auth-validify
Advanced tools
Comparing version 1.0.2 to 1.0.3
{ | ||
"name": "auth-validify", | ||
"version": "1.0.2", | ||
"version": "1.0.3", | ||
"type": "commonjs", | ||
@@ -5,0 +5,0 @@ "description": "Your go-to JavaScript package for hassle-free form validation. Simplify error-checking, enhance user experience, and make your forms shine effortlessly.", |
@@ -1,3 +0,85 @@ | ||
# Validity JS | ||
Auth Valdify: Powerful and Customizable Validation for Node.js | ||
<!-- auth-validify, auth-validity, auth-validation --> | ||
[](https://www.npmjs.com/package/auth-validify) | ||
**auth-validify** simplifies and strengthens data validation in your Node.js applications. It provides a comprehensive set of validation functions for common data types, along with options for customization to meet your specific needs. | ||
This library empowers you to: | ||
- Ensure the integrity and security of user-submitted data. | ||
- Prevent invalid inputs from reaching your backend logic. | ||
- Enhance user experience with clear and actionable error messages. | ||
## Installation | ||
```bash | ||
npm install auth-validify | ||
``` | ||
**Usage** | ||
Offer practical examples demonstrating how to use each validation function: | ||
## Usage | ||
**1. Basic Validation:** | ||
```javascript | ||
const { validator } = require('auth-validify'); | ||
const email = 'someone@email.com'; | ||
const password = 'StrongPassword123!'; | ||
if (validator.isEmail(email) && validator.isValidPassword(password)) { | ||
console.log('Valid email and password!'); | ||
} else { | ||
// Provide helpful error messages | ||
if (!validator.isEmail(email)) { | ||
console.error('Invalid email format.'); | ||
} | ||
if (!validator.isValidPassword(password)) { | ||
console.error('Password must be at least 8 characters long and contain a combination of letters, numbers, and special characters.'); | ||
} | ||
} | ||
``` | ||
```javascript | ||
const { validator } = require('auth-validify'); | ||
// Set custom password length (minimum 12 characters) | ||
const options = { minLength: 12 }; | ||
if (validator.isValidPassword(password, options)) { | ||
console.log('Password meets custom criteria!'); | ||
} else { | ||
// ... (error handling) | ||
} | ||
``` | ||
<!-- // (demonstrate validation methods for phone numbers, usernames, URLs, etc.) --> | ||
## Features and Benefits | ||
- **Comprehensive Validation:** Covers common data types like email, password, phone number, username, and URL. | ||
- **Customizable Rules:** Tailor validation criteria to your application's specific needs (e.g., minimum password length, allowed email domains). | ||
- **Clear Error Handling:** Throw informative exceptions or provide custom error messages for better debugging and user experience. | ||
- **Asynchronous Support:** Enables seamless integration with promises or async/await patterns. | ||
- **Well-Documented:** Provides detailed documentation with clear examples and API descriptions. | ||
## Contribution | ||
We welcome contributions to improve `auth-validify`. Feel free to submit pull requests for bug fixes, new features, or improved documentation. | ||
## License | ||
This project is licensed under the MIT License. See the LICENSE file for details. | ||
## Author | ||
- [Bankole Emmanuel](https://github.com/codewithdripzy) (https://buymeacoffee.com/thecodeguyy) | ||
**Buy Me a Coffee** | ||
If you find this library helpful, consider buying me a coffee to support further development: | ||
[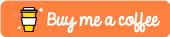](https://www.buymeacoffee.com/thecodeguyy) |
@@ -11,2 +11,6 @@ interface ValidatorType{ | ||
