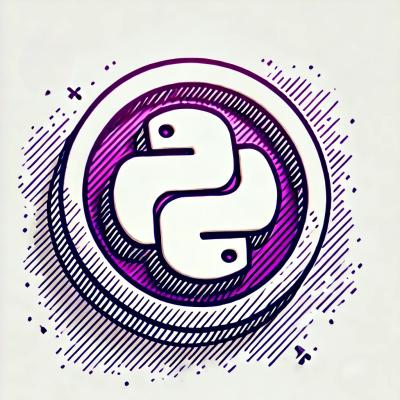
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
autonumeric
Advanced tools
autoNumeric is a library that provides live *as-you-type* formatting for international numbers and currencies. It supports most International numeric formats and currencies including those used in Europe, Asia, and North and South America.
autoNumeric is a standalone Javascript library that provides live as-you-type formatting for international numbers and currencies.
The latest stable branch is 3.*.
For older stable versions, please take a look here, while for the latest development version, check the next
branch.
Moreover, you can take a look at what could be the next features coming to autoNumeric on our project page (feel free to participate!).
autoNumeric main features are :
// Initialization
new AutoNumeric('.myInput', { currencySymbol : '$' });
// The options are...optional :)
const autoNumericOptionsEuro = {
digitGroupSeparator : '.',
decimalCharacter : ',',
decimalCharacterAlternative: '.',
currencySymbol : '\u202f€',
currencySymbolPlacement : AutoNumeric.options.currencySymbolPlacement.suffix,
roundingMethod : 'U',
};
// Initialization
new AutoNumeric(domElement, autoNumericOptionsEuro);
And also:
update
method or via a callbackinput
elements as well as most text elements, allowing you to place formatted numbers and currencies on just about any part of your pageWith that said, autoNumeric supports most international numeric formats and currencies including those used in Europe, Asia, and North and South America.
You can install autoNumeric with your preferred dependency manager:
# with `yarn` :
yarn add autonumeric
# or with `npm` :
npm install autonumeric
Simply include autoNumeric in your html <header>
tag.
No other files or libraries are required ; autoNumeric has no dependency.
<script src="autoNumeric.min.js" type="text/javascript"></script>
<!-- ...or, you may also directly use a CDN :-->
<script src="https://cdn.jsdelivr.net/autonumeric/3.0.0/autoNumeric.min.js"></script>
If you want to use AutoNumeric in your code, you can import the src/autoNumeric.js
file as an ES6 module using:
import AutoNumeric from 'autoNumeric';
Then you can initialize autoNumeric with or without options :
// autoNumeric with the defaults options
anElement = new AutoNumeric(domElement);
// autoNumeric with specific options being passed
anElement = new AutoNumeric(domElement, { options });
// autoNumeric with a css selector and a pre-defined language options
anElement = new AutoNumeric('.myCssClass > input').french();
(See the available language list here)
You're done!
Note : an AutoNumeric object can be initialized in various ways, check those out here
autoNumeric can be used in two ways ;
<input>
elements making them reactive (in a read/write mode), or<input>
elementsWhen used on an <input>
element, you'll be able to interact with its value and get a formatted input value as-you-type, using the full power of autoNumeric.
Only the following supported <input>
types are supported :
text
,tel
,hidden
, or<input type='text' value="1234.56">
<input type='tel' value="1234.56">
<input type='hidden' value="1234.56">
<input value="1234.56">
Note : the number
type is not supported simply because autoNumeric formats numbers as strings (ie. '123.456.789,00 €'
) that this input type does not allow.
You can use autoNumeric to format a DOM element value once on load.
This means it will then not react to any user interaction.
