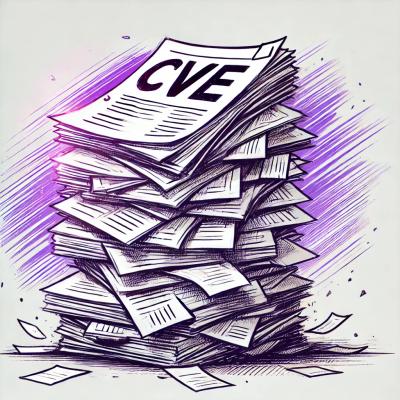
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
The avvio npm package is designed to handle the asynchronous bootstrapping of applications, particularly those built with Node.js. It allows developers to define a series of asynchronous (or synchronous) tasks that need to be executed in a specific order during the startup phase of an application. This can include initializing databases, setting up dependencies, or any other setup tasks that need to be completed before the application starts serving requests. Avvio ensures that each task is completed before moving on to the next, and it provides a clean API for managing this process.
Plugin System
Avvio allows you to structure your application initialization in a modular way using plugins. Each plugin can be an asynchronous function that performs some initialization tasks. The `use` method is used to register these plugins, and Avvio ensures they are executed in the order they are registered.
const avvio = require('avvio')(app);
avvio.use(function (instance, opts, done) {
// async initialization task
done();
});
Async/Await Support
Avvio supports modern JavaScript async/await syntax out of the box. This allows for cleaner and more readable asynchronous code within your initialization tasks.
const avvio = require('avvio')(app);
avvio.use(async function (instance, opts) {
// async task using await
});
Encapsulation and Context
Avvio provides a mechanism for encapsulation and context sharing across plugins. Using the `decorate` method, you can add properties or methods to the application instance that can be accessed by subsequent plugins, facilitating a shared context.
const avvio = require('avvio')(app);
avvio.use(function (instance, opts, done) {
instance.decorate('utility', () => {});
done();
});
Fastify is a web framework for Node.js that includes a powerful plugin architecture, somewhat similar to Avvio. While Fastify is more focused on building web applications and APIs, it uses an underlying plugin system inspired by Avvio for managing the application lifecycle.
Pino is a very fast JSON logger for Node.js, and while it doesn't offer plugin-based application bootstrapping like Avvio, it can be integrated into applications using Avvio for structured and efficient logging throughout the application lifecycle.
Seneca is a microservices toolkit for Node.js that allows you to write microservices easily and quickly. It offers a plugin system that can be seen as similar to Avvio's, focusing on the modular construction of applications, though it is more oriented towards microservice architecture.
Asynchronous bootstrapping is hard, different things can go wrong, error handling and load order just to name a few. The aim of this module is to made it simple.
avvio
is fully reentrant and graph-based. You can load
components/plugins within plugins, and be still sure that things will
happen in the right order. At the end of the loading, your application will start.
To install avvio
, simply use npm:
npm install avvio --save
The example below can be found here and ran using node example.js
.
It demonstrates how to use avvio
to load functions / plugins in order.
'use strict'
const app = require('avvio')()
app
.use(first, { hello: 'world' })
.after((err, cb) => {
console.log('after first and second')
cb()
})
app.use(third)
app.ready(function () {
console.log('application booted!')
})
function first (instance, opts, cb) {
console.log('first loaded', opts)
instance.use(second)
cb()
}
function second (instance, opts, cb) {
console.log('second loaded')
process.nextTick(cb)
}
// async/await or Promise support
async function third (instance, opts) {
console.log('third loaded')
}
avvio()
instance.use()
instance.after()
instance.ready()
instance.override()
instance.onClose()
instance.close()
avvio.express()
Starts the avvio sequence.
As the name suggest, instance
is the object representing your application.
Avvio will add the functions use
, after
and ready
to the instance.
const server = {}
require('avvio')(server)
server.use(function first (s, opts, cb) {
// s is the same of server
s.use(function second (s, opts, cb) {
cb()
})
cb()
}).after(function (err, cb) {
// after first and second are finished
cb()
})
Options:
expose
: a key/value property to change how use
, after
and ready
are exposed.Events:
'start'
when the application startsThe avvio
function can be used also as a
constructor to inherits from.
function Server () {}
const app = require('avvio')(new Server())
app.use(function (s, opts, done) {
// your code
done()
})
app.on('start', () => {
// you app can start
})
Loads one or more functions asynchronously.
