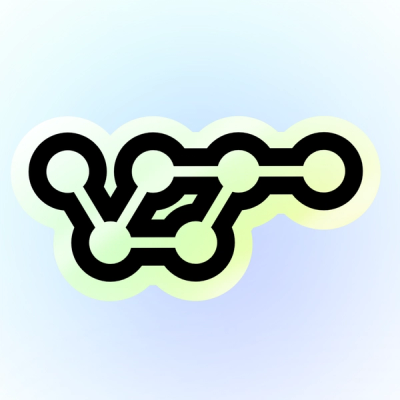
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
The AWS Cloud Development Kit (AWS CDK) is an open-source software development framework to define cloud infrastructure in code and provision it through AWS CloudFormation. It provides high-level components called constructs that preconfigure cloud resources with proven defaults, so you can build cloud applications without needing to be an expert in AWS services.
Defining Infrastructure
This feature allows you to define AWS infrastructure using familiar programming languages. The code sample demonstrates how to define a new VPC using the AWS CDK.
const vpc = new ec2.Vpc(this, 'VPC');
Creating a Lambda Function
With AWS CDK, you can create serverless functions such as AWS Lambda. The code sample shows how to define a Lambda function with the Node.js 12.x runtime.
const lambdaFunction = new lambda.Function(this, 'MyFunction', {
runtime: lambda.Runtime.NODEJS_12_X,
handler: 'index.handler',
code: lambda.Code.fromAsset('lambda')
});
Hosting a Static Website
AWS CDK can be used to host static websites on Amazon S3. The code sample illustrates how to create an S3 bucket configured for website hosting and how to deploy website content to it.
const bucket = new s3.Bucket(this, 'WebsiteBucket', {
websiteIndexDocument: 'index.html'
});
new s3deploy.BucketDeployment(this, 'DeployWebsite', {
sources: [s3deploy.Source.asset('./website-dist')],
destinationBucket: bucket
});
Setting up an API Gateway
This feature enables the creation of Amazon API Gateway endpoints. The code sample shows how to set up a REST API with a GET method integrated with a Lambda function.
const api = new apigateway.RestApi(this, 'MyApi', {
restApiName: 'Service'
});
const getLambdaIntegration = new apigateway.LambdaIntegration(getLambda);
api.root.addMethod('GET', getLambdaIntegration);
The Serverless Framework is similar to AWS CDK in that it allows you to define and deploy cloud infrastructure using code. It supports multiple cloud providers and is focused on building serverless applications. It uses a configuration file approach rather than an object-oriented programming model.
Terraform by HashiCorp is an infrastructure as code tool that allows you to define both cloud and on-premises resources with a declarative configuration language. It is cloud-agnostic and supports multiple providers, whereas AWS CDK is focused on AWS services.
Pulumi is an infrastructure as code tool that allows you to define infrastructure using general-purpose programming languages. Similar to AWS CDK, Pulumi provides an object-oriented approach but supports multiple cloud providers, not just AWS.
The AWS CDK Toolkit provides the cdk
command-line interface that can be used to work with AWS CDK applications.
Command | Description |
---|---|
cdk docs | Access the online documentation |
cdk init | Start a new CDK project (app or library) |
cdk list | List stacks in an application |
cdk synth | Synthesize a CDK app to CloudFormation template(s) |
cdk diff | Diff stacks against current state |
cdk deploy | Deploy a stack into an AWS account |
cdk destroy | Deletes a stack from an AWS account |
cdk bootstrap | Deploy a toolkit stack to support deploying large stacks & artifacts |
cdk doctor | Inspect the environment and produce information useful for troubleshooting |
This module is part of the AWS Cloud Development Kit project.
cdk docs
Outputs the URL to the documentation for the current toolkit version, and attempts to open a browser to that URL.
$ # Open the documentation in the default browser (using 'open')
$ cdk docs
https://awslabs.github.io/aws-cdk/versions/0.7.4-beta/
$ # Open the documentation in Chrome.
$ cdk docs --browser='chrome %u'
https://awslabs.github.io/aws-cdk/versions/0.7.4-beta/
cdk init
Creates a new CDK project.
$ # List the available template types & languages
$ cdk init --list
Available templates:
* app: Template for a CDK Application
└─ cdk init app --language=[java|typescript]
* lib: Template for a CDK Construct Library
└─ cdk init lib --language=typescript
$ # Create a new library application in typescript
$ cdk init lib --language=typescript
cdk list
Lists the stacks modeled in the CDK app.
