aug-compile
Advanced tools
Comparing version 1.0.2 to 2.0.0
@@ -6,2 +6,6 @@ /** | ||
*/ | ||
const augWebkitPrefix = typeof CSS !== "undefined" && CSS.supports && CSS.supports( | ||
"((--foo: , 0 0) and (-webkit-clip-path: polygon(0 0, 100% 0, 50% 50%)) and (not (clip-path: polygon(0 0, 100% 0, 50% 50%))))" | ||
) ? "-webkit-" : "" | ||
const augCompileSheet = (() => { | ||
@@ -15,4 +19,5 @@ const d = document | ||
})() | ||
augCompileSheet.insertRule( | ||
`[data-augmented-ui-compiled]::after { | ||
`[data-augmented-ui-border-compiled], [data-augmented-ui-compiled]::after { | ||
position: absolute; | ||
@@ -27,3 +32,3 @@ top: 0px; | ||
augCompileSheet.insertRule( | ||
`[data-augmented-ui-compiled]::before { | ||
`[data-augmented-ui-inlay-compiled], [data-augmented-ui-compiled]::before { | ||
position: absolute; | ||
@@ -44,12 +49,40 @@ top: 0px; | ||
const augCompileEl = function (augEl) { | ||
let x = augCompileSheet.cssRules.length | ||
const augCompileFeatureWrites = function (augEl, id, pseudoSelector, type, x, writes) { | ||
const cs = getComputedStyle(augEl, pseudoSelector) | ||
const clip = cs.getPropertyValue("clip-path") | ||
if (clip.length > 20) { | ||
const op = `opacity: ${cs.getPropertyValue("opacity")};` | ||
const bg = `background: ${cs.getPropertyValue(`--aug-${type}-bg`) || `var(--aug-${type}-bg)`};` | ||
writes.push({ fn: "css", css: `[data-augmented-ui-compiled~="${id}"]${pseudoSelector} { content: ""; ${augWebkitPrefix}clip-path: ${clip}; ${op} ${bg} }`, x }) | ||
x++ | ||
} | ||
return x | ||
} | ||
const augCompileDelegatesWrites = function (augEl, id, type, x, writes) { | ||
const daut = "data-augmented-ui-" + type | ||
augEl.querySelectorAll("[" + daut + "]").forEach(el => { | ||
if (el.closest("[data-augmented-ui]") === augEl) { | ||
const cs = getComputedStyle(el) | ||
const clip = cs.getPropertyValue("clip-path") | ||
if (clip.length > 20) { | ||
const op = `opacity: ${cs.getPropertyValue("opacity")};` | ||
const bg = `background: ${cs.getPropertyValue(`--aug-${type}-bg`) || `var(--aug-${type}-bg)`};` | ||
writes.push({ fn: "css", css: `[${daut}-compiled~="${id}"] { ${augWebkitPrefix}clip-path: ${clip}; ${op} ${bg} }`, x }) | ||
writes.push({ fn: "setAttribute", target: el, prop: daut + "-compiled", val: id + " " + (augEl.getAttribute(daut) || "") }) | ||
writes.push({ fn: "removeAttribute", target: el, prop: daut }) | ||
x++ | ||
} | ||
} | ||
}) | ||
return x | ||
} | ||
const augCompileElWrites = function (augEl, x, writes) { | ||
const id = "compile-" + x.toString(36) | ||
const val = (id + " " + augEl.getAttribute("data-augmented-ui")).trim() | ||
const hasAllAug = /\ball-./.test(val) | ||
const cs1 = getComputedStyle(augEl) | ||
const clip1 = cs1.getPropertyValue("clip-path") | ||
const usewebkit = CSS.supports("((--foo: , 0 0) and (-webkit-clip-path: polygon(0 0, 100% 0, 50% 50%)) and (not (clip-path: polygon(0 0, 100% 0, 50% 50%))))") | ||
const webkit = usewebkit ? "-webkit-" : "" | ||
if (clip1.length > 20) { | ||
const augs = (id + " " + augEl.getAttribute("data-augmented-ui")).trim() | ||
