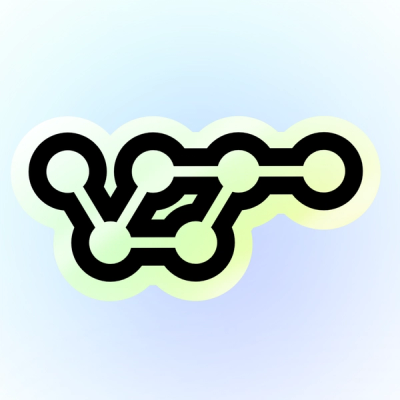
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Axios is a promise-based HTTP client for the browser and Node.js. It provides an easy-to-use API for sending asynchronous HTTP requests to REST endpoints and performing CRUD operations. It can be used to make XMLHttpRequests from the browser or HTTP requests from Node.js, supports the Promise API, and provides a way to intercept request and response, transform request and response data, and cancel requests.
Performing GET requests
This feature allows you to perform a GET request to retrieve data from a specified resource.
axios.get('/user?ID=12345').then(function (response) { console.log(response); }).catch(function (error) { console.log(error); });
Performing POST requests
This feature allows you to perform a POST request to send data to a server to create/update a resource.
axios.post('/user', { firstName: 'Fred', lastName: 'Flintstone' }).then(function (response) { console.log(response); }).catch(function (error) { console.log(error); });
Performing concurrent requests
This feature allows you to make multiple requests simultaneously.
axios.all([axios.get('/user/12345'), axios.get('/user/67890')]).then(axios.spread(function (acct, perms) { console.log(acct, perms); }));
Interceptors
This feature allows you to intercept requests or responses before they are handled by then or catch.
axios.interceptors.request.use(function (config) { config.headers.Authorization = AUTH_TOKEN; return config; }, function (error) { return Promise.reject(error); });
Creating instances
This feature allows you to create a new instance of axios with custom config defaults.
const instance = axios.create({ baseURL: 'https://api.example.com' }); instance.get('/users').then(function (response) { console.log(response); });
The Fetch API provides a JavaScript interface for accessing and manipulating parts of the HTTP pipeline, such as requests and responses. It is a modern alternative to XMLHttpRequest and is native to modern browsers. Unlike axios, it is not based on promises by default but can be used with promises.
Superagent is a light-weight progressive ajax API crafted for flexibility, readability, and a low learning curve after being frustrated with many of the existing request APIs. It is similar to axios but with a different API design and chainable methods.
Got is a human-friendly and powerful HTTP request library for Node.js. It is designed to be a simpler and more performant alternative to Node's native http module. Got offers stream support, promise APIs, and advanced features like retries and timeouts.
Request is a simplified HTTP request client 'simplified' with many features. It is one of the most popular HTTP client libraries but has been deprecated as of February 2020. It had a callback-based API, which is different from axios's promise-based API.
node-fetch is a light-weight module that brings the Fetch API to Node.js. It is an implementation of the window.fetch function for Node.js, aiming to provide a consistent API with the browser's fetch function. It is similar to axios in terms of promise-based requests but follows the Fetch API standard.
#axios
Promise based HTTP client for the browser and node.js
Using bower:
$ bower install axios
Using npm:
$ npm install axios
Tested to work with >=IE8, Chrome, Firefox, Safari, and Opera.
Performing a GET
request
// Make a request for a user with a given ID
axios.get('/user?ID=12345')
.then(function (response) {
console.log(response);
})
.catch(function (response) {
console.log(response);
});
// Optionally the request above could also be done as
axios.get('/user', {
params: {
ID: 12345
}
})
.then(function (response) {
console.log(response);
})
.catch(function (response) {
console.log(response);
});
Performing a POST
request
axios.post('/user', {
firstName: 'Fred',
lastName: 'Flintstone'
})
.then(function (response) {
console.log(response);
})
.catch(function (response) {
console.log(response);
});
Performing multiple concurrent requests
function getUserAccount() {
return axios.get('/user/12345');
}
function getUserPermissions() {
return axios.get('/user/12345/permissions');
}
axios.all([getUserAccount(), getUserPermissions()])
.then(axios.spread(function (acct, perms) {
// Both requests are now complete
}));
Requests can be made by passing the relevant config to axios
.
axios({
method: 'get',
url: '/user/12345'
});
For convenience aliases have been provided for all supported request methods.
