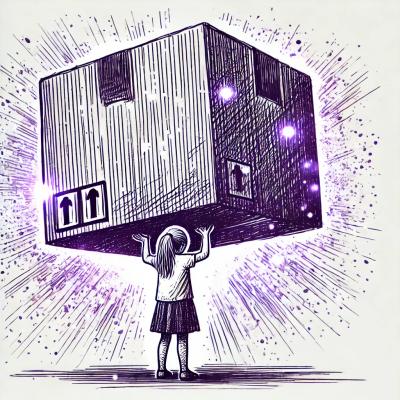
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Axios is a promise-based HTTP client for the browser and Node.js. It provides an easy-to-use API for sending asynchronous HTTP requests to REST endpoints and performing CRUD operations. It can be used to make XMLHttpRequests from the browser or HTTP requests from Node.js, supports the Promise API, and provides a way to intercept request and response, transform request and response data, and cancel requests.
Performing GET requests
This feature allows you to perform a GET request to retrieve data from a specified resource.
axios.get('/user?ID=12345').then(function (response) { console.log(response); }).catch(function (error) { console.log(error); });
Performing POST requests
This feature allows you to perform a POST request to send data to a server to create/update a resource.
axios.post('/user', { firstName: 'Fred', lastName: 'Flintstone' }).then(function (response) { console.log(response); }).catch(function (error) { console.log(error); });
Performing concurrent requests
This feature allows you to make multiple requests simultaneously.
axios.all([axios.get('/user/12345'), axios.get('/user/67890')]).then(axios.spread(function (acct, perms) { console.log(acct, perms); }));
Interceptors
This feature allows you to intercept requests or responses before they are handled by then or catch.
axios.interceptors.request.use(function (config) { config.headers.Authorization = AUTH_TOKEN; return config; }, function (error) { return Promise.reject(error); });
Creating instances
This feature allows you to create a new instance of axios with custom config defaults.
const instance = axios.create({ baseURL: 'https://api.example.com' }); instance.get('/users').then(function (response) { console.log(response); });
The Fetch API provides a JavaScript interface for accessing and manipulating parts of the HTTP pipeline, such as requests and responses. It is a modern alternative to XMLHttpRequest and is native to modern browsers. Unlike axios, it is not based on promises by default but can be used with promises.
Superagent is a light-weight progressive ajax API crafted for flexibility, readability, and a low learning curve after being frustrated with many of the existing request APIs. It is similar to axios but with a different API design and chainable methods.
Got is a human-friendly and powerful HTTP request library for Node.js. It is designed to be a simpler and more performant alternative to Node's native http module. Got offers stream support, promise APIs, and advanced features like retries and timeouts.
Request is a simplified HTTP request client 'simplified' with many features. It is one of the most popular HTTP client libraries but has been deprecated as of February 2020. It had a callback-based API, which is different from axios's promise-based API.
node-fetch is a light-weight module that brings the Fetch API to Node.js. It is an implementation of the window.fetch function for Node.js, aiming to provide a consistent API with the browser's fetch function. It is similar to axios in terms of promise-based requests but follows the Fetch API standard.
Promise based HTTP client for the browser and node.js
![]() | ![]() | ![]() | ![]() | ![]() | ![]() |
---|---|---|---|---|---|
Latest ✔ | Latest ✔ | Latest ✔ | Latest ✔ | Latest ✔ | 8+ ✔ |
Using cdn:
<script src="https://npmcdn.com/axios/dist/axios.min.js"></script>
Using npm:
$ npm install axios
Using bower:
$ bower install axios
Performing a GET
request
// Make a request for a user with a given ID
axios.get('/user?ID=12345')
.then(function (response) {
console.log(response);
})
.catch(function (response) {
console.log(response);
});
// Optionally the request above could also be done as
axios.get('/user', {
params: {
ID: 12345
}
})
.then(function (response) {
console.log(response);
})
.catch(function (response) {
console.log(response);
});
Performing a POST
request
axios.post('/user', {
firstName: 'Fred',
lastName: 'Flintstone'
})
.then(function (response) {
console.log(response);
})
.catch(function (response) {
console.log(response);
});
Performing multiple concurrent requests
function getUserAccount() {
return axios.get('/user/12345');
}
function getUserPermissions() {
return axios.get('/user/12345/permissions');
}
axios.all([getUserAccount(), getUserPermissions()])
.then(axios.spread(function (acct, perms) {
// Both requests are now complete
}));
Requests can be made by passing the relevant config to axios
.
