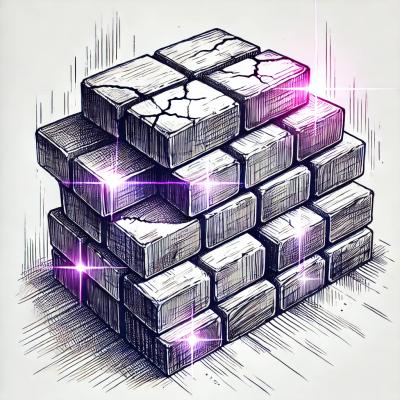
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
aws-core-utils
Advanced tools
Core utilities for working with Amazon Web Services (AWS), including ARNs, regions, stages, Lambdas, AWS errors, stream events, Kinesis, DynamoDB.DocumentClients, etc.
Core utilities for working with Amazon Web Services (AWS), including ARNs, regions, stages, Lambdas, AWS errors, stream events, Kinesis, DynamoDB.DocumentClients, etc.
Currently includes:
This module is exported as a Node.js module.
Using npm:
$ {sudo -H} npm i -g npm
$ npm i --save aws-core-utils
In Node.js:
const arns = require('aws-core-utils/arns');
const arnComponent = arns.getArnComponent(arn, index);
const arnPartition = arns.getArnPartition(arn);
const arnService = arns.getArnService(arn);
const arnRegion = arns.getArnRegion(arn);
const arnAccountId = arns.getArnAccountId(arn);
const arnResources = arns.getArnResources(arn);
const awsErrors = require('aws-core-utils/aws-errors');
const regions = require('aws-core-utils/regions');
// To get the current AWS region
const region = regions.getRegion();
// To configure a context with the current AWS region
regions.configureRegion(context, failFast)
const dynamoDBDocClientCache = require('aws-core-utils/dynamodb-doc-client-cache');
// Preamble to create a context and configure logging on the context
const context = {};
const logging = require('logging-utils');
logging.configureDefaultLogging(context); // or your own custom logging configuration (see logging-utils README.md)
// Define the DynamoDB.DocumentClient's constructor options that you want to use, e.g.
const dynamoDBDocClientOptions = {
// See http://docs.aws.amazon.com/AWSJavaScriptSDK/latest/AWS/DynamoDB/DocumentClient.html#constructor-property
// and http://docs.aws.amazon.com/AWSJavaScriptSDK/latest/AWS/DynamoDB.html#constructor-property
maxRetries: 0
// ...
};
// To create and cache a new AWS DynamoDB.DocumentClient instance with the given DynamoDB.DocumentClient constructor
// options for either the current region or the region specified in the given options OR reuse a previously cached
// DynamoDB.DocumentClient instance (if any) that is compatible with the given options
const dynamoDBDocClient = dynamoDBDocClientCache.setDynamoDBDocClient(dynamoDBDocClientOptions, context);
// To configure a new AWS.DynamoDB.DocumentClient instance (or re-use a cached instance) on a context
dynamoDBDocClientCache.configureDynamoDBDocClient(context, dynamoDBDocClientOptions);
console.log(context.dynamoDBDocClient);
// To get a previously set or configured AWS DynamoDB.DocumentClient instance for the current AWS region
const dynamoDBDocClient1 = dynamoDBDocClientCache.getDynamoDBDocClient();
// ... or for a specified region
const dynamoDBDocClient2 = dynamoDBDocClientCache.getDynamoDBDocClient('us-west-2');
// To get the original options that were used to construct a cached AWS DynamoDB.DocumentClient instance for the current or specified AWS region
const optionsUsed1 = dynamoDBDocClientCache.getDynamoDBDocClientOptionsUsed();
const optionsUsed2 = dynamoDBDocClientCache.getDynamoDBDocClientOptionsUsed('us-west-1');
// To delete and remove a cached DynamoDB.DocumentClient instance from the cache
const deleted = dynamoDBDocClientCache.deleteDynamoDBDocClient('eu-west-1');
const kinesisCache = require('aws-core-utils/kinesis-cache');
// Preamble to create a context and configure logging on the context
const context = {};
const logging = require('logging-utils');
logging.configureDefaultLogging(context); // or your own custom logging configuration (see logging-utils README.md)
// Define the Kinesis constructor options that you want to use, e.g.
const kinesisOptions = {
// See http://docs.aws.amazon.com/AWSJavaScriptSDK/latest/AWS/Kinesis.html#constructor-property for full details
maxRetries: 0
// ...
