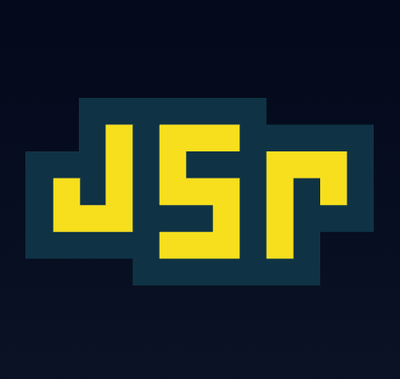
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The aws-sdk npm package is the official AWS SDK for JavaScript, providing JavaScript objects for AWS services including Amazon S3, EC2, DynamoDB, and more. It allows developers to interact with AWS services programmatically, enabling them to build scalable solutions with AWS infrastructure.
Interacting with Amazon S3
This code sample demonstrates how to retrieve an object from an Amazon S3 bucket using the aws-sdk.
{"const AWS = require('aws-sdk');
const s3 = new AWS.S3();
const params = { Bucket: 'myBucket', Key: 'myKey' };
s3.getObject(params, function(err, data) {
if (err) console.log(err, err.stack);
else console.log(data);
});"}
Managing EC2 Instances
This code sample shows how to describe EC2 instances, providing information about instances running in your AWS account.
{"const AWS = require('aws-sdk');
const ec2 = new AWS.EC2();
const params = { InstanceIds: ['i-1234567890abcdef0'] };
ec2.describeInstances(params, function(err, data) {
if (err) console.log(err, err.stack);
else console.log(data);
});"}
Working with DynamoDB
This code sample illustrates how to retrieve an item from a DynamoDB table using the aws-sdk.
{"const AWS = require('aws-sdk');
const dynamoDB = new AWS.DynamoDB();
const params = {
TableName: 'myTable',
Key: {
'myKey': { S: 'myKeyValue' }
}
};
dynamoDB.getItem(params, function(err, data) {
if (err) console.log(err, err.stack);
else console.log(data);
});"}
The google-cloud package is a client library for accessing Google Cloud services similar to how aws-sdk accesses AWS services. It supports services like Google Cloud Storage, BigQuery, and more. While aws-sdk is specific to AWS, google-cloud is tailored for Google Cloud Platform.
The ali-oss package is an SDK for Alibaba Cloud's OSS (Object Storage Service). It offers a subset of the features provided by aws-sdk, but specifically for Alibaba Cloud's storage service. It's a more specialized tool compared to the broad service coverage of aws-sdk.
The official AWS SDK for JavaScript, available for browsers and mobile devices, or Node.js backends
Release notes can be found at http://aws.amazon.com/releasenotes/SDK/JavaScript
If you are upgrading from 1.x to 2.0 of the SDK, please see the {file:UPGRADING.md} notes for information on how to migrate existing code to work with the new major version.
To use the SDK in the browser, simply add the following script tag to your HTML pages:
<script src="https://sdk.amazonaws.com/js/aws-sdk-2.0.6.min.js"></script>
The preferred way to install the AWS SDK for Node.js is to use the npm package manager for Node.js. Simply type the following into a terminal window:
npm install aws-sdk
You can find a getting started guide at:
http://docs.aws.amazon.com/AWSJavaScriptSDK/guide/
Note: Although all services are supported in the browser version of the SDK, not all of the services are available in the default hosted build (using the script tag provided above). A list of services in the hosted build are provided in the "Working With Services" section of the browser SDK guide, including instructions on how to build a custom version of the SDK with extra services.
The SDK currently supports the following services:
Service Name | Class Name | API Version |
---|---|---|
Amazon CloudFront | AWS.CloudFront | 2014-05-31 |
Amazon CloudSearch | AWS.CloudSearch | 2011-02-01 |
2013-01-01 | ||
Amazon CloudSearch Domain | AWS.CloudSearchDomain | 2013-01-01 |
Amazon CloudWatch | AWS.CloudWatch | 2010-08-01 |
Amazon CloudWatch Logs | AWS.CloudWatchLogs | 2014-03-28 |
Amazon Cognito Identity | AWS.CognitoIdentity | 2014-06-30 |
Amazon Cognito Sync | AWS.CognitoSync | 2014-06-30 |
Amazon DynamoDB | AWS.DynamoDB | 2011-12-05 |
2012-08-10 | ||
Amazon Elastic Compute Cloud | AWS.EC2 | 2014-05-01 |
Amazon Elastic MapReduce | AWS.EMR | 2009-03-31 |
Amazon Elastic Transcoder | AWS.ElasticTranscoder | 2012-09-25 |
Amazon ElastiCache | AWS.ElastiCache | 2014-03-24 |
Amazon Glacier | AWS.Glacier | 2012-06-01 |
Amazon Kinesis | AWS.Kinesis | 2013-12-02 |
Amazon Redshift | AWS.Redshift | 2012-12-01 |
Amazon Relational Database Service | AWS.RDS | 2013-01-10 |
2013-02-12 | ||
2013-09-09 | ||
Amazon Route 53 | AWS.Route53 | 2013-04-01 |
Amazon Simple Email Service | AWS.SES | 2010-12-01 |
Amazon Simple Notification Service | AWS.SNS | 2010-03-31 |
Amazon Simple Queue Service | AWS.SQS | 2012-11-05 |
Amazon Simple Storage Service | AWS.S3 | 2006-03-01 |
Amazon Simple Workflow Service | AWS.SWF | 2012-01-25 |
Amazon SimpleDB | AWS.SimpleDB | 2009-04-15 |
Auto Scaling | AWS.AutoScaling | 2011-01-01 |
AWS CloudFormation | AWS.CloudFormation | 2010-05-15 |
AWS CloudTrail | AWS.CloudTrail | 2013-11-01 |
AWS Data Pipeline | AWS.DataPipeline | 2012-10-29 |
AWS Direct Connect | AWS.DirectConnect | 2012-10-25 |
AWS Elastic Beanstalk | AWS.ElasticBeanstalk | 2010-12-01 |
AWS Identity and Access Management | AWS.IAM | 2010-05-08 |
AWS Import/Export | AWS.ImportExport | 2010-06-01 |
AWS OpsWorks | AWS.OpsWorks | 2013-02-18 |
AWS Security Token Service | AWS.STS | 2011-06-15 |
AWS Storage Gateway | AWS.StorageGateway | 2013-06-30 |
AWS Support | AWS.Support | 2013-04-15 |
Elastic Load Balancing | AWS.ELB | 2012-06-01 |
This SDK is distributed under the Apache License, Version 2.0, see LICENSE.txt and NOTICE.txt for more information.
FAQs
AWS SDK for JavaScript
The npm package aws-sdk receives a total of 6,955,867 weekly downloads. As such, aws-sdk popularity was classified as popular.
We found that aws-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.