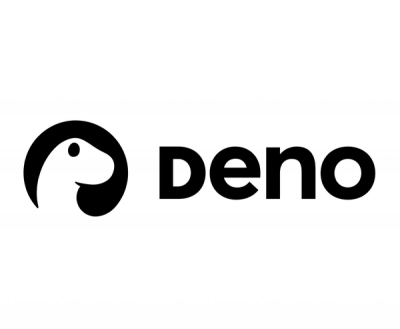
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
async-mqtt-web
Advanced tools
Promise wrapper over MQTT.js
IMPORTANT: Make sure you handle rejections from returned promises because they won't crash the process
The API is the same as MQTT.js, except the following functions now return promises instead of taking callbacks
const MQTT = require("async-mqtt");
const client = MQTT.connect("tcp://somehost.com:1883");
// When passing async functions as event listeners, make sure to have a try catch block
const doStuff = async () => {
console.log("Starting");
try {
await client.publish("wow/so/cool", "It works!");
// This line doesn't run until the server responds to the publish
await client.end();
// This line doesn't run until the client has disconnected without error
console.log("Done");
} catch (e){
// Do something about it!
console.log(e.stack);
process.exit();
}
}
client.on("connect", doStuff);
Alternately you can skip the event listeners and get a promise.
const MQTT = require("async-mqtt");
run()
async function run() {
const client = await MQTT.connectAsync("tcp://somehost.com:1883")
console.log("Starting");
try {
await client.publish("wow/so/cool", "It works!");
// This line doesn't run until the server responds to the publish
await client.end();
// This line doesn't run until the client has disconnected without error
console.log("Done");
} catch (e){
// Do something about it!
console.log(e.stack);
process.exit();
}
}
const { AsyncClient } = require("async-mqtt");
const client = getRegularMQTTClientFromSomewhere();
const asyncClient = new AsyncClient(client);
asyncClient.publish("foo/bar", "baz").then(() => {
console.log("We async now");
return asyncClient.end();
});
FAQs
Promise wrapper over MQTT.js
We found that async-mqtt-web demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.