};
// To create and cache a new AWS Kinesis instance with the given Kinesis constructor options for either the current
// region or the region specified in the given options OR reuse a previously cached Kinesis instance (if any) that is
// compatible with the given options
const kinesis = kinesisCache.setKinesis(kinesisOptions, context);
// To configure a new AWS.Kinesis instance (or re-use a cached instance) on a context
kinesisCache.configureKinesis(context, kinesisOptions);
console.log(context.kinesis);
// To get a previously set or configured AWS Kinesis instance for the current AWS region
const kinesis1 = kinesisCache.getKinesis();
// ... or for a specified region
const kinesis2 = kinesisCache.getKinesis('us-west-2');
// To get the original options that were used to construct a cached AWS Kinesis instance for the current or specified AWS region
const optionsUsed1 = kinesisCache.getKinesisOptionsUsed();
const optionsUsed2 = kinesisCache.getKinesisOptionsUsed('us-west-1');
// To delete and remove a cached Kinesis instance from the cache
const deleted = kinesisCache.deleteKinesis('eu-west-1');
const lambdas = require('aws-core-utils/lambdas');
// Fail a Lambda's callback with a standard error and preserve HTTP status codes (for non-API Gateway Lambdas)
// See core-functions/app-errors.js for standard errors to use
lambdas.failCallback(lambdaCallback, error, awsContext, message, code);
// Fail an API Gateway-exposed Lambda's callback with a standard error and preserve HTTP status codes
lambdas.failCallbackForApiGateway(lambdaCallback, error, awsContext, message, code, allowedHttpStatusCodes);
// To resolve the Lambda alias from an AWS Lambda context
const alias = lambdas.getAlias(awsContext);
// To extract other details from an AWS Lambda context
const functionName = lambdas.getFunctionName(awsContext);
const functionVersion = lambdas.getFunctionVersion(awsContext);
const functionNameVersionAndAlias = lambdas.getFunctionNameVersionAndAlias(awsContext);
const invokedFunctionArn = lambdas.getInvokedFunctionArn(awsContext);
const invokedFunctionArnFunctionName = lambdas.getInvokedFunctionArnFunctionName(awsContext);
// To configure stage-handling, which determines the behaviour of the functions numbered 1 to 6 below
const stages = require('aws-core-utils/stages');
const settings = undefined; // ... or your own custom settings
const options = require('./stages-options.json'); // ... or your own custom options
// ... EITHER using the default stage handling configuration partially customised via config.stageHandlingOptions
stages.configureDefaultStageHandling(context, options.stageHandlingOptions, otherSettings, otherOptions, forceConfiguration);
// ... OR using your own custom stage-handling configuration
const stageHandlingSettings = stages.getDefaultStageHandlingSettings(options.stageHandlingOptions);
// Optionally override the default stage handling functions with your own custom functions
// stageHandlingSettings.customToStage = undefined;
// stageHandlingSettings.convertAliasToStage = stages.DEFAULTS.convertAliasToStage;
// stageHandlingSettings.injectStageIntoStreamName = stages.DEFAULTS.toStageSuffixedStreamName;
// stageHandlingSettings.extractStageFromStreamName = stages.DEFAULTS.extractStageFromSuffixedStreamName;
// stageHandlingSettings.injectStageIntoResourceName = stages.DEFAULTS.toStageSuffixedResourceName;
// stageHandlingSettings.extractStageFromResourceName = stages.DEFAULTS.extractStageFromSuffixedResourceName;
stages.configureStageHandling(context, stageHandlingSettings, undefined, otherSettings, otherOptions, forceConfiguration);
// ... OR using completely customised stage handling settings
const stageHandlingSettings2 = {
envStageName: myEnvStageName,
