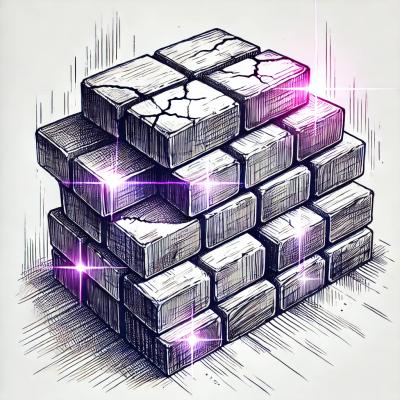
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
aws-core-utils
Advanced tools
Core utilities for working with Amazon Web Services (AWS), including ARNs, regions, stages, Lambdas, AWS errors, stream events, etc.
Core utilities for working with Amazon Web Services (AWS), including ARNs, regions, stages, Kinesis, Lambdas, AWS errors, stream events, etc.
Currently includes:
This module is exported as a Node.js module.
Using npm:
$ {sudo -H} npm i -g npm
$ npm i --save aws-core-utils
In Node.js:
const arns = require('aws-core-utils/arns');
const arnComponent = arns.getArnComponent(arn, index);
const arnPartition = arns.getArnPartition(arn);
const arnService = arns.getArnService(arn);
const arnRegion = arns.getArnRegion(arn);
const arnAccountId = arns.getArnAccountId(arn);
const arnResources = arns.getArnResources(arn);
const awsErrors = require('aws-core-utils/aws-errors');
const regions = require('aws-core-utils/regions');
// To get the current AWS region
const region = regions.getRegion();
// To configure a context with the current AWS region
regions.configureRegion(context, failFast)
const kinesisUtils = require('aws-core-utils/kinesis-utils');
// Preamble to create a context and configure logging on the context
const context = {};
const logging = require('logging-utils');
logging.configureDefaultLogging(context);
// Define the Kinesis constructor options that you want to use, e.g.
const kinesisOptions = {
// See http://docs.aws.amazon.com/AWSJavaScriptSDK/latest/AWS/Kinesis.html#constructor-property for full details
maxRetries: 0
// ...
};
// To create and cache a new AWS Kinesis instance with the given Kinesis constructor options for either the current
// region or the region specified in the given options OR reuse a previously cached Kinesis instance (if any) that is
// compatible with the given options
const kinesis = kinesisUtils.setKinesis(kinesisOptions, context);
// To configure a new AWS.Kinesis instance (or re-use a cached instance) on a context
kinesisUtils.configureKinesis(context, kinesisOptions);
console.log(context.kinesis);
// To get a previously set or configured AWS Kinesis instance for the current AWS region
const kinesis1 = kinesisUtils.getKinesis();
// ... or for a specified region
const kinesis2 = kinesisUtils.getKinesis('us-west-2');
// To get the original options that were used to construct a cached AWS Kinesis instance for the current or specified AWS region
const optionsUsed1 = kinesisUtils.getKinesisOptionsUsed();
const optionsUsed2 = kinesisUtils.getKinesisOptionsUsed('us-west-1');
// To delete and remove a cached Kinesis instance from the cache
const deleted = kinesisUtils.deleteKinesis('eu-west-1');
const lambdas = require('aws-core-utils/lambdas');
// Fail a Lambda's callback with a standard error and preserve HTTP status codes (for non-API Gateway Lambdas)
// See core-functions/app-errors.js for standard errors to use
lambdas.failCallback(lambdaCallback, error, awsContext, message, code);
// Fail an API Gateway-exposed Lambda's callback with a standard error and preserve HTTP status codes
lambdas.failCallbackForApiGateway(lambdaCallback, error, awsContext, message, code, allowedHttpStatusCodes);
// To resolve the Lambda alias from an AWS Lambda context
const alias = lambdas.getAlias(awsContext);
// To extract other details from an AWS Lambda context
const functionName = lambdas.getFunctionName(awsContext);
const functionVersion = lambdas.getFunctionVersion(awsContext);
const functionNameVersionAndAlias = lambdas.getFunctionNameVersionAndAlias(awsContext);
const invokedFunctionArn = lambdas.getInvokedFunctionArn(awsContext);
const invokedFunctionArnFunctionName = lambdas.getInvokedFunctionArnFunctionName(awsContext);
const stages = require('aws-core-utils/stages');
// To configure default stage handling, which sets the default behaviour of the next 4 functions
stages.configureDefaultStageHandling(context, forceConfiguration);
// 1. To resolve / derive a stage from an AWS event
const context = {};
const stage = stages.resolveStage(event, awsContext, context);
// 2. To configure a context with a resolved stage
stages.configureStage(context, event, awsContext, failFast)
// 3. To qualify an unqualified stream name with a stage
const unqualifiedStreamName = 'TestStream';
const stageQualifiedStreamName = stages.toStageQualifiedStreamName(unqualifiedStreamName, stage, context);
// 4. To extract a stage from a qualified stream name
const qualifiedStreamName = 'TestStream_PROD';
const stage2 = stages.extractStageFromQualifiedStreamName(qualifiedStreamName, context);
// 5. To qualify an unqualified resource name with a stage
const unqualifiedResourceName = 'TestResource';
const stageQualifiedResourceName = stages.toStageQualifiedResourceName(unqualifiedResourceName, stage, context);
// 6. To extract a stage from a qualified resource name
const qualifiedResourceName = 'TestResource_QA';
