique, SourceT> | null, | ||
next: Iterator<Key, Unique, SourceT> | null, | ||
value: Key | ||
) | ||
{ | ||
@@ -245,7 +249,8 @@ this.container_ = container; | ||
this.prev_ = null; | ||
this.next_ = null; | ||
this.value_ = changetype<Key>(0); | ||
this.prev_ = prev; | ||
this.next_ = next; | ||
this.value_ = value; | ||
} | ||
@inline() | ||
public static _Create<Key, | ||
@@ -260,11 +265,8 @@ Unique extends boolean, | ||
uid: usize, | ||
prev: Iterator<Key, Unique, SourceT> | null = null, | ||
next: Iterator<Key, Unique, SourceT> | null = null | ||
prev: Iterator<Key, Unique, SourceT> | null, | ||
next: Iterator<Key, Unique, SourceT> | null, | ||
value: Key | ||
): Iterator<Key, Unique, SourceT> | ||
{ | ||
const ret: Iterator<Key, Unique, SourceT> = new Iterator(container, uid); | ||
if (prev) ret.prev_ = prev; | ||
if (next) ret.next_ = next; | ||
return ret; | ||
return new Iterator(container, uid, prev, next, value); | ||
} | ||
@@ -296,14 +298,2 @@ | ||
@inline() | ||
public static _Set_value<Key, | ||
Unique extends boolean, | ||
SourceT extends ISetContainer<Key, Unique, SourceT, | ||
SetElementList<Key, Unique, SourceT>, | ||
Iterator<Key, Unique, SourceT>, | ||
ReverseIterator<Key, Unique, SourceT>>> | ||
(it: Iterator<Key, Unique, SourceT>, value: Key): void | ||
{ | ||
it.value_ = value;; | ||
} | ||
/* --------------------------------------------------------------- | ||
@@ -310,0 +300,0 @@ ITERATORS |
@@ -9,3 +9,3 @@ { | ||
}, | ||
"version": "0.0.0", | ||
"version": "0.0.1", | ||
"main": "./index.ts", | ||
@@ -12,0 +12,0 @@ "scripts": { |
# AssemblyScript Standard Template Library | ||
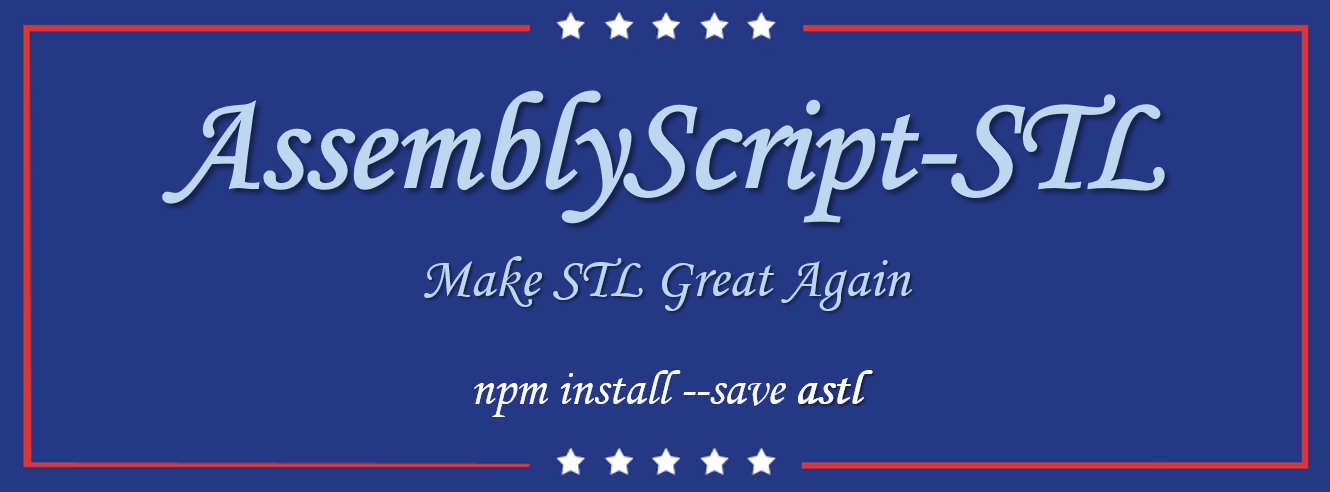 | ||
@@ -3,0 +4,0 @@ [](https://github.com/samchon/astl/blob/master/LICENSE) |
@@ -12,3 +12,3 @@ import std from "../../../index"; | ||
const elements: Array<i32> = []; | ||
for (let i: i32 = 0; i < 200; ++i) | ||
for (let i: i32 = 0; i < 10; ++i) | ||
for (let j: i32 = 0; j < 4; ++j) | ||
@@ -22,3 +22,3 @@ elements.push(i); | ||
adaptor.push_back(value); | ||
adaptor.push(value); | ||
elements.splice(index, 1); | ||
@@ -25,0 +25,0 @@ } |
@@ -1,3 +0,4 @@ | ||
import { test_hash } from "./features/functional/test_hash"; | ||
//---- | ||
// CONTAINERS | ||
//---- | ||
import { test_vector } from "./features/containers/test_vector"; | ||
@@ -9,9 +10,9 @@ import { test_deque } from "./features/containers/test_deque"; | ||
import { test_tree_map } from "./features/containers/test_tree_map"; | ||
import { test_tree_multimap } from "./features/containers/test_tree_multimap"; | ||
import { test_tree_multiset } from "./features/containers/test_tree_multiset"; | ||
import { test_tree_multi_map } from "./features/containers/test_tree_multi_map"; | ||
import { test_tree_multi_set } from "./features/containers/test_tree_multi_set"; | ||
import { test_tree_set } from "./features/containers/test_tree_set"; | ||
import { test_hash_map } from "./features/containers/test_hash_map"; | ||
import { test_hash_multimap } from "./features/containers/test_hash_multimap"; | ||
import { test_hash_multiset } from "./features/containers/test_hash_multiset"; | ||
import { test_hash_multi_map } from "./features/containers/test_hash_multi_map"; | ||
import { test_hash_multi_set } from "./features/containers/test_hash_multi_set"; | ||
import { test_hash_set } from "./features/containers/test_hash_set"; | ||
@@ -24,2 +25,4 @@ | ||
import { test_hash } from "./features/functional/test_hash"; | ||
export function main(): void | ||
@@ -30,2 +33,7 @@ { | ||
//---- | ||
// ALGORITHM | ||
//---- | ||
//---- | ||
// CONTAINERS | ||
@@ -41,9 +49,9 @@ //---- | ||
test_tree_map(); | ||
test_tree_multimap(); | ||
test_tree_multiset(); | ||
test_tree_multi_map(); | ||
test_tree_multi_set(); | ||
test_tree_set(); | ||
test_hash_map(); | ||
test_hash_multimap(); | ||
test_hash_multiset(); | ||
test_hash_multi_map(); | ||
test_hash_multi_set(); | ||
test_hash_set(); | ||
@@ -56,3 +64,4 @@ | ||
// ETC | ||
test_storing_objects(); | ||
} |
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
263741
122
6954
87