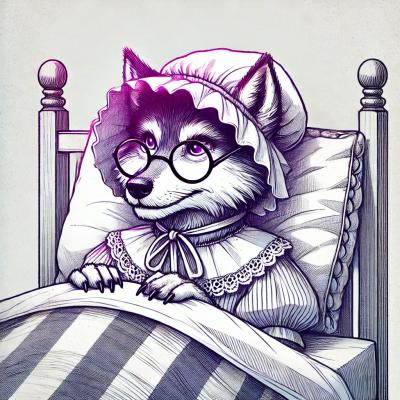
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
async-eventemitter
Advanced tools
Just like EventEmitter, but with support for callbacks and interuption of the listener-chain
The async-eventemitter npm package is an extension of the Node.js EventEmitter that supports asynchronous event handlers. It allows you to handle events with asynchronous functions, making it easier to work with promises and async/await in event-driven programming.
Basic Asynchronous Event Handling
This feature allows you to register an asynchronous event handler using the `on` method. The event handler can use async/await to handle asynchronous operations.
const AsyncEventEmitter = require('async-eventemitter');
const emitter = new AsyncEventEmitter();
emitter.on('event', async (data) => {
await new Promise(resolve => setTimeout(resolve, 1000));
console.log('Event handled with data:', data);
});
emitter.emit('event', 'some data');
Handling Multiple Asynchronous Listeners
This feature demonstrates how multiple asynchronous listeners can be registered for the same event. Each listener will handle the event asynchronously and independently.
const AsyncEventEmitter = require('async-eventemitter');
const emitter = new AsyncEventEmitter();
emitter.on('event', async (data) => {
await new Promise(resolve => setTimeout(resolve, 1000));
console.log('First listener handled with data:', data);
});
emitter.on('event', async (data) => {
await new Promise(resolve => setTimeout(resolve, 500));
console.log('Second listener handled with data:', data);
});
emitter.emit('event', 'some data');
Waiting for All Listeners to Complete
This feature shows how to wait for all asynchronous listeners to complete before proceeding. The `emit` method returns a promise that resolves when all listeners have finished handling the event.
const AsyncEventEmitter = require('async-eventemitter');
const emitter = new AsyncEventEmitter();
emitter.on('event', async (data) => {
await new Promise(resolve => setTimeout(resolve, 1000));
console.log('First listener handled with data:', data);
});
emitter.on('event', async (data) => {
await new Promise(resolve => setTimeout(resolve, 500));
console.log('Second listener handled with data:', data);
});
(async () => {
await emitter.emit('event', 'some data');
console.log('All listeners have completed');
})();
The `events` package is the standard Node.js EventEmitter module. It provides basic event handling capabilities but does not natively support asynchronous event handlers. You can use promises or async/await within event handlers, but the EventEmitter itself does not manage the asynchronous flow.
The `eventemitter2` package is an enhanced version of the Node.js EventEmitter with additional features like wildcard event names and event namespacing. While it offers more flexibility in event handling, it does not specifically focus on asynchronous event handling like async-eventemitter.
The `eventemitter3` package is a lightweight and fast EventEmitter implementation. It is designed for performance and simplicity but does not include built-in support for asynchronous event handlers. It is suitable for scenarios where performance is critical and asynchronous handling is managed manually.
An EventEmitter that supports serial execution of asynchronous event listeners. It also supports event listeners without callbacks (synchronous), as well as interrupting the call-chain (similar to the DOM's e.stopPropagation()).
var AsyncEventEmitter = require('async-eventemitter');
var events = new AsyncEventEmitter();
events.on('test', function (e, next) {
// The next event listener will wait til this is done
setTimeout(next, 1000);
});
events
.on('test', function (e) {
// This is behaves a synchronous event listener (note the lack of a second
// callback argument)
console.log(e);
// { data: 'data' }
})
.on('test', function (e, next) {
// Even if you're not truly asynchronous you can use next() to stop propagation
next(new Error('You shall not pass'));
});
events.trigger('test', { data: 'data' }, function (err) {
// This is run after all of the event listeners are done
console.log(err);
// [Error: You shall not pass]
});
More examples are found in the test
-folder.
The API and behavior of AsyncEventEmitter is as far as possible and meaningful identical to that of the native EventEmitter. However there are some important differences which should be noted.
eg emit(data)
) must always be zero or
one argument, and can not be a function.function(e, next){}
)..emit()
will
be executed before any event listeners.For addListener() on() once() removeListener() removeAllListeners() setMaxListeners() listeners()
see the EventEmitter docs,
nothing new here.
emit(event, [data], [callback])
Executes all listeners for the event in order with the supplied data argument. The optional callback is called when all of the listeners are done.
.first(event, new)
Adds a listener to the beginning of the listeners array for the specified event.
.at(event, index, listener)
Adds a listener at the specified index in the listeners array for the specified event.
.before(event, target, listener)
Adds a listener before the target listener in the listeners array for the specified event.
.after(event, target, listener)
Adds a listener after the target listener in the listeners array for the specified event.
The MIT License (MIT)
Copyright © 2013 Andreas Hultgren
FAQs
Just like EventEmitter, but with support for callbacks and interuption of the listener-chain
The npm package async-eventemitter receives a total of 100,064 weekly downloads. As such, async-eventemitter popularity was classified as popular.
We found that async-eventemitter demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.