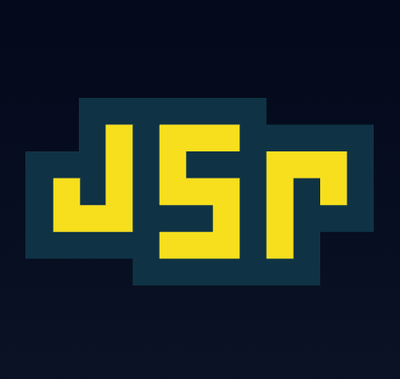
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
babel-plugin-react-docgen
Advanced tools
The babel-plugin-react-docgen npm package is a Babel plugin that extracts type and prop information from React components. It uses the react-docgen library to parse and collect documentation from React component files, which can then be used to generate documentation or for other purposes in the development workflow.
Extracting prop types information
This feature allows you to automatically extract prop types information from your React components. The plugin will parse the propTypes definition and attach it to the component object, which can then be used for documentation purposes.
import React from 'react';
import PropTypes from 'prop-types';
class MyComponent extends React.Component {
static propTypes = {
name: PropTypes.string.isRequired,
age: PropTypes.number
};
render() {
return <div>{this.props.name}</div>;
}
}
Extracting default prop values
This feature extracts the default values for props defined in a React component. The plugin will parse the defaultProps definition and attach it to the component object, which can be used to enhance the generated documentation with default values for each prop.
import React from 'react';
import PropTypes from 'prop-types';
class MyComponent extends React.Component {
static propTypes = {
name: PropTypes.string
};
static defaultProps = {
name: 'Default Name'
};
render() {
return <div>{this.props.name}</div>;
}
}
Extracting component description
This feature extracts the description of the component which is provided in the comment block above the component declaration. This description can then be included in the generated documentation to provide more context about the component's purpose and usage.
/**
* This is MyComponent.
* It is an example component.
*/
import React from 'react';
class MyComponent extends React.Component {
render() {
return <div>Hello, World!</div>;
}
}
react-docgen is the underlying library used by babel-plugin-react-docgen. It parses React component files to extract prop types and descriptions. While babel-plugin-react-docgen integrates with Babel to perform this extraction during the build process, react-docgen can be used standalone or integrated into other build tools and scripts.
prop-types-docs is another tool that can be used to generate documentation from prop types. It differs from babel-plugin-react-docgen in that it is not a Babel plugin and may require separate integration into the build process. It provides a CLI to generate markdown documentation from prop types.
storybook-addon-react-docgen is an addon for Storybook that uses react-docgen to extract and display prop types information within Storybook stories. It enhances the Storybook experience by providing prop tables and documentation automatically. It is similar to babel-plugin-react-docgen in that it uses react-docgen, but it is specifically tailored for use with Storybook.
react-docgen allows you to write propType descriptions, class descriptions and access propType metadata programatically.
This babel plugin allow you to access those information right inside your React class.
For an example, let's say you've a React class like this:
/**
This is an awesome looking button for React.
*/
import React from 'react';
export default class Button extends React.Component {
render() {
const { label, onClick } = this.props;
return (
<button onClick={onClick}>{ label }</button>
);
}
}
Button.propTypes = {
/**
Label for the button.
*/
label: React.PropTypes.string,
/**
Triggered when clicked on the button.
*/
onClick: React.PropTypes.func,
};
With this babel plugin, you can access all these information right inside your app with:
console.log(Button.__docgenInfo);
{
description: 'This is an awesome looking button for React.',
props: {
label: {
type: {
name: 'string'
},
required: false,
description: 'Label for the button.'
},
onClick: {
type: {
name: 'func'
},
required: false,
description: 'Triggered when clicked on the button.'
}
}
}
This will be pretty useful for documentations and some other React devtools like Storybook.
Install the plugin:
npm install -D babel-plugin-react-docgen
Use it inside your .babelrc
{
"plugins": ["react-docgen"]
}
option | description | default |
---|---|---|
resolver | react-docgen has 3 built in resolvers which may be used. Resolvers define how/what the doc generator will inspect. You may inspect the existing resolvers in react-docgen/tree/master/src/resolver. | "findAllExportedComponentDefinition" |
removeMethods | optionally remove docgen information about methods | false |
Sometimes, it's a pretty good idea to collect all of the docgen info into a collection. Then you could use that to render style guide or similar.
So, we allow you to collect all the docgen info into a global collection. To do that, add following config to when loading this babel plugin:
{
"plugins":[
[
"babel-plugin-react-docgen",
{
"DOC_GEN_COLLECTION_NAME": "MY_REACT_DOCS",
"resolver": "findAllComponentDefinitions", // optional (default: findAllComponentDefinitions)
"removeMethods": true // optional (default: false)
}
]
]
}
Then you need to create a global variable(an object) in your app called MY_REACT_DOCS
before any code get's executed.
Then we'll save them into that object. We do it by adding a code block like this to the transpiled file:
if (typeof MY_REACT_DOCS !== 'undefined') {
MY_REACT_DOCS['test/fixtures/case4/actual.js'] = {
name: 'Button',
docgenInfo: Button.__docgenInfo,
path: 'path/to/my/button.js'
};
}
Now, we parse your code with react-docgen
to get these info.
But we only do it for files which has a React component.
Yes, this will add some overhead to your project. But once you turned on babel cache directory this won't be a big issue.
Yes this increase the output size of your transpiled files. The size increase varies depending on various factors like:
Most of the time, you need this plugin when you are developing your app or with another tool like Storybook. So, you may not need to use this on the production version of your app.
v2.0.1
29-01-2019
react-docgen@^3.0.0
FAQs
Babel plugin to add react-docgen info into your code
The npm package babel-plugin-react-docgen receives a total of 819,246 weekly downloads. As such, babel-plugin-react-docgen popularity was classified as popular.
We found that babel-plugin-react-docgen demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.