The following elements are accepted :
const allowedTagList = [
'b', 'caption', 'cite', 'code', 'const', 'dd', 'del', 'div', 'dfn', 'dt', 'em', 'h1', 'h2', 'h3',
'h4', 'h5', 'h6', 'ins', 'kdb', 'label', 'li', 'option', 'output', 'p', 'q', 's', 'sample',
'span', 'strong', 'td', 'th', 'u'
]
Multiple options allow you to customize precisely how a form input will format your key strokes as you type :
Option | Description | Default Value |
---|---|---|
allowDecimalPadding | Allow padding the decimal places with zeros | true |
currencySymbol | Currency symbol | '' |
currencySymbolPlacement | Placement of the currency sign, relative to the number (as a prefix or a suffix) | 'p' |
decimalCharacter | Decimal separator character | '.' |
decimalCharacterAlternative | Allow to declare alternative decimal separator which is automatically replaced by the real decimal character (useful in countries where the keyboard numeric pad have a period as the decimal character) | null |
decimalPlacesOverride | Maximum number of decimal places (used to override decimal places set by the minimumValue & maximumValue values) | null |
decimalPlacesShownOnFocus | Expanded decimal places visible when input has focus | null |
defaultValueOverride | Helper option for the ASP.NET-specific postback issue | null |
digitalGroupSpacing | Digital grouping for the thousand separator | '3' |
digitGroupSeparator | Thousand separator character | ',' |
emptyInputBehavior | Define what to display when the input value is empty (possible options are focus , press , always and zero ) | 'focus' |
failOnUnknownOption | This option is the 'strict mode' (aka 'debug' mode), which allows autoNumeric to strictly analyse the options passed, and fails if an unknown options is used in the settings object. | false |
formatOnPageLoad | Determine if the default value will be formatted on initialization | true |
isCancellable | Determine if the user can 'cancel' the last modifications done to the element value when using the Escape key | true |
leadingZero | Controls the leading zero behavior (possible options are allow , deny and keep ) | 'deny' |
maximumValue | Maximum possible value | '9999999999999.99' |
minimumValue | Minimum possible value | '-9999999999999.99' |
modifyValueOnWheel | Determine if the element value can be incremented / decremented with the mouse wheel. The wheel behavior is modified with the wheelStep option. | true |
negativeBracketsTypeOnBlur | Adds brackets [] , parenthesis () , curly braces {} or <> on negative values when unfocused | null |
negativePositiveSignPlacement | Placement of negative/positive sign relative to the currency symbol (possible options are l (left), r (right), p (prefix) and s (suffix)) | null |
noEventListeners | Defines if the element should have event listeners activated on it | false |
noSeparatorOnFocus | Remove the thousand separator, currency symbol and suffix on focus | false |
onInvalidPaste | Manage how autoNumeric react when the user tries to paste an invalid number (possible options are error , ignore , clamp , truncate or replace ) | 'error' |
outputFormat | Defines the localized output format of the get , getString & getArray methods | null |
overrideMinMaxLimits | Override minimum and maximum limits (possible options are ceiling , floor and ignore ) | null |
readOnly | Defines if the <input> element should be set as read only on initialization | false |
roundingMethod | Method used for rounding (possible options are S , A , s , a , B , U , D , C , F , N05 , U05 or D05 ) | 'S' |
saveValueToSessionStorage | Allow the decimalPlacesShownOnFocus value to be saved into session storage | false |
scaleDecimalPlaces | The number of decimal places when unfocused | null |
scaleDivisor | This option decides the onfocus value and places the result in the input on focusout | null |
scaleSymbol | Symbol placed as a suffix when unfocused | null |
serializeSpaces | Defines how the serialize functions should treat spaces when serializing (convert them to '%20' or '+' ) | '+' |
selectNumberOnly | Determine if the select all keyboard command will select the complete input text, or only the input numeric value | false |
showPositiveSign | Allow the positive sign symbol + to be displayed for positive numbers | false |
showWarnings | Defines if warnings should be shown | true |
suffixText | Additional text suffix that is added after the number | '' |
unformatOnSubmit | Removes formatting on submit event | false |
wheelStep | Used in conjonction with the modifyValueOnWheel option, this allow to either define a fixed step (ie. 1000 ), or a progressive one | 'progressive' |
Using the noEventListeners
option allow autoNumeric to only format without adding any event listeners to an input, or any other DOM elements (that the function would accept as a parameter). This would be useful for read-only values for instance.
// Initialize without setting up any event listeners
anElement = new AutoNumeric(domElement, 12345.789, { options }).remove(); // This is the default existing way of doing that...
// ...but you can also directly pass a special option `noEventListeners` to prevent the initial creation of those event listeners
anElement = new AutoNumeric(domElement, 12345.789, { noEventListeners: true });
In the latter case, it initialize the AutoNumeric element, except it does not add any event listeners. Which means it format the value only once and then let the user modify it freely.
Note: The value can then be formatted via a call to set
.
AutoNumeric can initialize an <input>
element with the readonly
property by setting the readOnly
option to true
in the settings:
anElement = new AutoNumeric(domElement, 12345.789, { readOnly: true });
For more detail on how to use each options, please take a look at the detailed comments in the source code for the defaultSettings
object.
Sometime you do not want to have to configure every single aspect of your format, specially if it's a common one.
Hence, we provide multiple default options for the most common currencies.
You can use those pre-defined language option like so :
new AutoNumeric('.mySelector > input').french();
Currently, the predefined options are :
Option name | |
---|---|
:fr: | French |
:es: | Spanish |
:us: | NorthAmerican |
:uk: | British |
🇨🇭 | Swiss |
:jp: | Japanese |
:cn: | Chinese |
If you feel a common currency option is missing, please create a pull request and we'll add it!
An AutoNumeric object can be initialized in various ways.
It always takes either a DOM element reference as its first argument, or a css string selector.
Note: only one element can be selected this way, since under the hood document.querySelector
is called, and this only return one element.