const hasAllAug = /\ball-./.test(augs) | ||
const cs = getComputedStyle(augEl) | ||
const clip = cs.getPropertyValue("clip-path") | ||
if (clip.length > 20) { | ||
// augmented-ui internal static minification map | ||
@@ -59,39 +92,101 @@ // "--aug__elwidth": "--aug_at", | ||
// reading the vars instead of width/height directly mantains the author's (possibly flexible) units instead of forcing px | ||
const wd = hasAllAug ? `width: calc(${cs1.getPropertyValue("--aug_at") || cs1.getPropertyValue("--aug__elwidth")});` : "" | ||
const ht = hasAllAug ? `height: calc(${cs1.getPropertyValue("--aug_au") || cs1.getPropertyValue("--aug__elheight")});` : "" | ||
augCompileSheet.insertRule( `[data-augmented-ui-compiled~="${id}"] { ${webkit}clip-path: ${clip1}; ${wd} ${ht} }`, x ) | ||
const wd = hasAllAug ? `width: calc(${cs.getPropertyValue("--aug_at") || cs.getPropertyValue("--aug__elwidth")});` : "" | ||
const ht = hasAllAug ? `height: calc(${cs.getPropertyValue("--aug_au") || cs.getPropertyValue("--aug__elheight")});` : "" | ||
writes.push({ fn: "css", css: `[data-augmented-ui-compiled~="${id}"] { ${augWebkitPrefix}clip-path: ${clip}; ${wd} ${ht} }`, x }) | ||
x++ | ||
} | ||
const cs2 = getComputedStyle(augEl, "::before") | ||
const clip2 = cs2.getPropertyValue("clip-path") | ||
if (clip2.length > 20) { | ||
const op = `opacity: ${cs2.getPropertyValue("opacity")};` | ||
const bg = `background: ${cs2.getPropertyValue("background") || cs2.getPropertyValue("--aug-inlay-bg") || "var(--aug-inlay-bg)"};` | ||
augCompileSheet.insertRule( `[data-augmented-ui-compiled~="${id}"]::before { content: ""; ${webkit}clip-path: ${clip2}; ${op} ${bg} }`, x ) | ||
x++ | ||
if (cs.getPropertyValue("--aug-delegated-inlay") === "") { // initial in js land is an empty string | ||
x = augCompileDelegatesWrites(augEl, id, "inlay", x, writes) | ||
} else { | ||
x = augCompileFeatureWrites(augEl, id, "::before", "inlay", x, writes) | ||
} | ||
const cs3 = getComputedStyle(augEl, "::after") | ||
const clip3 = cs3.getPropertyValue("clip-path") | ||
if (clip3.length > 20) { | ||
const op = `opacity: ${cs3.getPropertyValue("opacity")};` | ||
const bg = `background: ${cs3.getPropertyValue("background") || cs3.getPropertyValue("--aug-border-bg") || "var(--aug-border-bg)"};` | ||
augCompileSheet.insertRule( `[data-augmented-ui-compiled~="${id}"]::after { content: ""; ${webkit}clip-path: ${clip3}; ${op} ${bg} }`, x ) | ||
x++ | ||
if (cs.getPropertyValue("--aug-delegated-border") === "") { // initial in js land is an empty string | ||
x = augCompileDelegatesWrites(augEl, id, "border", x, writes) | ||
} else { | ||
x = augCompileFeatureWrites(augEl, id, "::after", "border", x, writes) | ||
} | ||
augEl.removeAttribute("data-augmented-ui") | ||
augEl.setAttribute("data-augmented-ui-compiled", val) | ||
writes.push({ fn: "removeAttribute", target: augEl, prop: "data-augmented-ui" }) | ||
writes.push({ fn: "setAttribute", target: augEl, prop: "data-augmented-ui-compiled", val: augs }) | ||
return x | ||
} | ||
const augCompileAll = function (qs) { | ||