customToStage: myCustomToStageFunction, // or undefined if not needed
convertAliasToStage: myConvertAliasToStageFunction, // or undefined to knockout using AWS aliases as stages
injectStageIntoStreamName: myInjectStageIntoStreamNameFunction,
extractStageFromStreamName: myExtractStageFromStreamNameFunction,
streamNameStageSeparator: myStreamNameStageSeparator,
injectStageIntoResourceName: myInjectStageIntoResourceNameFunction,
extractStageFromResourceName: myExtractStageFromResourceNameFunction,
resourceNameStageSeparator: myResourceNameStageSeparator,
injectInCase: myInjectInCase,
extractInCase: myExtractInCase,
defaultStage: myDefaultStage, // or undefined
}
stages.configureStageHandling(context, stageHandlingSettings2, undefined, otherSettings, otherOptions, forceConfiguration);
// ... OR using custom stage handling settings and/or options and configuring dependencies at the same time
stages.configureStageHandling(context, stageHandlingSettings, stageHandlingOptions, otherSettings, otherOptions, forceConfiguration);
// To check if stage handling is configured
const configured = stages.isStageHandlingConfigured(context);
// To look up stage handling settings and functions
const setting = stages.getStageHandlingSetting(context, settingName);
const fn = stages.getStageHandlingFunction(context, functionSettingName);
// 1. To resolve / derive a stage from an AWS event
const context = {};
const stage = stages.resolveStage(event, awsContext, context);
// 2. To configure a context with a resolved stage
stages.configureStage(context, event, awsContext, failFast)
// 3. To qualify an unqualified stream name with a stage
const unqualifiedStreamName = 'TestStream';
const stageQualifiedStreamName = stages.toStageQualifiedStreamName(unqualifiedStreamName, stage, context);
// 4. To extract a stage from a qualified stream name
const qualifiedStreamName = 'TestStream_PROD';
const stage2 = stages.extractStageFromQualifiedStreamName(qualifiedStreamName, context);
// 5. To qualify an unqualified resource name with a stage
const unqualifiedResourceName = 'TestResource';
const stageQualifiedResourceName = stages.toStageQualifiedResourceName(unqualifiedResourceName, stage, context);
// 6. To extract a stage from a qualified resource name
const qualifiedResourceName = 'TestResource_QA';
const stage3 = stages.extractStageFromQualifiedResourceName(qualifiedResourceName, context);
const streamEvents = require('aws-core-utils/stream-events');
// To extract event soure ARNs from AWS events
const eventSourceARNs = streamEvents.getEventSourceARNs(event);
// To extract Kinesis stream names from AWS events and event records
const eventSourceStreamNames = streamEvents.getKinesisEventSourceStreamNames(event);
const eventSourceStreamName = streamEvents.getKinesisEventSourceStreamName(record);
// To extract DynamoDB table names from DynamoDB stream event records
const dynamoDBEventSourceTableName = streamEvents.getDynamoDBEventSourceTableName(record);
// To extract DynamoDB table names and stream timestamps/suffixes from DynamoDB stream event records
const tableNameAndStreamTimestamp = streamEvents.getDynamoDBEventSourceTableNameAndStreamTimestamp(record);
const dynamoDBEventSourceTableName1 = tableNameAndStreamTimestamp[0];
const dynamoDBEventSourceStreamTimestamp = tableNameAndStreamTimestamp[1];
// Simple checks to validate existance of some of the properties of Kinesis & DynamoDB stream event records
try {
streamEvents.validateStreamEventRecord(record);
streamEvents.validateKinesisStreamEventRecord(record);
streamEvents.validateDynamoDBStreamEventRecord(record);
} catch (err) {
// ...
}
This module's unit tests were developed with and must be run with tape. The unit tests have been tested on Node.js v4.3.2.