If you need to be able to select and initialize multiple elements in one call, then consider using the static AutoNumeric.multiple()
function
anElement = new AutoNumeric(domElement); // With the default options
anElement = new AutoNumeric(domElement, { options }); // With one option object
anElement = new AutoNumeric(domElement).french(); // With one pre-defined language object
anElement = new AutoNumeric(domElement).french({ options });// With one pre-defined language object and additional options that will override those defaults
// ...or init and set the value in one call :
anElement = new AutoNumeric(domElement, 12345.789); // With the default options, and an initial value
anElement = new AutoNumeric(domElement, 12345.789, { options });
anElement = new AutoNumeric(domElement, '12345.789', { options });
anElement = new AutoNumeric(domElement, null, { options }); // With a null initial value
anElement = new AutoNumeric(domElement, 12345.789).french({ options });
anElement = new AutoNumeric(domElement, 12345.789, { options }).french({ options }); // Not really helpful, but possible
// The AutoNumeric constructor class can also accept a string as a css selector. Under the hood this use `QuerySelector` and limit itself to only the first element it finds.
anElement = new AutoNumeric('.myCssClass > input');
anElement = new AutoNumeric('.myCssClass > input', { options });
anElement = new AutoNumeric('.myCssClass > input', 12345.789);
anElement = new AutoNumeric('.myCssClass > input', 12345.789, { options });
anElement = new AutoNumeric('.myCssClass > input', null, { options }); // With a null initial value
anElement = new AutoNumeric('.myCssClass > input', 12345.789).french({ options });
Note: AutoNumeric also accepts a limited tag list that it will format on page load, but without adding any event listeners
If you know you want to initialize multiple elements in one call, you must then use the static AutoNumeric.multiple()
function:
// Init multiple DOM elements in one call (and possibly pass multiple values that will be mapped to each DOM element)
[anElement1, anElement2, anElement3] = AutoNumeric.multiple([domElement1, domElement2, domElement3], { options });
[anElement1, anElement2, anElement3] = AutoNumeric.multiple([domElement1, domElement2, domElement3], 12345.789, { options });
[anElement1, anElement2, anElement3] = AutoNumeric.multiple.french([domElement1, domElement2, domElement3], [12345.789, 234.78, null], { options });
// Special case, if a <form> element is passed (or any other 'parent' (or 'root') DOM element), then autoNumeric will initialize each child `<input>` elements recursively, ignoring those referenced in the `exclude` attribute
[anElement1, anElement2] = AutoNumeric.multiple({ rootElement: formElement }, { options });
[anElement1, anElement2] = AutoNumeric.multiple({ rootElement: formElement, exclude : [hiddenElement, tokenElement] }, { options });
[anElement1, anElement2] = AutoNumeric.multiple({ rootElement: formElement, exclude : [hiddenElement, tokenElement] }, [12345.789, null], { options });
// If you want to select multiple elements via a css selector, then you must use the `multiple` function. Under the hood `QuerySelectorAll` is used.
[anElement1, anElement2] = AutoNumeric.multiple('.myCssClass > input', { options }); // This always return an Array, even if there is only one element selected
[anElement1, anElement2] = AutoNumeric.multiple('.myCssClass > input', [null, 12345.789], { options }); // Idem above, but with passing the initial values too
Options can be added and/or modified after the initialization has been done.
Either by passing an options object that contains multiple options...
anElement.update({ moreOptions });
anElement.update(AutoNumeric.getLanguages().NorthAmerican); // Update the settings (and immediately reformat the element accordingly)
...or by changing the options one by one (or by calling a pre-defined option object)
anElement.options.minimumValue('12343567.89');
anElement.options.allowDecimalPadding(false);
Lastly, the option object can be accessed directly, thus allowing to query each options globally too
anElement.getSettings(); // Return the options object containing all the current autoNumeric settings in effect
autoNumeric provides numerous methods to access and modify the element value, formatted or unformatted, at any point in time.
It does so by providing access to those methods via the AutoNumeric object class (declared as an ES6 Module).
First. you need to get a reference to the AutoNumeric module that you need to import:
import AutoNumeric from 'autoNumeric.min';
Then you'll be able to access either the methods on the instantiated AutoNumeric object, or the static functions directly by using the AutoNumeric
class.
Method | Description | Call example |
---|---|---|
set | Set the value (that will be formatted immediately) | anElement.set(42.76); |
set | Set the value and update the setting in one go | anElement.set(42.76, { options }); |
setUnformatted | Set the value (that will not be formatted immediately) | anElement.setUnformatted(42.76); |
setUnformatted | Set the value and update the setting in one go (the value will not be formatted immediately) | anElement.setUnformatted(42.76, { options }); |
getNumericString | Return the unformatted number as a string | anElement.getNumericString(); |
get | Alias for the .getNumericString() method | anElement.get(); |
getFormatted | Return the formatted string | anElement.getFormatted(); |
getNumber | Return the unformatted number as a number | anElement.getNumber(); |
getLocalized | Return the localized unformatted number as a string | anElement.getLocalized(); |
getLocalized | Return the localized unformatted number as a string, using the outputFormat option override passed as a parameter | anElement.getLocalized(forcedOutputFormat); |
reformat | Force the element to reformat its value again (in case the formatting has been lost) | anElement.reformat(); |
unformat | Remove the formatting and keep only the raw unformatted value in the element (as a numeric string) | anElement.unformat(); |
unformatLocalized | Remove the formatting and keep only the localized unformatted value in the element | anElement.unformatLocalized(); |
unformatLocalized | Idem above, but using the outputFormat option override passed as a parameter | anElement.unformatLocalized(forcedOutputFormat); |
isPristine | Return true if the current value is the same as when the element got initialized | anElement.isPristine(); |
select | Select the formatted element content, based on the selectNumberOnly option | anElement.select(); |