const augWriteAll = function (writes) { | ||
let cssCount = 0 | ||
for (let i = 0; i < writes.length; i++) { | ||
const write = writes[i] | ||
switch (write.fn) { | ||
case "css": { | ||
augCompileSheet.insertRule(write.css, write.x) | ||
cssCount++ | ||
break | ||
} | ||
case "setAttribute": { | ||
write.target.setAttribute(write.prop, write.val) | ||
break | ||
} | ||
case "removeAttribute": { | ||
write.target.removeAttribute(write.prop) | ||
break | ||
} | ||
} | ||
} | ||
return cssCount | ||
} | ||
const augCompileElSync = function (augEl) { | ||
// gather writes for the end of the frame, do all reads first | ||
const writes = [] | ||
augCompileElWrites(augEl, augCompileSheet.cssRules.length, writes) | ||
return augWriteAll(writes) | ||
} | ||
const augCompileEl = function (augEl) { | ||
const onFrame = ts => augCompileElSync(augEl) | ||
return (new Promise(requestAnimationFrame)).then(onFrame) | ||
} | ||
const augCompileAllSync = function (qs) { | ||
// gather writes for the end of the frame, do all reads first | ||
const writes = [] | ||
const targets = document.querySelectorAll(qs || '[data-augmented-ui~="compile"]') | ||
targets.forEach(augCompileEl) | ||
let x = augCompileSheet.cssRules.length | ||
targets.forEach(el => { | ||
x = augCompileElWrites(el, x, writes) | ||
}) | ||
return augWriteAll(writes) | ||
} | ||
const augCompileAll = function (qs) { | ||
const onFrame = ts => augCompileAllSync(qs, ts) | ||
return (new Promise(requestAnimationFrame)).then(onFrame) | ||
} | ||
const augCompileRevertEl = function (el) { | ||
const val = el.getAttribute("data-augmented-ui-compiled").replace(/compile-[a-z0-9]+/gi, "") | ||
let id | ||
const augs = el.getAttribute("data-augmented-ui-compiled").replace(/compile-[a-z0-9]+/gi, _ => ((id = _), "")) | ||
el.removeAttribute("data-augmented-ui-compiled") | ||
el.setAttribute("data-augmented-ui", val) | ||
el.setAttribute("data-augmented-ui", augs) | ||
el.querySelectorAll("[data-augmented-ui-inlay-compiled~=" + id + "]").forEach(el => { | ||
el.setAttribute("data-augmented-ui-inlay", el.getAttribute("data-augmented-ui-inlay-compiled").replace(/compile-[a-z0-9]+/gi, "")) | ||
el.removeAttribute("data-augmented-ui-inlay-compiled") | ||
}) | ||
el.querySelectorAll("[data-augmented-ui-border-compiled~=" + id + "]").forEach(el => { | ||
el.setAttribute("data-augmented-ui-border", el.getAttribute("data-augmented-ui-border-compiled").replace(/compile-[a-z0-9]+/gi, "")) | ||
el.removeAttribute("data-augmented-ui-border-compiled") | ||
}) | ||
const idTest = new RegExp("\\b" + id + "\\b") | ||
const rules = augCompileSheet.cssRules | ||
const rlen = rules.length | ||
let deleted = 0 | ||
for (let i = rlen - 1; i >= 0; i--) { | ||
const r = rules[i] | ||
if (idTest.test(r.selectorText)) { | ||
augCompileSheet.deleteRule(i) | ||
deleted++ | ||
} | ||
} | ||
return deleted | ||
} | ||
export { augCompileEl, augCompileAll, augCompileRevertEl } | ||
export { augCompileEl, augCompileElSync, augCompileAll, augCompileAllSync, augCompileRevertEl } | ||
export default augCompileAll |
@@ -6,2 +6,6 @@ /** | ||
*/ | ||
