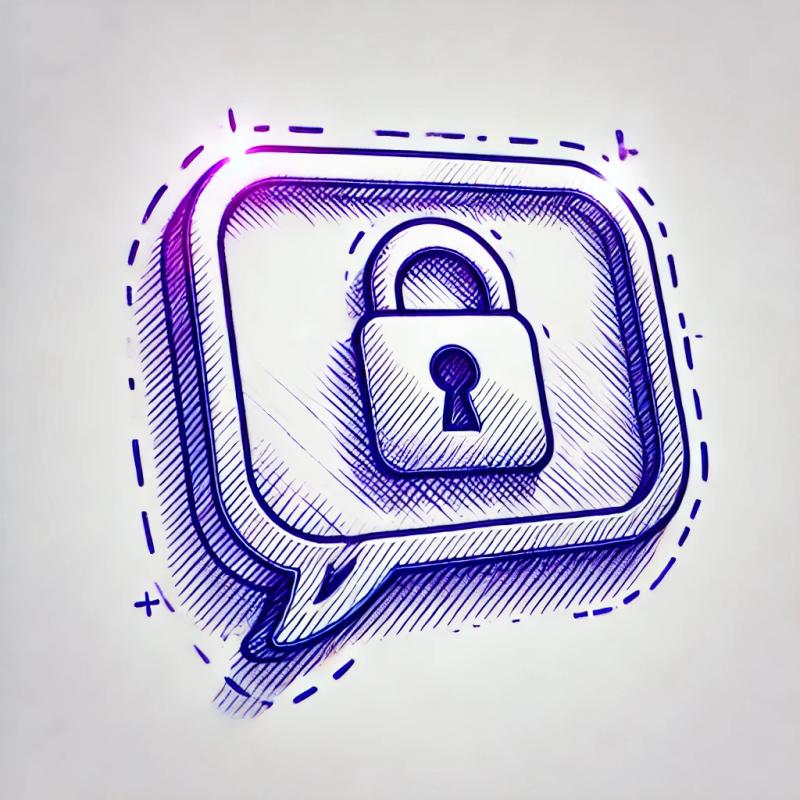
Security News
OpenSSF Warns of Reputation Farming Leveraging Closed GitHub Issues and PRs
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.