selectNumber | Select only the numbers in the formatted element content, leaving out the currency symbol, whatever the value of the selectNumberOnly option | anElement.selectNumber(); |
selectInteger | Select only the integer part in the formatted element content, whatever the value of selectNumberOnly | anElement.selectInteger(); |
selectDecimal | Select only the decimal part in the formatted element content, whatever the value of selectNumberOnly | anElement.selectDecimal(); |
clear | Reset the element value to the empty string '' (or the currency sign, depending on the emptyInputBehavior option value) | anElement.clear(); |
clear | Reset the element value to the empty string '' as above, no matter the emptyInputBehavior option value | anElement.clear(true); |
Method | Description | Call example |
---|---|---|
remove | Remove the autoNumeric listeners from the element (previous name : 'destroy'). Keep the element content intact. | anElement.remove(); |
wipe | Remove the autoNumeric listeners from the element, and reset its value to '' | anElement.wipe(); |
nuke | Remove the autoNumeric listeners from the element, and delete the DOM element altogether | anElement.nuke(); |
Method | Description | Call example |
---|---|---|
node | Return the DOM element reference of the autoNumeric-managed element | anElement.node(); |
parent | Return the DOM element reference of the parent node of the autoNumeric-managed element | anElement.parent(); |
detach | Detach the current AutoNumeric element from the shared 'init' list (which means any changes made on that local shared list will not be transmitted to that element anymore) | anElement.detach(); |
detach | Idem above, but detach the given AutoNumeric element, not the current one | anElement.detach(otherAnElement); |
attach | Attach the given AutoNumeric element to the shared local 'init' list. When doing that, by default the DOM content is left untouched. The user can force a reformat with the new shared list options by passing a second argument to true . | anElement.attach(otherAnElement, reFormat = true); |
This allows to format or unformat numbers, strings or directly other DOM elements without having to specify the options each time, since the current AutoNumeric object already has those settings set.
Method | Description | Call example |
---|---|---|
formatOther | This use the same function signature that when using the static AutoNumeric method directly (cf. below: AutoNumeric.format ), but without having to pass the options | anElement.formatOther(12345, { options }); |
formatOther | Idem above, but apply the formatting to the DOM element content directly | anElement.formatOther(domElement5, { options }); |
unformatOther | This use the same function signature that when using the static AutoNumeric method directly (cf. below: AutoNumeric.unformat ), but without having to pass the options | anElement.unformatOther('1.234,56 €', { options }); |
unformatOther | Idem above, but apply the unformatting to the DOM element content directly | anElement.unformatOther(domElement5, { options }); |
Once you have an AutoNumeric element already setup correctly with the right options, you can use it as many times you want to initialize as many other DOM elements as needed (this works only on elements that can be managed by autoNumeric).
Whenever init
is used to initialize other DOM element, a shared 'local' list of those elements is stored in the AutoNumeric objects.
This allows for neat things like modifying all those linked AutoNumeric elements globally, with one call.
Method | Description | Call example |
---|---|---|
init | Use an existing AutoNumeric element to initialize another DOM element with the same options | const anElement2 = anElement.init(domElement2); |
init | If true is set as the second argument, then the newly generated AutoNumeric element will not share the same local element list as anElement | const anElement2 = anElement.init(domElement2, true); |
This local list can be used to perform global operations on all those AutoNumeric elements, with one function call.
To do so, you must call the wanted function by prefixing .global
before the method name (ie. anElement.global.set(42)
).
Below are listed all the supported methods than can be called globally:
anElement.global.set(2000); // Set the value 2000 in all the autoNumeric-managed elements that are shared on this element
anElement.global.setUnformatted(69);
[result1, result2, result3] = anElement.global.get(); // Return an array of results
[result1, result2, result3] = anElement.global.getNumericString(); // Return an array of results
[result1, result2, result3] = anElement.global.getFormatted(); // Return an array of results
[result1, result2, result3] = anElement.global.getNumber(); // Return an array of results
[result1, result2, result3] = anElement.global.getLocalized(); // Return an array of results
anElement.global.reformat();
anElement.global.unformat();
anElement.global.unformatLocalized();
anElement.global.unformatLocalized(forcedOutputFormat);
anElement.global.isPristine(); // Return `true` is *all* the autoNumeric-managed elements are pristine, if their raw value hasn't changed
anElement.global.isPristine(false); // Idem as above, but also checks that the formatted value hasn't changed
anElement.global.clear(); // Clear the value in all the autoNumeric-managed elements that are shared on this element
anElement.global.remove();
anElement.global.wipe();
The shared local list also provide list-specific methods to manipulate it:
anElement.global.has(domElementOrAutoNumericObject); // Return `true` if the given AutoNumeric object (or DOM element) is in the local AutoNumeric element list
anElement.global.addObject(domElementOrAutoNumericObject); // Add an existing AutoNumeric object (or DOM element) to the local AutoNumeric element list, using the DOM element as the key
anElement.global.removeObject(domElementOrAutoNumericObject); // Remove the given AutoNumeric object (or DOM element) from the local AutoNumeric element list, using the DOM element as the key
anElement.global.empty(); // Remove all elements from the shared list, effectively emptying it
[anElement0, anElement1, anElement2, anElement3] = anElement.global.elements(); // Return an array containing all the AutoNumeric elements that have been initialized by each other
anElement.global.getList(); // Return the `Map` object directly
anElement.global.size(); // Return the number of elements in the local AutoNumeric element list
autoNumeric elements provide special functions to manipulate the form they are a part of.