const augWebkitPrefix = typeof CSS !== "undefined" && CSS.supports && CSS.supports( | ||
"((--foo: , 0 0) and (-webkit-clip-path: polygon(0 0, 100% 0, 50% 50%)) and (not (clip-path: polygon(0 0, 100% 0, 50% 50%))))" | ||
) ? "-webkit-" : "" | ||
const augCompileSheet = (() => { | ||
@@ -15,4 +19,5 @@ const d = document | ||
})() | ||
augCompileSheet.insertRule( | ||
`[data-augmented-ui-compiled]::after { | ||
`[data-augmented-ui-border-compiled], [data-augmented-ui-compiled]::after { | ||
position: absolute; | ||
@@ -27,3 +32,3 @@ top: 0px; | ||
augCompileSheet.insertRule( | ||
`[data-augmented-ui-compiled]::before { | ||
`[data-augmented-ui-inlay-compiled], [data-augmented-ui-compiled]::before { | ||
position: absolute; | ||
@@ -44,12 +49,40 @@ top: 0px; | ||
const augCompileEl = function (augEl) { | ||
let x = augCompileSheet.cssRules.length | ||
const augCompileFeatureWrites = function (augEl, id, pseudoSelector, type, x, writes) { | ||
const cs = getComputedStyle(augEl, pseudoSelector) | ||
const clip = cs.getPropertyValue("clip-path") | ||
if (clip.length > 20) { | ||
const op = `opacity: ${cs.getPropertyValue("opacity")};` | ||
const bg = `background: ${cs.getPropertyValue(`--aug-${type}-bg`) || `var(--aug-${type}-bg)`};` | ||
writes.push({ fn: "css", css: `[data-augmented-ui-compiled~="${id}"]${pseudoSelector} { content: ""; ${augWebkitPrefix}clip-path: ${clip}; ${op} ${bg} }`, x }) | ||
x++ | ||
} | ||
return x | ||
} | ||
const augCompileDelegatesWrites = function (augEl, id, type, x, writes) { | ||
const daut = "data-augmented-ui-" + type | ||
augEl.querySelectorAll("[" + daut + "]").forEach(el => { | ||
if (el.closest("[data-augmented-ui]") === augEl) { | ||
const cs = getComputedStyle(el) | ||
const clip = cs.getPropertyValue("clip-path") | ||
if (clip.length > 20) { | ||
const op = `opacity: ${cs.getPropertyValue("opacity")};` | ||
const bg = `background: ${cs.getPropertyValue(`--aug-${type}-bg`) || `var(--aug-${type}-bg)`};` | ||
writes.push({ fn: "css", css: `[${daut}-compiled~="${id}"] { ${augWebkitPrefix}clip-path: ${clip}; ${op} ${bg} }`, x }) | ||
writes.push({ fn: "setAttribute", target: el, prop: daut + "-compiled", val: id + " " + (augEl.getAttribute(daut) || "") }) | ||
writes.push({ fn: "removeAttribute", target: el, prop: daut }) | ||
x++ | ||
} | ||
} | ||
}) | ||
return x | ||
} | ||
const augCompileElWrites = function (augEl, x, writes) { | ||
const id = "compile-" + x.toString(36) | ||
const val = (id + " " + augEl.getAttribute("data-augmented-ui")).trim() | ||
const hasAllAug = /\ball-./.test(val) | ||
const cs1 = getComputedStyle(augEl) | ||
const clip1 = cs1.getPropertyValue("clip-path") | ||
const usewebkit = CSS.supports("((--foo: , 0 0) and (-webkit-clip-path: polygon(0 0, 100% 0, 50% 50%)) and (not (clip-path: polygon(0 0, 100% 0, 50% 50%))))") | ||
const webkit = usewebkit ? "-webkit-" : "" | ||
if (clip1.length > 20) { | ||
const augs = (id + " " + augEl.getAttribute("data-augmented-ui")).trim() | ||
const hasAllAug = /\ball-./.test(augs) | ||
const cs = getComputedStyle(augEl) | ||
