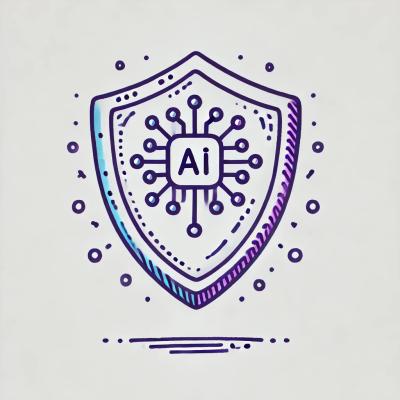
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
async-source
Advanced tools
AsyncSource
is a stateful, reactive data source designed for managing asynchronous requests in modern JavaScript and TypeScript projects. It provides robust features for error handling, throttling, and caching, making it ideal for applications that require efficient data management.
Stateful Management: Track the state of your requests, including (isLoading
, isFetched
, data
).
Error Handling: Automatically manage errors with customizable handlers, ensuring a smooth user experience.
Debounce Control: Integrate built-in debouncing to manage rapid requests, preventing redundant calls and ensuring only the latest call is processed.
Cache Support: Cache responses locally with configurable storage options and expiration times, enhancing performance and reducing unnecessary network requests.
Automatic State Consistency: In cases of multiple calls, only the last call will be processed, maintaining data integrity and consistency.
AsyncSource
to dynamic selects to efficiently handle user interactions and data fetching.npm install --save async-source
// javascript
import AsyncSource from 'async-source';
const source = new AsyncSource(request);
async function loadItems() {
await source.update(...requestParams);
const response = source.data;
}
// typescript
import AsyncSource from 'async-source';
const source = new AsyncSource<RespnonsType>(request);
async function loadItems() {
await source.update(...requestParams);
const response = source.data;
}
Parameter | Is required | Description |
---|---|---|
serviceMethod | true | Method that returns promise |
errorHandler | false | Function that will be called in case of service method rejected |
delay | false | delay in ms |
Property | Description | Type |
---|---|---|
data | Returns your method response | Your method response |
isLoading | Returns true when request pending | Boolean |
isFetch | Returns true after first load | Boolean |
Method | Description | Params |
---|---|---|
update | Calls be request | Your method request params |
push | Calls be request and handles success response | Success handler, Your method request params |
updateIfEmpty | Calls be request in source if data is empty | Your method request params |
updateOnce | Calls be request in source if data is empty and waits till other request resolved | Your method request params |
updateImmediate | Calls be request in source ignoring debounce | Your method request params |
clear | Set source data to initial state(null) | --- |
<template>
<ul v-loading="isLoading">
<li v-for="item in items" :key="item.id">
{{ item.name }}
</li>
</ul>
</template>
<script>
import AsyncSource from 'async-source';
import { computed, reactive } from 'vue';
export default {
name: 'MyComponent',
setup() {
function errorHandler(error) {
console.error(error);
}
const source = reactive(new AsyncSource(request, errorHandler, 300));
source.update();
const items = computed(() => source.data || []);
const isLoading = computed(() => source.isLoading);
return {
items,
isLoading
};
}
}
</script>
<template>
<ul v-loading="isLoading">
<li v-for="item in items" :key="item.id">
{{ item.name }}
</li>
</ul>
</template>
<script>
import AsyncSource from 'async-source';
export default {
name: 'MyComponent',
data() {
return {
source: new AsyncSource(request, this.errorHandler, 300)
}
},
computed: {
items() {
return this.source.data || [];
},
isLoading() {
return this.source.isLoading;
}
},
created() {
this.source.update();
},
methods: {
errorHandler(error) {
console.error(error);
}
}
}
</script>
import AsyncSource from 'async-source';
AsyncSource.setConfig({
cacheStorage: localStorage, // or any custom sync/async storage
cacheTime: 12 * 60 * 60 * 1000, // cache for 12 hours
cachePrefix: 'MyAppAsyncSource' // optional prefix for cache keys
});
new AsyncSource<T>(
serviceMethod: (...args: any[]) => Promise<T>,
errorHandler?: (error: any) => void,
configOrDelay?: number | ConfigOptions
)
Parameter | Required | Description |
---|---|---|
serviceMethod | Yes | Method that returns a promise. |
errorHandler | No | Function to handle errors in the service method. |
configOrDelay | No | Debounce delay (in ms) or an object with config options. |
interface ConfigOptions {
debounceTime?: number; // Delay before request execution (in milliseconds)
requestCacheKey?: string; // Request cache key (required for enable cache)
cacheTime?: number; // Cache expiration in milliseconds
cacheStorage?: CacheStorage; // Storage (e.g., localStorage, sessionStorage, indexDB)
isUpdateCache?: boolean; // Refetch cache every time when true.
}
Option | Type | Default | Description |
---|---|---|---|
debounceTime | number | 300 | Delay before request execution (in milliseconds). |
requestCacheKey | string | Request cache key (required for enabling cache). | |
cacheTime | number | 43200000 | Cache expiration time in milliseconds (default: 12 hours). |
cacheStorage | CacheStorage | localStorage | Storage interface (e.g., localStorage, sessionStorage, indexedDB). |
isUpdateCache | boolean | true | Refetch cache every time when true. |
<template>
<ul v-loading="isLoading">
<li v-for="item in items" :key="item.id">
{{ item.name }}
</li>
</ul>
</template>
<script>
import AsyncSource from 'async-source';
import { computed, reactive } from 'vue';
export default {
name: 'MyComponent',
setup() {
function errorHandler(error) {
console.error(error);
}
const source = reactive(
new AsyncSource(request, errorHandler, {
requestCacheKey: 'key',
cacheStorage: sessionStorage,
isUpdateCache: false,
debounceTime: 100,
cacheTime: 24 * 60 * 60 * 1000 // 24h
})
);
source.update();
const items = computed(() => source.data || []);
const isLoading = computed(() => source.isLoading);
return {
items,
isLoading
};
}
}
</script>
<template>
<ul v-loading="isLoading">
<li v-for="item in items" :key="item.id">
{{ item.name }}
</li>
</ul>
</template>
<script>
import AsyncSource from 'async-source';
import { computed, reactive } from 'vue';
import { get, set, del } from 'idb-keyval';
export default {
name: 'MyComponent',
setup() {
function errorHandler(error) {
console.error(error);
}
const storage = {
getItem: (key: string) => get(key),
setItem: (key: string, value: string) => set(key, value),
removeItem: (key: string) => del(key)
};
const source = reactive(
new AsyncSource(request, errorHandler, {
requestCacheKey: 'key',
cacheStorage: storage,
isUpdateCache: false,
debounceTime: 100,
cacheTime: 24 * 60 * 60 * 1000 // 24h
})
);
source.update();
const items = computed(() => source.data || []);
const isLoading = computed(() => source.isLoading);
return {
items,
isLoading
};
}
}
</script>
FAQs
async requests wrapper
The npm package async-source receives a total of 0 weekly downloads. As such, async-source popularity was classified as not popular.
We found that async-source demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.