The function must have the signature: instance, options, done
Plugin example:
function plugin (server, opts, done) {
done()
}
app.use(plugin)
done
must be called only once, when your plugin is ready to go.
async/await is also supported:
async function plugin (server, opts) {
await sleep(10)
}
app.use(plugin)
use
returns the instance on which use
is called, to support a chainable API.
If you need to add more than a function and you don't need to use a different options object or callback, you can pass an array of functions to .use
.
app.use([first, second, third], opts)
The functions will be loaded in the same order as they are inside the array.
In order to handle errors in the loading plugins, you must use the
.ready()
method, like so:
app.use(function (instance, opts, done) {
done(new Error('error'))
}, opts)
app.ready(function (err) {
if (err) throw err
})
Calls a function after all the previously defined plugins are loaded, including
all their dependencies. The 'start'
event is not emitted yet.
The callback changes basing on the parameters your are giving:
error
object.error
object, the second will be the done
callback.error
object, the second will be the top level context
unless you have specified both server and override, in that case the context
will be what the override returns, and the third the done
callback.const server = {}
const app = require('avvio')(server)
...
// after with one parameter
app.after(function (err) {
if (err) throw err
})
// after with two parameter
app.after(function (err, done) {
if (err) throw err
done()
})
// after with three parameters
app.after(function (err, context, done) {
if (err) throw err
assert.equal(context, server)
done()
})
done
must be called only once.
Returns the instance on which after
is called, to support a chainable API.
Calls a function after all the plugins and after
call are completed, but before 'start'
is emitted. ready
callbacks are executed one at a time.
The callback changes basing on the parameters your are giving:
error
object.error
object, the second will be the done
callback.error
object, the second will be the top level context
unless you have specified both server and override, in that case the context
will be what the override returns, and the third the done
callback.const server = {}
const app = require('avvioo')(server)
...
// ready with one parameter
app.ready(function (err) {
if (err) throw err
})
// ready with two parameter
app.ready(function (err, done) {
if (err) throw err
done()
})
// ready with three parameters
app.ready(function (err, context, done) {
if (err) throw err
assert.equal(context, server)
done()
})
done
must be called only once.
Returns the instance on which ready
is called, to support a chainable API.
Same as:
const app = express()
const avvio = require('avvio')
avvio(app, {
expose: {
use: 'load'
}
})
Allows to override the instance of the server for each loading plugin. It allows the creation of an inheritance chain for the server instances. The first parameter is the server instance and the second is the plugin function while the third is the options object that you give to use.
const assert = require('assert')
const server = { count: 0 }
const app = require('avvio')(server)
console.log(app !== server, 'override must be set on the Avvio instance')
app.override = function (s, fn, opts) {
// create a new instance with the
// server as the prototype
const res = Object.create(s)
res.count = res.count + 1
return res
}
app.use(function first (s1, opts, cb) {
assert(s1 !== server)
assert(server.isPrototypeOf(s1))
assert(s1.count === 1)
s1.use(second)
cb()
function second (s2, opts, cb) {
assert(s2 !== s1)
assert(s1.isPrototypeOf(s2))
assert(s2.count === 2)
cb()
}
})
Registers a new callback that will be fired once then close
api is called.
The callback changes basing on the parameters your are giving:
context
.context
unless you have specified both server and override, in that case the context
will be what the override returns, the second will be the done
callback.const server = {}
const app = require('avvio')(server)
...
// onClose with one parameter
app.onClose(function (context) {
// ...
})
// onClose with two parameter
app.onClose(function (context, done) {
// ...
done()
})
done
must be called only once.
Returns the instance on which onClose
is called, to support a chainable API.
Starts the shotdown procedure, the callback is called once all the registered callbacks with onClose
has been executed.
The callback changes basing on the parameters your are giving:
error
object.error
object, the second will be the done
callback.error
object, the second will be the top level context
unless you have specified both server and override, in that case the context
will be what the override returns, and the third the done
callback.const server = {}
const app = require('avvio')(server)
...
// close with one parameter
app.close(function (err) {
if (err) throw err
})
// close with two parameter
app.close(function (err, done) {
if (err) throw err
done()
})
// close with three parameters
app.close(function (err, context, done) {
if (err) throw err
assert.equal(context, server)
done()
})
done
must be called only once.
This project was kindly sponsored by nearForm.
Copyright Matteo Collina 2016-2017, Licensed under MIT.
FAQs
Asynchronous bootstrapping of Node applications
The npm package avvio receives a total of 1,687,475 weekly downloads. As such, avvio popularity was classified as popular.
We found that avvio demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 10 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.