const clip = cs.getPropertyValue("clip-path") | ||
if (clip.length > 20) { | ||
// augmented-ui internal static minification map | ||
@@ -59,36 +92,98 @@ // "--aug__elwidth": "--aug_at", | ||
// reading the vars instead of width/height directly mantains the author's (possibly flexible) units instead of forcing px | ||
const wd = hasAllAug ? `width: calc(${cs1.getPropertyValue("--aug_at") || cs1.getPropertyValue("--aug__elwidth")});` : "" | ||
const ht = hasAllAug ? `height: calc(${cs1.getPropertyValue("--aug_au") || cs1.getPropertyValue("--aug__elheight")});` : "" | ||
augCompileSheet.insertRule( `[data-augmented-ui-compiled~="${id}"] { ${webkit}clip-path: ${clip1}; ${wd} ${ht} }`, x ) | ||
const wd = hasAllAug ? `width: calc(${cs.getPropertyValue("--aug_at") || cs.getPropertyValue("--aug__elwidth")});` : "" | ||
const ht = hasAllAug ? `height: calc(${cs.getPropertyValue("--aug_au") || cs.getPropertyValue("--aug__elheight")});` : "" | ||
writes.push({ fn: "css", css: `[data-augmented-ui-compiled~="${id}"] { ${augWebkitPrefix}clip-path: ${clip}; ${wd} ${ht} }`, x }) | ||
x++ | ||
} | ||
const cs2 = getComputedStyle(augEl, "::before") | ||
const clip2 = cs2.getPropertyValue("clip-path") | ||
if (clip2.length > 20) { | ||
const op = `opacity: ${cs2.getPropertyValue("opacity")};` | ||
const bg = `background: ${cs2.getPropertyValue("background") || cs2.getPropertyValue("--aug-inlay-bg") || "var(--aug-inlay-bg)"};` | ||
augCompileSheet.insertRule( `[data-augmented-ui-compiled~="${id}"]::before { content: ""; ${webkit}clip-path: ${clip2}; ${op} ${bg} }`, x ) | ||
x++ | ||
if (cs.getPropertyValue("--aug-delegated-inlay") === "") { // initial in js land is an empty string | ||
x = augCompileDelegatesWrites(augEl, id, "inlay", x, writes) | ||
} else { | ||
x = augCompileFeatureWrites(augEl, id, "::before", "inlay", x, writes) | ||
} | ||
const cs3 = getComputedStyle(augEl, "::after") | ||
const clip3 = cs3.getPropertyValue("clip-path") | ||
if (clip3.length > 20) { | ||
const op = `opacity: ${cs3.getPropertyValue("opacity")};` | ||
const bg = `background: ${cs3.getPropertyValue("background") || cs3.getPropertyValue("--aug-border-bg") || "var(--aug-border-bg)"};` | ||
augCompileSheet.insertRule( `[data-augmented-ui-compiled~="${id}"]::after { content: ""; ${webkit}clip-path: ${clip3}; ${op} ${bg} }`, x ) | ||
x++ | ||
if (cs.getPropertyValue("--aug-delegated-border") === "") { // initial in js land is an empty string | ||
x = augCompileDelegatesWrites(augEl, id, "border", x, writes) | ||
} else { | ||
x = augCompileFeatureWrites(augEl, id, "::after", "border", x, writes) | ||
} | ||
augEl.removeAttribute("data-augmented-ui") | ||
augEl.setAttribute("data-augmented-ui-compiled", val) | ||
writes.push({ fn: "removeAttribute", target: augEl, prop: "data-augmented-ui" }) | ||
writes.push({ fn: "setAttribute", target: augEl, prop: "data-augmented-ui-compiled", val: augs }) | ||
return x | ||
} | ||
const augCompileAll = function (qs) { | ||
const augWriteAll = function (writes) { | ||
let cssCount = 0 | ||
for (let i = 0; i < writes.length; i++) { | ||
