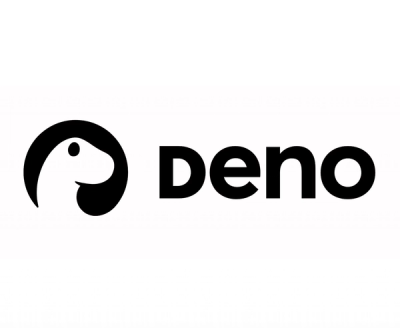
Security News
Deno 2.4 Brings Back deno bundle, Improves Dependency Management and Observability
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
babel-plugin-transform-react-jsx
Advanced tools
The babel-plugin-transform-react-jsx package is a Babel plugin that transforms JSX syntax into JavaScript. This allows developers to write HTML-like syntax in their JavaScript code, which is then converted to React.createElement calls.
Transform JSX to React.createElement
This feature allows you to write JSX syntax, which is more readable and expressive, and have it automatically transformed into React.createElement calls, which is what React uses under the hood.
const element = <h1>Hello, world!</h1>;
// Transforms to:
const element = React.createElement('h1', null, 'Hello, world!');
Custom JSX Pragma
This feature allows you to specify a custom function to be used instead of React.createElement. This can be useful if you are using a different library that uses JSX syntax.
/* @jsx customCreateElement */
const element = <h1>Hello, world!</h1>;
// Transforms to:
const element = customCreateElement('h1', null, 'Hello, world!');
Automatic Fragment Support
This feature allows you to use the shorthand syntax for React fragments, which are used to group multiple elements without adding extra nodes to the DOM.
const element = <><h1>Hello, world!</h1><p>This is a paragraph.</p></>;
// Transforms to:
const element = React.createElement(React.Fragment, null, React.createElement('h1', null, 'Hello, world!'), React.createElement('p', null, 'This is a paragraph.'));
babel-plugin-jsx is a Babel plugin that also transforms JSX syntax into JavaScript. It is similar to babel-plugin-transform-react-jsx but offers more flexibility in terms of customization and supports multiple JSX libraries.
babel-plugin-inferno is a Babel plugin specifically designed for the Inferno library, which is a fast, React-like library for building user interfaces. It transforms JSX syntax into Inferno.createElement calls.
Turn JSX into React function calls
In
var profile = <div>
<img src="avatar.png" className="profile" />
<h3>{[user.firstName, user.lastName].join(' ')}</h3>
</div>;
Out
var profile = React.createElement("div", null,
React.createElement("img", { src: "avatar.png", className: "profile" }),
React.createElement("h3", null, [user.firstName, user.lastName].join(" "))
);
In
/** @jsx dom */
var { dom } = require("deku");
var profile = <div>
<img src="avatar.png" className="profile" />
<h3>{[user.firstName, user.lastName].join(' ')}</h3>
</div>;
Out
/** @jsx dom */
var dom = require("deku").dom;
var profile = dom( "div", null,
dom("img", { src: "avatar.png", className: "profile" }),
dom("h3", null, [user.firstName, user.lastName].join(" "))
);
npm install --save-dev babel-plugin-transform-react-jsx
.babelrc
(Recommended).babelrc
Without options:
{
"plugins": ["transform-react-jsx"]
}
With options:
{
"plugins": [
["transform-react-jsx", {
"pragma": "dom" // default pragma is React.createElement
}]
]
}
babel --plugins transform-react-jsx script.js
require("babel-core").transform("code", {
plugins: ["transform-react-jsx"]
});
pragma
string
, defaults to React.createElement
.
Replace the function used when compiling JSX expressions.
Note that the @jsx React.DOM
pragma has been deprecated as of React v0.12
useBuiltIns
boolean
, defaults to false
.
When spreading props, use Object.assign
directly instead of Babel's extend helper.
FAQs
Turn JSX into React function calls
The npm package babel-plugin-transform-react-jsx receives a total of 998,539 weekly downloads. As such, babel-plugin-transform-react-jsx popularity was classified as popular.
We found that babel-plugin-transform-react-jsx demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.