When using the alias methods url
, method
, and data
properties don't need to be specified in config.
Helper functions for dealing with concurrent requests.
This is the available config options for making requests. Only the url
is required. Requests will default to GET
if method
is not specified.
{
// `url` is the server URL that will be used for the request
url: '/user',
// `method` is the request method to be used when making the request
method: 'get', // default
// `transformRequest` allows changes to the request data before it is sent to the server
// This is only applicable for request methods 'PUT', 'POST', and 'PATCH'
// The last function in the array must return a string or an ArrayBuffer
transformRequest: [function (data) {
// Do whatever you want to transform the data
return data;
}],
// `transformResponse` allows changes to the response data to be made before
// it is passed to then/catch
transformResponse: [function (data) {
// Do whatever you want to transform the data
return data;
}],
// `headers` are custom headers to be sent
headers: {'X-Requested-With': 'XMLHttpRequest'},
// `param` are the URL parameters to be sent with the request
params: {
ID: 12345
},
// `data` is the data to be sent as the request body
// Only applicable for request methods 'PUT', 'POST', and 'PATCH'
// When no `transformRequest` is set, must be a string, an ArrayBuffer or a hash
data: {
firstName: 'Fred'
},
// `withCredentials` indicates whether or not cross-site Access-Control requests
// should be made using credentials
withCredentials: false, // default
// `responseType` indicates the type of data that the server will respond with
// options are 'arraybuffer', 'blob', 'document', 'json', 'text'
responseType: 'json', // default
// `xsrfCookieName` is the name of the cookie to use as a value for xsrf token
xsrfCookieName: 'XSRF-TOKEN', // default
// `xsrfHeaderName` is the name of the http header that carries the xsrf token value
xsrfHeaderName: 'X-XSRF-TOKEN' // default
}
The response for a request contains the following information.
{
// `data` is the response that was provided by the server
data: {},
// `status` is the HTTP status code from the server response
status: 200,
// `headers` the headers that the server responded with
headers: {},
// `config` is the config that was provided to `axios` for the request
config: {}
}
When using then
or catch
, you will receive the response as follows:
axios.get('/user/12345')
.then(function(response) {
console.log(response.data);
console.log(response.status);
console.log(response.headers);
console.log(response.config);
});
You can intercept requests or responses before they are handled by then
or catch
.
// Add a request interceptor
axios.interceptors.request.use(function (config) {
// Do something before request is sent
return config;
}, function (error) {
// Do something with request error
return Promise.reject(error);
});
// Add a response interceptor
axios.interceptors.response.use(function (response) {
// Do something with response data
return response;
}, function (error) {
// Do something with response error
return Promise.reject(error);
});
If you may need to remove an interceptor later you can.
var myInterceptor = axios.interceptors.request.use(function () {/*...*/});
axios.interceptors.request.eject(myInterceptor);
axios.get('/user/12345')
.catch(function (response) {
if (response instanceof Error) {
// Something happened in setting up the request that triggered an Error
console.log('Error', response.message);
} else {
// The request was made, but the server responded with a status code
// that falls out of the range of 2xx
console.log(response.data);
console.log(response.status);
console.log(response.headers);
console.log(response.config);
}
});
axios includes a TypeScript definition.
/// <reference path="axios.d.ts" />
import axios = require('axios');
axios.get('/user?ID=12345');
axios is heavily inspired by the $http service provided in Angular. Ultimately axios is an effort to provide a standalone $http
-like service for use outside of Angular.
axios uses the es6-promise polyfill by Jake Archibald. Until we can use ES6 Promises natively in all browsers, this polyfill is a life saver.
MIT
FAQs
Promise based HTTP client for the browser and node.js
The npm package axios receives a total of 37,137,984 weekly downloads. As such, axios popularity was classified as popular.
We found that axios demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.