$ # List all stacks in the CDK app 'node bin/main.js'
$ cdk list --app='node bin/main.js'
Foo
Bar
Baz
$ # List all stack including all details (add --json to output JSON instead of YAML)
$ cdk list --app='node bin/main.js' --long
-
name: Foo
environment:
name: 000000000000/bermuda-triangle-1
account: '000000000000'
region: bermuda-triangle-1
-
name: Bar
environment:
name: 111111111111/bermuda-triangle-2
account: '111111111111'
region: bermuda-triangle-2
-
name: Baz
environment:
name: 333333333333/bermuda-triangle-3
account: '333333333333'
region: bermuda-triangle-3
cdk synthesize
Synthesize the CDK app and outputs CloudFormation templates. If the application contains multiple stacks and no
stack name is provided in the command-line arguments, the --output
option is mandatory and a CloudFormation template
will be generated in the output folder for each stack.
By default, templates are generated in YAML format. The --json
option can be used to switch to JSON.
$ # Generate the template for StackName and output it to STDOUT
$ cdk synthesize --app='node bin/main.js' MyStackName
$ # Generate the template for MyStackName and save it to template.yml
$ cdk synth --app='node bin/main.js' MyStackName --output=template.yml
$ # Generate templates for all the stacks and save them into templates/
$ cdk synth --app='node bin/main.js' --output=templates
cdk diff
Computes differences between the infrastructure specified in the current state of the CDK app and the currently deployed application (or a user-specified CloudFormation template). This command returns non-zero if any differences are found.
$ # Diff against the currently deployed stack
$ cdk diff --app='node bin/main.js' MyStackName
$ # Diff against a specific template document
$ cdk diff --app='node bin/main.js' MyStackName --template=path/to/template.yml
cdk deploy
Deploys a stack of your CDK app to it's environment. During the deployment, the toolkit will output progress
indications, similar to what can be observed in the AWS CloudFormation Console. If the environment was never
bootstrapped (using cdk bootstrap
), only stacks that are not using assets and synthesize to a template that is under
51,200 bytes will successfully deploy.
$ cdk deploy --app='node bin/main.js' MyStackName
cdk destroy
Deletes a stack from it's environment. This will cause the resources in the stack to be destroyed (unless they were
configured with a DeletionPolicy
of Retain
). During the stack destruction, the command will output progress
information similar to what cdk deploy
provides.
$ cdk destroy --app='node bin/main.js' MyStackName
cdk bootstrap
Deploys a CDKToolkit
CloudFormation stack into the specified environment(s), that provides an S3 bucket that
cdk deploy
will use to store synthesized templates and the related assets, before triggering a CloudFormation stack
update. The name of the deployed stack can be configured using the --toolkit-stack-name
argument.
$ # Deploys to all environments
$ cdk bootstrap --app='node bin/main.js'
$ # Deploys only to environments foo and bar
$ cdk bootstrap --app='node bin/main.js' foo bar
cdk doctor
Inspect the current command-line environment and configurations, and collect information that can be useful for troubleshooting problems. It is usually a good idea to include the information provided by this command when submitting a bug report.
$ cdk doctor
ℹ️ CDK Version: 0.7.4-beta (build 0191444)
ℹ️ AWS environment variables:
- AWS_EC2_METADATA_DISABLED = 1
- AWS_SDK_LOAD_CONFIG = 1
On top of passing configuration through command-line arguments, it is possible to use JSON configuration files. The configuration's order of precedence is:
./cdk.json
)~/.cdk.json
)Some of the interesting keys that can be used in the JSON configuration files:
{
"app": "node bin/main.js", // Command to start the CDK app (--app='node bin/main.js')
"context": { // Context entries (--context=key=value)
"key": "value",
},
"toolkitStackName": "foo", // Customize 'bootstrap' stack name (--toolkit-stack-name=foo)
"versionReporting": false, // Opt-out of version reporting (--no-version-reporting)
}
FAQs
CDK Toolkit, the command line tool for CDK apps
The npm package aws-cdk receives a total of 1,938,338 weekly downloads. As such, aws-cdk popularity was classified as popular.
We found that aws-cdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.