const write = writes[i] | ||
switch (write.fn) { | ||
case "css": { | ||
augCompileSheet.insertRule(write.css, write.x) | ||
cssCount++ | ||
break | ||
} | ||
case "setAttribute": { | ||
write.target.setAttribute(write.prop, write.val) | ||
break | ||
} | ||
case "removeAttribute": { | ||
write.target.removeAttribute(write.prop) | ||
break | ||
} | ||
} | ||
} | ||
return cssCount | ||
} | ||
const augCompileElSync = function (augEl) { | ||
// gather writes for the end of the frame, do all reads first | ||
const writes = [] | ||
augCompileElWrites(augEl, augCompileSheet.cssRules.length, writes) | ||
return augWriteAll(writes) | ||
} | ||
const augCompileEl = function (augEl) { | ||
const onFrame = ts => augCompileElSync(augEl) | ||
return (new Promise(requestAnimationFrame)).then(onFrame) | ||
} | ||
const augCompileAllSync = function (qs) { | ||
// gather writes for the end of the frame, do all reads first | ||
const writes = [] | ||
const targets = document.querySelectorAll(qs || '[data-augmented-ui~="compile"]') | ||
targets.forEach(augCompileEl) | ||
let x = augCompileSheet.cssRules.length | ||
targets.forEach(el => { | ||
x = augCompileElWrites(el, x, writes) | ||
}) | ||
return augWriteAll(writes) | ||
} | ||
const augCompileAll = function (qs) { | ||
const onFrame = ts => augCompileAllSync(qs, ts) | ||
return (new Promise(requestAnimationFrame)).then(onFrame) | ||
} | ||
const augCompileRevertEl = function (el) { | ||
const val = el.getAttribute("data-augmented-ui-compiled").replace(/compile-[a-z0-9]+/gi, "") | ||
let id | ||
const augs = el.getAttribute("data-augmented-ui-compiled").replace(/compile-[a-z0-9]+/gi, _ => ((id = _), "")) | ||
el.removeAttribute("data-augmented-ui-compiled") | ||
el.setAttribute("data-augmented-ui", val) | ||
el.setAttribute("data-augmented-ui", augs) | ||
el.querySelectorAll("[data-augmented-ui-inlay-compiled~=" + id + "]").forEach(el => { | ||
el.setAttribute("data-augmented-ui-inlay", el.getAttribute("data-augmented-ui-inlay-compiled").replace(/compile-[a-z0-9]+/gi, "")) | ||
el.removeAttribute("data-augmented-ui-inlay-compiled") | ||
}) | ||
el.querySelectorAll("[data-augmented-ui-border-compiled~=" + id + "]").forEach(el => { | ||
el.setAttribute("data-augmented-ui-border", el.getAttribute("data-augmented-ui-border-compiled").replace(/compile-[a-z0-9]+/gi, "")) | ||
el.removeAttribute("data-augmented-ui-border-compiled") | ||
}) | ||
const idTest = new RegExp("\\b" + id + "\\b") | ||
const rules = augCompileSheet.cssRules | ||
const rlen = rules.length | ||
let deleted = 0 | ||
for (let i = rlen - 1; i >= 0; i--) { | ||
const r = rules[i] | ||
if (idTest.test(r.selectorText)) { | ||
augCompileSheet.deleteRule(i) | ||
deleted++ | ||
} | ||
} | ||
return deleted | ||
} |
{ | ||
"name": "aug-compile", | ||
"version": "1.0.2", | ||
"version": "2.0.0", | ||
"description": "Get the most out of your runtime performance by compiling static augmented-ui configurations up front", | ||
@@ -5,0 +5,0 @@ "main": "aug-compile-es6.js", |
@@ -6,2 +6,4 @@  | ||
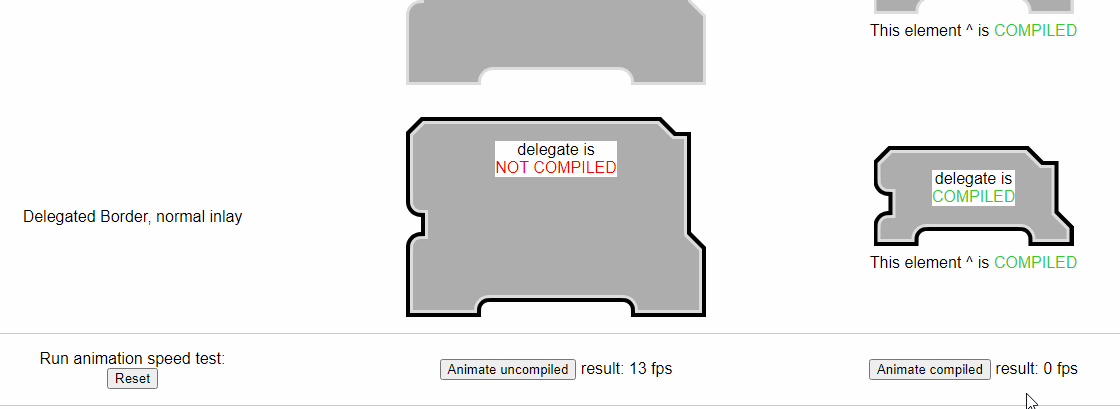 | ||