Those special functions really work on the parent <form>
element, instead of the <input>
element itself.
Method | Description | Call example |
---|---|---|
form | Return a reference to the parent element, null if it does not exist | anElement.form(); |
formNumericString | Return a string in standard URL-encoded notation with the form input values being unformatted | anElement.formNumericString(); |
formFormatted | Return a string in standard URL-encoded notation with the form input values being formatted | anElement.formFormatted(); |
formLocalized | Return a string in standard URL-encoded notation with the form input values, with localized values | anElement.formLocalized(); |
formLocalized(forcedOutputFormat) | Idem above, but with the possibility of overriding the outputFormat option | anElement.formLocalized(forcedOutputFormat); |
formArrayNumericString | Return an array containing an object for each form <input> element, with the values as numeric strings | anElement.formArrayNumericString(); |
formArrayFormatted | Return an array containing an object for each form <input> element, with the values as formatted strings | anElement.formArrayFormatted(); |
formArrayLocalized | Return an array containing an object for each form <input> element, with the values as localized numeric strings | anElement.formArrayLocalized(); |
formArrayLocalized(forcedOutputFormat) | Idem above, but with the possibility of overriding the outputFormat option | anElement.formArrayLocalized(forcedOutputFormat); |
formJsonNumericString | Return a JSON string containing an object representing the form input values. This is based on the result of the formArrayNumericString() function. | anElement.formJsonNumericString(); |
formJsonFormatted | Return a JSON string containing an object representing the form input values. This is based on the result of the formArrayFormatted() function. | anElement.formJsonFormatted(); |
formJsonLocalized | Return a JSON string containing an object representing the form input values. This is based on the result of the formArrayLocalized() function. | anElement.formJsonLocalized(); |
formJsonLocalized(forcedOutputFormat) | Idem above, but with the possibility of overriding the outputFormat option | anElement.formJsonLocalized(forcedOutputFormat); |
formUnformat | Unformat all the autoNumeric-managed elements that are a child to the parent element of this anElement input, to numeric strings | anElement.formUnformat(); |
formUnformatLocalized | Unformat all the autoNumeric-managed elements that are a child to the parent element of this anElement input, to localized strings | anElement.formUnformatLocalized(); |
formReformat | Reformat all the autoNumeric-managed elements that are a child to the parent element of this anElement input | anElement.formReformat(); |
The following functions can either take a callback, or not. If they don't, the default form.submit()
function will be called.
Method | Description | Call example |
---|---|---|
formSubmitNumericString(callback) | Run the callback(value) with value being equal to the result of formNumericString() | anElement.formSubmitNumericString(callback); |
formSubmitFormatted(callback) | Run the callback(value) with value being equal to the result of formFormatted() | anElement.formSubmitFormatted(callback); |
formSubmitLocalized(callback) | Run the callback(value) with value being equal to the result of formLocalized() | anElement.formSubmitLocalized(callback); |
formSubmitLocalized(forcedOutputFormat, callback) | Idem above, but with the possibility of overriding the outputFormat option | anElement.formSubmitLocalized(forcedOutputFormat, callback); |
For the following methods, the callback is mandatory:
Method | Description | Call example |
---|---|---|
formSubmitArrayNumericString(callback) | Run the callback(value) with value being equal to the result of formArrayNumericString() | anElement.formSubmitArrayNumericString(callback); |
formSubmitArrayFormatted(callback) | Run the callback(value) with value being equal to the result of formArrayFormatted() | anElement.formSubmitArrayFormatted(callback); |
formSubmitArrayLocalized(callback, forcedOutputFormat) | Idem above, but with the possibility of overriding the outputFormat option | anElement.formSubmitArrayLocalized(callback, forcedOutputFormat); |
formSubmitJsonNumericString(callback) | Run the callback(value) with value being equal to the result of formJsonNumericString() | anElement.formSubmitJsonNumericString(callback); |
formSubmitJsonFormatted(callback) | Run the callback(value) with value being equal to the result of formJsonFormatted() | anElement.formSubmitJsonFormatted(callback); |
formSubmitJsonLocalized(callback, forcedOutputFormat) | Idem above, but with the possibility of overriding the outputFormat option | anElement.formSubmitJsonLocalized(callback, forcedOutputFormat); |
Most of those functions can be chained which allow to be less verbose and more concise.
anElement.french()
.set(42)
.update({ options })
.formSubmitJsonNumericString(callback)
.clear();
Without having to initialize any AutoNumeric object, you can directly use the static AutoNumeric
class functions.