Install tape globally if you want to run multiple tests at once:
$ npm install tape -g
Run all unit tests with:
$ npm test
or with tape:
$ tape test/*.js
See the package source for more details.
logging-utils
dependency to version 3.0.8stages-defs.js
module to type-defs.js
to synchronize with other modulesstage-handling-type-defs.js
module to stages-type-defs.js
stage-handling-type-defs.js
module to hold all of the stage handling related typedefs
StageHandlingOptions
and StageHandlingSettings
typedefs from stages.js
StageHandling
and StageAware
typedefsstages.js
module:
StageHandlingOptions
and StageHandlingSettings
typedefslogging-utils
dependency to version 3.0.7core-functions
dependency to version 2.0.11logging-utils
dependency to version 3.0.6node-uuid
dependency with uuid
dependency in test\package.json
stream-events
module:
getEventSources
functiongetDynamoDBEventSourceTableNames
functionstages
module:
resolveStage
function to resolve the event's eventSource and when its DynamoDB to use the table names
of the DynamoDB stream event as a stage source, instead of always assuming the event is a Kinesis stream event
and only using the stream names of the Kinesis stream event as a stage sourcecore-functions
dependency to version 2.0.10logging-utils
dependency to version 3.0.5core-functions
dependency to version 2.0.9logging-utils
dependency to version 3.0.3core-functions
dependency to version 2.0.8logging-utils
dependency to version 3.0.2stages.js
module:
configureStageHandlingWithSettings
to FOR_TESTING_ONLY
README.md
stages.js
module:
configureStageHandling
function to use core-functions/objects
module's copy
and
merge
functions to ensure that any and all given custom settings and options are not lostgetDefaultStageHandlingSettings
& loadDefaultStageHandlingOptions
functions to use
core-functions/objects
module's copy
and merge
functions to ensure that any and all given
custom options are not lostkinesis-cache.js
module:
setKinesis
to only modify a copy of the given kinesisOptions to avoid side-effectsdynamodb-doc-client-cache.js
module:
setDynamoDBDocClient
to only modify a copy of the given dynamoDBDocClientOptions to avoid side-effectscore-functions
dependency to version 2.0.7tape
dependency to 4.6.3arns.js
module:
getArnResources
function to support DynamoDB eventSourceARNsstream-events.js
module:
getEventSourceStreamNames
function to getKinesisEventSourceStreamNames
getEventSourceStreamName
function to getKinesisEventSourceStreamName
getDynamoDBEventSourceTableName
functiongetDynamoDBEventSourceTableNameAndStreamTimestamp
functionstages.js
module:
configureStageHandling
function to configureStageHandlingWithSettings
configureStageHandlingAndDependencies
function to configureStageHandling
configureDependenciesIfNotConfigured
functionconfigureDefaultStageHandlingIfNotConfigured
functionconfigureStageHandlingIfNotConfigured
functionconfig.json
to stages-options.json
core-functions
dependency to version 2.0.5logging-utils
dependency to version 3.0.0kinesis-utils
module to kinesis-cache
to better reflect its actual purposedynamodb-doc-clients
module to dynamodb-doc-client-cache
to better reflect its actual purposedynamodb-utils
modulestages.js
module:
configureStageHandlingAndDependencies
function to enable configuration of stage handling settings and
all stage handling dependencies (currently just logging) at the same timeconfigureDependencies
function, which is used by the new configureStageHandlingAndDependencies
functionenvStageName
setting to enable configuration of the name of the process.env
environment variable to be
checked for a stage value during execution of the resolveStage
or configureStage
functionsresolveStage
function to first attempt to resolve a stage from a named process.env
environment variable
(if available), which must be configured using AWS Lambda's new environment supportstages.js
module:
configureDependenciesIfNotConfigured
function to configure stage handling dependencies (i.e. only logging for now)configureStageHandlingIfNotConfigured
function to first invoke new configureDependenciesIfNotConfigured
functionconfigureStageHandling
function to accept otherSettings
and otherOptions
as 3rd & 4th arguments to
enable configuration of dependencies and to first invoke invoke new configureDependenciesIfNotConfigured