// Send a POST request
axios({
method: 'post',
url: '/user/12345',
data: {
firstName: 'Fred',
lastName: 'Flintstone'
}
});
// Send a GET request (default method)
axios('/user/12345');
For convenience aliases have been provided for all supported request methods.
When using the alias methods url
, method
, and data
properties don't need to be specified in config.
Helper functions for dealing with concurrent requests.
You can create a new instance of axios with a custom config.
var instance = axios.create({
baseURL: 'https://some-domain.com/api/',
timeout: 1000,
headers: {'X-Custom-Header': 'foobar'}
});
The available instance methods are listed below. The specified config will be merged with the instance config.
These are the available config options for making requests. Only the url
is required. Requests will default to GET
if method
is not specified.
{
// `url` is the server URL that will be used for the request
url: '/user',
// `method` is the request method to be used when making the request
method: 'get', // default
// `baseURL` will be prepended to `url` unless `url` is absolute.
// It can be convenient to set `baseURL` for an instance of axios to pass relative URLs
// to methods of that instance.
baseURL: 'https://some-domain.com/api/',
// `transformRequest` allows changes to the request data before it is sent to the server
// This is only applicable for request methods 'PUT', 'POST', and 'PATCH'
// The last function in the array must return a string, an ArrayBuffer, or a Stream
transformRequest: [function (data) {
// Do whatever you want to transform the data
return data;
}],
// `transformResponse` allows changes to the response data to be made before
// it is passed to then/catch
transformResponse: [function (data) {
// Do whatever you want to transform the data
return data;
}],
// `headers` are custom headers to be sent
headers: {'X-Requested-With': 'XMLHttpRequest'},
// `params` are the URL parameters to be sent with the request
params: {
ID: 12345
},
// `paramsSerializer` is an optional function in charge of serializing `params`
// (e.g. https://www.npmjs.com/package/qs, http://api.jquery.com/jquery.param/)
paramsSerializer: function(params) {
return Qs.stringify(params, {arrayFormat: 'brackets'})
},
// `data` is the data to be sent as the request body
// Only applicable for request methods 'PUT', 'POST', and 'PATCH'
// When no `transformRequest` is set, must be a string, an ArrayBuffer, a hash, or a Stream
data: {
firstName: 'Fred'
},
// `timeout` specifies the number of milliseconds before the request times out.
// If the request takes longer than `timeout`, the request will be aborted.
timeout: 1000,
// `withCredentials` indicates whether or not cross-site Access-Control requests
// should be made using credentials
withCredentials: false, // default
// `adapter` allows custom handling of requests which makes testing easier.
// Call `resolve` or `reject` and supply a valid response (see [response docs](#response-api)).
adapter: function (resolve, reject, config) {
/* ... */
},
// `auth` indicates that HTTP Basic auth should be used, and supplies credentials.
// This will set an `Authorization` header, overwriting any existing
// `Authorization` custom headers you have set using `headers`.
auth: {
username: 'janedoe',
password: 's00pers3cret'
}
// `responseType` indicates the type of data that the server will respond with
// options are 'arraybuffer', 'blob', 'document', 'json', 'text', 'stream'
responseType: 'json', // default
// `xsrfCookieName` is the name of the cookie to use as a value for xsrf token
xsrfCookieName: 'XSRF-TOKEN', // default
// `xsrfHeaderName` is the name of the http header that carries the xsrf token value
xsrfHeaderName: 'X-XSRF-TOKEN', // default
// `progress` allows handling of progress events for 'POST' and 'PUT uploads'
// as well as 'GET' downloads
progress: function (progressEvent) {
// Do whatever you want with the native progress event
},
// `maxContentLength` defines the max size of the http response content allowed
maxContentLength: 2000,
// `validateStatus` defines whether to resolve or reject the promise for a given
// HTTP response status code. If `validateStatus` returns `true` (or is set to `null`
// or `undefined`), the promise will be resolved; otherwise, the promise will be
// rejected.
validateStatus: function (status) {
return status >= 200 && status < 300; // default
}
}
The response for a request contains the following information.