If you've got animations or other dynamic content that causes augmented-ui elements to be repainted unnecessarily (like setting any --css-var on any ancestor in the dom), you can use `aug-compile` to remove all the customizable overhead (and the associated memory overhead) from static augmented-ui elements for an as-fast-as-possible repaint. | ||
@@ -22,3 +24,3 @@ | ||
or | ||
import { augCompileEl, augCompileAll, augCompileRevertEl } from "aug-compile" | ||
import { augCompileEl, augCompileElSync, augCompileAll, augCompileAllSync, augCompileRevertEl } from "aug-compile" | ||
``` | ||
@@ -64,4 +66,6 @@ | ||
The `augCompileRevertEl(compiledAugEl)` function takes a compiled element and restores it to the original state. This does not remove the compiled rule that was generated originally. (you could compile one as a template and copy the data-augmented-ui-compiled attribute to other elements to re-use the compiled state) | ||
The `augCompileRevertEl(compiledAugEl)` function takes a compiled element and restores it to the original state. This also removes the compiled rules that were generated originally. (if you compile one as a template and copy the data-augmented-ui-compiled attribute to other elements to re-use the compiled state, be sure not to revert any of them unless you revert all of them!) | ||
`augCompileRevertEl` runs synchronously and returns the number of rules removed. | ||
```js | ||
@@ -81,2 +85,6 @@ augCompileRevertEl(document.querySelector("[data-augmented-ui-compiled]")) | ||
`augCompileAll` and `augCompileEl` run on the next animation frame and return a promise that resolves to the number of rules inserted. To optimize DOM manipulation, all reads are done first, all writes are grouped together and done last. | ||
`augCompileAllSync` and `augCompileElSync` do the same as their counterparts but do not wait for the next animation frame and return the values directly. | ||
The stylesheet generated for this is inserted as the first child of the `<head>` and has a `data-aug-compile-styles` attribute to make it easier to find and query. You can access the styles and manually manage them if you wish: | ||
@@ -88,1 +96,14 @@ | ||
``` | ||
If you're using delegates for your augmented inlay or augmented border, they will be compiled/reverted along with the augmented parent element. No further setup is required to compile/revert delegates. | ||
# Changelog | ||
Version 2.0.0 - 11/09/2020 | ||
* `augCompileAll` and `augCompileEl` run asynchronously on requestAnimationFrame | ||
* `augCompileAll` and `augCompileEl` resolve with the number of rules inserted | ||
* `augCompileAll` and `augCompileEl` group writes for the end of the call so all reads happen first to optimize DOM manipulation | ||
* `augCompileAllSync` and `augCompileElSync` added | ||
* `augCompileRevertEl` removes the rules now | ||
* Augmented delegates now supported automatically | ||
* Multiple (informal) tests added, including animation FPS measurements of uncompiled vs compiled |
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
28695
348
105
1