functionconfigureDefaultStageHandling
function to accept otherSettings
and otherOptions
as 3rd & 4th arguments
to enable configuration of dependencies and to always invoke configureStageHandling
stages.js
module:
configureStageHandlingIfNotConfigured
functionconfigureDefaultStageHandlingIfNotConfigured
function to use new configureStageHandlingIfNotConfigured
functionlogging-utils
dependency to version 2.0.3stages.js
module:
StageHandlingOptions
to better define parameters and return typesgetDefaultStageHandlingSettings
function to accept an options
argument of type StageHandlingOptions
instead of an arbitrary config
object and to also load default options from config.json
configureDefaultStageHandling
function to accept a new options
argument of type StageHandlingOptions
to enable optional, partial overriding of default stage handling settingsstageHandlingSettings
to stageHandlingOptions
in config.json
logging-utils
linklogging-utils
dependency to version 2.0.1dynamodb-doc-clients
and kinesis-utils
modules.dynamodb-doc-clients
module to enable creation and configuration of AWS DynamoDB.DocumentClient instances
and caching of a DynamoDB.DocumentClient instance per region. Note that this new module is an almost exact replica of
the kinesis-utils
module, but for getting, setting and caching DynamoDB.DocumentClient instances instead of Kinesis
instances.
setDynamoDBDocClient
, getDynamoDBDocClient
, getDynamoDBDocClientOptionsUsed
, deleteDynamoDBDocClient
and configureDynamoDBDocClient
functions and unit tests for same.core-functions
dependency to version 2.0.3logging-utils
dependency to version 1.0.6getDefaultStageHandlingSettings
function to get the default stage handling settingsstages
module:
configureStageHandling
function to accept a setting object instead of the multiple fixed parameters,
to simplify configuration of new, custom settings.kinesis-utils
module to enable full configuration of an AWS Kinesis instance and caching of a Kinesis
instance per region.
setKinesis
, getKinesis
, getKinesisOptionsUsed
& deleteKinesis
functions and unit tests for same.configureKinesis
function to use the new setKinesis
function and patched its unit tests.kinesis-utils
module to provide basic configuration and caching of an AWS.Kinesis instance for Lambdastream-events
module:
validateStreamEventRecord
function to check if a record is either a valid Kinesis or DynamoDB stream event recordvalidateKinesisStreamEventRecord
function to check if a record is a valid Kinesis stream event recordvalidateDynamoDBStreamEventRecord
function to check if a record is a valid DynamoDB stream event recordsetRegionIfNotSet
to eliminate unnecessary logging when regions are the samecore-functions
dependency to version 2.0.2logging-utils
dependency to version 1.0.5stages
:
resolveStage
-specific configuration to general stage handling configuration.
configureStage
function.toStageQualifiedStreamName
and default toStageSuffixedStreamName
functions.extractStageFromQualifiedStreamName
and default extractStageFromSuffixedStreamName
functions.toStageQualifiedResourceName
and default toStageSuffixedResourceName
functions.extractStageFromQualifiedResourceName
and default extractStageFromSuffixedResourceName
functions.stages
module.regions
:
configureRegion
function.getRegion
function needed for configureRegion
function.aws-errors
:
lambdas
:
failCallback
function to fail non-API Gateway Lambda callbacks with standard app errors to facilitate mapping of errors to HTTP status codesfailCallbackForApiGateway
function to fail API Gateway Lambda callbacks with standard app errors to facilitate mapping of errors to HTTP status codesstream-events
module and unit tests for it.core-functions
dependency to version 1.2.0.logging-utils
1.0.2 dependency.5.0.9
logging-utils
dependency to version 3.0.8stages-defs.js
module to type-defs.js
to synchronize with other modulesFAQs
Core utilities for working with Amazon Web Services (AWS), including ARNs, regions, stages, Lambdas, AWS errors, stream events, Kinesis, DynamoDB.DocumentClients, etc.
The npm package aws-core-utils receives a total of 24 weekly downloads. As such, aws-core-utils popularity was classified as not popular.
We found that aws-core-utils demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.