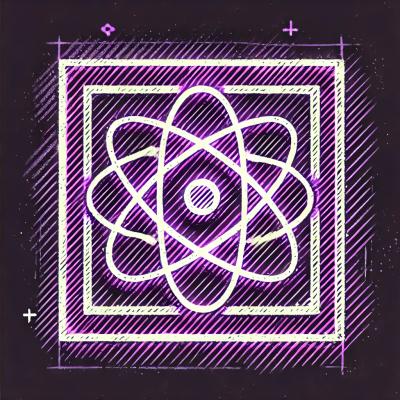
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
auto-encoder.ts
Advanced tools
A simple Auto Encoder typescript library for experimentation and dimensionality reduction. Supports automatic scaling.
A simple Auto Encoder typescript library for experimentation and dimensionality reduction. Supports automatic scaling.
This is a Typescript wrapper on top of autoencoder.
With additional helper functions: exportAutoEncoder(autoEncoder)
and restoreAutoEncoder(json)
.
npm install auto-encoder.ts
You can also install auto-encoder.ts
with pnpm, yarn, or slnpm
There are two ways to initialize a model:
import { createAutoEncoder } from 'auto-encoder.ts'
const model = createAutoEncoder({
nInputs: 10,
nHidden: 2,
nLayers: 2, // (default 2) - number of layers in each encoder/decoder
activation: 'relu', // (default 'relu') - applied to all, but the last layer
})
import { createAutoEncoder } from 'auto-encoder.ts'
const model = createAutoEncoder({
encoder: [
{ nOut: 10, activation: 'tanh' },
{ nOut: 2, activation: 'tanh' },
],
decoder: [{ nOut: 2, activation: 'tanh' }, { nOut: 10 }],
scale: false, // (default true)
})
Activation functions: relu
, tanh
, sigmoid
Similar to other neural networks, auto-encoder is very sensitive to input scaling.
To make it easier the scaling is enabled by default.
you can control it with an extra parameter scale
that can be true
or false
.
model.fit(X, {
batchSize: 100,
iterations: 5000,
method: 'adagrad', // (default 'adagrad')
stepSize: 0.01, // (default 0.05)
})
Optimization methods: sgd
, adagrad
, adam
const Y = model.encode(X)
const Xd = model.decode(Y)
// Similar to model.decode(model.encode(X))
const Xp = model.predict(X)
Try the package in the browser on StatSim Vis. Choose a CSV file, change the Projection method to Autoencoder, then click Run.
Below are the exported function and types:
import { ActivationFunctionName, OptimizationMethodName } from 'adnn.ts'
function createAutoEncoder(options: AutoEncoderOptions): AutoEncoder
function exportAutoEncoder(autoEncoder: AutoEncoder): AutoEncoderJSON
function restoreAutoEncoder(json: AutoEncoderJSON): AutoEncoder
interface AutoEncoder {
fit(X: BatchValues, options?: FitOptions): void
encode(X: BatchValues): BatchValues
decode(X: BatchValues): BatchValues
/** @description Similar to this.decode(this.encode(X)) */
predict(X: BatchValues): BatchValues
}
type AutoEncoderJSON = {
scale: boolean | undefined
max: number[]
min: number[]
nInputs: number
nHidden: number
encoder: unknown
decoder: unknown
}
type FitOptions = {
/** @default round(totalSize/50) */
batchSize?: number
/** @default 100 */
iterations?: number
/** @default 'adagrad' */
method?: OptimizationMethodName
/** @default 0.05 */
stepSize?: number
}
type BatchValues = Values[]
type Values = number[]
type AutoEncoderOptions =
| {
/** @default true */
scale?: boolean
/** @description number of input features */
nInputs: number
/** @description number of embedding features */
nHidden: number
/**
* @description number of layers in each encoder/decoder
* @default 2
*/
nLayers?: number
/**
* @description applied to all, but the last layer
* @default 'relu'
*/
activation?: ActivationFunctionName
}
| {
/** @default true */
scale?: boolean
encoder: LayerOptions[]
decoder: LayerOptions[]
}
type LayerOptions = {
nOut: number
/** @description no activation function in the last layer of decoder gives better result */
activation?: ActivationFunctionName
}
This project is licensed with BSD-2-Clause
This is free, libre, and open-source software. It comes down to four essential freedoms [ref]:
FAQs
A simple Auto Encoder typescript library for experimentation and dimensionality reduction. Supports automatic scaling.
The npm package auto-encoder.ts receives a total of 0 weekly downloads. As such, auto-encoder.ts popularity was classified as not popular.
We found that auto-encoder.ts demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.