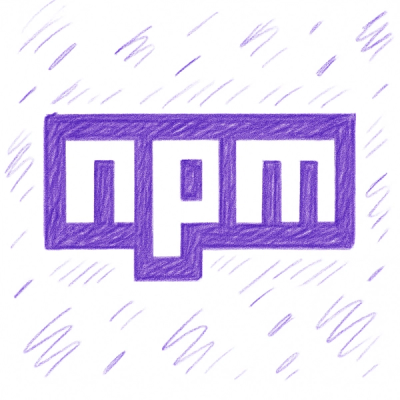
Security News
npm Adopts OIDC for Trusted Publishing in CI/CD Workflows
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
await-lock
Advanced tools
The await-lock npm package provides a simple mechanism for managing asynchronous locks in JavaScript. It allows you to ensure that only one asynchronous operation can proceed at a time, which is useful for managing resources that should not be accessed concurrently.
Basic Locking
This feature allows you to create a lock and use it to ensure that a critical section of code is not executed concurrently by multiple asynchronous functions. The code sample demonstrates acquiring a lock before entering a critical section and releasing it afterward.
const AwaitLock = require('await-lock');
const lock = new AwaitLock();
async function criticalSection() {
await lock.acquireAsync();
try {
// Perform operations that require exclusive access
} finally {
lock.release();
}
}
async function run() {
await Promise.all([criticalSection(), criticalSection()]);
}
run();
Try Lock
The try lock feature allows you to attempt to acquire a lock without waiting. If the lock is already held, the operation can proceed without blocking. This is useful for scenarios where you want to perform an operation only if it can be done immediately.
const lock = new AwaitLock();
async function tryLockExample() {
if (lock.tryAcquire()) {
try {
// Perform operations that require exclusive access
} finally {
lock.release();
}
} else {
console.log('Lock is already acquired by another operation.');
}
}
tryLockExample();
The async-mutex package provides similar functionality to await-lock by offering mutexes for asynchronous code. It allows for more complex locking mechanisms, such as reentrant locks and read-write locks, which can be more flexible than the simple locks provided by await-lock.
The semaphore-async-await package provides semaphore-based locking for asynchronous operations. Unlike await-lock, which provides a simple lock, this package allows you to control the number of concurrent operations, making it suitable for scenarios where you want to limit concurrency rather than enforce exclusivity.
Mutex locks for async functions
This package is published only as an ES module. In addition to importing ES modules from ES modules, modern versions of Node.js support requiring ES modules from CommonJS modules.
import AwaitLock from 'await-lock';
let lock = new AwaitLock();
async function runSerialTaskAsync() {
await lock.acquireAsync();
try {
// IMPORTANT: Do not return a promise from here because the finally clause
// may run before the promise settles, and the catch clause will not run if
// the promise is rejected
} finally {
lock.release();
}
}
You can also use AwaitLock with co and generator functions.
import AwaitLock from 'await-lock';
let runSerialTaskAsync = co.wrap(function*() {
yield lock.acquireAsync();
try {
// Run async code in the critical section
} finally {
lock.release();
}
});
FAQs
Mutex locks for async functions
The npm package await-lock receives a total of 189,815 weekly downloads. As such, await-lock popularity was classified as popular.
We found that await-lock demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
Research
/Security News
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Security News
The CNA Scorecard ranks CVE issuers by data completeness, revealing major gaps in patch info and software identifiers across thousands of vulnerabilities.