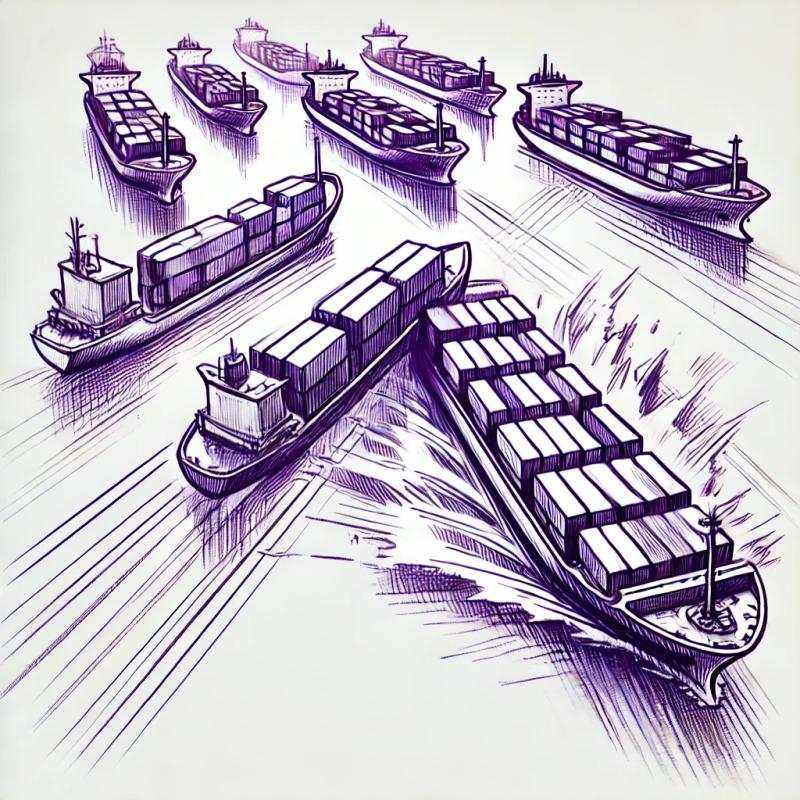
Security News
Namecheap Takes Down Polyfill.io Service Following Supply Chain Attack
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
babel-plugin-transform-remove-debugger
Advanced tools
Package description
The babel-plugin-transform-remove-debugger npm package is a Babel plugin that removes all debugger statements from your JavaScript code. This is particularly useful for production builds where you want to ensure that no debugging code is left in the final output.
Remove debugger statements
This feature removes all debugger statements from the input JavaScript code. In the provided code sample, the debugger statement inside the test function is removed, leaving only the console.log statement.
const code = `function test() { debugger; console.log('test'); }`;
const output = Babel.transform(code, { plugins: ['transform-remove-debugger'] }).code;
console.log(output); // function test() { console.log('test'); }
The babel-plugin-transform-remove-console package removes all console.* statements from your JavaScript code. This is similar to babel-plugin-transform-remove-debugger but focuses on removing console statements instead of debugger statements.
The babel-plugin-minify-dead-code-elimination package removes dead code from your JavaScript files. While it does not specifically target debugger statements, it can remove unreachable code, which may include debugger statements if they are part of dead code.
The babel-plugin-transform-remove-undefined package removes undefined variables and expressions from your JavaScript code. This is another form of code cleanup, similar to removing debugger statements, but it focuses on undefined values.
Readme
This plugin removes all debugger;
statements.
In
debugger;
Out
npm install babel-plugin-transform-remove-debugger --save-dev
.babelrc
(Recommended).babelrc
{
"plugins": ["transform-remove-debugger"]
}
babel --plugins transform-remove-debugger script.js
require("@babel/core").transform("code", {
plugins: ["transform-remove-debugger"]
});
FAQs
Remove debugger statements
We found that babel-plugin-transform-remove-debugger demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.
Security News
A JavaScript library maintainer is under fire after merging a controversial PR to support legacy versions of Node.js.