Method | Description | Call example |
---|---|---|
validate | Check if the given option object is valid, and that each option is valid as well. This throws an error if it's not. | AutoNumeric.validate({ options }) |
areSettingsValid | Return true in the settings are valid | AutoNumeric.areSettingsValid({ options }) |
getDefaultConfig | Return the default autoNumeric settings | AutoNumeric.getDefaultConfig() |
getLanguages | Return all the predefined language options in one object | AutoNumeric.getLanguages() |
getLanguages | Return a specific pre-defined language options object | AutoNumeric.getLanguages().French |
format | Format the given number with the given options. This returns the formatted value as a string. | AutoNumeric.format(12345.21, { options }); |
format | Idem above, but using a numeric string as the first parameter | AutoNumeric.format('12345.21', { options }); |
format | Format the domElement value (or textContent ) with the given options and returns the formatted value as a string. This does not update that element value. | AutoNumeric.format(domElement, { options }); |
formatAndSet | Format the domElement value with the given options and returns the formatted value as a string. This function does update that element value with the newly formatted value in the process. | AutoNumeric.formatAndSet(domElement, { options }); |
unformat | Unformat the given formatted string with the given options. This returns a numeric string. | AutoNumeric.unformat('1.234,56 €', { options }); |
unformat | Unformat the domElement value with the given options and returns the unformatted numeric string. This does not update that element value. | AutoNumeric.unformat(domElement, { options }); |
unformatAndSet | Unformat the domElement value with the given options and returns the unformatted value as a numeric string. This function does update that element value with the newly unformatted value in the process. | AutoNumeric.unformatAndSet(domElement, { options }); |
unformatAndSet | Recursively unformat all the autoNumeric-managed elements that are a child to the referenceToTheDomElement element given as a parameter (this is usually the parent <form> element) | AutoNumeric.unformatAndSet(referenceToTheDomElement); |
reformatAndSet | Recursively format all the autoNumeric-managed elements that are a child to the referenceToTheDomElement element given as a parameter (this is usually the parent <form> element), with the settings of each AutoNumeric elements. | AutoNumeric.reformatAndSet(referenceToTheDomElement); |
localize | Unformat and localize the given formatted string with the given options. This returns a string. | AutoNumeric.localize('1.234,56 €', { options }); |
localize | Idem as above, but return the localized DOM element value. This does not update that element value. | AutoNumeric.localize(domElement, { options }); |
localizeAndSet | Unformat and localize the domElement value with the given options and returns the localized value as a string. This function does update that element value with the newly localized value in the process. | AutoNumeric.localizeAndSet(domElement, { options }); |
test | Test if the given domElement is already managed by AutoNumeric (if it is initialized) | AutoNumeric.test(domElement); |
version | Return the AutoNumeric version number (for debugging purpose) | AutoNumeric.version(); |
For questions and support please use the Gitter chat room or IRC on Freenode #autoNumeric.
The issue list of this repository is exclusively for bug reports and feature requests.
Contributors and pull requests are welcome.
Feel free to contact us for any questions.
git clone -b next https://github.com/BobKnothe/autoNumeric.git
# or the following if you are authentified on github :
# `git clone -b next git@github.com:BobKnothe/autoNumeric.git`
cd autoNumeric
First things first, in order to be able to compile the ES6 source to something that can be interpreted by the browsers, and get the tools (linter, test runners, etc.) used by the developers, you need to install them by doing :
yarn install
Note: you need to have yarn
installed before executing this command.
You can install yarn
globally by doing npm install -g yarn
as root.
Once you made your changes, you can build the library with :
yarn build
This will generate the autoNumeric.js
and autoNumeric.min.js
files in the dist
folder, that you'll then be able to use in the browsers.
If you want to clean the generated .js
and .min.js
files as well as development specific ones like coverage and log files, use :
yarn run clean
Note: do not use yarn clean
as it's a different command entirely.
We strive to keep the tests green at all times. Hence whenever you change the source, be sure to :
eslint
does not return any errors regarding the coding style.Tests must always be green :white_check_mark: before pushing. Any commit that make the tests fails will be ignored.
To run the tests, you have multiple options :
# Run unit testing as well as end-to-end testing
yarn test
# Run unit testing only
yarn test:unit
# Run end-to-end testing only
yarn test:e2e
# Run unit testing only...
yarn test:unitp # ...with PhantomJS only
yarn test:unitf # ...with Firefox only
yarn test:unitc # ...with Chrome only
Behind the scene, all unit and end-to-end tests are written with Jasmine.
Karma is used to run the unit tests, while Webdriver.io is used to run end-to-end tests.
Linting allow us to keep a coherent code style in all the source files.
In order to check that everything is well formatted, run eslint with :
yarn lint
If any errors are shown, you can try to automatically correct them by running :
# Use the path of the faulty file there :
./node_modules/eslint/bin/eslint.js --fix src/autoNumeric.js
Every changes that you pushed in its own branch in your personal autoNumeric copy should be based on the latest version of the next
branch.
When you create a pull request, make sure to push against the next
branch.
Your commit must not contain any generated files (ie. files in the /dist/
directory or logs).
Note: Generated dist
files (ie. autoNumeric.js
and autoNumeric.min.js
) are built and force-added to the git repository only once for each official release on master
.
Currently, autoNumeric depends on jQuery (which is pretty logical since it's a jQuery plugin ;P).
