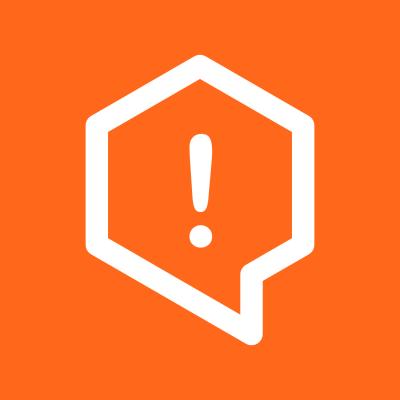
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
azure-arm-compute
Advanced tools
ComputeManagementClient Library with typescript type definitions for node
This project provides a Node.js package that makes it easy to manage Microsoft Azure Compute Resources. Right now it supports:
npm install azure-arm-compute
var msRestAzure = require('ms-rest-azure');
var computeManagementClient = require('azure-arm-compute');
// Interactive Login
// It provides a url and code that needs to be copied and pasted in a browser and authenticated over there. If successful,
// the user will get a DeviceTokenCredentials object.
msRestAzure.interactiveLogin(function(err, credentials) {
var client = new computeManagementClient(credentials, 'your-subscription-id');
client.virtualMachineImages.list('westus', 'MicrosoftWindowsServer', 'WindowsServer', '2012-R2-Datacenter', function(err, result, request, response) {
if (err) console.log(err);
console.log(result);
});
});
FAQs
ComputeManagementClient Library with typescript type definitions for node
The npm package azure-arm-compute receives a total of 10,596 weekly downloads. As such, azure-arm-compute popularity was classified as popular.
We found that azure-arm-compute demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.