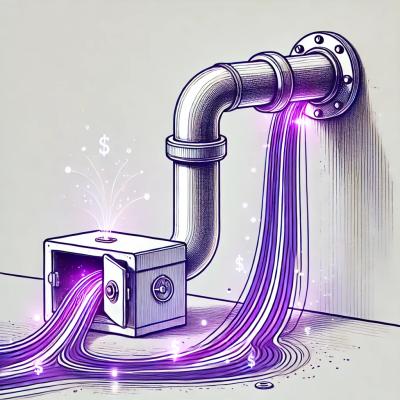
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
azure-storage-fs
Advanced tools
A drop-in "fs" replacement for accessing Azure Storage with Node.js "fs" API
A drop-in "fs" replacement for accessing Azure Storage with Node.js "fs" API.
This package is designed to support ftpd.
azure-storage-fs
is designed to replace Node.js "fs" package and integrate with ftpd
package.
const fs = require('azure-storage-fs').blob(accountName, secret, container);
fs.readFile('helloworld.txt', (err, data) => {
console.log(err || data);
});
If you want to bring your own BlobService
and prefer a Promise interface like mz/fs
.
const { createBlobService } = require('azure-storage');
const fs = require('azure-storage-fs').blob(createBlobService(), container).promise;
const data = await fs.readFile('helloworld.txt');
console.log(data);
ftpd
supports custom "fs" implementation and azure-storage-fs
is designed to be a "fs" provider for ftpd
.
To use a custom "fs" implementation, in your ftpd
authorization code (command:pass
event), add require('azure-storage-fs').blob(accountName, secret, container)
to the success
callback. For example,
connection.on('command:pass', (password, success, failure) => {
if (auth(username, password)) {
success(username, require('azure-storage-fs').blob(accountName, secret, container));
} else {
failure();
}
});
ftpd
will call fs.readdir()
first, then fs.stat()
for every file
fs.stat()
on a directory will result in 2 calls to AzurePaths will be normalized with the following rules:
For example, \Users\Documents\HelloWorld.txt
will become Users/Documents/HelloWorld.txt
.
Since Blob service is flat natively, it does not support directory tree. It use delimiter /
to provides a hierarchical view of its flat structure. A hidden empty blob named $$$.$$$
is used to represent empty directory.
Only block blob is supported and is the default blob type when creating a new blob.
createReadStream
encoding
is not supportedsnapshot
options for specifying ID of the snapshot to readcreateWriteStream
encoding
is not supportedmetadata
option to specify blob metadatamkdir
EEXIST
if the directory already exists$$$.$$$
open
r
, w
, and wx
snapshot
options for specifying ID of the snapshot to openreaddir
readFile
createReadStream
encoding
is not supportedsnapshot
options for specifying ID of the snapshot to readrename
rmdir
$$$.$$$
if existsENOTEMPTY
if notsas(path, options)
(New)
flag
(optional)
r
, a
, c
, w
, d
start
(optional)
expiry
(optional)
setMetadata(metadata, options)
(New)
snapshot
(optional)
snapshot(path, options)
(New)
snapshot
(optional)
stat(path, options)
isDirectory()
mode
always equals to R_OK | W_OK
mtime
size
0
for directoryurl
for the actual URL (not support Shared Access Signature yet)metadata
(default set to false
)
true
, the call will also return metadatasnapshot
options, the call will also return metadata for snapshotssnapshot
(default set to false
)
true
, the call will also return an array named snapshots
, each with the following properties
id
is the snapshot IDmtime
size
url
unlink(pathname, options)
snapshot
(default set to true
)
true
will delete all snapshots associated with the blobwriteFile
createWriteStream
encoding
is not supportedmetadata
option to specify blob metadataSnapshot is supported and snapshot ID can be specified for most read APIs. stat
can also set to return snapshot information. By default this is disabled to save transaction cost.
In future, we plan to support Azure Storage File, which is another file storage service accessible thru HTTP interface and SMB on Azure.
BlobService
contentSettings
supportcontentSettings
supportcontentSettings
supportmetadata
supportmetadata
supportmetadata
supportmetadata
supportEEXIST
when the directory already existsENOTEMPTY
when the directory still contains one or more blobs after deleting $$$.$$$
placeholderexpiry
optionflag
to r
if not setsas()
to create a blob SAS token synchronouslysnapshot()
to create a blob snapshotrmdir
now only delete hidden blob $$$.$$$
, instead of delete blobs recursivelyunlink
now fail if the blob does not existLike us? Star us.
Doesn't work as expected? File us an issue with minimal code for bug repro.
[0.4.0] - 2017-12-07
BlobService
FAQs
A drop-in "fs" replacement for accessing Azure Storage with Node.js "fs" API
We found that azure-storage-fs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.