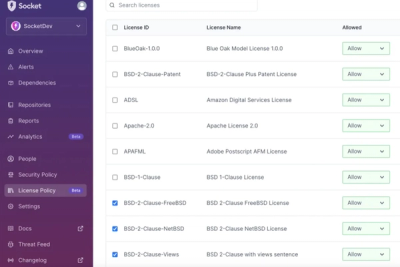
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
A utility for creating aysnc functions.
npm install asyncy
The code is not transpiled for ES5 or lower and requires support of async/await ... which is kind of the point. The intent is to support converting callback or Promise based code to async/await code. If your target environment does not support async/await, you could use this as part of a project that you would then transpile in its entirety.
The current releases of Chrome, Firefox, Edge, and Node.js support the raw, un-transpiled code.
For the highest performance you can use asyncy
inline like this:
const {err,result,args} = await asyncy.inline(fs,fs.readFile,"mystuff.json");
The first argument can either be null
or the scope in which the function should be invoked, i.e. the this
context.
err
will be null
or an Error
instance
result
will be undefined
or an Array
(in order to support callbacks that tak emultiple arguments). In most cases, the calling code will access result[0].
args
will be the arguments that were provided. This is useful for debugging why an Error
was generated.
As should be evident from the above, asyncy
is designed to return
rather than throw
errors in order to keep application logic a little cleaner.
For those desiring throw
behavior, just insert a throw below the await
, e.g:
const {err,result,args} = await asyncy.inline(fs,fs.readFile,"mystuff.json");
if(err) throw err;
If the last argument to asyncy.inline
is a function, it will be treated like a callback and called prior to await
returning, e.g.:
const {err,result,args} = await asyncy.inline(fs,fs.readFile,"mystuff.json",(err,arg1[,arg2...]) => { console.log(arg1,"allocated"); });
Note how the "normal" call signature will be used for the callback, if there are multiple arguments.
For highly standardized functions that invoke callbacks with the signature <cb>(err,result)
, like fs.readFile
, it is unlikely one would do the above; however, if
there is an existing code-base with complex code in a callback, it may be more convenient to execute the code in the callback rather
that move it to a sequential step after the await like the below:
const {err,result,args} = await asyncy.inline(fs,fs.readFile,"mystuff.json");
if(err) ... handle error ...
console.log(result[0],"allocated");
To redefine functions, you can use asyncy
like this:
const readFile = asyncy(fs.readFile),
{err,result,args} = await readFile("mystuff.json");
There is no contect provided at re-define time; however, it can be provided at invocation time, e.g. readFile.call(scope,fileName)
.
You can also async
an entire object (asyncy
will skip functions with names that end in the token "Sync", where "S" is capitalized.):
fs = asyncy(fs,true),
{err,result,args} = await fs.readFile("mystuff.json");
you can optionallly provide an output target when asyncing objects so as not to overwrite the methods on the object being asynced:
const myfs = {};
asyncy(fs,true,myfs),
{err,result,args} = await myfs.readFile("mystuff.json");
2017-06-03 v0.0.3 improved documentation
2017-06-03 v0.0.2 Simplified code, added documentation
2017-06-02 Initial public release
FAQs
The Asyncy node module. Helpers library to interact with the asyncy ecosystem
We found that asyncy demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.