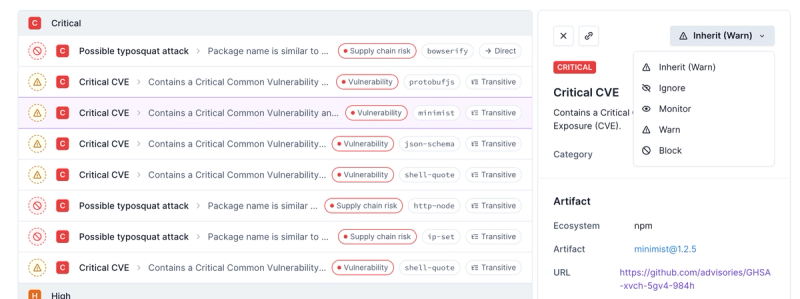
Product
Introducing Enhanced Alert Actions and Triage Functionality
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
babel-plugin-ember-template-compilation
Advanced tools
Package description
The babel-plugin-ember-template-compilation package is a Babel plugin designed to precompile Ember.js templates. This plugin allows developers to write templates in a more concise and readable format, which are then compiled into JavaScript functions that can be used within Ember applications.
Precompiling Templates
This feature allows you to precompile Ember.js templates using the Ember template compiler. The precompiled templates are then transformed into JavaScript functions that can be used within your Ember application.
module.exports = function (babel) {
return {
plugins: [
['babel-plugin-ember-template-compilation', {
precompile: require('ember-source/dist/ember-template-compiler').precompile
}]
]
};
};
Custom Template Compiler
This feature allows you to use a custom template compiler instead of the default Ember template compiler. This can be useful if you have specific requirements for how your templates should be compiled.
module.exports = function (babel) {
return {
plugins: [
['babel-plugin-ember-template-compilation', {
precompile: customTemplateCompiler
}]
]
};
};
function customTemplateCompiler(templateString) {
// Custom compilation logic
return compiledTemplate;
}
The ember-cli-htmlbars package is an Ember CLI addon that provides a preprocessor for compiling Handlebars templates in Ember.js applications. It is similar to babel-plugin-ember-template-compilation in that it precompiles templates, but it is specifically designed to work with Ember CLI and integrates more tightly with the Ember build process.
The babel-plugin-htmlbars-inline-precompile package is a Babel plugin that allows you to precompile Handlebars templates inline within JavaScript files. It is similar to babel-plugin-ember-template-compilation in that it precompiles templates, but it is designed to be used directly within JavaScript files rather than as part of a broader build process.
Readme
Babel plugin that implements Ember's low-level template-compilation API.
This plugin implements precompileTemplate
from RFC 496:
import { precompileTemplate } from '@ember/template-compilation';
For backward compatibility, it also has an enableLegacyModules
option that can enable each of these widely-used older patterns:
import { hbs } from 'ember-cli-htmlbars';
import hbs from 'ember-cli-htmlbars-inline-precompile';
import hbs from 'htmlbars-inline-precompile';
This package has both a Node implementation and a portable implementation that works in browsers.
The exported modules are:
babel-plugin-ember-template-compilation
: automatically chooses between the node and browser implementations (using package.json exports
feature).babel-plugin-ember-template-compilation/browser
: the core implementation that works in browsers (and anywhere else) without using any Node-specific APIs.babel-plugin-ember-template-compilation/node
: the Node-specific implementation that adds the ability to automatically load plugins from disk, etc.The options are:
interface Options {
// The ember-template-compiler.js module that ships within your ember-source version. In the browser implementation, this is mandatory. In the Node implementation you can use compilerPath instead.
compiler?: EmberTemplateCompiler;
// The on-disk path to the ember-template-compiler.js module for our current
// ember version. You need to either set `compilerPath` or set `compiler`.
// This will get resolved from the current working directory, so a package name
// like "ember-source/dist/ember-template-compiler" is acceptable.
compilerPath?: string;
// Allows you to remap what imports will be emitted in our compiled output. By
// example:
//
// outputModuleOverrides: {
// '@ember/template-factory': {
// createTemplateFactory: ['createTemplateFactory', '@glimmer/core'],
// }
// }
//
// Normal Ember apps shouldn't need this, it exists to support other
// environments like standalone GlimmerJS
outputModuleOverrides?: Record<string, Record<string, [string, string]>>;
// By default, this plugin implements only Ember's stable public API for
// template compilation, which is:
//
// import { precompileTemplate } from '@ember/template-compilation';
//
// But historically there are several other importable syntaxes in widespread
// use, and we can enable those too by including their module names in this
// list. See `type LegacyModuleName` below.
enableLegacyModules?: LegacyModuleName[];
// Controls the output format.
//
// "wire": The default. In the output, your templates are ready to execute in
// the most performant way.
//
// "hbs": In the output, your templates will still be in HBS format.
// Generally this means they will still need further processing before
// they're ready to execute. The purpose of this mode is to support things
// like codemods and pre-publication transformations in libraries.
targetFormat?: 'wire' | 'hbs';
// Optional list of custom transforms to apply to the handlebars AST before
// compilation. See `type Transform` below.
transforms?: Transform[];
}
// The legal legacy module names. These are the only ones that are supported,
// because these are the ones in widespread community use. We don't want people
// creating new ones -- prefer `@ember/template-compilation` in new code.
type LegacyModuleName =
| 'ember-cli-htmlbars'
| 'ember-cli-htmlbars-inline-precompile'
| 'htmlbars-inline-precompile';
// Each transform can be
// - the actual AST transform function (this is the only kind that works in non-Node environments)
// - a path to a module where we will find the AST transform function as the default export
// - an array of length two containing the path to a module and an arguments object.
// In this case we will pass the arguments to the default export from the module and
// it should return the actual AST transform function.
// All the path resolving happens relative to the current working directory and
// respects node_modules resolution.
type Transform = Function | string | [string, unknown];
AST transforms are plugins for modifying HBS templates at build time. Because those templates are embedded in Javascript and can access the Javascript scope, an AST plugin may want to introduce some new things into Javascript scope. That is what the JSUtils API is for.
Your AST transform can access the JSUtils API via env.meta.jsutils
. Here's an example transform.
function myAstTransform(env) {
return {
name: 'my-ast-transform',
visitor: {
PathExpression(node, path) {
if (node.original === 'onePlusOne') {
let name = env.meta.jsutils.bindExpression('1+1', path, { nameHint: 'two' });
return env.syntax.builders.path(name);
}
},
},
};
}
The example transform above would rewrite:
import { precompileTemplate } from '@ember/template-compilation';
precompileTemplate('<Counter @value={{onePlusOne}} />>');
To:
import { precompileTemplate } from '@ember/template-compilation';
let two = 1 + 1;
precompileTemplate('<Counter @value={{two}} />', { scope: () => ({ two }) });
See the jsdoc comments in js-utils.js for details on the methods available.
This repo derives from https://github.com/ember-cli/babel-plugin-htmlbars-inline-precompile
FAQs
Babel implementation of Ember's low-level template-compilation API
The npm package babel-plugin-ember-template-compilation receives a total of 121,947 weekly downloads. As such, babel-plugin-ember-template-compilation popularity was classified as popular.
We found that babel-plugin-ember-template-compilation demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 17 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports four distinct alert actions instead of the previous two, and alert triaging allows users to override the actions taken for all individual alerts.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.