export type { ValidatorType, PasswordType }; | ||
interface ValidatorOptions{ | ||
minLength : number | ||
} | ||
export type { ValidatorType, PasswordType, ValidatorOptions }; |
import * as bcrypt from "bcrypt"; | ||
import { PasswordType, ValidatorType } from "./global/interfaces"; | ||
import { PasswordType, ValidatorOptions, ValidatorType } from "./global/interfaces"; | ||
/** | ||
* @module auth-validify | ||
* @description A comprehensive validation library for Node.js applications. | ||
*/ | ||
/** | ||
* @typedef {Object} ValidatorOptions | ||
* @property {number} [minLength=8] - Minimum password length (default: 8). | ||
* @property {boolean} [requireSpecial=true] - Whether to require at least one special character (default: true). | ||
* @property {string[]} [allowedEmailDomains] - Array of allowed email domain names (optional). | ||
*/ | ||
/** | ||
* @class Validator | ||
*/ | ||
class Validator implements ValidatorType{ | ||
/** | ||
* Validates an email address. | ||
* | ||
* @param {string} email - The email address to validate. | ||
* @param {ValidatorOptions} [options] - Customization options. | ||
* @returns {boolean} True if the email is valid, false otherwise. | ||
* | ||
* @throws {TypeError} If the email is not a string. | ||
* @throws {InvalidEmailError} If the email format is invalid. | ||
*/ | ||
isEmail(email : string) : boolean{ | ||
@@ -15,6 +40,24 @@ const pattern = /([A-Za-z])\w+@((gmail)|(outlook)|(yahoo)|(hotmail)|(icloud)|(me)|(aol)|(protonmail)|(yandex)|(zoho))\.(com)/ | ||
isValidPassword(password : string) : boolean{ | ||
const pattern = /^(?=.*[0-9])(?=.*[!@#$%^&*])[a-zA-Z0-9!@#$%^&*]{8,16}$/ | ||
/** | ||
* Validates a password. | ||
* | ||
* @param {string} password - The password to validate. | ||
* @param {ValidatorOptions} [options] - Customization options. | ||
* @returns {boolean} True if the password is valid, false otherwise. | ||
* | ||
* @throws {TypeError} If the password is not a string. | ||
* @throws {InvalidPasswordError} If the password format is invalid. | ||
*/ | ||
isValidPassword(password : string, options: ValidatorOptions = { minLength: 8 }): boolean { | ||
const { minLength } = options; | ||
const pattern = new RegExp(`^(?=.*[0-9])(?=.*[!@#$%^&*])[a-zA-Z0-9!@#$%^&*]{${minLength},16}$`); | ||
return pattern.test(password); | ||
} | ||
isValidPhoneNumber(phoneNumber : string) : boolean{ | ||
const pattern = /^(\+?[\d{1,3}\s]?-\?)?\(?([0-9]{2,5})\)?[- \.]?([0-9]{3})[- \.]?([0-9]{4})$/ | ||
if(password.match(pattern)){ | ||
if(phoneNumber.match(pattern)){ | ||
return true; | ||
@@ -27,3 +70,14 @@ } | ||
/** | ||
* @class Password | ||
*/ | ||
class Password implements PasswordType { | ||
/** | ||
* Hashes a password securely using bcrypt. | ||
* | ||
* @param {string} password - The password to hash. | ||
* @returns {Promise<string>} A promise that resolves with the hashed password. | ||
* | ||
* @throws {TypeError} If the password is not a string. | ||
*/ | ||
hash(password : string) : string{ | ||
@@ -36,2 +90,11 @@ const salt = bcrypt.genSaltSync(10); | ||
/** | ||
* Verifies a password against a stored hash. | ||
* | ||
* @param {string} password - The password to verify. | ||
* @param {string} hash - The stored password hash. | ||
* @returns {Promise<boolean>} A promise that resolves with true if the password matches the hash, false otherwise. | ||
* | ||
* @throws {TypeError} If either `password` or `hash` is not a string. | ||
*/ | ||
verify(password : string, hash : string) : boolean{ | ||
@@ -38,0 +101,0 @@ const validity = bcrypt.compareSync(password, hash); |
11063
186
86