const stage3 = stages.extractStageFromQualifiedResourceName(qualifiedResourceName, context);
// To configure completely customised stage handling of the above 6 functions
const settings = {
customToStage: customToStage,
convertAliasToStage: convertAliasToStage,
injectStageIntoStreamName: injectStageIntoStreamName,
extractStageFromStreamName: extractStageFromStreamName,
streamNameStageSeparator: streamNameStageSeparator,
injectStageIntoResourceName: injectStageIntoResourceName,
extractStageFromResourceName: extractStageFromResourceName,
resourceNameStageSeparator: resourceNameStageSeparator,
injectInCase: injectInCase,
extractInCase: extractInCase,
defaultStage: defaultStage,
}
stages.configureStageHandling(context, settings, forceConfiguration);
// To check if stage handling is configured
stages.isStageHandlingConfigured(context);
// To look up stage handling settings and functions
const setting = stages.getStageHandlingSetting(context, settingName);
const fn = stages.getStageHandlingFunction(context, settingName);
const streamEvents = require('aws-core-utils/stream-events');
// To extract stream names form AWS event source ARNs
const eventSourceARNs = streamEvents.getEventSourceARNs(event);
const eventSourceStreamNames = streamEvents.getEventSourceStreamNames(event);
const eventSourceStreamName = streamEvents.getEventSourceStreamName(record);
// Simple checks to validate existance of some of parameters of Kinesis & DynamoDB stream event records
try {
streamEvents.validateStreamEventRecord(record);
streamEvents.validateKinesisStreamEventRecord(record);
streamEvents.validateDynamoDBStreamEventRecord(record);
} catch (err) {
// ...
}
This module's unit tests were developed with and must be run with tape. The unit tests have been tested on Node.js v4.3.2.
Install tape globally if you want to run multiple tests at once:
$ npm install tape -g
Run all unit tests with:
$ npm test
or with tape:
$ tape test/*.js
See the package source for more details.
stages
module:
configureStageHandling
function to accept a setting object instead of the multiple fixed parameters,
to simplify configuration of new, custom settings.kinesis-utils
module to enable full configuration of an AWS Kinesis instance and caching of a Kinesis
instance per region.
setKinesis
, getKinesis
, getKinesisOptionsUsed
& deleteKinesis
functions and unit tests for same.configureKinesis
function to use the new setKinesis
function and patched its unit tests.kinesis-utils
module to provide basic configuration and caching of an AWS.Kinesis instance for Lambdastream-events
module:
validateStreamEventRecord
function to check if a record is either a valid Kinesis or DynamoDB stream event recordvalidateKinesisStreamEventRecord
function to check if a record is a valid Kinesis stream event recordvalidateDynamoDBStreamEventRecord
function to check if a record is a valid DynamoDB stream event recordsetRegionIfNotSet
to eliminate unnecessary logging when regions are the samecore-functions
dependency to version 2.0.2logging-utils
dependency to version 1.0.5stages
:
resolveStage
-specific configuration to general stage handling configuration.
configureStage
function.toStageQualifiedStreamName
and default toStageSuffixedStreamName
functions.extractStageFromQualifiedStreamName
and default extractStageFromSuffixedStreamName
functions.toStageQualifiedResourceName
and default toStageSuffixedResourceName
functions.extractStageFromQualifiedResourceName
and default extractStageFromSuffixedResourceName
functions.stages
module.regions
:
configureRegion
function.getRegion
function needed for configureRegion
function.aws-errors
:
lambdas
:
failCallback
function to fail non-API Gateway Lambda callbacks with standard app errors to facilitate mapping of errors to HTTP status codesfailCallbackForApiGateway
function to fail API Gateway Lambda callbacks with standard app errors to facilitate mapping of errors to HTTP status codesstream-events
module and unit tests for it.core-functions
dependency to version 1.2.0.logging-utils
1.0.2 dependency.Initial commit
2.1.0
stages
module:
configureStageHandling
function to accept a setting object instead of the multiple fixed parameters,
to simplify configuration of new, custom settings.kinesis-utils
module to enable full configuration of an AWS Kinesis instance and caching of a Kinesis
instance per region.
setKinesis
, getKinesis
, getKinesisOptionsUsed
& deleteKinesis
functions and unit tests for same.configureKinesis
function to use the new setKinesis
function and patched its unit tests.FAQs
Core utilities for working with Amazon Web Services (AWS), including ARNs, regions, stages, Lambdas, AWS errors, stream events, Kinesis, DynamoDB.DocumentClients, etc.
The npm package aws-core-utils receives a total of 24 weekly downloads. As such, aws-core-utils popularity was classified as not popular.
We found that aws-core-utils demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.