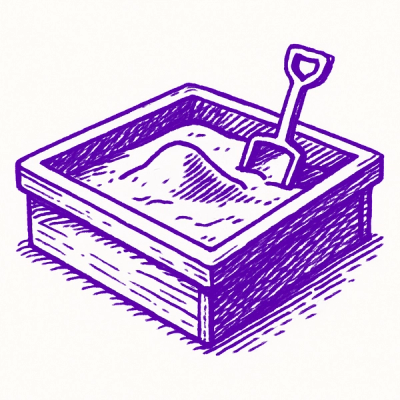
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
async-retry
Advanced tools
The async-retry npm package is designed to handle the execution of asynchronous operations that may fail by retrying them a specified number of times with customizable options. It is useful for dealing with operations like network requests or any other tasks that might intermittently fail due to temporary issues.
Basic retry functionality
This feature allows you to automatically retry an asynchronous operation if it fails. The code sample shows how to use async-retry to fetch data from an API with up to 5 retries.
const retry = require('async-retry');
async function fetchData() {
return retry(async bail => {
// if anything throws, we retry
const result = await fetch('https://api.example.com/data');
if (result.status >= 400) {
throw new Error('Request failed');
}
return result.json();
}, {
retries: 5
});
}
Custom retry strategies
This feature allows you to define a custom retry strategy, including the number of retries, the factor by which the timeout increases, and the minimum and maximum timeout values. The code sample demonstrates setting these options for a more sophisticated retry strategy.
const retry = require('async-retry');
async function fetchDataWithCustomStrategy() {
return retry(async bail => {
// if anything throws, we retry
const result = await fetch('https://api.example.com/data');
if (result.status >= 400) {
throw new Error('Request failed');
}
return result.json();
}, {
retries: 5,
factor: 2,
minTimeout: 1000,
maxTimeout: 5000,
randomize: true
});
}
Bailing out of retries
This feature allows you to bail out of the retry loop early under certain conditions. The code sample shows how to stop retrying if a 404 status is encountered or after a certain number of attempts.
const retry = require('async-retry');
async function fetchDataWithBail() {
return retry(async (bail, attempt) => {
try {
const result = await fetch('https://api.example.com/data');
if (result.status === 404) {
// Don't retry upon 404
bail(new Error('Not found'));
return;
}
return result.json();
} catch (error) {
if (attempt >= 3) {
// Bail on the third attempt
bail(error);
return;
}
throw error;
}
}, {
retries: 5
});
}
The 'retry' package provides similar functionality for retrying operations, with a focus on both synchronous and asynchronous control flow. It offers a more extensive set of options for customizing retry behavior but does not provide an async/await interface out of the box.
The 'p-retry' package is another alternative that supports retrying asynchronous operations using Promises. It has a similar API to async-retry but is built specifically for use with Promises and provides slightly different options for customizing the retry behavior.
The 'retry-axios' package is designed to work as an interceptor for axios, a popular HTTP client. It allows you to automatically retry failed HTTP requests, and it is specifically tailored for axios users, unlike async-retry which is more generic and can be used with any asynchronous operation.
Retrying made simple, easy, and async.
// Packages
const retry = require('async-retry');
const fetch = require('node-fetch');
await retry(
async (bail) => {
// if anything throws, we retry
const res = await fetch('https://google.com');
if (403 === res.status) {
// don't retry upon 403
bail(new Error('Unauthorized'));
return;
}
const data = await res.text();
return data.substr(0, 500);
},
{
retries: 5,
}
);
retry(retrier : Function, opts : Object) => Promise
async
or not. In other words, it can be a function that returns a Promise
or a value.Function
you can invoke to abort the retrying (bail)Number
identifying the attempt. The absolute first attempt (before any retries) is 1
.opts
are passed to node-retry
. Read its docs
retries
: The maximum amount of times to retry the operation. Default is 10
.factor
: The exponential factor to use. Default is 2
.minTimeout
: The number of milliseconds before starting the first retry. Default is 1000
.maxTimeout
: The maximum number of milliseconds between two retries. Default is Infinity
.randomize
: Randomizes the timeouts by multiplying with a factor between 1
to 2
. Default is true
.onRetry
: an optional Function
that is invoked after a new retry is performed. It's passed the Error
that triggered it as a parameter.FAQs
Retrying made simple, easy and async
The npm package async-retry receives a total of 10,248,607 weekly downloads. As such, async-retry popularity was classified as popular.
We found that async-retry demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 80 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.