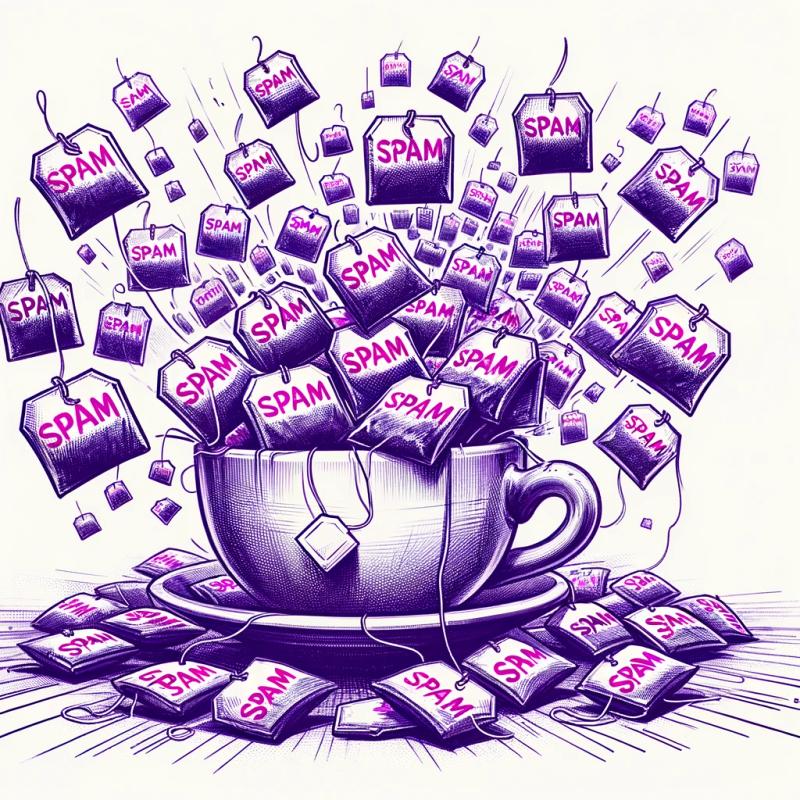
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
audio-loader
Advanced tools
Readme
An simple and flexible audio buffer loader for browser and node:
var load = require('audio-loader')
// load one file
load('http://example.net/audio/file.mp3').then(function (buffer) {
console.log(buffer) // => <AudioBuffer>
})
// load a collection of files
load({ snare: 'samples/snare.wav', kick: 'samples/kick.wav' },
{ from: 'http://example.net/'} ).then(function (audio) {
console.log(audio) // => { snare: <AudioBuffer>, kick: <AudioBuffer> }
})
Npm
Via npm: npm i --save audio-loader
Browser
Download the minified distribution (4kb) which exports loadAudio
as window global:
<script src="audio-loader.min.js"></script>
<script>
loadAudio('file.wav').then(..)
</script>
You can load individual or collection of files:
load('http://path/to/file.mp3').then(function (buffer) {
// buffer is an AudioBuffer
play(buffer)
})
// apply a prefix using options.from
load(['snare.mp3', 'kick.mp3'], { from: 'http://server.com/audio/' }).then(function (buffers) {
// buffers is an array of AudioBuffers
play(buffers[0])
})
// the options.from can be a function
function toUrl (name) { return 'http://server.com/samples' + name + '?key=secret' }
load({ snare: 'snare.mp3', kick: 'kick.mp3' }, { from: toUrl }).then(function (buffers) {
// buffers is a hash of names to AudioBuffers
play(buffers['snare'])
})
audio-loader
will detect if some of the values of an object is an audio file name and try to fetch it:
var inst = { name: 'piano', gain: 0.2, audio: 'samples/piano.mp3' }
load(inst).then(function (piano) {
console.log(piano.name) // => 'piano' (it's not an audio file)
console.log(piano.gain) // => 0.2 (it's not an audio file)
console.log(piano.audio) // => <AudioBuffer> (it loaded the file)
})
If you provide a .js
file, audio-loader
will interpret it as a midi.js soundfont file and try to load it:
load('acoustic_grand_piano-ogg.js').then(function (buffers) {
buffers['C2'] // => <AudioBuffer>
})
This is a repository of them: https://github.com/gleitz/midi-js-soundfonts
load(source, [options])
Param | Type | Description |
---|---|---|
source | Object | the object to be loaded: can be an URL string, ArrayBuffer with encoded data or an array/map of sources |
options | Object | (Optional) the load options for that source |
Possible options
keys are:
load('snare.mp3', { from: 'http://audio.net/samples/' })
If it's a function it receives the file name and should return the url as string.load('piano.json', { only: ['C2', 'D2'] })
audio-context
To run the test, clone this repo and:
npm install
npm test
To run the browser example:
npm i -g budo
npm run example-browser
To run the node (audiojs) example:
node example/node.js
MIT License
FAQs
A flexible web audio sample loader for browser and node
The npm package audio-loader receives a total of 654 weekly downloads. As such, audio-loader popularity was classified as not popular.
We found that audio-loader demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.