{
// `data` is the response that was provided by the server
data: {},
// `status` is the HTTP status code from the server response
status: 200,
// `statusText` is the HTTP status message from the server response
statusText: 'OK',
// `headers` the headers that the server responded with
headers: {},
// `config` is the config that was provided to `axios` for the request
config: {}
}
When using then
or catch
, you will receive the response as follows:
axios.get('/user/12345')
.then(function(response) {
console.log(response.data);
console.log(response.status);
console.log(response.statusText);
console.log(response.headers);
console.log(response.config);
});
You can specify config defaults that will be applied to every request.
axios.defaults.baseURL = 'https://api.example.com';
axios.defaults.headers.common['Authorization'] = AUTH_TOKEN;
axios.defaults.headers.post['Content-Type'] = 'application/x-www-form-urlencoded';
// Set config defaults when creating the instance
var instance = axios.create({
baseURL: 'https://api.example.com'
});
// Alter defaults after instance has been created
instance.defaults.headers.common['Authorization'] = AUTH_TOKEN;
Config will be merged with an order of precedence. The order is library defaults found in lib/defaults.js
, then defaults
property of the instance, and finally config
argument for the request. The latter will take precedence over the former. Here's an example.
// Create an instance using the config defaults provided by the library
// At this point the timeout config value is `0` as is the default for the library
var instance = axios.create();
// Override timeout default for the library
// Now all requests will wait 2.5 seconds before timing out
instance.defaults.timeout = 2500;
// Override timeout for this request as it's known to take a long time
instance.get('/longRequest', {
timeout: 5000
});
You can intercept requests or responses before they are handled by then
or catch
.
// Add a request interceptor
axios.interceptors.request.use(function (config) {
// Do something before request is sent
return config;
}, function (error) {
// Do something with request error
return Promise.reject(error);
});
// Add a response interceptor
axios.interceptors.response.use(function (response) {
// Do something with response data
return response;
}, function (error) {
// Do something with response error
return Promise.reject(error);
});
If you may need to remove an interceptor later you can.
var myInterceptor = axios.interceptors.request.use(function () {/*...*/});
axios.interceptors.request.eject(myInterceptor);
You can add interceptors to a custom instance of axios.
var instance = axios.create();
instance.interceptors.request.use(function () {/*...*/});
axios.get('/user/12345')
.catch(function (response) {
if (response instanceof Error) {
// Something happened in setting up the request that triggered an Error
console.log('Error', response.message);
} else {
// The request was made, but the server responded with a status code
// that falls out of the range of 2xx
console.log(response.data);
console.log(response.status);
console.log(response.headers);
console.log(response.config);
}
});
You can define a custom HTTP status code error range using the validateStatus
config option.
axios.get('/user/12345', {
validateStatus: function (status) {
return status < 500; // Reject only if the status code is greater than or equal to 500
}
})
Until axios reaches a 1.0
release, breaking changes will be released with a new minor version. For example 0.5.1
, and 0.5.4
will have the same API, but 0.6.0
will have breaking changes.
axios depends on a native ES6 Promise implementation to be supported. If your environment doesn't support ES6 Promises, you can polyfill.
axios includes a TypeScript definition.
/// <reference path="axios.d.ts" />
import * as axios from 'axios';
axios.get('/user?ID=12345');
axios is heavily inspired by the $http service provided in Angular. Ultimately axios is an effort to provide a standalone $http
-like service for use outside of Angular.
MIT
FAQs
Promise based HTTP client for the browser and node.js
The npm package axios receives a total of 43,978,728 weekly downloads. As such, axios popularity was classified as popular.
We found that axios demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.