Some work is in progress to provide a jQuery-free version of autoNumeric.
The previous stable autoNumeric version v1.9.46 can be found here, and the v2 branch can be found here.
For integration into Rails projects, you can use the autonumeric-rails project.
A more detailed documentation can be found in the Documentation file.
For more examples and an option code generator (that may be outdated), take a look here.
autoNumeric is an MIT-licensed open source project, and its authors are credited in AUTHORS.md.
Feel free to donate via Paypal or Patreon to support autoNumeric development.
3.0.0-beta.3
src/main.js
for bundling the library.autoNumeric.js
, which contains the AutoNumeric class,AutoNumericEnum.js
which contains the enumerations used by AutoNumeric, andAutoNumericHelper.js
which contains the AutoNumericHelper class which provides static helper functions.allowedTagList
, keyCode
and keyName
into AutoNumericEnum
.isEmptyString
, isNumberOrArabic
, isFunction
, isElement
, isInputElement
, arabicToLatinNumbers
, triggerEvent
, randomString
, getElementValue
, setElementValue
, cloneObject
, camelize
, text
, setText
and filterOut
functions to the helper functions.preparePastedText
, runCallbacksFoundInTheSettingsObject
, maximumVMinAndVMaxDecimalLength
, stripAllNonNumberCharacters
, toggleNegativeBracket
, convertToNumericString
, toLocale
, modifyNegativeSignAndDecimalCharacterForRawValue
, modifyNegativeSignAndDecimalCharacterForFormattedValue
, checkEmpty
, addGroupSeparators
, truncateZeros
, roundValue
, truncateDecimal
, checkIfInRangeWithOverrideOption
functions into the AutoNumeric object.character()
method to take into account the quirks of some obsolete browsers.getCurrentElement()
function since we now only need to access the this.domElement
property.AutoNumericHolder
class and the getAutoNumericHolder()
function since we are now using the AutoNumeric class as the 'property holder'._setArgumentsValues()
method).serializeSpaces
option that allows the user to defines how the serialize function will managed the spaces, either by converting them to '%20'
, or to the '+'
string, the latter now being the default.noEventListeners
option that allows the user to initialize an AutoNumeric <input>
element without adding any AutoNumeric event listeners.readOnly
option to the settings that allow the <input>
element to be set to read-only on initialization..global.*
functions).isPristine()
method to test if an AutoNumeric-managed element value
/textContent
has been changed since its initialization.unset
to unformat
.reSet
to reformat
.unformatLocalized()
function to unformat the element value while using the outputFormat
setting.clear()
method to empty the element value.nuke()
method to remove the DOM element from the DOM tree..global.has()
method to check if the given AutoNumeric object (or DOM element) is in the local AutoNumeric element list..global.addObject()
method that adds an existing AutoNumeric object (or DOM element) to the local AutoNumeric element list..global.removeObject()
method that removes the given AutoNumeric object (or DOM element) from the local AutoNumeric element list..global.empty()
method to remove all elements from the shared list..global.elements()
method to retrieve all the AutoNumeric object that share the same local list..global.getList()
method to retrieve the local AutoNumeric element list.version()
method to output the current AutoNumeric version (for debug purpose).set()
method so that the rawValue
is updated when the value is set to ''
.setUnformatted()
method to set the value given value directly as the DOM element value, without formatting it beforehand.get()
method to the renamed getNumericString()
which bares more meaning.getFormatted()
method to retrieve the current formatted value of the AutoNumeric element as a string.getNumber()
method that returns the element unformatted value as a real Javascript number.getLocalized()
method that returns the unformatted value, but following the outputFormat
setting.unformatLocalized()
method that unformats the element value by removing the formatting and keeping only the localized unformatted value in the element.selectNumber()
method that select only the numbers in the formatted element content, leaving out the currency symbol, whatever the value of the selectNumberOnly
option.selectInteger()
method that select only the integer part in the formatted element content, whatever the value of the selectNumberOnly
option.selectDecimal()
method that select only the decimal part in the formatted element content, whatever the value of selectNumberOnly
.node()
method that returns the DOM element reference of the autoNumeric-managed element.parent()
method that returns the DOM element reference of the parent node of the autoNumeric-managed element.detach()
method that detach the current AutoNumeric element from the shared local 'init' list.attach()
method that attach the given AutoNumeric element to the shared local 'init' list.formatOther()
method that format and return the given value, or set the formatted value into the given DOM element if one is passed as an argument.unformatOther()
method that unformat and return the raw numeric string corresponding to the given value, or directly set the unformatted value into the given DOM element if one is passed as an argument.init()
method that allows to use the current AutoNumeric element settings to initialize the DOM element given as a parameter. This effectively link the two AutoNumeric element by making them share the same local AutoNumeric element list.form()
method that return a reference to the parent <form> element if it exists, otherwise return null
.formNumericString()
method that returns a string in standard URL-encoded notation with the form input values being unformatted.formFormatted()
method that returns a string in standard URL-encoded notation with the form input values being formatted.formLocalized()
method that returns a string in standard URL-encoded notation with the form input values, with localized values.formArrayNumericString()
method that returns an array containing an object for each form <input>
element.formArrayFormatted()
method that returns an array containing an object for each form <input>
