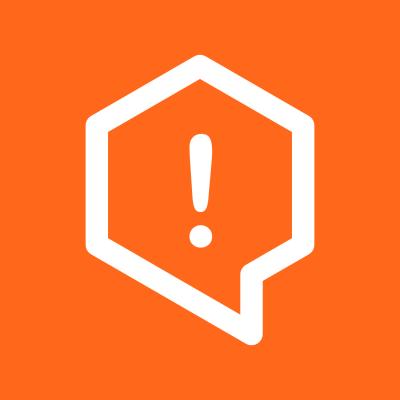
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
azure-translator-code
Advanced tools
Azure Cognitive Services Translator Text API Code for Use with Common Languages
Azure Translator Code is a powerful library for translating JSON files into multiple languages using the Azure Cognitive Translator service. This library supports translating JSON files located in multiple folders or within a single folder, depending on your needs.
Install the library using npm or yarn:
npm install -D azure-translator-code
or
yarn add -D azure-translator-code
npm install azure-translator-code
or
yarn add azure-translator-code
You can import the JSON file you want to translate in two ways:
const jsonFile = require("./jsonFileToTranslate/en.json");
// or
const jsonFile = {
HomePage: {
welcome: "Welcome",
hello: "Hello",
SubText: {
subText: "This is a subtext",
},
},
};
After importing the JSON file, you can use the library to translate it:
const {
translate,
translateText,
translateToMultipleFolders,
translateToUnicFolder,
updateTranslationsMulti,
updateTranslationsUnic,
} = require("azure-translator-code");
// or
import {
translate,
translateText,
translateToMultipleFolders,
translateToUnicFolder,
updateTranslationsMulti,
updateTranslationsUnic,
} from "azure-translator-code";
import type { TranslationType } from "azure-translator-code";
Define your Azure API details and languages:
const key = "YOUR_AZURE_KEY"; // Replace with your Azure API key
const endpoint = "https://api.cognitive.microsofttranslator.com/";
const location = "eastus";
const fromLang = "en";
const toLangs = [
"pt",
"de",
"es",
"fr",
"it",
"ja",
"ko",
"nl",
"ru",
"zh",
"pt-pt",
"ar",
"tlh-Latn",
];
const jsonFile = {
translation: {
welcome: "Welcome",
hello: "Hello",
},
};
// Translate to multiple folders
translateToMultipleFolders(key, endpoint, location, fromLang, toLangs, jsonFile);
// This will create a folder called multiFolderGeneratedTranslations
// Translate to a single folder
translateToUnicFolder(key, endpoint, location, fromLang, toLangs, jsonFile);
// This will create a folder called unicFolderGeneratedTranslations
// Update translations in multiple folders
updateTranslationsMulti(key, endpoint, location, fromLang, toLangs, jsonFile);
// Only new keys will be translated in the multiFolderGeneratedTranslations folder
// Update translations in a single folder
updateTranslationsUnic(key, endpoint, location, fromLang, toLangs, jsonFile);
// Only new keys will be translated in the unicFolderGeneratedTranslations folder
You can specify the folder name or location where translations will be saved. Saving always starts from the project root folder.
const key = "YOUR_AZURE_KEY";
const endpoint = "https://api.cognitive.microsofttranslator.com/";
const location = "eastus";
const fromLang = "en";
const toLangs = ["pt", "de"];
const jsonFile = {
translation: {
welcome: "Welcome",
hello: "Hello",
},
};
// Save translations in custom folder
translateToMultipleFolders(
key,
endpoint,
location,
fromLang,
toLangs,
jsonFile,
"myFolder",
);
// This will create a folder called myFolder
translateToUnicFolder(
key,
endpoint,
location,
fromLang,
toLangs,
jsonFile,
"./myFolder/OtherFolder/etc",
);
// This will create a folder at ./myFolder/OtherFolder/etc
// If you just want to update the file for new keys, use the update functions to avoid unnecessary requests.
You can also log the translation results to the console for verification.
const { translate, translateText } = require("azure-translator-code");
const key = "YOUR_AZURE_KEY";
const endpoint = "https://api.cognitive.microsofttranslator.com/";
const location = "eastus";
const fromLang = "en";
const toLangs = ["pt"];
const jsonFile = {
HomePage: {
Welcome: "Welcome",
Hello: "Hello",
},
};
translate(key, endpoint, location, fromLang, toLangs, jsonFile).then((res) => {
console.log(res);
});
// Output
/**
{
HomePage: {
Welcome: "Bem-vindo",
Hello: "Olá",
},
};
*/
// or
translateText("Hello World!", fromLang, toLangs, endpoint, key, location).then(
(res) => {
console.log(res[0].translations);
},
);
// Output -> [{ text: "Olá, mundo!", to: "pt" }]
Make sure to replace the key
, location
and endpoint
with your actual Azure access credentials.
If you would like to contribute to this project, feel free to open an issue or submit a pull request on our GitHub repository.
FAQs
Azure Cognitive Services Translator Text API Code for Use with Common Languages
The npm package azure-translator-code receives a total of 18 weekly downloads. As such, azure-translator-code popularity was classified as not popular.
We found that azure-translator-code demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.