element, with the value formatted.formArrayLocalized()
method that returns an array containing an object for each form <input>
element, with the value localized.formJsonNumericString()
method that returns a JSON string containing an object representing the form input values.formJsonFormatted()
method that returns a JSON string containing an object representing the form input values, with the value formatted.formJsonLocalized()
method that returns a JSON string containing an object representing the form input values, with the value localized.formUnformat()
method that unformat all the autoNumeric-managed elements that are a child of the parent <form> element of this DOM element, to numeric strings.formUnformatLocalized()
method that unformat all the autoNumeric-managed elements that are a child of the parent <form> element of this DOM element, to localized strings.formReformat()
method that reformat all the autoNumeric-managed elements that are a child of the parent <form> element of this DOM element.formSubmitNumericString()
method that convert the input values to numeric strings, submit the form, then reformat those back.formSubmitFormatted()
method that submit the form with the current formatted values.formSubmitLocalized()
method that convert the input values to localized strings, submit the form, then reformat those back.formSubmitArrayNumericString()
method that generate an array of numeric strings from the <input>
elements, and pass it to the given callback.formSubmitArrayFormatted()
method that generate an array of the current formatted values from the <input>
elements, and pass it to the given callback.formSubmitArrayLocalized()
method that generate an array of localized strings from the <input>
elements, and pass it to the given callback.formSubmitJsonNumericString()
method that generate a JSON string with the numeric strings values from the <input>
elements, and pass it to the given callback.formSubmitJsonFormatted()
method that generate a JSON string with the current formatted values from the <input>
elements, and pass it to the given callback.formSubmitJsonLocalized()
method that generate a JSON string with the localized strings values from the <input>
elements, and pass it to the given callback.test()
method that if the given domElement is already managed by AutoNumeric (if it has been initialized on the current page)._mergeSettings()
and _cloneAndMergeSettings()
to do what they are named about.format()
method so that it formats the given number (or numeric string) with the given options, and returns the formatted value as a string.formatAndSet()
method that format the given DOM element value, and set the resulting value back as the element value.unformat()
method so that it unformats the given formatted string with the given options, and returns a numeric string.unformatAndSet()
method that unformat the given DOM element value, and set the resulting value back as the element value.localize()
method that unformat and localize the given formatted string with the given options, and returns a numeric string.isManagedByAutoNumeric()
method that returns true
is the given DOM element has an AutoNumeric object that manages it.getAutoNumericElement()
method that returns the AutoNumeric object that manages the given DOM element.french()
, northAmerican()
, british()
, swiss()
, japanese()
, spanish()
and chinese()
methods that update the settings to use the named pre-defined language options.setReal
from some functions.this.settings
directly).isNan()
on non-number elements like strings.this.isFocused
variable.getElementValue()
function to retrieve the element value
or textContent
(depending if the element in an <input>
or another tag), which allow AutoNumeric to perform some operations on non-input elements too. This is the first changes needed for the goal of managing the non-input tags with contentEditable
with AutoNumeric.getElementValue()
function as well in order to be able to set the value
or textContent
transparently where needed._updateAutoNumericHolderProperties()
to _updateInternalProperties()
._checkElement()
method). This simplify the process by calling the new _isElementTagSupported()
, _isInputElement()
and _isInputTypeSupported()
functions which respect the separation of concerns._formatDefaultValueOnPageLoad()
method now accepts a 'forced' initial value instead of the default one.set()
methods, since those cannot change once AutoNumeric has been initialized, simplifying the code.settings.formatOnPageLoad
inside the formatDefaultValueOnPageLoad()
function that is only called if settings.formatOnPageLoad
is already set).getInitialSettings()
method to _setSettings()
._getSignPosition()
function._serialize()
function that take care of serializing the form data into multiple output as needed, which is called by the _serializeNumericString()
, _serializeFormatted()
,_serializeLocalized()
, _serializeNumericStringArray()
, _serializeFormattedArray()
and _serializeLocalizedArray()
methods.AutoNumeric.defaultSettings
.AutoNumeric.options
object that gives access to all the possible options values, with a semantic name (handy for IDE autocompletion).AutoNumeric.languageOptions
object.AutoNumeric.multiple()
function that allows to initialize numerous AutoNumeric object (on numerous DOM elements) in one call (and possibly pass multiple values that will be mapped to each DOM element).<input>
tags.noEventListeners
or readOnly
options.serializeSpaces
, noEventListeners
and readOnly
options.rawValue
was correctly set when using getSettings()
..global.*
methods.AutoNumeric.multiple()
methods.selectDecimal
, selectInteger
, reformat
, unformat
and unformatLocalized
methods..form*
methods.babel-plugin-transform-object-assign
dev dependency in order to be able to use Object.assign()
in the ES6 source.FAQs
autoNumeric is a standalone Javascript library that provides live *as-you-type* formatting for international numbers and currencies. It supports most international numeric formats and currencies including those used in Europe, Asia, and North and South Am
The npm package autonumeric receives a total of 27,150 weekly downloads. As such, autonumeric popularity was classified as popular.
We found that autonumeric demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.